QCA::Cipher
#include <QtCrypto>
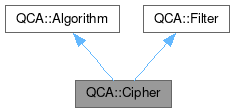
Public Types | |
enum | Mode { CBC , CFB , ECB , OFB , CTR , GCM , CCM } |
enum | Padding { DefaultPadding , NoPadding , PKCS7 } |
Static Public Member Functions | |
static QStringList | supportedTypes (const QString &provider=QString()) |
static QString | withAlgorithms (const QString &cipherType, Mode modeType, Padding paddingType) |
Additional Inherited Members | |
![]() | |
Algorithm () | |
Algorithm (const QString &type, const QString &provider) | |
Detailed Description
General class for cipher (encryption / decryption) algorithms.
Cipher is the class for the various algorithms that perform low level encryption and decryption within QCA.
AES128, AES192 and AES256 are recommended for new applications.
Standard names for ciphers are:
- Blowfish - "blowfish"
- TripleDES - "tripledes"
- DES - "des"
- AES128 - "aes128"
- AES192 - "aes192"
- AES256 - "aes256"
- CAST5 (CAST-128) - "cast5"
When checking for the availability of a particular kind of cipher operation (e.g. AES128 in CBC mode with PKCS7 padding), you append the mode and padding type (in that example "aes128-cbc-pkcs7"). CFB and OFB modes don't use padding, so they are always just the cipher name followed by the mode (e.g. "blowfish-cfb" or "aes192-ofb"). If you are not using padding with CBC mode (i.e. you are ensuring block size operations yourself), just use the cipher name followed by "-cbc" (e.g. "blowfish-cbc" or "aes256-cbc").
- Examples
- aes-cmac.cpp, and ciphertest.cpp.
Definition at line 581 of file qca_basic.h.
Member Enumeration Documentation
◆ Mode
enum QCA::Cipher::Mode |
Mode settings for cipher algorithms.
- Note
- ECB is almost never what you want, unless you are trying to implement a Cipher variation that is not supported by QCA.
Enumerator | |
---|---|
CBC | operate in Cipher Block Chaining mode |
CFB | operate in Cipher FeedBack mode |
ECB | operate in Electronic Code Book mode |
OFB | operate in Output FeedBack Mode |
CTR | operate in CounTer Mode |
GCM | operate in Galois Counter Mode |
CCM | operate in Counter with CBC-MAC |
Definition at line 591 of file qca_basic.h.
◆ Padding
enum QCA::Cipher::Padding |
Padding variations for cipher algorithms.
See the Padding description for more details on padding schemes.
Enumerator | |
---|---|
DefaultPadding | Default for cipher-mode. |
NoPadding | Do not use padding. |
PKCS7 | Pad using the scheme in PKCS#7. |
Definition at line 608 of file qca_basic.h.
Constructor & Destructor Documentation
◆ Cipher() [1/3]
QCA::Cipher::Cipher | ( | const QString & | type, |
Mode | mode, | ||
Padding | pad = DefaultPadding, | ||
Direction | dir = Encode, | ||
const SymmetricKey & | key = SymmetricKey(), | ||
const InitializationVector & | iv = InitializationVector(), | ||
const QString & | provider = QString() ) |
Standard constructor.
- Parameters
-
type the name of the cipher specialisation to use (e.g. "aes128") mode the operating Mode to use (e.g. QCA::Cipher::CBC) pad the type of Padding to use dir the Direction that this Cipher should use (Encode for encryption, Decode for decryption) key the SymmetricKey array that is the key iv the InitializationVector to use (not used for ECB mode) provider the name of the Provider to use
- Note
- Padding only applies to CBC and ECB modes. CFB and OFB ciphertext is always the length of the plaintext.
◆ Cipher() [2/3]
QCA::Cipher::Cipher | ( | const QString & | type, |
Mode | mode, | ||
Padding | pad, | ||
Direction | dir, | ||
const SymmetricKey & | key, | ||
const InitializationVector & | iv, | ||
const AuthTag & | tag, | ||
const QString & | provider = QString() ) |
Standard constructor.
