QCA::Hash
#include <QtCrypto>
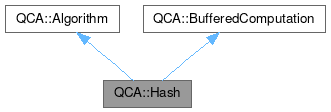
Public Member Functions | |
Hash (const Hash &from) | |
Hash (const QString &type, const QString &provider=QString()) | |
void | clear () override |
MemoryRegion | final () override |
MemoryRegion | hash (const MemoryRegion &array) |
QString | hashToString (const MemoryRegion &array) |
Hash & | operator= (const Hash &from) |
QString | type () const |
void | update (const char *data, int len=-1) |
void | update (const MemoryRegion &a) override |
void | update (const QByteArray &a) |
void | update (QIODevice *file) |
![]() | |
Algorithm (const Algorithm &from) | |
void | change (const QString &type, const QString &provider) |
void | change (Provider::Context *c) |
Provider::Context * | context () |
const Provider::Context * | context () const |
Algorithm & | operator= (const Algorithm &from) |
Provider * | provider () const |
Provider::Context * | takeContext () |
QString | type () const |
![]() | |
MemoryRegion | process (const MemoryRegion &a) |
Static Public Member Functions | |
static QStringList | supportedTypes (const QString &provider=QString()) |
Additional Inherited Members | |
![]() | |
Algorithm () | |
Algorithm (const QString &type, const QString &provider) | |
Detailed Description
General class for hashing algorithms.
Hash is the class for the various hashing algorithms within QCA. SHA256, SHA1 or RIPEMD160 are recommended for new applications, although MD2, MD4, MD5 or SHA0 may be applicable (for interoperability reasons) for some applications.
To perform a hash, you create a Hash object, call update() with the data that needs to be hashed, and then call final(), which returns a QByteArray of the hash result. An example (using the SHA1 hash, with 1000 updates of a 1000 byte string) is shown below:
If you only have a simple hash requirement - a single string that is fully available in memory at one time - then you may be better off with one of the convenience methods. So, for example, instead of creating a QCA::Hash object, then doing a single update() and the final() call; you could simply call QCA::Hash("algoName").hash() with the data that you would otherwise have provided to the update() call.
For more information on hashing algorithms, see Hashing Algorithms.
- Examples
- hashtest.cpp, and md5crypt.cpp.
Definition at line 208 of file qca_basic.h.
Constructor & Destructor Documentation
◆ Hash() [1/2]
Constructor.
- Parameters
-
type label for the type of hash to be created (for example, "sha1" or "md2") provider the name of the provider plugin for the subclass (eg "qca-ossl")
◆ Hash() [2/2]
Member Function Documentation
◆ clear()
|
overridevirtual |
Reset a hash, dumping all previous parts of the message.
This method clears (or resets) the hash algorithm, effectively undoing any previous update() calls. You should use this call if you are re-using a Hash sub-class object to calculate additional hashes.
Implements QCA::BufferedComputation.
◆ final()
|
overridevirtual |
Finalises input and returns the hash result.
After calling update() with the required data, the hash results are finalised and produced.
Note that it is not possible to add further data (with update()) after calling final(), because of the way the hashing works - null bytes are inserted to pad the results up to a fixed size. If you want to reuse the Hash object, you should call clear() and start to update() again.
Implements QCA::BufferedComputation.
- Examples
- hashtest.cpp.
◆ hash()
MemoryRegion QCA::Hash::hash | ( | const MemoryRegion & | array | ) |
Hash a byte array, returning it as another byte array
This is a convenience method that returns the hash of a SecureArray.
- Parameters
-
array the QByteArray to hash
If you need more flexibility (e.g. you are constructing a large byte array object just to pass it to hash(), then consider creating an Hash object, and then calling update() and final().
◆ hashToString()
QString QCA::Hash::hashToString | ( | const MemoryRegion & | array | ) |
Hash a byte array, returning it as a printable string
This is a convenience method that returns the hash of a SecureArray as a hexadecimal representation encoded in a QString.
- Parameters
-
array the QByteArray to hash
If you need more flexibility, you can create a Hash object, call Hash::update() as required, then call Hash::final(), before using the static arrayToHex() method.
- Examples
- hashtest.cpp.
◆ operator=()
Assignment operator.
- Parameters
-
from the Hash object to copy state from
◆ supportedTypes()
|
static |
Returns a list of all of the hash types available.
- Parameters
-
provider the name of the provider to get a list from, if one provider is required. If not specified, available hash types from all providers will be returned.
◆ type()
QString QCA::Hash::type | ( | ) | const |
Return the hash type.
◆ update() [1/4]
void QCA::Hash::update | ( | const char * | data, |
int | len = -1 ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.This method is provided to assist with code that already exists, and is being ported to QCA.
You are better off passing a SecureArray (as shown above) if you are writing new code.
- Parameters
-
data pointer to a char array len the length of the array. If not specified (or specified as a negative number), the length will be determined with strlen(), which may not be what you want if the array contains a null (0x00) character.
◆ update() [2/4]
|
overridevirtual |
Update a hash, adding more of the message contents to the digest.
The whole message needs to be added using this method before you call final().
If you find yourself only calling update() once, you may be better off using a convenience method such as hash() or hashToString() instead.
- Parameters
-
a the byte array to add to the hash
Implements QCA::BufferedComputation.
- Examples
- hashtest.cpp.
◆ update() [3/4]
void QCA::Hash::update | ( | const QByteArray & | a | ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters
-
a the QByteArray to add to the hash
◆ update() [4/4]
void QCA::Hash::update | ( | QIODevice * | file | ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.This allows you to read from a file or other I/O device.
Note that the device must be already open for reading
- Parameters
-
file an I/O device
If you are trying to calculate the hash of a whole file (and it isn't already open), you might want to use code like this:
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:01:48 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.