QCA::SecureArray
#include <QtCrypto>
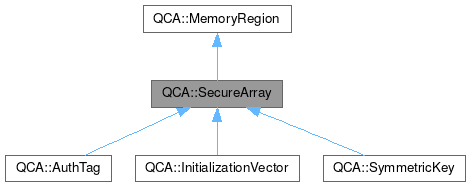
Public Member Functions | |
SecureArray () | |
SecureArray (const char *str) | |
SecureArray (const MemoryRegion &a) | |
SecureArray (const QByteArray &a) | |
SecureArray (const SecureArray &from) | |
SecureArray (int size, char ch=0) | |
SecureArray & | append (const SecureArray &a) |
char & | at (int index) |
const char & | at (int index) const |
void | clear () |
const char * | constData () const |
char * | data () |
const char * | data () const |
void | fill (char fillChar, int fillToPosition=-1) |
bool | isEmpty () const |
bool | operator!= (const MemoryRegion &other) const |
SecureArray & | operator+= (const SecureArray &a) |
SecureArray & | operator= (const QByteArray &a) |
SecureArray & | operator= (const SecureArray &from) |
bool | operator== (const MemoryRegion &other) const |
char & | operator[] (int index) |
const char & | operator[] (int index) const |
bool | resize (int size) |
int | size () const |
QByteArray | toByteArray () const |
![]() | |
MemoryRegion (const char *str) | |
MemoryRegion (const MemoryRegion &from) | |
MemoryRegion (const QByteArray &from) | |
const char & | at (int index) const |
const char * | constData () const |
const char * | data () const |
bool | isEmpty () const |
bool | isNull () const |
bool | isSecure () const |
MemoryRegion & | operator= (const MemoryRegion &from) |
MemoryRegion & | operator= (const QByteArray &from) |
int | size () const |
QByteArray | toByteArray () const |
Protected Member Functions | |
void | set (const QByteArray &from) |
void | set (const SecureArray &from) |
![]() | |
MemoryRegion (bool secure) | |
MemoryRegion (const QByteArray &from, bool secure) | |
MemoryRegion (int size, bool secure) | |
char & | at (int index) |
char * | data () |
bool | resize (int size) |
void | set (const QByteArray &from, bool secure) |
void | setSecure (bool secure) |
Detailed Description
Secure array of bytes.
The SecureArray provides an array of memory from a pool that is, at least partly, secure. In this sense, secure means that the contents of the memory should not be made available to other applications. By comparison, a QByteArray or QString may be held in pages that might be swapped to disk or free'd without being cleared first.
Note that this class is implicitly shared (that is, copy on write).
- Examples
- aes-cmac.cpp, ciphertest.cpp, eventhandlerdemo.cpp, hashtest.cpp, keyloader.cpp, mactest.cpp, md5crypt.cpp, publickeyexample.cpp, randomtest.cpp, and rsatest.cpp.
Definition at line 316 of file qca_tools.h.
Constructor & Destructor Documentation
◆ SecureArray() [1/6]
QCA::SecureArray::SecureArray | ( | ) |
Construct a secure byte array, zero length.
◆ SecureArray() [2/6]
|
explicit |
Construct a secure byte array of the specified length.
- Parameters
-
size the number of bytes in the array ch the value every byte should be set to
◆ SecureArray() [3/6]
QCA::SecureArray::SecureArray | ( | const char * | str | ) |
Construct a secure byte array from a string.
Note that this copies, rather than references the source array.
- Parameters
-
str the source of the data (as a null terminated string).
◆ SecureArray() [4/6]
QCA::SecureArray::SecureArray | ( | const QByteArray & | a | ) |
Construct a secure byte array from a QByteArray.
Note that this copies, rather than references the source array.
- Parameters
-
a the source of the data.
- See also
- operator=()
◆ SecureArray() [5/6]
QCA::SecureArray::SecureArray | ( | const MemoryRegion & | a | ) |
Construct a secure byte array from a MemoryRegion.
Note that this copies, rather than references the source array
- Parameters
-
a the source of the data.
- See also
- operator=()
◆ SecureArray() [6/6]
QCA::SecureArray::SecureArray | ( | const SecureArray & | from | ) |
Construct a (shallow) copy of another secure byte array.
- Parameters
-
from the source of the data and length.
Member Function Documentation
◆ append()
SecureArray & QCA::SecureArray::append | ( | const SecureArray & | a | ) |
Append a secure byte array to the end of this array.
- Parameters
-
a the array to append to this array
- Examples
- ciphertest.cpp, and md5crypt.cpp.
◆ at() [1/2]
char & QCA::SecureArray::at | ( | int | index | ) |
Returns a reference to the byte at the index position.
