QCA::TLS
#include <QtCrypto>
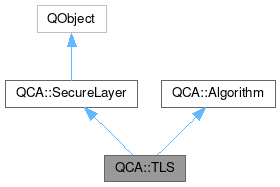
Public Types | |
enum | Error { ErrorSignerExpired , ErrorSignerInvalid , ErrorCertKeyMismatch , ErrorInit , ErrorHandshake , ErrorCrypt } |
enum | IdentityResult { Valid , HostMismatch , InvalidCertificate , NoCertificate } |
enum | Mode { Stream , Datagram } |
enum | Version { TLS_v1 , SSL_v3 , SSL_v2 , DTLS_v1 } |
![]() | |
typedef | QObjectList |
Signals | |
void | certificateRequested () |
void | handshaken () |
void | hostNameReceived () |
void | peerCertificateAvailable () |
![]() | |
void | closed () |
void | error () |
void | readyRead () |
void | readyReadOutgoing () |
Public Member Functions | |
TLS (Mode mode, QObject *parent=nullptr, const QString &provider=QString()) | |
TLS (QObject *parent=nullptr, const QString &provider=QString()) | |
~TLS () override | |
int | bytesAvailable () const override |
int | bytesOutgoingAvailable () const override |
bool | canCompress () const |
bool | canSetHostName () const |
int | cipherBits () const |
int | cipherMaxBits () const |
QString | cipherSuite () const |
void | close () override |
bool | compressionEnabled () const |
void | continueAfterStep () |
int | convertBytesWritten (qint64 encryptedBytes) override |
Error | errorCode () const |
QString | hostName () const |
bool | isClosable () const override |
bool | isCompressed () const |
bool | isHandshaken () const |
QList< CertificateInfoOrdered > | issuerList () const |
CertificateChain | localCertificateChain () const |
PrivateKey | localPrivateKey () const |
int | packetMTU () const |
int | packetsAvailable () const |
int | packetsOutgoingAvailable () const |
CertificateChain | peerCertificateChain () const |
Validity | peerCertificateValidity () const |
IdentityResult | peerIdentityResult () const |
QByteArray | read () override |
QByteArray | readOutgoing (int *plainBytes=nullptr) override |
QByteArray | readUnprocessed () override |
void | reset () |
TLSSession | session () const |
void | setCertificate (const CertificateChain &cert, const PrivateKey &key) |
void | setCertificate (const KeyBundle &kb) |
void | setCompressionEnabled (bool b) |
void | setConstraints (const QStringList &cipherSuiteList) |
void | setConstraints (int minSSF, int maxSSF) |
void | setConstraints (SecurityLevel s) |
void | setIssuerList (const QList< CertificateInfoOrdered > &issuers) |
void | setPacketMTU (int size) const |
void | setSession (const TLSSession &session) |
void | setTrustedCertificates (const CertificateCollection &trusted) |
void | startClient (const QString &host=QString()) |
void | startServer () |
QStringList | supportedCipherSuites (const Version &version=TLS_v1) const |
CertificateCollection | trustedCertificates () const |
Version | version () const |
void | write (const QByteArray &a) override |
void | writeIncoming (const QByteArray &a) override |
![]() | |
SecureLayer (QObject *parent=nullptr) | |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
Algorithm (const Algorithm &from) | |
void | change (const QString &type, const QString &provider) |
void | change (Provider::Context *c) |
Provider::Context * | context () |
const Provider::Context * | context () const |
Algorithm & | operator= (const Algorithm &from) |
Provider * | provider () const |
Provider::Context * | takeContext () |
QString | type () const |
Protected Member Functions | |
void | connectNotify (const QMetaMethod &signal) override |
void | disconnectNotify (const QMetaMethod &signal) override |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | customEvent (QEvent *event) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
Algorithm () | |
Algorithm (const QString &type, const QString &provider) | |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Detailed Description
Transport Layer Security / Secure Socket Layer.
