QCA::PKey
#include <QtCrypto>
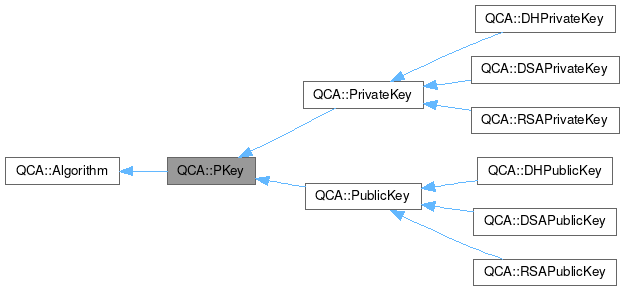
Public Types | |
enum | Type { RSA , DSA , DH } |
Public Member Functions | |
PKey () | |
PKey (const PKey &from) | |
int | bitSize () const |
bool | canExport () const |
bool | canKeyAgree () const |
bool | isDH () const |
bool | isDSA () const |
bool | isNull () const |
bool | isPrivate () const |
bool | isPublic () const |
bool | isRSA () const |
bool | operator!= (const PKey &a) const |
PKey & | operator= (const PKey &from) |
bool | operator== (const PKey &a) const |
PrivateKey | toPrivateKey () const |
PublicKey | toPublicKey () const |
Type | type () const |
![]() | |
Algorithm (const Algorithm &from) | |
void | change (const QString &type, const QString &provider) |
void | change (Provider::Context *c) |
Provider::Context * | context () |
const Provider::Context * | context () const |
Algorithm & | operator= (const Algorithm &from) |
Provider * | provider () const |
Provider::Context * | takeContext () |
QString | type () const |
Static Public Member Functions | |
static QList< Type > | supportedIOTypes (const QString &provider=QString()) |
static QList< Type > | supportedTypes (const QString &provider=QString()) |
Protected Member Functions | |
PKey (const QString &type, const QString &provider) | |
void | set (const PKey &k) |
DHPrivateKey | toDHPrivateKey () const |
DHPublicKey | toDHPublicKey () const |
DSAPrivateKey | toDSAPrivateKey () const |
DSAPublicKey | toDSAPublicKey () const |
RSAPrivateKey | toRSAPrivateKey () const |
RSAPublicKey | toRSAPublicKey () const |
![]() | |
Algorithm () | |
Algorithm (const QString &type, const QString &provider) | |
Detailed Description
General superclass for public (PublicKey) and private (PrivateKey) keys used with asymmetric encryption techniques.
Definition at line 250 of file qca_publickey.h.
Member Enumeration Documentation
◆ Type
enum QCA::PKey::Type |
Types of public key cryptography keys supported by QCA.
Enumerator | |
---|---|
RSA | RSA key. |
DSA | DSA key. |
DH | Diffie Hellman key. |
Definition at line 256 of file qca_publickey.h.
Constructor & Destructor Documentation
◆ PKey() [1/3]
QCA::PKey::PKey | ( | ) |
Standard constructor.
◆ PKey() [2/3]
QCA::PKey::PKey | ( | const PKey & | from | ) |
Standard copy constructor.
- Parameters
-
from the key to copy from
◆ PKey() [3/3]
Create a key of the specified type.
- Parameters
-
type the name of the type of key to create provider the name of the provider to create the key in
Member Function Documentation
◆ bitSize()
int QCA::PKey::bitSize | ( | ) | const |
Report the number of bits in the key.
- Examples
- keyloader.cpp.
◆ canExport()
bool QCA::PKey::canExport | ( | ) | const |
Test if the key data can be exported.
If the key resides on a smart card or other such device, this will likely return false.
◆ canKeyAgree()
bool QCA::PKey::canKeyAgree | ( | ) | const |
Test if the key can be used for key agreement.
◆ isDH()
bool QCA::PKey::isDH | ( | ) | const |
Test if the key is a Diffie Hellman key.
◆ isDSA()
bool QCA::PKey::isDSA | ( | ) | const |
Test if the key is a DSA key.
◆ isNull()
bool QCA::PKey::isNull | ( | ) | const |
Test if the key is null (empty)
- Returns
- true if the key is null
- Examples
- rsatest.cpp, and sslservtest.cpp.
