QCA::SecureMessage
#include <QtCrypto>
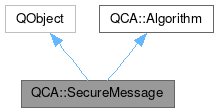
Public Types | |
enum | Error { ErrorPassphrase , ErrorFormat , ErrorSignerExpired , ErrorSignerInvalid , ErrorEncryptExpired , ErrorEncryptUntrusted , ErrorEncryptInvalid , ErrorNeedCard , ErrorCertKeyMismatch , ErrorUnknown , ErrorSignerRevoked , ErrorSignatureExpired , ErrorEncryptRevoked } |
enum | Format { Binary , Ascii } |
enum | SignMode { Message , Clearsign , Detached } |
enum | Type { OpenPGP , CMS } |
Signals | |
void | bytesWritten (int bytes) |
void | finished () |
void | readyRead () |
Public Member Functions | |
SecureMessage (SecureMessageSystem *system) | |
bool | bundleSignerEnabled () const |
int | bytesAvailable () const |
bool | canClearsign () const |
bool | canSignAndEncrypt () const |
bool | canSignMultiple () const |
QString | diagnosticText () const |
void | end () |
Error | errorCode () const |
Format | format () const |
QString | hashName () const |
QByteArray | read () |
SecureMessageKeyList | recipientKeys () const |
void | reset () |
void | setBundleSignerEnabled (bool b) |
void | setFormat (Format f) |
void | setRecipient (const SecureMessageKey &key) |
void | setRecipients (const SecureMessageKeyList &keys) |
void | setSigner (const SecureMessageKey &key) |
void | setSigners (const SecureMessageKeyList &keys) |
void | setSMIMEAttributesEnabled (bool b) |
QByteArray | signature () const |
SecureMessageSignature | signer () const |
SecureMessageKeyList | signerKeys () const |
SecureMessageSignatureList | signers () const |
bool | smimeAttributesEnabled () const |
void | startDecrypt () |
void | startEncrypt () |
void | startSign (SignMode m=Message) |
void | startSignAndEncrypt () |
void | startVerify (const QByteArray &detachedSig=QByteArray()) |
bool | success () const |
Type | type () const |
void | update (const QByteArray &in) |
bool | verifySuccess () const |
bool | waitForFinished (int msecs=30000) |
bool | wasSigned () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
Algorithm (const Algorithm &from) | |
void | change (const QString &type, const QString &provider) |
void | change (Provider::Context *c) |
Provider::Context * | context () |
const Provider::Context * | context () const |
Algorithm & | operator= (const Algorithm &from) |
Provider * | provider () const |
Provider::Context * | takeContext () |
QString | type () const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
Algorithm () | |
Algorithm (const QString &type, const QString &provider) | |
Detailed Description
Class representing a secure message.
SecureMessage presents a unified interface for working with both OpenPGP and CMS (S/MIME) messages. Prepare the object by calling setFormat(), setRecipient(), and setSigner() as necessary, and then begin the operation by calling an appropriate 'start' function, such as startSign().
Here is an example of how to perform a Clearsign operation using PGP:
Performing a CMS sign operation is similar. Simply set up the SecureMessageKey with a Certificate instead of a PGPKey, and operate on a CMS object instead of an OpenPGP object.
- Examples
- publickeyexample.cpp.
Definition at line 319 of file qca_securemessage.h.
Member Enumeration Documentation
◆ Error
Errors for secure messages.
Definition at line 354 of file qca_securemessage.h.
◆ Format
Formats for secure messages.
Enumerator | |
---|---|
Binary | DER/binary. |
Ascii | PEM/ascii-armored. |
Definition at line 345 of file qca_securemessage.h.
◆ SignMode
The type of message signature.
Enumerator | |
---|---|
Message | the message includes the signature |
Clearsign | the message is clear signed |
Detached | the signature is detached |
Definition at line 335 of file qca_securemessage.h.
◆ Type
The type of secure message.
Enumerator | |
---|---|
OpenPGP | a Pretty Good Privacy message |
CMS | a Cryptographic Message Syntax message |
Definition at line 326 of file qca_securemessage.h.
Constructor & Destructor Documentation
◆ SecureMessage()
QCA::SecureMessage::SecureMessage | ( | SecureMessageSystem * | system | ) |
Create a new secure message.
This constructor uses an existing SecureMessageSystem object (for example, an OpenPGP or CMS object) to generate a specific kind of secure message.
- Parameters
-
system a pre-existing and configured SecureMessageSystem object
Member Function Documentation
◆ bundleSignerEnabled()
bool QCA::SecureMessage::bundleSignerEnabled | ( | ) | const |
Returns true if bundling of the signer certificate chain is enabled.
