QCA::MemoryRegion
#include <QtCrypto>
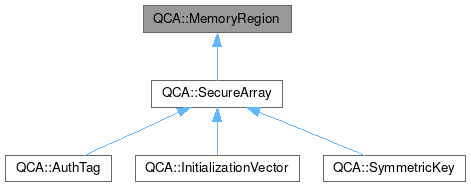
Public Member Functions | |
MemoryRegion (const char *str) | |
MemoryRegion (const MemoryRegion &from) | |
MemoryRegion (const QByteArray &from) | |
const char & | at (int index) const |
const char * | constData () const |
const char * | data () const |
bool | isEmpty () const |
bool | isNull () const |
bool | isSecure () const |
MemoryRegion & | operator= (const MemoryRegion &from) |
MemoryRegion & | operator= (const QByteArray &from) |
int | size () const |
QByteArray | toByteArray () const |
Protected Member Functions | |
MemoryRegion (bool secure) | |
MemoryRegion (const QByteArray &from, bool secure) | |
MemoryRegion (int size, bool secure) | |
char & | at (int index) |
char * | data () |
bool | resize (int size) |
void | set (const QByteArray &from, bool secure) |
void | setSecure (bool secure) |
Detailed Description
Array of bytes that may be optionally secured.
This class is mostly unusable on its own. Either use it as a SecureArray subclass or call toByteArray() to convert to QByteArray.
Note that this class is implicitly shared (that is, copy on write).
- Examples
- aes-cmac.cpp.
Definition at line 90 of file qca_tools.h.
Constructor & Destructor Documentation
◆ MemoryRegion() [1/6]
QCA::MemoryRegion::MemoryRegion | ( | const char * | str | ) |
Constructs a new Memory Region from a null terminated character array.
- Parameters
-
str pointer to the array of data to copy
◆ MemoryRegion() [2/6]
QCA::MemoryRegion::MemoryRegion | ( | const QByteArray & | from | ) |
Constructs a new MemoryRegion from the data in a byte array.
- Parameters
-
from the QByteArray to copy from
◆ MemoryRegion() [3/6]
QCA::MemoryRegion::MemoryRegion | ( | const MemoryRegion & | from | ) |
Standard copy constructor.
- Parameters
-
from the MemoryRegion to copy from
◆ MemoryRegion() [4/6]
|
protected |
◆ MemoryRegion() [5/6]
|
protected |
Create a memory region, optionally using secure storage.
- Parameters
-
size the number of bytes in the memory region. secure if this is true, the memory region will use secure storage.
◆ MemoryRegion() [6/6]
|
protected |
Create a memory region, optionally using secure storage.
This constructor variant allows you to initialize the memory region from an existing array.
- Parameters
-
from the byte array to copy from. secure if this is true, the memory region will use secure storage.
Member Function Documentation
◆ at() [1/2]
|
protected |
◆ at() [2/2]
const char & QCA::MemoryRegion::at | ( | int | index | ) | const |
◆ constData()
const char * QCA::MemoryRegion::constData | ( | ) | const |
Convert the contents of the memory region to a C-compatible character array.
This consists of size() bytes, followed by a null terminator.
- See also
- toByteArray for an alternative approach.
- data which is equivalent to this method
◆ data() [1/2]
|
protected |
Convert the contents of the memory region to a C-compatible character array.
This consists of size() bytes, followed by a null terminator.
◆ data() [2/2]
const char * QCA::MemoryRegion::data | ( | ) | const |
Convert the contents of the memory region to a C-compatible character array.
This consists of size() bytes, followed by a null terminator.
- See also
- toByteArray for an alternative approach.
- constData, which is equivalent to this method, but avoids the possibility that the compiler picks the wrong version.
◆ isEmpty()
bool QCA::MemoryRegion::isEmpty | ( | ) | const |
Returns true if the size of the memory region is zero.
◆ isNull()
bool QCA::MemoryRegion::isNull | ( | ) | const |
Test if the MemoryRegion is null (i.e.
was created as a null array, and hasn't been resized).
This is probably not what you are trying to do. If you are trying to determine whether there are any bytes in the array, use isEmpty() instead.
◆ isSecure()
bool QCA::MemoryRegion::isSecure | ( | ) | const |
Test if the MemoryRegion is using secure memory, or not.
In this context, memory is secure if it will not be paged out to disk.
- Returns
- true if the memory region is secure
◆ operator=() [1/2]
MemoryRegion & QCA::MemoryRegion::operator= | ( | const MemoryRegion & | from | ) |
Standard assignment operator.
- Parameters
-
from the MemoryRegion to copy from
◆ operator=() [2/2]
MemoryRegion & QCA::MemoryRegion::operator= | ( | const QByteArray & | from | ) |
Standard assignment operator.
- Parameters
-
from the QByteArray to copy from
◆ resize()
|
protected |
Resize the memory region to the specified size.
- Parameters
-
size the new size of the region.
◆ set()
|
protected |
Modify the memory region to match a specified byte array.
This resizes the memory region as required to match the byte array size.
- Parameters
-
from the byte array to copy from. secure if this is true, the memory region will use secure storage.
◆ setSecure()
|
protected |
Convert the memory region to use the specified memory type.
This may involve copying data from secure to insecure storage, or from insecure to secure storage.
- Parameters
-
secure if true, use secure memory; otherwise use insecure memory.
◆ size()
int QCA::MemoryRegion::size | ( | ) | const |
Returns the number of bytes in the memory region.
◆ toByteArray()
QByteArray QCA::MemoryRegion::toByteArray | ( | ) | const |
Convert this memory region to a byte array.
- Note
- For secure data, this will make it insecure
- See also
- data() and constData() for other ways to convert to an "accessible" format.
- Examples
- aes-cmac.cpp.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 24 2025 11:46:50 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.