QCA::PublicKey
#include <QtCrypto>
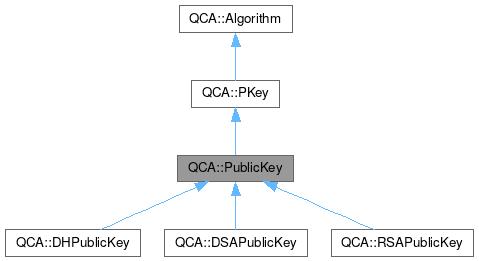
Static Public Member Functions | |
static PublicKey | fromDER (const QByteArray &a, ConvertResult *result=nullptr, const QString &provider=QString()) |
static PublicKey | fromPEM (const QString &s, ConvertResult *result=nullptr, const QString &provider=QString()) |
static PublicKey | fromPEMFile (const QString &fileName, ConvertResult *result=nullptr, const QString &provider=QString()) |
![]() | |
static QList< Type > | supportedIOTypes (const QString &provider=QString()) |
static QList< Type > | supportedTypes (const QString &provider=QString()) |
Protected Member Functions | |
PublicKey (const QString &type, const QString &provider) | |
![]() | |
PKey (const QString &type, const QString &provider) | |
void | set (const PKey &k) |
DHPrivateKey | toDHPrivateKey () const |
DHPublicKey | toDHPublicKey () const |
DSAPrivateKey | toDSAPrivateKey () const |
DSAPublicKey | toDSAPublicKey () const |
RSAPrivateKey | toRSAPrivateKey () const |
RSAPublicKey | toRSAPublicKey () const |
![]() | |
Algorithm () | |
Algorithm (const QString &type, const QString &provider) | |
Additional Inherited Members | |
![]() | |
enum | Type { RSA , DSA , DH } |
Detailed Description
Constructor & Destructor Documentation
◆ PublicKey() [1/5]
QCA::PublicKey::PublicKey | ( | ) |
Create an empty (null) public key.
◆ PublicKey() [2/5]
QCA::PublicKey::PublicKey | ( | const PrivateKey & | k | ) |
Create a public key based on a specified private key.
- Parameters
-
k the private key to extract the public key parts from
◆ PublicKey() [3/5]
QCA::PublicKey::PublicKey | ( | const QString & | fileName | ) |
Import a public key from a PEM representation in a file.
- Parameters
-
fileName the name of the file containing the public key
- See also
- fromPEMFile for an alternative method
◆ PublicKey() [4/5]
QCA::PublicKey::PublicKey | ( | const PublicKey & | from | ) |
Copy constructor.
- Parameters
-
from the PublicKey to copy from
◆ PublicKey() [5/5]
Create a new key of a specified type.
- Parameters
-
type the type of key to create provider the provider to use, if required
Member Function Documentation
◆ canDecrypt()
bool QCA::PublicKey::canDecrypt | ( | ) | const |
Test if this key can be used for decryption.
- Returns
- true if the key can be used for decryption
◆ canEncrypt()
bool QCA::PublicKey::canEncrypt | ( | ) | const |
Test if this key can be used for encryption.
- Returns
- true if the key can be used for encryption
- Examples
- rsatest.cpp.
◆ canVerify()
bool QCA::PublicKey::canVerify | ( | ) | const |
Test if the key can be used for verifying signatures.
- Returns
- true of the key can be used for verification
- Examples
- rsatest.cpp.
◆ decrypt()
bool QCA::PublicKey::decrypt | ( | const SecureArray & | in, |
SecureArray * | out, | ||
EncryptionAlgorithm | alg ) |
Decrypt the message.
- Parameters
-
in the cipher (encrypted) data out the plain text data alg the algorithm to use
- Note
- This synchronous operation may require event handling, and so it must not be called from the same thread as an EventHandler.
◆ encrypt()
SecureArray QCA::PublicKey::encrypt | ( | const SecureArray & | a, |
EncryptionAlgorithm | alg ) |
Encrypt a message using a specified algorithm.
- Parameters
-
a the message to encrypt alg the algorithm to use
- Examples
- rsatest.cpp.
◆ fromDER()
|
static |
Import a key in Distinguished Encoding Rules (DER) format.
This function takes a binary array, which is assumed to contain a public key in DER encoding, and returns the key. Unless you don't care whether the import succeeded, you should test the result, as shown below.
- Parameters
-
a the array containing a DER encoded key result pointer to a variable, which returns whether the conversion succeeded (ConvertGood) or not provider the name of the provider to use for the import.