Transport Layer Security (TLS) is the current state-of-the-art in secure transport mechanisms over the internet. It can be used in a way where only one side of the link needs to authenticate to the other. This makes it very useful for servers to provide their identity to clients. Note that is is possible to use TLS to authenticate both client and server.
TLS is a IETF standard (RFC2712 for TLS version 1.0, and RFC4346 for TLS version 1.1) based on earlier Netscape work on Secure Socket Layer (SSL version 2 and SSL version 3). New applications should use at least TLS 1.0, and SSL version 2 should be avoided due to known security problems.
- Examples
- sslservtest.cpp, ssltest.cpp, and tlssocket.cpp.
Definition at line 289 of file qca_securelayer.h.
Member Enumeration Documentation
◆ Error
enum QCA::TLS::Error |
Type of error.
Definition at line 316 of file qca_securelayer.h.
◆ IdentityResult
Type of identity.
Enumerator | |
---|---|
Valid | identity is verified |
HostMismatch | valid cert provided, but wrong owner |
InvalidCertificate | invalid cert |
NoCertificate | identity unknown |
Definition at line 329 of file qca_securelayer.h.
◆ Mode
enum QCA::TLS::Mode |
Operating mode.
Enumerator | |
---|---|
Stream | stream mode |
Datagram | datagram mode |
Definition at line 296 of file qca_securelayer.h.
◆ Version
enum QCA::TLS::Version |
Version of TLS or SSL.
Enumerator | |
---|---|
TLS_v1 | Transport Layer Security, version 1. |
SSL_v3 | Secure Socket Layer, version 3. |
SSL_v2 | Secure Socket Layer, version 2. |
DTLS_v1 | Datagram Transport Layer Security, version 1. |
Definition at line 305 of file qca_securelayer.h.
Constructor & Destructor Documentation
◆ TLS() [1/2]
Constructor for Transport Layer Security connection.
This produces a Stream (normal TLS) rather than Datagram (DTLS) object. If you want to do DTLS, see below.
- Parameters
-
parent the parent object for this object provider the name of the provider, if a specific provider is required
◆ TLS() [2/2]
|
explicit |
Constructor for Transport Layer Security connection.
This constructor can be used for both normal TLS (set mode to TLS::Stream) or DTLS (set mode to TLS::Datagram).
- Parameters
-
mode the connection Mode parent the parent object for this object provider the name of the provider, if a specific provider is required
◆ ~TLS()
|
override |
Destructor.
Member Function Documentation
◆ bytesAvailable()
|
overridevirtual |
Returns the number of bytes available to be read() on the application side.
Implements QCA::SecureLayer.
◆ bytesOutgoingAvailable()
|
overridevirtual |
Returns the number of bytes available to be readOutgoing() on the network side.
Implements QCA::SecureLayer.
◆ canCompress()
bool QCA::TLS::canCompress | ( | ) | const |
Test if the link can use compression.
- Returns
- true if the link can use compression
◆ canSetHostName()
bool QCA::TLS::canSetHostName | ( | ) | const |
Test if the link can specify a hostname (Server Name Indication)
- Returns
- true if the link can specify a hostname
◆ certificateRequested
|
signal |
Emitted when the server requests a certificate.
At this time, the client can inspect the issuerList().
You must call continueAfterStep() in order for TLS processing to resume after this signal is emitted.
This signal is only emitted in client mode.
- See also
- continueAfterStep
- Examples
- ssltest.cpp.
◆ cipherBits()
int QCA::TLS::cipherBits | ( | ) | const |
The number of effective bits of security being used for this connection.
This can differ from the actual number of bits in the cipher for certain older "export ciphers" that are deliberately crippled. If you want that information, use cipherMaxBits().
- Examples
- ssltest.cpp.
◆ cipherMaxBits()
int QCA::TLS::cipherMaxBits | ( | ) | const |
The number of bits of security that the cipher could use.
This is normally the same as cipherBits(), but can be greater for older "export ciphers".
- Examples
- ssltest.cpp.
◆ cipherSuite()
QString QCA::TLS::cipherSuite | ( | ) | const |
The cipher suite that has been negotiated for this connection.