◆ bytesAvailable()
int QCA::SecureMessage::bytesAvailable | ( | ) | const |
The number of bytes available to be read.
◆ bytesWritten
|
signal |
This signal is emitted when data has been accepted by the message processor.
- Parameters
-
bytes the number of bytes written
◆ canClearsign()
bool QCA::SecureMessage::canClearsign | ( | ) | const |
True if the SecureMessageSystem can clearsign messages.
- Note
- CMS cannot clearsign - this is normally only available for PGP
◆ canSignAndEncrypt()
bool QCA::SecureMessage::canSignAndEncrypt | ( | ) | const |
True if the SecureMessageSystem can both sign and encrypt (in the same operation).
◆ canSignMultiple()
bool QCA::SecureMessage::canSignMultiple | ( | ) | const |
Test if the message type supports multiple (parallel) signatures.
- Returns
- true if the secure message support multiple parallel signatures
- Note
- PGP cannot do this - it is primarily a CMS feature
◆ diagnosticText()
QString QCA::SecureMessage::diagnosticText | ( | ) | const |
Returns a log of technical information about the operation, which may be useful for presenting to the user in an advanced error dialog.
◆ end()
void QCA::SecureMessage::end | ( | ) |
Complete an operation.
You need to call this method after you have processed the message (which you pass in as the argument to update().
- Note
- the results of the operation are not available as soon as this method returns. You need to wait for the finished() signal, or use waitForFinished().
- Examples
- publickeyexample.cpp.
◆ errorCode()
Error QCA::SecureMessage::errorCode | ( | ) | const |
◆ finished
|
signal |
This signal is emitted when the message is fully processed.
◆ format()
Format QCA::SecureMessage::format | ( | ) | const |
Return the format type set for this message.
◆ hashName()
QString QCA::SecureMessage::hashName | ( | ) | const |
The name of the hash used for the signature process.
◆ read()
QByteArray QCA::SecureMessage::read | ( | ) |
Read the available data.
- Note
- For detached signatures, you don't get anything back using this method. Use signature() to get the detached signature().
- Examples
- publickeyexample.cpp.
◆ readyRead
|
signal |
This signal is emitted when there is some data to read.
Typically you connect this signal to a slot that does a read() of the available data.
- Note
- This signal does not mean that the processing of a message is necessarily complete - see finished().
◆ recipientKeys()
SecureMessageKeyList QCA::SecureMessage::recipientKeys | ( | ) | const |
Return the recipient(s) set for this message with setRecipient() or setRecipients()
◆ reset()
void QCA::SecureMessage::reset | ( | ) |
Reset the object state to that of original construction.
Now a new operation can be performed immediately.
◆ setBundleSignerEnabled()
void QCA::SecureMessage::setBundleSignerEnabled | ( | bool | b | ) |
For CMS only, this will bundle the signer certificate chain into the message.
This allows a message to be verified on its own, without the need to have obtained the signer's certificate in advance. Email clients using S/MIME often bundle the signer, greatly simplifying key management.
This behavior is enabled by default.
- Parameters
-
b whether to bundle (if true) or not (false)
◆ setFormat()
void QCA::SecureMessage::setFormat | ( | Format | f | ) |
◆ setRecipient()
void QCA::SecureMessage::setRecipient | ( | const SecureMessageKey & | key | ) |
Set the recipient for an encrypted message.
- Parameters
-
key the recipient's key
- See also
- setRecipients
- Examples
- publickeyexample.cpp.
◆ setRecipients()
void QCA::SecureMessage::setRecipients | ( | const SecureMessageKeyList & | keys | ) |
Set the list of recipients for an encrypted message.
For a list with one item, this has the same effect as setRecipient.
- Parameters
-
keys the recipients' key
- See also
- setRecipient
◆ setSigner()
void QCA::SecureMessage::setSigner | ( | const SecureMessageKey & | key | ) |
Set the signer for a signed message.
This is used for both creating signed messages as well as for verifying CMS messages that have no signer bundled.
- Parameters
-
key the key associated with the signer
- See also
- setSigners
◆ setSigners()
void QCA::SecureMessage::setSigners | ( | const SecureMessageKeyList & | keys | ) |
◆ setSMIMEAttributesEnabled()
void QCA::SecureMessage::setSMIMEAttributesEnabled | ( | bool | b | ) |
For CMS only, this will put extra attributes into the message related to S/MIME, such as the preferred type of algorithm to use in replies.
The attributes used are decided by the provider.