- Parameters
-
type the name of the cipher specialisation to use (e.g. "aes128") mode the operating Mode to use (e.g. QCA::Cipher::CBC) pad the type of Padding to use dir the Direction that this Cipher should use (Encode for encryption, Decode for decryption) key the SymmetricKey array that is the key iv the InitializationVector to use (not used for ECB mode) tag the AuthTag to use (only for GCM and CCM modes) provider the name of the Provider to use
- Note
- Padding only applies to CBC and ECB modes. CFB and OFB ciphertext is always the length of the plaintext.
◆ Cipher() [3/3]
QCA::Cipher::Cipher | ( | const Cipher & | from | ) |
Standard copy constructor.
- Parameters
-
from the Cipher to copy state from
Member Function Documentation
◆ blockSize()
int QCA::Cipher::blockSize | ( | ) | const |
return the block size for the cipher object
◆ clear()
|
overridevirtual |
reset the cipher object, to allow re-use
Implements QCA::Filter.
◆ direction()
Direction QCA::Cipher::direction | ( | ) | const |
Return the cipher direction.
◆ final()
|
overridevirtual |
complete the block of data, padding as required, and returning the completed block
Implements QCA::Filter.
- Examples
- ciphertest.cpp.
◆ keyLength()
KeyLength QCA::Cipher::keyLength | ( | ) | const |
Return acceptable key lengths.
◆ mode()
Mode QCA::Cipher::mode | ( | ) | const |
Return the cipher mode.
◆ ok()
|
overridevirtual |
Test if an update() or final() call succeeded.
- Returns
- true if the previous call succeeded
Implements QCA::Filter.
- Examples
- ciphertest.cpp.
◆ operator=()
Assignment operator.
- Parameters
-
from the Cipher to copy state from
◆ padding()
Padding QCA::Cipher::padding | ( | ) | const |
Return the cipher padding type.
◆ setup() [1/2]
void QCA::Cipher::setup | ( | Direction | dir, |
const SymmetricKey & | key, | ||
const InitializationVector & | iv, | ||
const AuthTag & | tag ) |
Reset / reconfigure the Cipher.
You can use this to re-use an existing Cipher, rather than creating a new object with a slightly different configuration.
- Parameters
-
dir the Direction that this Cipher should use (Encode for encryption, Decode for decryption) key the SymmetricKey array that is the key iv the InitializationVector to use (not used for ECB Mode) tag the AuthTag to use (only for GCM and CCM modes)
- Note
- You should not leave iv empty for any Mode except ECB.
◆ setup() [2/2]
void QCA::Cipher::setup | ( | Direction | dir, |
const SymmetricKey & | key, | ||
const InitializationVector & | iv = InitializationVector() ) |
Reset / reconfigure the Cipher.
You can use this to re-use an existing Cipher, rather than creating a new object with a slightly different configuration.
- Parameters
-
dir the Direction that this Cipher should use (Encode for encryption, Decode for decryption) key the SymmetricKey array that is the key iv the InitializationVector to use (not used for ECB Mode)
- Note
- You should not leave iv empty for any Mode except ECB.
- Examples
- ciphertest.cpp.
◆ supportedTypes()
|
static |
Returns a list of all of the cipher types available.
- Parameters
-
provider the name of the provider to get a list from, if one provider is required. If not specified, available cipher types from all providers will be returned.
◆ tag()
AuthTag QCA::Cipher::tag | ( | ) | const |
return the authentication tag for the cipher object
◆ type()
QString QCA::Cipher::type | ( | ) | const |
Return the cipher type.
◆ update()
|
overridevirtual |
pass in a byte array of data, which will be encrypted or decrypted (according to the Direction that was set in the constructor or in setup() ) and returned.
- Parameters
-
a the array of data to encrypt / decrypt
Implements QCA::Filter.
- Examples
- ciphertest.cpp.
◆ validKeyLength()
bool QCA::Cipher::validKeyLength | ( | int | n | ) | const |
Test if a key length is valid for the cipher algorithm.
- Parameters
-
n the key length in bytes
- Returns
- true if the key would be valid for the current algorithm
◆ withAlgorithms()
|
static |
Construct a Cipher type string.
- Parameters
-
cipherType the name of the algorithm (eg AES128, DES) modeType the mode to operate the cipher in (eg QCA::CBC, QCA::CFB) paddingType the padding required (eg QCA::NoPadding, QCA::PCKS7)
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Feb 28 2025 11:49:14 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.