The name returned here is the name used in the applicable RFC (or Internet Draft, where there is no RFC).
- Examples
- ssltest.cpp.
◆ close()
|
overridevirtual |
Close the link.
Note that this may not be meaningful / possible for all implementations.
- See also
- isClosable() for a test that verifies if the link can be closed.
Reimplemented from QCA::SecureLayer.
- Examples
- sslservtest.cpp.
◆ compressionEnabled()
bool QCA::TLS::compressionEnabled | ( | ) | const |
Returns true if compression is enabled.
This only indicates whether or not the object is configured to use compression, not whether or not the link is actually compressed. Use isCompressed() for that.
◆ connectNotify()
|
overrideprotectedvirtual |
Called when a connection is made to a particular signal.
- Parameters
-
signal the name of the signal that has been connected to.
Reimplemented from QObject.
◆ continueAfterStep()
void QCA::TLS::continueAfterStep | ( | ) |
Resumes TLS processing.
Call this function after hostNameReceived(), certificateRequested() peerCertificateAvailable() or handshaken() is emitted. By requiring this function to be called in order to proceed, applications are given a chance to perform user interaction between steps in the TLS process.
- Examples
- sslservtest.cpp, and ssltest.cpp.
◆ convertBytesWritten()
|
overridevirtual |
Convert encrypted bytes written to plain text bytes written.
- Parameters
-
encryptedBytes the number of bytes to convert
Implements QCA::SecureLayer.
- Examples
- sslservtest.cpp.
◆ disconnectNotify()
|
overrideprotectedvirtual |
Called when a connection is removed from a particular signal.
- Parameters
-
signal the name of the signal that has been disconnected from.
Reimplemented from QObject.
◆ errorCode()
Error QCA::TLS::errorCode | ( | ) | const |
This method returns the type of error that has occurred.
You should only need to check this if the error() signal is emitted.
- Examples
- sslservtest.cpp, and ssltest.cpp.
◆ handshaken
|
signal |
Emitted when the protocol handshake is complete.
At this time, all available information about the TLS session can be inspected.
You must call continueAfterStep() in order for TLS processing to resume after this signal is emitted.
- See also
- continueAfterStep
- isHandshaken
- Examples
- sslservtest.cpp, ssltest.cpp, and tlssocket.cpp.
◆ hostName()
QString QCA::TLS::hostName | ( | ) | const |
Returns the host name specified or an empty string if no host name is specified.
◆ hostNameReceived
|
signal |
Emitted if a host name is set by the client.
At this time, the server can inspect the hostName().
You must call continueAfterStep() in order for TLS processing to resume after this signal is emitted.
This signal is only emitted in server mode.
- See also
- continueAfterStep
◆ isClosable()
|
overridevirtual |
Returns true if the layer has a meaningful "close".
Reimplemented from QCA::SecureLayer.
◆ isCompressed()
bool QCA::TLS::isCompressed | ( | ) | const |
test if the link is compressed
- Returns
- true if the link is compressed
◆ isHandshaken()
bool QCA::TLS::isHandshaken | ( | ) | const |
◆ issuerList()
QList< CertificateInfoOrdered > QCA::TLS::issuerList | ( | ) | const |
Retrieve the list of allowed issuers by the server, if the server has provided them.
Only DN types will be present.
- Examples
- ssltest.cpp.
◆ localCertificateChain()
CertificateChain QCA::TLS::localCertificateChain | ( | ) | const |
The CertificateChain for the local host certificate.
◆ localPrivateKey()
PrivateKey QCA::TLS::localPrivateKey | ( | ) | const |
The PrivateKey for the local host certificate.
◆ packetMTU()
int QCA::TLS::packetMTU | ( | ) | const |
Return the currently configured maximum packet size.
- Note
- this is only used with DTLS
◆ packetsAvailable()
int QCA::TLS::packetsAvailable | ( | ) | const |
Determine the number of packets available to be read on the application side.
- Note
- this is only used with DTLS.