- Parameters
-
index the zero-based offset to obtain
◆ at() [2/2]
const char & QCA::SecureArray::at | ( | int | index | ) | const |
Returns a reference to the byte at the index position.
- Parameters
-
index the zero-based offset to obtain
◆ clear()
void QCA::SecureArray::clear | ( | ) |
Clears the contents of the array and makes it empty.
◆ constData()
const char * QCA::SecureArray::constData | ( | ) | const |
Pointer to the data in the secure array.
You can use this for memcpy and similar functions. If you are trying to obtain data at a particular offset, you might be better off using at() or operator[]
◆ data() [1/2]
char * QCA::SecureArray::data | ( | ) |
Pointer to the data in the secure array.
You can use this for memcpy and similar functions. If you are trying to obtain data at a particular offset, you might be better off using at() or operator[]
- Examples
- ciphertest.cpp, hashtest.cpp, mactest.cpp, md5crypt.cpp, publickeyexample.cpp, and rsatest.cpp.
◆ data() [2/2]
const char * QCA::SecureArray::data | ( | ) | const |
Pointer to the data in the secure array.
You can use this for memcpy and similar functions. If you are trying to obtain data at a particular offset, you might be better off using at() or operator[]
◆ fill()
void QCA::SecureArray::fill | ( | char | fillChar, |
int | fillToPosition = -1 ) |
Fill the data array with a specified character.
- Parameters
-
fillChar the character to use as the fill fillToPosition the number of characters to fill to. If not specified (or -1), fills array to current length.
- Note
- This function does not extend the array - if you ask for fill beyond the current length, only the current length will be used.
- The number of characters is 1 based, so if you ask for fill('x', 10), it will fill from
- Examples
- md5crypt.cpp.
◆ isEmpty()
bool QCA::SecureArray::isEmpty | ( | ) | const |
Test if the array contains any bytes.
This is equivalent to testing (size() != 0). Note that if the array is allocated, isEmpty() is false (even if no data has been added)
- Returns
- true if the array has zero length, otherwise false
- Examples
- rsatest.cpp.
◆ operator!=()
|
inline |
Inequality operator.
Returns true if both arrays have different length, or the same length but different data.
- Parameters
-
other the MemoryRegion to compare to
Definition at line 516 of file qca_tools.h.
◆ operator+=()
SecureArray & QCA::SecureArray::operator+= | ( | const SecureArray & | a | ) |
Append a secure byte array to the end of this array.
- Parameters
-
a the array to append to this array
◆ operator=() [1/2]
SecureArray & QCA::SecureArray::operator= | ( | const QByteArray & | a | ) |
Creates a copy, rather than references.
- Parameters
-
a the array to copy from
◆ operator=() [2/2]
SecureArray & QCA::SecureArray::operator= | ( | const SecureArray & | from | ) |
Creates a reference, rather than a deep copy.
- Parameters
-
from the array to reference
◆ operator==()
bool QCA::SecureArray::operator== | ( | const MemoryRegion & | other | ) | const |
Equality operator.
Returns true if both arrays have the same data (and the same length, of course).
- Parameters
-
other the MemoryRegion to compare to
◆ operator[]() [1/2]
char & QCA::SecureArray::operator[] | ( | int | index | ) |
Returns a reference to the byte at the index position.
- Parameters
-
index the zero-based offset to obtain
◆ operator[]() [2/2]
const char & QCA::SecureArray::operator[] | ( | int | index | ) | const |
Returns a reference to the byte at the index position.
- Parameters
-
index the zero-based offset to obtain
◆ resize()
bool QCA::SecureArray::resize | ( | int | size | ) |
Change the length of this array If the new length is less than the old length, the extra information is (safely) discarded.
If the new length is equal to or greater than the old length, the existing data is copied into the array.
- Parameters
-
size the new length
- Examples
- aes-cmac.cpp.
◆ set() [1/2]
|
protected |
Assign the contents of a provided byte array to this object.
- Parameters
-
from the byte array to copy
◆ set() [2/2]
|
protected |
Assign the contents of a provided byte array to this object.
- Parameters
-
from the byte array to copy
◆ size()
int QCA::SecureArray::size | ( | ) | const |
Returns the number of bytes in the array.
- Examples
- aes-cmac.cpp, and md5crypt.cpp.
◆ toByteArray()
QByteArray QCA::SecureArray::toByteArray | ( | ) | const |
Copy the contents of the secure array out to a standard QByteArray.
Note that this performs a deep copy of the data.
- Examples
- ciphertest.cpp, eventhandlerdemo.cpp, hashtest.cpp, mactest.cpp, md5crypt.cpp, and rsatest.cpp.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:01:48 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.