QCA::PrivateKey
#include <QtCrypto>
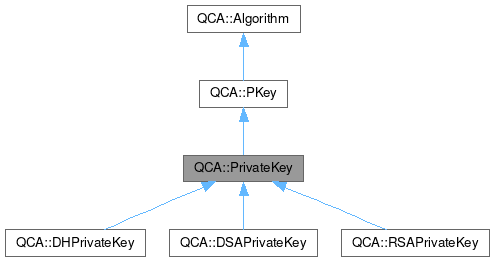
Static Public Member Functions | |
static PrivateKey | fromDER (const SecureArray &a, const SecureArray &passphrase=SecureArray(), ConvertResult *result=nullptr, const QString &provider=QString()) |
static PrivateKey | fromPEM (const QString &s, const SecureArray &passphrase=SecureArray(), ConvertResult *result=nullptr, const QString &provider=QString()) |
static PrivateKey | fromPEMFile (const QString &fileName, const SecureArray &passphrase=SecureArray(), ConvertResult *result=nullptr, const QString &provider=QString()) |
static QList< PBEAlgorithm > | supportedPBEAlgorithms (const QString &provider=QString()) |
![]() | |
static QList< Type > | supportedIOTypes (const QString &provider=QString()) |
static QList< Type > | supportedTypes (const QString &provider=QString()) |
Protected Member Functions | |
PrivateKey (const QString &type, const QString &provider) | |
![]() | |
PKey (const QString &type, const QString &provider) | |
void | set (const PKey &k) |
DHPrivateKey | toDHPrivateKey () const |
DHPublicKey | toDHPublicKey () const |
DSAPrivateKey | toDSAPrivateKey () const |
DSAPublicKey | toDSAPublicKey () const |
RSAPrivateKey | toRSAPrivateKey () const |
RSAPublicKey | toRSAPublicKey () const |
![]() | |
Algorithm () | |
Algorithm (const QString &type, const QString &provider) | |
Additional Inherited Members | |
![]() | |
enum | Type { RSA , DSA , DH } |
Detailed Description
Generic private key.
- Examples
- publickeyexample.cpp, rsatest.cpp, and sslservtest.cpp.
Definition at line 832 of file qca_publickey.h.
Constructor & Destructor Documentation
◆ PrivateKey() [1/4]
QCA::PrivateKey::PrivateKey | ( | ) |
Create an empty private key.
◆ PrivateKey() [2/4]
|
explicit |
Import a private key from a PEM representation in a file.
- Parameters
-
fileName the name of the file containing the private key passphrase the pass phrase for the private key
- See also
- fromPEMFile for an alternative method
- Note
- This synchronous operation may require event handling, and so it must not be called from the same thread as an EventHandler.
◆ PrivateKey() [3/4]
QCA::PrivateKey::PrivateKey | ( | const PrivateKey & | from | ) |
Copy constructor.
- Parameters
-
from the PrivateKey to copy from
◆ PrivateKey() [4/4]
Create a new private key.
- Parameters
-
type the type of key to create provider the provider to use, if a specific provider is required.
Member Function Documentation
◆ canDecrypt()
bool QCA::PrivateKey::canDecrypt | ( | ) | const |
Test if this key can be used for decryption.
- Returns
- true if the key can be used for decryption
- Examples
- publickeyexample.cpp.
◆ canEncrypt()
bool QCA::PrivateKey::canEncrypt | ( | ) | const |
Test if this key can be used for encryption.
- Returns
- true if the key can be used for encryption
◆ canSign()
bool QCA::PrivateKey::canSign | ( | ) | const |
Test if this key can be used for signing.
- Returns
- true if the key can be used to make a signature
- Examples
- rsatest.cpp.
◆ decrypt()
bool QCA::PrivateKey::decrypt | ( | const SecureArray & | in, |
SecureArray * | out, | ||
EncryptionAlgorithm | alg ) |
Decrypt the message.
- Parameters
-
in the cipher (encrypted) data out the plain text data alg the algorithm to use
- Note
- This synchronous operation may require event handling, and so it must not be called from the same thread as an EventHandler.
- Examples
- publickeyexample.cpp, and rsatest.cpp.
◆ deriveKey()
SymmetricKey QCA::PrivateKey::deriveKey | ( | const PublicKey & | theirs | ) |
Derive a shared secret key from a public key.
- Parameters
-
theirs the public key to derive from
◆ encrypt()
SecureArray QCA::PrivateKey::encrypt | ( | const SecureArray & | a, |
EncryptionAlgorithm | alg ) |
Encrypt a message using a specified algorithm.
- Parameters
-
a the message to encrypt alg the algorithm to use
◆ fromDER()
|
static |
Import the key from Distinguished Encoding Rules (DER) format.
- Parameters
-
a the array containing the DER representation of the key passphrase the pass phrase that is used to protect the key result a pointer to a ConvertResult, that if specified, will be set to reflect the result of the import provider the provider to use, if a particular provider is required
- See also
- toDER provides an inverse of fromDER, exporting the key to an array
- QCA::KeyLoader for an asynchronous loader approach.
- Note
- This synchronous operation may require event handling, and so it must not be called from the same thread as an EventHandler.
◆ fromPEM()
|
static |
Import the key from Privacy Enhanced Mail (PEM) format.