◆ packetsOutgoingAvailable()
int QCA::TLS::packetsOutgoingAvailable | ( | ) | const |
Determine the number of packets available to be read on the network side.
- Note
- this is only used with DTLS.
◆ peerCertificateAvailable
|
signal |
Emitted when a certificate is received from the peer.
At this time, you may inspect peerIdentityResult(), peerCertificateValidity(), and peerCertificateChain().
You must call continueAfterStep() in order for TLS processing to resume after this signal is emitted.
- See also
- continueAfterStep
◆ peerCertificateChain()
CertificateChain QCA::TLS::peerCertificateChain | ( | ) | const |
The CertificateChain from the peer (other end of the connection to the trusted root certificate).
- Examples
- ssltest.cpp.
◆ peerCertificateValidity()
Validity QCA::TLS::peerCertificateValidity | ( | ) | const |
After the SSL/TLS handshake is valid, this method allows you to check if the received certificate from the other end is valid.
As noted in peerIdentityResult(), you also need to check that the certificate matches the entity you are trying to communicate with.
- Examples
- ssltest.cpp.
◆ peerIdentityResult()
IdentityResult QCA::TLS::peerIdentityResult | ( | ) | const |
After the SSL/TLS handshake is complete, this method allows you to determine if the other end of the connection (if the application is a client, this is the server; if the application is a server, this is the client) has a valid identity.
Note that the security of TLS/SSL depends on checking this. It is not enough to check that the certificate is valid - you must check that the certificate is valid for the entity that you are trying to communicate with.
- Note
- If this returns QCA::TLS::InvalidCertificate, you may wish to use peerCertificateValidity() to determine whether to proceed or not.
- Examples
- ssltest.cpp, and tlssocket.cpp.
◆ read()
|
overridevirtual |
This method reads decrypted (plain) data from the SecureLayer implementation.
You normally call this function on the application side after receiving the readyRead() signal.
Implements QCA::SecureLayer.
- Examples
- sslservtest.cpp, ssltest.cpp, and tlssocket.cpp.
◆ readOutgoing()
|
overridevirtual |
This method provides encoded (typically encrypted) data.
You normally call this function to get data to write out to the network socket (e.g. using QTcpSocket::write()) after receiving the readyReadOutgoing() signal.
- Parameters
-
plainBytes the number of bytes that were read.
Implements QCA::SecureLayer.
- Examples
- sslservtest.cpp, ssltest.cpp, and tlssocket.cpp.
◆ readUnprocessed()
|
overridevirtual |
This allows you to read data without having it decrypted first.
This is intended to be used for protocols that close off the connection and return to plain text transfer. You do not normally need to use this function.
Reimplemented from QCA::SecureLayer.
◆ reset()
void QCA::TLS::reset | ( | ) |
Reset the connection.
- Examples
- tlssocket.cpp.
◆ session()
TLSSession QCA::TLS::session | ( | ) | const |
The session object of the TLS connection, which can be used for resuming.
◆ setCertificate() [1/2]
void QCA::TLS::setCertificate | ( | const CertificateChain & | cert, |
const PrivateKey & | key ) |
The local certificate to use.
This is the certificate that will be provided to the peer. This is almost always required on the server side (because the server has to provide a certificate to the client), and may be used on the client side.
- Parameters
-
cert a chain of certificates that link the host certificate to a trusted root certificate. key the private key for the certificate chain
- Examples
- sslservtest.cpp.
◆ setCertificate() [2/2]
void QCA::TLS::setCertificate | ( | const KeyBundle & | kb | ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.Allows setting a certificate from a KeyBundle.
- Parameters
-
kb key bundle containing the local certificate and associated private key.
◆ setCompressionEnabled()
void QCA::TLS::setCompressionEnabled | ( | bool | b | ) |
Set the link to use compression.
- Parameters
-
b true if the link should use compression, or false to disable compression
◆ setConstraints() [1/3]
void QCA::TLS::setConstraints | ( | const QStringList & | cipherSuiteList | ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters
-
cipherSuiteList a list of the names of cipher suites that can be used for this link.
