QCA::SASL
#include <QtCrypto>
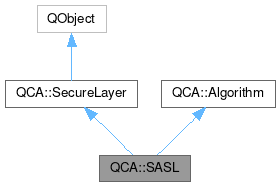
Classes | |
class | Params |
Public Types | |
enum | AuthCondition { AuthFail , NoMechanism , BadProtocol , BadServer , BadAuth , NoAuthzid , TooWeak , NeedEncrypt , Expired , Disabled , NoUser , RemoteUnavailable } |
enum | AuthFlags { AuthFlagsNone = 0x00 , AllowPlain = 0x01 , AllowAnonymous = 0x02 , RequireForwardSecrecy = 0x04 , RequirePassCredentials = 0x08 , RequireMutualAuth = 0x10 , RequireAuthzidSupport = 0x20 } |
enum | ClientSendMode { AllowClientSendFirst , DisableClientSendFirst } |
enum | Error { ErrorInit , ErrorHandshake , ErrorCrypt } |
enum | ServerSendMode { AllowServerSendLast , DisableServerSendLast } |
Signals | |
void | authCheck (const QString &user, const QString &authzid) |
void | authenticated () |
void | clientStarted (bool clientInit, const QByteArray &clientInitData) |
void | needParams (const QCA::SASL::Params ¶ms) |
void | nextStep (const QByteArray &stepData) |
void | serverStarted () |
![]() | |
void | closed () |
void | error () |
void | readyRead () |
void | readyReadOutgoing () |
Public Member Functions | |
SASL (QObject *parent=nullptr, const QString &provider=QString()) | |
AuthCondition | authCondition () const |
int | bytesAvailable () const override |
int | bytesOutgoingAvailable () const override |
void | continueAfterAuthCheck () |
void | continueAfterParams () |
int | convertBytesWritten (qint64 encryptedBytes) override |
Error | errorCode () const |
QString | mechanism () const |
QStringList | mechanismList () const |
void | putServerFirstStep (const QString &mech) |
void | putServerFirstStep (const QString &mech, const QByteArray &clientInit) |
void | putStep (const QByteArray &stepData) |
QByteArray | read () override |
QByteArray | readOutgoing (int *plainBytes=nullptr) override |
QStringList | realmList () const |
void | reset () |
void | setAuthzid (const QString &auth) |
void | setConstraints (AuthFlags f, int minSSF, int maxSSF) |
void | setConstraints (AuthFlags f, SecurityLevel s=SL_None) |
void | setExternalAuthId (const QString &authid) |
void | setExternalSSF (int strength) |
void | setLocalAddress (const QString &addr, quint16 port) |
void | setPassword (const SecureArray &pass) |
void | setRealm (const QString &realm) |
void | setRemoteAddress (const QString &addr, quint16 port) |
void | setUsername (const QString &user) |
int | ssf () const |
void | startClient (const QString &service, const QString &host, const QStringList &mechlist, ClientSendMode mode=AllowClientSendFirst) |
void | startServer (const QString &service, const QString &host, const QString &realm, ServerSendMode mode=DisableServerSendLast) |
void | write (const QByteArray &a) override |
void | writeIncoming (const QByteArray &a) override |
![]() | |
SecureLayer (QObject *parent=nullptr) | |
virtual void | close () |
virtual bool | isClosable () const |
virtual QByteArray | readUnprocessed () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
Algorithm (const Algorithm &from) | |
void | change (const QString &type, const QString &provider) |
void | change (Provider::Context *c) |
Provider::Context * | context () |
const Provider::Context * | context () const |
Algorithm & | operator= (const Algorithm &from) |
Provider * | provider () const |
Provider::Context * | takeContext () |
QString | type () const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
Algorithm () | |
Algorithm (const QString &type, const QString &provider) | |
Detailed Description
Simple Authentication and Security Layer protocol implementation.
This class implements the Simple Authenication and Security Layer protocol, which is described in RFC2222 - see http://www.ietf.org/rfc/rfc2222.txt.
As the name suggests, SASL provides authentication (eg, a "login" of some form), for a connection oriented protocol, and can also provide protection for the subsequent connection.
The SASL protocol is designed to be extensible, through a range of "mechanisms", where a mechanism is the actual authentication method. Example mechanisms include Anonymous, LOGIN, Kerberos V4, and GSSAPI. Mechanisms can be added (potentially without restarting the server application) by the system administrator.
It is important to understand that SASL is neither "network aware" nor "protocol aware". That means that SASL does not understand how the client connects to the server, and SASL does not understand the actual application protocol.
Definition at line 831 of file qca_securelayer.h.
Member Enumeration Documentation
◆ AuthCondition
Possible authentication error states.
Definition at line 848 of file qca_securelayer.h.
