QCA
Taking a hint from the similarly-named Java Cryptography Architecture, QCA aims to provide a straightforward and cross-platform cryptographic API, using Qt datatypes and conventions. QCA separates the API from the implementation, using plugins known as Providers. The advantage of this model is to allow applications to avoid linking to or explicitly depending on any particular cryptographic library. This allows one to easily change or upgrade Provider implementations without even needing to recompile the application!
QCA should work everywhere Qt does, including Windows/Unix/MacOSX. This version of QCA is for Qt4 or Qt5, and requires no Qt3 compatibility code.
Features
This library provides an easy API for the following features:
- Secure byte arrays (QCA::SecureArray)
- Arbitrary precision integers (QCA::BigInteger)
- Random number generation (QCA::Random)
- SSL/TLS (QCA::TLS)
- X509 certificates (QCA::Certificate and QCA::CertificateCollection)
- X509 certificate revocation lists (QCA::CRL)
- Built-in support for operating system certificate root storage (QCA::systemStore)
- Simple Authentication and Security Layer (SASL) (QCA::SASL)
- Cryptographic Message Syntax (e.g., for S/MIME) (QCA::CMS)
- PGP messages (QCA::OpenPGP)
- Unified PGP/CMS API (QCA::SecureMessage)
- Subsystem for managing Smart Cards and PGP keyrings (QCA::KeyStore)
- Simple but flexible logging system (QCA::Logger)
- RSA (QCA::RSAPrivateKey and QCA::RSAPublicKey)
- DSA (QCA::DSAPrivateKey and QCA::DSAPublicKey)
- Diffie-Hellman (QCA::DHPrivateKey and QCA::DHPublicKey)
- Hashing (QCA::Hash) with
- SHA-0
- SHA-1
- MD2
- MD4
- MD5
- RIPEMD160
- SHA-224
- SHA-256
- SHA-384
- SHA-512
- Whirlpool
- Ciphers (QCA::Cipher) using
- BlowFish
- Triple DES
- DES
- AES (128, 192 and 256 bit)
- CAST5 (also known as CAST-128)
- Message Authentication Code (QCA::MessageAuthenticationCode), using
- HMAC with SHA-1
- HMAC with MD5
- HMAC with RIPEMD160
- HMAC with SHA-224
- HMAC with SHA-256
- HMAC with SHA-384
- HMAC with SHA-512
- Encoding and decoding of hexadecimal (QCA::Hex) and Base64 (QCA::Base64) strings.
Functionality is supplied via plugins. This is useful for avoiding dependence on a particular crypto library and makes upgrading easier, as there is no need to recompile your application when adding or upgrading a crypto plugin. Also, by pushing crypto functionality into plugins, your application is free of legal issues, such as export regulation.
And of course, you get a very simple crypto API for Qt, where you can do things like:
Using QCA
The application simply includes <QtCrypto> and links to libqca, which provides the 'wrapper API' and plugin loader. Crypto functionality is determined during runtime, and plugins are loaded from the 'crypto' subfolder of the Qt library paths. There are additional examples available.
Introduction
Using QCA is much like using Qt, and if you are familiar with Qt, then it should feel "natural". There are a few things you do need to know though, to build reliable applications:
- QCA needs to be initialized before you use any class that requires plugin support, or uses secure memory. That is most of QCA, so you should assume that you need to perform initialization. The easiest way to do this is to instantiate a QCA::Initializer object and ensure it is not deleted (or allowed to go out of scope) until you have finished using QCA.
- Most features/algorithms are provided by plugins/Providers. You should check that the required feature is actually available (using QCA::isSupported()) before trying to create it. If you try to create a class and suitable provider support is not available, you will get back a null object, and when you try to use one of the methods, your application will segfault. Also, for features that take algorithm names (e.g. QCA::Hash, which takes the name of the hashing algorithm such as "md5" or "sha256"), the name is looked up at run-time, so if you make a typographical error (e.g. "md56") it will compile correctly, but segfault at run-time.
Thoughts on security
QCA tries to be flexible in what it supports. That does not mean that every possible combination of features makes sense though.
We strongly recommend against coming up with your own design made up of low-level cryptographic primitives (e.g. QCA::Hash, QCA::Cipher and similar features) and trying to use higher level capabilities. In particular, we recommend looking at QCA::TLS, QCA::SASL, QCA::CMS and QCA::OpenPGP as starting points.
When selecting a particular cryptographic feature, you should make sure that you understand what sort of threats your application is likely to be exposed to, and how that threat can be effectively countered. In addition, you should consider whether you can avoid adding cryptographic features directly to your application (e.g. for secure transport, you may be able to tunnel your application over SSH).
Also, you may need to look beyond QCA for some security needs (e.g. for authentication, your situation may be more suited to using Kerberos than SASL or TLS).
Design
The architecture of QCA is shown below:
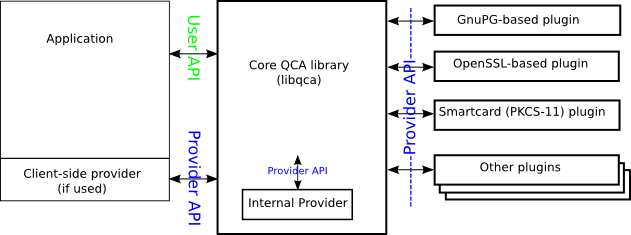
Application authors normally only need to use the User API. The provider API is available for plugin authors, but can also be used by application authors to provide very specific capabilities.
For more information on the design of QCA, you might like to review the Architecture description.
Availability
Releases
The latest release packages can be found in the QCA 2.x download area.
See the project web site for further information about QCA releases.
Current development
The latest version of the code is available from the KDE Git server (there is no formal release of the current version at this time). Naturally you will need Qt properly set up and configured in order to build and use QCA.
The Git code can be browsed via the web
Use
git clone https://invent.kde.org/libraries/qca.git
to get the latest sources.
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:50:48 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.