QCA::CertificateChain
#include <QtCrypto>
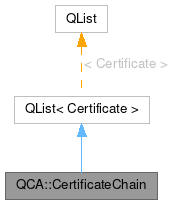
Public Member Functions | |
CertificateChain () | |
CertificateChain (const Certificate &primary) | |
CertificateChain | complete (const QList< Certificate > &issuers=QList< Certificate >(), Validity *result=nullptr) const |
const Certificate & | primary () const |
Validity | validate (const CertificateCollection &trusted, const QList< CRL > &untrusted_crls=QList< CRL >(), UsageMode u=UsageAny, ValidateFlags vf=ValidateAll) const |
![]() | |
QList (const QList< T > &other) | |
QList (InputIterator first, InputIterator last) | |
QList (QList< T > &&other) | |
QList (qsizetype size) | |
QList (qsizetype size, parameter_type value) | |
QList (std::initializer_list< T > args) | |
void | append (const QList< T > &value) |
void | append (parameter_type value) |
void | append (QList< T > &&value) |
void | append (rvalue_ref value) |
const_reference | at (qsizetype i) const const |
reference | back () |
const_reference | back () const const |
iterator | begin () |
const_iterator | begin () const const |
qsizetype | capacity () const const |
const_iterator | cbegin () const const |
const_iterator | cend () const const |
void | clear () |
const_iterator | constBegin () const const |
const_pointer | constData () const const |
const_iterator | constEnd () const const |
const T & | constFirst () const const |
const T & | constLast () const const |
bool | contains (const AT &value) const const |
qsizetype | count () const const |
qsizetype | count (const AT &value) const const |
const_reverse_iterator | crbegin () const const |
const_reverse_iterator | crend () const const |
pointer | data () |
const_pointer | data () const const |
iterator | emplace (const_iterator before, Args &&... args) |
iterator | emplace (qsizetype i, Args &&... args) |
reference | emplace_back (Args &&... args) |
reference | emplaceBack (Args &&... args) |
bool | empty () const const |
iterator | end () |
const_iterator | end () const const |
bool | endsWith (parameter_type value) const const |
iterator | erase (const_iterator begin, const_iterator end) |
iterator | erase (const_iterator pos) |
qsizetype | erase (QList< T > &list, const AT &t) |
qsizetype | erase_if (QList< T > &list, Predicate pred) |
QList< T > & | fill (parameter_type value, qsizetype size) |
T & | first () |
const T & | first () const const |
QList< T > | first (qsizetype n) const const |
reference | front () |
const_reference | front () const const |
qsizetype | indexOf (const AT &value, qsizetype from) const const |
iterator | insert (const_iterator before, parameter_type value) |
iterator | insert (const_iterator before, qsizetype count, parameter_type value) |
iterator | insert (const_iterator before, rvalue_ref value) |
iterator | insert (qsizetype i, parameter_type value) |
iterator | insert (qsizetype i, qsizetype count, parameter_type value) |
iterator | insert (qsizetype i, rvalue_ref value) |
bool | isEmpty () const const |
T & | last () |
const T & | last () const const |
QList< T > | last (qsizetype n) const const |
qsizetype | lastIndexOf (const AT &value, qsizetype from) const const |
qsizetype | length () const const |
QList< T > | mid (qsizetype pos, qsizetype length) const const |
void | move (qsizetype from, qsizetype to) |
bool | operator!= (const QList< T > &other) const const |
QList< T > | operator+ (const QList< T > &other) && |
QList< T > | operator+ (const QList< T > &other) const &const |
QList< T > | operator+ (QList< T > &&other) && |
QList< T > | operator+ (QList< T > &&other) const &const |
QList< T > & | operator+= (const QList< T > &other) |
QList< T > & | operator+= (parameter_type value) |
QList< T > & | operator+= (QList< T > &&other) |
QList< T > & | operator+= (rvalue_ref value) |
bool | operator< (const QList< T > &other) const const |
QList< T > & | operator<< (const QList< T > &other) |
QList< T > & | operator<< (parameter_type value) |
QDataStream & | operator<< (QDataStream &out, const QList< T > &list) |
QList< T > & | operator<< (QList< T > &&other) |
QList< T > & | operator<< (rvalue_ref value) |
bool | operator<= (const QList< T > &other) const const |
QList< T > & | operator= (const QList< T > &other) |
QList< T > & | operator= (QList< T > &&other) |
QList< T > & | operator= (std::initializer_list< T > args) |
bool | operator== (const QList< T > &other) const const |
bool | operator> (const QList< T > &other) const const |
bool | operator>= (const QList< T > &other) const const |
QDataStream & | operator>> (QDataStream &in, QList< T > &list) |
reference | operator[] (qsizetype i) |
const_reference | operator[] (qsizetype i) const const |
void | pop_back () |
void | pop_front () |
