QCA::Certificate
#include <QtCrypto>
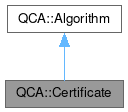
Static Public Member Functions | |
static Certificate | fromDER (const QByteArray &a, ConvertResult *result=nullptr, const QString &provider=QString()) |
static Certificate | fromPEM (const QString &s, ConvertResult *result=nullptr, const QString &provider=QString()) |
static Certificate | fromPEMFile (const QString &fileName, ConvertResult *result=nullptr, const QString &provider=QString()) |
Additional Inherited Members | |
![]() | |
Algorithm () | |
Algorithm (const QString &type, const QString &provider) | |
Detailed Description
Public Key (X.509) certificate.
This class contains one X.509 certificate
- Examples
- certtest.cpp, publickeyexample.cpp, sslservtest.cpp, and ssltest.cpp.
Definition at line 856 of file qca_cert.h.
Constructor & Destructor Documentation
◆ Certificate() [1/4]
QCA::Certificate::Certificate | ( | ) |
Create an empty Certificate.
◆ Certificate() [2/4]
QCA::Certificate::Certificate | ( | const QString & | fileName | ) |
Create a Certificate from a PEM encoded file.
- Parameters
-
fileName the name (and path, if required) of the file that contains the PEM encoded certificate
◆ Certificate() [3/4]
QCA::Certificate::Certificate | ( | const CertificateOptions & | opts, |
const PrivateKey & | key, | ||
const QString & | provider = QString() ) |
Create a Certificate with specified options and a specified private key.
- Parameters
-
opts the options to use key the private key for this certificate provider the provider to use to create this key, if a particular provider is required
◆ Certificate() [4/4]
QCA::Certificate::Certificate | ( | const Certificate & | from | ) |
Standard copy constructor.
- Parameters
-
from the certificate to copy from
Member Function Documentation
◆ change()
void QCA::Certificate::change | ( | CertContext * | c | ) |
- Parameters
-
c context (internal)
◆ commonName()
QString QCA::Certificate::commonName | ( | ) | const |
The common name of the subject of the certificate.
Common names are normally the name of a person, company or organisation
- Examples
- ssltest.cpp.
◆ constraints()
Constraints QCA::Certificate::constraints | ( | ) | const |
The constraints that apply to this certificate.
◆ crlLocations()
QStringList QCA::Certificate::crlLocations | ( | ) | const |
◆ fromDER()
|
static |
Import the certificate from DER.
- Parameters
-
a the array containing the certificate in DER format result a pointer to a ConvertResult, which if not-null will be set to the conversion status provider the provider to use, if a specific provider is required
- Returns
- the Certificate corresponding to the certificate in the provided array
◆ fromPEM()
|
static |
Import the certificate from PEM format.
- Parameters
-
s the string containing the certificate in PEM format result a pointer to a ConvertResult, which if not-null will be set to the conversion status provider the provider to use, if a specific provider is required
- Returns
- the Certificate corresponding to the certificate in the provided string
- Examples
- sslservtest.cpp, and ssltest.cpp.
◆ fromPEMFile()
|
static |
Import the certificate from a file.
- Parameters
-
fileName the name (and path, if required) of the file containing the certificate in PEM format result a pointer to a ConvertResult, which if not-null will be set to the conversion status provider the provider to use, if a specific provider is required
- Returns
- the Certificate corresponding to the certificate in the provided string
◆ isCA()
bool QCA::Certificate::isCA | ( | ) | const |
Test if the Certificate is valid as a Certificate Authority.
- Returns
- true if the Certificate is valid as a Certificate Authority
- Examples
- certtest.cpp.
◆ isIssuerOf()
bool QCA::Certificate::isIssuerOf | ( | const Certificate & | other | ) | const |
Test if the Certificate has signed another Certificate object and is therefore the issuer.
- Parameters
-
other the certificate to test
- Returns
- true if this certificate is the issuer of the argument
◆ isNull()
bool QCA::Certificate::isNull | ( | ) | const |
Test if the certificate is empty (null)
- Returns
- true if the certificate is null
- Examples
- publickeyexample.cpp, and sslservtest.cpp.
◆ isSelfSigned()
bool QCA::Certificate::isSelfSigned | ( | ) | const |
Test if the Certificate is self-signed.
- Returns
- true if the certificate is self-signed
- Examples
- certtest.cpp.
◆ issuerInfo()
CertificateInfo QCA::Certificate::issuerInfo | ( | ) | const |
Properties of the issuer of the certificate.
- See also
- subjectInfo for how the return value works.
- Examples
- certtest.cpp.
◆ issuerInfoOrdered()
CertificateInfoOrdered QCA::Certificate::issuerInfoOrdered | ( | ) | const |
Properties of the issuer of the certificate, as an ordered list (QList of CertificateInfoPair).
This allows access to the certificate information in the same order as they appear in a certificate. Each pair in the list has a type and a value.