- Parameters
-
s the string containing the PEM representation of the key passphrase the pass phrase that is used to protect the key result a pointer to a ConvertResult, that if specified, will be set to reflect the result of the import provider the provider to use, if a particular provider is required
- See also
- toPEM provides an inverse of fromPEM, exporting the key to a string in PEM encoding.
- QCA::KeyLoader for an asynchronous loader approach.
- Note
- This synchronous operation may require event handling, and so it must not be called from the same thread as an EventHandler.
- Examples
- sslservtest.cpp.
◆ fromPEMFile()
|
static |
Import the key in Privacy Enhanced Mail (PEM) format from a file.
- Parameters
-
fileName the name (and path, if required) of the file containing the PEM representation of the key passphrase the pass phrase that is used to protect the key result a pointer to a ConvertResult, that if specified, will be set to reflect the result of the import provider the provider to use, if a particular provider is required
- See also
- toPEMFile provides an inverse of fromPEMFile
- fromPEM which allows import from a string
- QCA::KeyLoader for an asynchronous loader approach.
- Note
- there is also a constructor form, that allows you to create the key directly
- This synchronous operation may require event handling, and so it must not be called from the same thread as an EventHandler.
- Examples
- publickeyexample.cpp, and rsatest.cpp.
◆ maximumEncryptSize()
int QCA::PrivateKey::maximumEncryptSize | ( | EncryptionAlgorithm | alg | ) | const |
The maximum message size that can be encrypted with a specified algorithm.
- Parameters
-
alg the algorithm to check
◆ operator=()
PrivateKey & QCA::PrivateKey::operator= | ( | const PrivateKey & | from | ) |
Assignment operator.
- Parameters
-
from the PrivateKey to copy from
◆ signature()
QByteArray QCA::PrivateKey::signature | ( | ) |
The resulting signature.
- Note
- This synchronous operation may require event handling, and so it must not be called from the same thread as an EventHandler.
- Examples
- rsatest.cpp.
◆ signMessage()
QByteArray QCA::PrivateKey::signMessage | ( | const MemoryRegion & | a, |
SignatureAlgorithm | alg, | ||
SignatureFormat | format = DefaultFormat ) |
One step signature process.
- Parameters
-
a the message to sign alg the algorithm to use for the signature format the signature format to use, for DSA
- Returns
- the signature
- Note
- This synchronous operation may require event handling, and so it must not be called from the same thread as an EventHandler.
◆ startSign()
void QCA::PrivateKey::startSign | ( | SignatureAlgorithm | alg, |
SignatureFormat | format = DefaultFormat ) |
Initialise the message signature process.
- Parameters
-
alg the algorithm to use for the message signature process format the signature format to use, for DSA
- Note
- This synchronous operation may require event handling, and so it must not be called from the same thread as an EventHandler.
- Examples
- rsatest.cpp.
◆ supportedPBEAlgorithms()
|
static |
List the supported Password Based Encryption Algorithms that can be used to protect the key.
- Parameters
-
provider the provider to use, if a particular provider is required
◆ toDER()
SecureArray QCA::PrivateKey::toDER | ( | const SecureArray & | passphrase = SecureArray(), |
PBEAlgorithm | pbe = PBEDefault ) const |
Export the key in Distinguished Encoding Rules (DER) format.
- Parameters
-
passphrase the pass phrase to use to protect the key pbe the symmetric encryption algorithm to use to protect the key
- See also
- fromDER provides an inverse of toDER, converting the DER encoded key back to a PrivateKey
◆ toDH()
DHPrivateKey QCA::PrivateKey::toDH | ( | ) | const |
Interpret / convert the key to a Diffie-Hellman key.
◆ toDSA()
DSAPrivateKey QCA::PrivateKey::toDSA | ( | ) | const |
Interpret / convert the key to a DSA key.
◆ toPEM()
QString QCA::PrivateKey::toPEM | ( | const SecureArray & | passphrase = SecureArray(), |
PBEAlgorithm | pbe = PBEDefault ) const |
Export the key in Privacy Enhanced Mail (PEM) format.
- Parameters
-
passphrase the pass phrase to use to protect the key pbe the symmetric encryption algorithm to use to protect the key
- See also
- toPEMFile provides a convenient way to save the PEM encoded key to a file
- fromPEM provides an inverse of toPEM, converting the PEM encoded key back to a PrivateKey
◆ toPEMFile()
bool QCA::PrivateKey::toPEMFile | ( | const QString & | fileName, |
const SecureArray & | passphrase = SecureArray(), | ||
PBEAlgorithm | pbe = PBEDefault ) const |
Export the key in Privacy Enhanced Mail (PEM) format to a file.
- Parameters
-
fileName the name (and path, if required) that the key should be exported to. passphrase the pass phrase to use to protect the key pbe the symmetric encryption algorithm to use to protect the key
- Returns
- true if the export succeeds
- See also
- toPEM provides a convenient way to save the PEM encoded key to a file
- fromPEM provides an inverse of toPEM, converting the PEM encoded key back to a PrivateKey
- Examples
- rsatest.cpp.
◆ toRSA()
RSAPrivateKey QCA::PrivateKey::toRSA | ( | ) | const |
Interpret / convert the key to an RSA key.
◆ update()
void QCA::PrivateKey::update | ( | const MemoryRegion & | a | ) |
Update the signature process.
- Parameters
-
a the message to use to update the signature
- Note
- This synchronous operation may require event handling, and so it must not be called from the same thread as an EventHandler.
- Examples
- rsatest.cpp.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:01:48 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.