This behavior is enabled by default.
- Parameters
-
b whether to embed extra attribues (if true) or not (false)
◆ signature()
QByteArray QCA::SecureMessage::signature | ( | ) | const |
The signature for the message.
This is only used for Detached signatures. For other message types, you get the message and signature together using read().
◆ signer()
SecureMessageSignature QCA::SecureMessage::signer | ( | ) | const |
Information on the signer for the message.
◆ signerKeys()
SecureMessageKeyList QCA::SecureMessage::signerKeys | ( | ) | const |
Return the signer(s) set for this message with setSigner() or setSigners()
◆ signers()
SecureMessageSignatureList QCA::SecureMessage::signers | ( | ) | const |
Information on the signers for the message.
This is only meaningful if the message type supports multiple signatures (see canSignMultiple() for a suitable test).
◆ smimeAttributesEnabled()
bool QCA::SecureMessage::smimeAttributesEnabled | ( | ) | const |
Returns true if inclusion of S/MIME attributes is enabled.
◆ startDecrypt()
void QCA::SecureMessage::startDecrypt | ( | ) |
Start an decryption operation.
You will normally use this with some code along these lines:
Each update() may (or may not) result in some decrypted data, as indicated by the readyRead() signal being emitted. Alternatively, you can wait until the whole message is available (using either waitForFinished(), or the finished() signal). The decrypted message can then be read using the read() method.
- Note
- If decrypted result is also signed (not for CMS), then the signature will be verified during this operation.
◆ startEncrypt()
void QCA::SecureMessage::startEncrypt | ( | ) |
Start an encryption operation.
You will normally use this with some code along these lines:
Each update() may (or may not) result in some encrypted data, as indicated by the readyRead() signal being emitted. Alternatively, you can wait until the whole message is available (using either waitForFinished(), or use the finished() signal. The encrypted message can then be read using the read() method.
- Examples
- publickeyexample.cpp.
◆ startSign()
Start a signing operation.
You will normally use this with some code along these lines:
For Detached signatures, you won't get any results until the whole process is done - you either waitForFinished(), or use the finished() signal, to figure out when you can get the signature (using the signature() method, not using read()). For other formats, you can use the readyRead() signal to determine when there may be part of a signed message to read().
- Parameters
-
m the mode that will be used to generate the signature
◆ startSignAndEncrypt()
void QCA::SecureMessage::startSignAndEncrypt | ( | ) |
Start a combined signing and encrypting operation.
You use this in the same way as startEncrypt().
- Note
- This may not be possible (e.g. CMS cannot do this) - see canSignAndEncrypt() for a suitable test.
◆ startVerify()
void QCA::SecureMessage::startVerify | ( | const QByteArray & | detachedSig = QByteArray() | ) |
Start a verification operation.
- Parameters
-
detachedSig the detached signature to verify. Do not pass a signature for other signature types.
◆ success()
bool QCA::SecureMessage::success | ( | ) | const |
Indicates whether or not the operation was successful or failed.
If this function returns false, then the reason for failure can be obtained with errorCode().
- See also
- errorCode
- diagnosticText
- Examples
- publickeyexample.cpp.
◆ type()
◆ update()
void QCA::SecureMessage::update | ( | const QByteArray & | in | ) |
Process a message (or the next part of a message) in the current operation.
You need to have already set up the message (startEncrypt(), startDecrypt(), startSign(), startSignAndEncrypt() and startVerify()) before calling this method.
- Parameters
-
in the data to process
- Examples
- publickeyexample.cpp.
◆ verifySuccess()
bool QCA::SecureMessage::verifySuccess | ( | ) | const |
Verify that the message signature is correct.
- Returns
- true if the signature is valid for the message, otherwise return false
◆ waitForFinished()
bool QCA::SecureMessage::waitForFinished | ( | int | msecs = 30000 | ) |
Block until the operation (encryption, decryption, signing or verifying) completes.
- Parameters
-
msecs the number of milliseconds to wait for the operation to complete. Pass -1 to wait indefinitely.
- Note
- You should not use this in GUI applications where the blocking behaviour looks like a hung application. Instead, connect the finished() signal to a slot that handles the results.
- This synchronous operation may require event handling, and so it must not be called from the same thread as an EventHandler.
- Examples
- publickeyexample.cpp.
◆ wasSigned()
bool QCA::SecureMessage::wasSigned | ( | ) | const |
Test if the message was signed.
This is true for OpenPGP if the decrypted message was also signed.
- Returns
- true if the message was signed.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 18 2025 12:07:58 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.