◆ AuthFlags
enum QCA::SASL::AuthFlags |
Authentication requirement flag values.
- Examples
- saslclient.cpp, and saslserver.cpp.
Definition at line 867 of file qca_securelayer.h.
◆ ClientSendMode
Mode options for client side sending.
Definition at line 881 of file qca_securelayer.h.
◆ Error
enum QCA::SASL::Error |
Possible errors that may occur when using SASL.
Enumerator | |
---|---|
ErrorInit | problem starting up SASL |
ErrorHandshake | problem during the authentication process |
ErrorCrypt | problem at anytime after |
Definition at line 838 of file qca_securelayer.h.
◆ ServerSendMode
Mode options for server side sending.
Definition at line 890 of file qca_securelayer.h.
Constructor & Destructor Documentation
◆ SASL()
Standard constructor.
- Parameters
-
parent the parent object for this SASL connection provider if specified, the provider to use. If not specified, or specified as empty, then any provider is acceptable.
Member Function Documentation
◆ authCheck
This signal is emitted when the server needs to perform the authentication check.
If the user and authzid are valid, call continueAfterAuthCheck().
- Parameters
-
user the user identification name authzid the user authorization name
- Examples
- saslserver.cpp.
◆ authCondition()
AuthCondition QCA::SASL::authCondition | ( | ) | const |
Return the reason for authentication failure.
◆ authenticated
|
signal |
This signal is emitted when authentication is complete.
- Examples
- saslclient.cpp, and saslserver.cpp.
◆ bytesAvailable()
|
overridevirtual |
Returns the number of bytes available to be read() on the application side.
Implements QCA::SecureLayer.
◆ bytesOutgoingAvailable()
|
overridevirtual |
Returns the number of bytes available to be readOutgoing() on the network side.
Implements QCA::SecureLayer.
◆ clientStarted
|
signal |
This signal is emitted when the client has been successfully started.
- Parameters
-
clientInit true if the client should send an initial response to the server clientInitData the initial response to send to the server. Do note that there is a difference in SASL between an empty initial response and no initial response, and so even if clientInitData is an empty array, you still need to send an initial response if clientInit is true.
- Examples
- saslclient.cpp.
◆ continueAfterAuthCheck()
void QCA::SASL::continueAfterAuthCheck | ( | ) |
Continue negotiation after auth ids have been checked (server)
◆ continueAfterParams()
void QCA::SASL::continueAfterParams | ( | ) |
Continue negotiation after parameters have been set (client)
◆ convertBytesWritten()
|
overridevirtual |
Convert encrypted bytes written to plain text bytes written.
- Parameters
-
encryptedBytes the number of bytes to convert
Implements QCA::SecureLayer.
◆ errorCode()
Error QCA::SASL::errorCode | ( | ) | const |
Return the error code.
◆ mechanism()
QString QCA::SASL::mechanism | ( | ) | const |
Return the mechanism selected (client)
◆ mechanismList()
QStringList QCA::SASL::mechanismList | ( | ) | const |
Return the mechanism list (server)
◆ needParams
|
signal |
This signal is emitted when the client needs additional parameters.
After receiving this signal, the application should set the required parameter values appropriately and then call continueAfterParams().
- Parameters
-
params the parameters that are required by the client
- Examples
- saslclient.cpp.
◆ nextStep
|
signal |
This signal is emitted when there is data required to be sent over the network to complete the next step in the authentication process.
- Parameters
-
stepData the data to send over the network
- Examples
- saslclient.cpp, and saslserver.cpp.
◆ putServerFirstStep() [1/2]
void QCA::SASL::putServerFirstStep | ( | const QString & | mech | ) |
Process the first step in server mode (server)
Call this with the mechanism selected by the client. If there is initial client data, call the other version of this function instead.
- Parameters
-
mech the mechanism to be used.
◆ putServerFirstStep() [2/2]
void QCA::SASL::putServerFirstStep | ( | const QString & | mech, |
const QByteArray & | clientInit ) |
Process the first step in server mode (server)
Call this with the mechanism selected by the client, and initial client data. If there is no initial client data, call the other version of this function instead.
- Parameters
-
mech the mechanism to be used clientInit the initial data provided by the client side
◆ putStep()
void QCA::SASL::putStep | ( | const QByteArray & | stepData | ) |
Process an authentication step.
Call this with authentication data received from the network. The only exception is the first step in server mode, in which case putServerFirstStep must be called.
- Parameters
-
stepData the authentication data from the network
◆ read()
|
overridevirtual |
This method reads decrypted (plain) data from the SecureLayer implementation.
You normally call this function on the application side after receiving the readyRead() signal.
Implements QCA::SecureLayer.
◆ readOutgoing()
|
overridevirtual |
This method provides encoded (typically encrypted) data.