- Note
- the names are the same as the names in the applicable IETF RFCs (or Internet Drafts if there is no applicable RFC).
◆ setConstraints() [2/3]
void QCA::TLS::setConstraints | ( | int | minSSF, |
int | maxSSF ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters
-
minSSF the minimum Security Strength Factor required for this link. maxSSF the maximum Security Strength Factor required for this link.
◆ setConstraints() [3/3]
void QCA::TLS::setConstraints | ( | SecurityLevel | s | ) |
The security level required for this link.
- Parameters
-
s the level required for this link.
◆ setIssuerList()
void QCA::TLS::setIssuerList | ( | const QList< CertificateInfoOrdered > & | issuers | ) |
Sets the issuer list to present to the client.
For use with servers only. Only DN types are allowed.
- Parameters
-
issuers the list of valid issuers to be used.
◆ setPacketMTU()
void QCA::TLS::setPacketMTU | ( | int | size | ) | const |
Set the maximum packet size to use.
- Parameters
-
size the number of bytes to set as the MTU.
- Note
- this is only used with DTLS.
◆ setSession()
void QCA::TLS::setSession | ( | const TLSSession & | session | ) |
Resume a TLS session using the given session object.
- Parameters
-
session the session state to use for resumption.
◆ setTrustedCertificates()
void QCA::TLS::setTrustedCertificates | ( | const CertificateCollection & | trusted | ) |
Set up the set of trusted certificates that will be used to verify that the certificate provided is valid.
Typically, this will be the collection of root certificates from the system, which you can get using QCA::systemStore(), however you may choose to pass whatever certificates match your assurance needs.
- Parameters
-
trusted a bundle of trusted certificates.
- Examples
- ssltest.cpp, and tlssocket.cpp.
◆ startClient()
Start the TLS/SSL connection as a client.
Typically, you'll want to perform RFC 2818 validation on the server's certificate, based on the hostname you're intending to connect to. Pass a value for host in order to have the validation for you. If you want to bypass this behavior and do the validation yourself, pass an empty string for host.
If the host is an internationalized domain name, then it must be provided in unicode format, not in IDNA ACE/punycode format.
- Parameters
-
host the hostname that you want to connect to
- Note
- The hostname will be used for Server Name Indication extension (see RFC 3546 Section 3.1) if supported by the backend provider.
- Examples
- ssltest.cpp, and tlssocket.cpp.
◆ startServer()
void QCA::TLS::startServer | ( | ) |
Start the TLS/SSL connection as a server.
- Examples
- sslservtest.cpp.
◆ supportedCipherSuites()
QStringList QCA::TLS::supportedCipherSuites | ( | const Version & | version = TLS_v1 | ) | const |
Get the list of cipher suites that are available for use.
A cipher suite is a combination of key exchange, encryption and hashing algorithms that are agreed during the initial handshake between client and server.
- Parameters
-
version the protocol Version that the cipher suites are required for
- Returns
- list of the names of the cipher suites supported.
◆ trustedCertificates()
CertificateCollection QCA::TLS::trustedCertificates | ( | ) | const |
Return the trusted certificates set for this object.
◆ version()
Version QCA::TLS::version | ( | ) | const |
The protocol version that is in use for this connection.
◆ write()
|
overridevirtual |
This method writes unencrypted (plain) data to the SecureLayer implementation.
You normally call this function on the application side.
- Parameters
-
a the source of the application-side data
Implements QCA::SecureLayer.
- Examples
- sslservtest.cpp, ssltest.cpp, and tlssocket.cpp.
◆ writeIncoming()
|
overridevirtual |
This method accepts encoded (typically encrypted) data for processing.
You normally call this function using data read from the network socket (e.g. using QTcpSocket::readAll()) after receiving a signal that indicates that the socket has data to read.
- Parameters
-
a the ByteArray to take network-side data from
Implements QCA::SecureLayer.
- Examples
- sslservtest.cpp, ssltest.cpp, and tlssocket.cpp.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Dec 20 2024 11:47:12 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.