- See also
- issuerInfo for an unordered version
- subjectInfoOrdered for the ordered information on the subject
- CertificateInfoPair for the elements in the list
◆ issuerKeyId()
QByteArray QCA::Certificate::issuerKeyId | ( | ) | const |
The key identifier associated with the issuer.
◆ issuerLocations()
QStringList QCA::Certificate::issuerLocations | ( | ) | const |
List of URI locations for issuer certificate files.
Each URI refers to the same issuer file
◆ matchesHostName()
bool QCA::Certificate::matchesHostName | ( | const QString & | host | ) | const |
Test if the subject of the certificate matches a specified host name.
This will return true (indicating a match), if the specified host name meets the RFC 2818 validation rules with this certificate.
If the host is an internationalized domain name, then it must be provided in unicode format, not in IDNA ACE/punycode format.
- Parameters
-
host the name of the host to compare to
◆ notValidAfter()
QDateTime QCA::Certificate::notValidAfter | ( | ) | const |
The latest date that the certificate is valid.
- Examples
- certtest.cpp, and ssltest.cpp.
◆ notValidBefore()
QDateTime QCA::Certificate::notValidBefore | ( | ) | const |
The earliest date that the certificate is valid.
- Examples
- certtest.cpp, and ssltest.cpp.
◆ ocspLocations()
QStringList QCA::Certificate::ocspLocations | ( | ) | const |
List of URI locations for OCSP services.
◆ operator!=()
|
inline |
Inequality operator.
- Parameters
-
other the certificate to compare this certificate with
Definition at line 1176 of file qca_cert.h.
◆ operator=()
Certificate & QCA::Certificate::operator= | ( | const Certificate & | from | ) |
Standard assignment operator.
- Parameters
-
from the Certificate to assign from
◆ operator==()
bool QCA::Certificate::operator== | ( | const Certificate & | a | ) | const |
Test for equality of two certificates.
- Parameters
-
a the certificate to compare this certificate with
- Returns
- true if the two certificates are the same
◆ pathLimit()
int QCA::Certificate::pathLimit | ( | ) | const |
The upper bound of the number of links in the certificate chain, if any.
◆ policies()
QStringList QCA::Certificate::policies | ( | ) | const |
The policies that apply to this certificate.
Policies are specified as strings containing OIDs
◆ serialNumber()
BigInteger QCA::Certificate::serialNumber | ( | ) | const |
The serial number of the certificate.
- Examples
- certtest.cpp.
◆ signatureAlgorithm()
SignatureAlgorithm QCA::Certificate::signatureAlgorithm | ( | ) | const |
The signature algorithm used for the signature on this certificate.
◆ subjectInfo()
CertificateInfo QCA::Certificate::subjectInfo | ( | ) | const |
Properties of the subject of the certificate, as a QMultiMap.
This is the method that provides information on the subject organisation, common name, DNS name, and so on. The list of information types (i.e. the key to the multi-map) is a CertificateInfoType. The values are a list of QString.
An example of how you can iterate over the list is:
- Examples
- certtest.cpp.
◆ subjectInfoOrdered()
CertificateInfoOrdered QCA::Certificate::subjectInfoOrdered | ( | ) | const |
Properties of the subject of the certificate, as an ordered list (QList of CertificateInfoPair).
This allows access to the certificate information in the same order as they appear in a certificate. Each pair in the list has a type and a value.
For example:
- See also
- subjectInfo for an unordered version
- issuerInfoOrdered for the ordered information on the issuer
- CertificateInfoPair for the elements in the list
◆ subjectKeyId()
QByteArray QCA::Certificate::subjectKeyId | ( | ) | const |
The key identifier associated with the subject.
◆ subjectPublicKey()
PublicKey QCA::Certificate::subjectPublicKey | ( | ) | const |
The public key associated with the subject of the certificate.
◆ toDER()
QByteArray QCA::Certificate::toDER | ( | ) | const |
Export the Certificate into a DER format.
◆ toPEM()
QString QCA::Certificate::toPEM | ( | ) | const |
Export the Certificate into a PEM format.
- Examples
- certtest.cpp, and ssltest.cpp.
◆ toPEMFile()
bool QCA::Certificate::toPEMFile | ( | const QString & | fileName | ) | const |
Export the Certificate into PEM format in a file.
- Parameters
-
fileName the name of the file to use
◆ validate()
Validity QCA::Certificate::validate | ( | const CertificateCollection & | trusted, |
const CertificateCollection & | untrusted, | ||
UsageMode | u = UsageAny, | ||
ValidateFlags | vf = ValidateAll ) const |
Check the validity of a certificate.
- Parameters
-
trusted a collection of trusted certificates untrusted a collection of additional certificates, not necessarily trusted u the use required for the certificate vf the conditions to validate
- Note
- This function may block
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:01:48 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.