void | prepend (parameter_type value) |
void | prepend (rvalue_ref value) |
void | push_back (parameter_type value) |
void | push_back (rvalue_ref value) |
void | push_front (parameter_type value) |
void | push_front (rvalue_ref value) |
size_t | qHash (const QList< T > &key, size_t seed) |
reverse_iterator | rbegin () |
const_reverse_iterator | rbegin () const const |
void | remove (qsizetype i, qsizetype n) |
qsizetype | removeAll (const AT &t) |
void | removeAt (qsizetype i) |
void | removeFirst () |
qsizetype | removeIf (Predicate pred) |
void | removeLast () |
bool | removeOne (const AT &t) |
reverse_iterator | rend () |
const_reverse_iterator | rend () const const |
void | replace (qsizetype i, parameter_type value) |
void | replace (qsizetype i, rvalue_ref value) |
void | reserve (qsizetype size) |
void | resize (qsizetype size) |
void | resize (qsizetype size, parameter_type c) |
void | shrink_to_fit () |
qsizetype | size () const const |
QList< T > | sliced (qsizetype pos) const const |
QList< T > | sliced (qsizetype pos, qsizetype n) const const |
void | squeeze () |
bool | startsWith (parameter_type value) const const |
void | swap (QList< T > &other) |
void | swapItemsAt (qsizetype i, qsizetype j) |
T | takeAt (qsizetype i) |
value_type | takeFirst () |
value_type | takeLast () |
QList< T > | toList () const const |
QList< T > | toVector () const const |
T | value (qsizetype i) const const |
T | value (qsizetype i, parameter_type defaultValue) const const |
Additional Inherited Members | |
![]() | |
QList< T > | fromList (const QList< T > &list) |
QList< T > | fromVector (const QList< T > &list) |
![]() | |
typedef | const_pointer |
typedef | const_reference |
typedef | const_reverse_iterator |
typedef | ConstIterator |
typedef | difference_type |
typedef | Iterator |
typedef | parameter_type |
typedef | pointer |
typedef | reference |
typedef | reverse_iterator |
typedef | rvalue_ref |
typedef | size_type |
typedef | value_type |
Detailed Description
A chain of related Certificates.
CertificateChain is a list (a QList) of certificates that are related by the signature from one to another. If Certificate C signs Certificate B, and Certificate B signs Certificate A, then C, B and A form a chain.
The normal use of a CertificateChain is from a end-user Certificate (called the primary, equivalent to QList::first()) through some intermediate Certificates to some other Certificate (QList::last()), which might be a root Certificate Authority, but does not need to be.
You can build up the chain using normal QList operations, such as QList::append().
- See also
- QCA::CertificateCollection for an alternative way to represent a group of Certificates that do not necessarily have a chained relationship.
- Examples
- publickeyexample.cpp.
Definition at line 1225 of file qca_cert.h.
Constructor & Destructor Documentation
◆ CertificateChain() [1/2]
|
inline |
Create an empty certificate chain.
Definition at line 1231 of file qca_cert.h.
◆ CertificateChain() [2/2]
|
inline |
Create a certificate chain, starting at the specified certificate.
- Parameters
-
primary the end-user certificate that forms one end of the chain
Definition at line 1241 of file qca_cert.h.
Member Function Documentation
◆ complete()
|
inline |
Complete a certificate chain for the primary certificate, using the rest of the certificates in the chain object, as well as those in issuers, as possible issuers in the chain.
If there are issuers missing, then the chain might be incomplete (at the worst case, if no issuers exist for the primary certificate, then the resulting chain will consist of just the primary certificate). Use the result argument to find out if there was a problem during completion. A result of ValidityGood means the chain was completed successfully.
The newly constructed CertificateChain is returned.
If the certificate chain is empty, then this will return an empty CertificateChain object.
- Parameters
-
issuers a pool of issuers to draw from as necessary result the result of the completion operation
- Note
- This function may block
- See also
- validate
Definition at line 1309 of file qca_cert.h.
◆ primary()
|
inline |
Return the primary (end-user) Certificate.
Definition at line 1249 of file qca_cert.h.
◆ validate()
|
inline |
Check the validity of a certificate chain.
- Parameters
-
trusted a collection of trusted certificates untrusted_crls a list of additional CRLs, not necessarily trusted u the use required for the primary certificate vf the conditions to validate
- Note
- This function may block
- See also
- Certificate::validate()
Definition at line 1299 of file qca_cert.h.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:01:48 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.