◆ fromPEM()
|
static |
Import a key in Privacy Enhanced Mail (PEM) format.
This function takes a string, which is assumed to contain a public key in PEM encoding, and returns that key. Unless you don't care whether the import succeeded, you should test the result, as shown below.
- Parameters
-
s the string containing a PEM encoded key result pointer to a variable, which returns whether the conversion succeeded (ConvertGood) or not provider the name of the provider to use for the import.
- See also
- toPEM, which provides an inverse of fromPEM()
- fromPEMFile, which provides an import direct from a file.
◆ fromPEMFile()
|
static |
Import a key in Privacy Enhanced Mail (PEM) format from a file.
This function takes the name of a file, which is assumed to contain a public key in PEM encoding, and returns that key. Unless you don't care whether the import succeeded, you should test the result, as shown below.
- Parameters
-
fileName a string containing the name of the file result pointer to a variable, which returns whether the conversion succeeded (ConvertGood) or not provider the name of the provider to use for the import.
- See also
- toPEMFile, which provides an inverse of fromPEMFile()
- fromPEM, which provides an import from a string
- Note
- there is also a constructor form that can import from a file
◆ maximumEncryptSize()
int QCA::PublicKey::maximumEncryptSize | ( | EncryptionAlgorithm | alg | ) | const |
The maximum message size that can be encrypted with a specified algorithm.
- Parameters
-
alg the algorithm to check
◆ operator=()
Assignment operator.
- Parameters
-
from the PublicKey to copy from
◆ startVerify()
void QCA::PublicKey::startVerify | ( | SignatureAlgorithm | alg, |
SignatureFormat | format = DefaultFormat ) |
Initialise the signature verification process.
- Parameters
-
alg the algorithm to use for signing format the specific format to use, for DSA
- Examples
- rsatest.cpp.
◆ toDER()
QByteArray QCA::PublicKey::toDER | ( | ) | const |
Export the key in Distinguished Encoding Rules (DER) format.
◆ toDH()
DHPublicKey QCA::PublicKey::toDH | ( | ) | const |
Convenience method to convert this key to a DHPublicKey.
Note that if the key is not an DH key (eg it is DSA or RSA), then this will produce a null key.
◆ toDSA()
DSAPublicKey QCA::PublicKey::toDSA | ( | ) | const |
Convenience method to convert this key to a DSAPublicKey.
Note that if the key is not an DSA key (eg it is RSA or DH), then this will produce a null key.
◆ toPEM()
QString QCA::PublicKey::toPEM | ( | ) | const |
◆ toPEMFile()
bool QCA::PublicKey::toPEMFile | ( | const QString & | fileName | ) | const |
Export the key in Privacy Enhanced Mail (PEM) to a file.
- Parameters
-
fileName the name (and path, if necessary) of the file to save the PEM encoded key to.
- See also
- toPEM for a version that exports to a QString, which may be useful if you need to do more sophisticated handling
- fromPEMFile provides an inverse of toPEMFile, reading a PEM encoded key from a file
◆ toRSA()
RSAPublicKey QCA::PublicKey::toRSA | ( | ) | const |
Convenience method to convert this key to an RSAPublicKey.
Note that if the key is not an RSA key (eg it is DSA or DH), then this will produce a null key.
◆ update()
void QCA::PublicKey::update | ( | const MemoryRegion & | a | ) |
Update the signature verification process with more data.
- Parameters
-
a the array containing the data that should be added to the signature
- Examples
- rsatest.cpp.
◆ validSignature()
bool QCA::PublicKey::validSignature | ( | const QByteArray & | sig | ) |
Check the signature is valid for the message.
The process to check that a signature is correct is shown below:
- Parameters
-
sig the signature to check
- Returns
- true if the signature is correct
- Examples
- rsatest.cpp.
◆ verifyMessage()
bool QCA::PublicKey::verifyMessage | ( | const MemoryRegion & | a, |
const QByteArray & | sig, | ||
SignatureAlgorithm | alg, | ||
SignatureFormat | format = DefaultFormat ) |
Single step message verification.
If you have the whole message to be verified, then this offers a more convenient approach to verification.
- Parameters
-
a the message to check the signature on sig the signature to be checked alg the algorithm to use format the signature format to use, for DSA
- Returns
- true if the signature is valid for the message
- Examples
- rsatest.cpp.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:01:48 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.