You normally call this function to get data to write out to the network socket (e.g. using QTcpSocket::write()) after receiving the readyReadOutgoing() signal.
- Parameters
-
plainBytes the number of bytes that were read.
Implements QCA::SecureLayer.
◆ realmList()
QStringList QCA::SASL::realmList | ( | ) | const |
Return the realm list, if available (client)
◆ reset()
void QCA::SASL::reset | ( | ) |
Reset the SASL mechanism.
◆ serverStarted
|
signal |
This signal is emitted after the server has been successfully started.
- Examples
- saslserver.cpp.
◆ setAuthzid()
void QCA::SASL::setAuthzid | ( | const QString & | auth | ) |
Specify the authorization identity to use in authentication.
- Parameters
-
auth the authorization identity to use
◆ setConstraints() [1/2]
void QCA::SASL::setConstraints | ( | AuthFlags | f, |
int | minSSF, | ||
int | maxSSF ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.Unless you have a specific reason for directly specifying a strength factor, you probably should use the method above.
- Parameters
-
f the authentication requirements, which you typically build using a binary OR function (eg AllowPlain | AllowAnonymous) minSSF the minimum security strength factor that is required maxSSF the maximum security strength factor that is required
- Note
- Security strength factors are a rough approximation to key length in the encryption function (eg if you are securing with plain DES, the security strength factor would be 56).
◆ setConstraints() [2/2]
void QCA::SASL::setConstraints | ( | AuthFlags | f, |
SecurityLevel | s = SL_None ) |
Specify connection constraints.
SASL supports a range of authentication requirements, and a range of security levels. This method allows you to specify the requirements for your connection.
- Parameters
-
f the authentication requirements, which you typically build using a binary OR function (eg AllowPlain | AllowAnonymous) s the security level of the encryption, if used. See SecurityLevel for details of what each level provides.
◆ setExternalAuthId()
void QCA::SASL::setExternalAuthId | ( | const QString & | authid | ) |
Specify the id of the externally secured connection.
- Parameters
-
authid the id of the connection
◆ setExternalSSF()
void QCA::SASL::setExternalSSF | ( | int | strength | ) |
Specify a security strength factor for an externally secured connection.
- Parameters
-
strength the security strength factor of the connection
◆ setLocalAddress()
void QCA::SASL::setLocalAddress | ( | const QString & | addr, |
quint16 | port ) |
Specify the local address.
- Parameters
-
addr the address of the local part of the connection port the port number of the local part of the connection
◆ setPassword()
void QCA::SASL::setPassword | ( | const SecureArray & | pass | ) |
Specify the password to use in authentication.
- Parameters
-
pass the password to use
◆ setRealm()
void QCA::SASL::setRealm | ( | const QString & | realm | ) |
Specify the realm to use in authentication.
- Parameters
-
realm the realm to use
◆ setRemoteAddress()
void QCA::SASL::setRemoteAddress | ( | const QString & | addr, |
quint16 | port ) |
Specify the peer address.
- Parameters
-
addr the address of the peer side of the connection port the port number of the peer side of the connection
◆ setUsername()
void QCA::SASL::setUsername | ( | const QString & | user | ) |
Specify the username to use in authentication.
- Parameters
-
user the username to use
◆ ssf()
int QCA::SASL::ssf | ( | ) | const |
Return the security strength factor of the connection.
◆ startClient()
void QCA::SASL::startClient | ( | const QString & | service, |
const QString & | host, | ||
const QStringList & | mechlist, | ||
ClientSendMode | mode = AllowClientSendFirst ) |
Initialise the client side of the connection.
startClient must be called on the client side of the connection. clientStarted will be emitted when the operation is completed.
- Parameters
-
service the name of the service host the client side host name mechlist the list of mechanisms which can be used mode the mode to use on the client side
◆ startServer()
void QCA::SASL::startServer | ( | const QString & | service, |
const QString & | host, | ||
const QString & | realm, | ||
ServerSendMode | mode = DisableServerSendLast ) |
Initialise the server side of the connection.
startServer must be called on the server side of the connection. serverStarted will be emitted when the operation is completed.
- Parameters
-
service the name of the service host the server side host name realm the realm to use mode which mode to use on the server side
◆ write()
|
overridevirtual |
This method writes unencrypted (plain) data to the SecureLayer implementation.
You normally call this function on the application side.
- Parameters
-
a the source of the application-side data
Implements QCA::SecureLayer.
◆ writeIncoming()
|
overridevirtual |
This method accepts encoded (typically encrypted) data for processing.
You normally call this function using data read from the network socket (e.g. using QTcpSocket::readAll()) after receiving a signal that indicates that the socket has data to read.
- Parameters
-
a the ByteArray to take network-side data from
Implements QCA::SecureLayer.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:01:48 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.