◆ isPrivate()
bool QCA::PKey::isPrivate | ( | ) | const |
Test if the key is a private key.
◆ isPublic()
bool QCA::PKey::isPublic | ( | ) | const |
Test if the key is a public key.
◆ isRSA()
bool QCA::PKey::isRSA | ( | ) | const |
Test if the key is an RSA key.
◆ operator!=()
bool QCA::PKey::operator!= | ( | const PKey & | a | ) | const |
test if two keys are not equal
- Parameters
-
a the key to compare with this key
◆ operator=()
Standard assignment operator.
- Parameters
-
from the PKey to copy from
◆ operator==()
bool QCA::PKey::operator== | ( | const PKey & | a | ) | const |
test if two keys are equal
- Parameters
-
a the key to compare with this key
◆ set()
|
protected |
Set the key.
- Parameters
-
k the key to assign from
◆ supportedIOTypes()
Test what types of keys are supported for IO operations.
If you are using PKey DER or PEM operations, then you need to check for appropriate support using this method. For example, if you want to check if you can export or import an RSA key, then you need to do something like:
Note that if you only want to check for basic functionality (ie not PEM or DER import/export), then you can use supportedTypes(). There is no need to use both - if the key type is supported for IO, then is also supported for basic operations.
- Parameters
-
provider the name of the provider to use, if a particular provider is required.
- See also
- supportedTypes()
◆ supportedTypes()
Test what types of keys are supported.
Normally you would just test if the capability is present, however for PKey, you also need to test which types of keys are available. So if you want to figure out if RSA keys are supported, you need to do something like:
To make things a bit more complex, supportedTypes() only checks for basic functionality. If you want to check that you can do operations with PEM or DER (eg toPEM(), fromPEM(), and the equivalent DER and PEMfile operations, plus anything else that uses them, including the constructor form that takes a fileName), then you need to check for supportedIOTypes() instead.
- Parameters
-
provider the name of the provider to use, if a particular provider is required.
- See also
- supportedIOTypes()
◆ toDHPrivateKey()
|
protected |
Interpret this key as a DHPrivateKey.
- Note
- This function is essentially a convenience cast - if the key was created as a DSA key, this function cannot turn it into a DH key.
- See also
- toPrivateKey() for the public version of this method
◆ toDHPublicKey()
|
protected |
Interpret this key as an DHPublicKey.
- Note
- This function is essentially a convenience cast - if the key was created as a DSA key, this function cannot turn it into a DH key.
- See also
- toPublicKey() for the public version of this method
◆ toDSAPrivateKey()
|
protected |
Interpret this key as a DSAPrivateKey.
- Note
- This function is essentially a convenience cast - if the key was created as an RSA key, this function cannot turn it into a DSA key.
- See also
- toPrivateKey() for the public version of this method
◆ toDSAPublicKey()
|
protected |
Interpret this key as an DSAPublicKey.
- Note
- This function is essentially a convenience cast - if the key was created as an RSA key, this function cannot turn it into a DSA key.
- See also
- toPublicKey() for the public version of this method
◆ toPrivateKey()
PrivateKey QCA::PKey::toPrivateKey | ( | ) | const |
Interpret this key as a PrivateKey.
◆ toPublicKey()
PublicKey QCA::PKey::toPublicKey | ( | ) | const |
Interpret this key as a PublicKey.
- See also
- toRSAPublicKey(), toDSAPublicKey() and toDHPublicKey() for protected forms of this call.
- Examples
- rsatest.cpp.
◆ toRSAPrivateKey()
|
protected |
Interpret this key as an RSAPrivateKey.
- Note
- This function is essentially a convenience cast - if the key was created as a DSA key, this function cannot turn it into a RSA key.
- See also
- toPrivateKey() for the public version of this method
◆ toRSAPublicKey()
|
protected |
Interpret this key as an RSAPublicKey.
- Note
- This function is essentially a convenience cast - if the key was created as a DSA key, this function cannot turn it into an RSA key.
- See also
- toPublicKey() for the public version of this method
◆ type()
Type QCA::PKey::type | ( | ) | const |
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Feb 28 2025 11:49:14 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.