QCA::KeyStore
#include <QtCrypto>
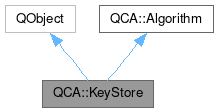
Public Types | |
enum | Type { System , User , Application , SmartCard , PGPKeyring } |
Signals | |
void | entryRemoved (bool success) |
void | entryWritten (const QString &entryId) |
void | unavailable () |
void | updated () |
Public Member Functions | |
KeyStore (const QString &id, KeyStoreManager *keyStoreManager) | |
QList< KeyStoreEntry > | entryList () const |
bool | holdsIdentities () const |
bool | holdsPGPPublicKeys () const |
bool | holdsTrustedCertificates () const |
QString | id () const |
bool | isReadOnly () const |
bool | isValid () const |
QString | name () const |
bool | removeEntry (const QString &id) |
void | startAsynchronousMode () |
Type | type () const |
QString | writeEntry (const Certificate &cert) |
QString | writeEntry (const CRL &crl) |
QString | writeEntry (const KeyBundle &kb) |
QString | writeEntry (const PGPKey &key) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
Algorithm (const Algorithm &from) | |
void | change (const QString &type, const QString &provider) |
void | change (Provider::Context *c) |
Provider::Context * | context () |
const Provider::Context * | context () const |
Algorithm & | operator= (const Algorithm &from) |
Provider * | provider () const |
Provider::Context * | takeContext () |
QString | type () const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
Algorithm () | |
Algorithm (const QString &type, const QString &provider) | |
Detailed Description
General purpose key storage object.
Examples of use of this are:
- systemstore: System TrustedCertificates
- accepted self-signed: Application TrustedCertificates
- apple keychain: User Identities
- smartcard: SmartCard Identities
- gnupg: PGPKeyring Identities,PGPPublicKeys
- Note
- there can be multiple KeyStore objects referring to the same id
- when a KeyStore is constructed, it refers to a given id (deviceId) and internal contextId. if the context goes away, the KeyStore becomes invalid (isValid() == false), and unavailable() is emitted. even if the device later reappears, the KeyStore remains invalid. a new KeyStore will have to be created to use the device again.
Definition at line 416 of file qca_keystore.h.
Member Enumeration Documentation
◆ Type
enum QCA::KeyStore::Type |
The type of keystore.
Definition at line 423 of file qca_keystore.h.
Constructor & Destructor Documentation
◆ KeyStore()
QCA::KeyStore::KeyStore | ( | const QString & | id, |
KeyStoreManager * | keyStoreManager ) |
Obtain a specific KeyStore.
- Parameters
-
id the identification for the key store keyStoreManager the parent manager for this keystore
Member Function Documentation
◆ entryList()
QList< KeyStoreEntry > QCA::KeyStore::entryList | ( | ) | const |
A list of the KeyStoreEntry objects in this store.
- Note
- This synchronous operation may require event handling, and so it must not be called from the same thread as an EventHandler (this is not a concern if asynchronous mode is enabled).
- See also
- startAsynchronousMode
◆ entryRemoved
|
signal |
Emitted when an entry has been removed, in asynchronous mode.
- Parameters
-
success indicates if the removal succeeded (true) or not (false).
◆ entryWritten
|
signal |
Emitted when an entry has been written, in asynchronous mode.
- Parameters
-
entryId is the newly written entry id on success, or an empty string if the write failed.
◆ holdsIdentities()
bool QCA::KeyStore::holdsIdentities | ( | ) | const |
◆ holdsPGPPublicKeys()
bool QCA::KeyStore::holdsPGPPublicKeys | ( | ) | const |
test if the KeyStore holds PGPPublicKey objects
◆ holdsTrustedCertificates()
bool QCA::KeyStore::holdsTrustedCertificates | ( | ) | const |
test if the KeyStore holds trusted certificates (and CRLs)
◆ id()
◆ isReadOnly()
bool QCA::KeyStore::isReadOnly | ( | ) | const |
◆ isValid()
bool QCA::KeyStore::isValid | ( | ) | const |
◆ name()
◆ removeEntry()
bool QCA::KeyStore::removeEntry | ( | const QString & | id | ) |
Delete the a specified KeyStoreEntry from this KeyStore.
- Parameters
-
id the ID for the entry to be deleted
- Note
- This synchronous operation may require event handling, and so it must not be called from the same thread as an EventHandler (this is not a concern if asynchronous mode is enabled).
- See also
- startAsynchronousMode
◆ startAsynchronousMode()
void QCA::KeyStore::startAsynchronousMode | ( | ) |
Turns on asynchronous mode for this KeyStore instance.
Normally, entryList() and writeEntry() are blocking calls. However, if startAsynchronousMode() is called, then these functions will return immediately. entryList() will return with the latest known entries, or an empty list if none are known yet (in this mode, updated() will be emitted once the initial entries are known, even if the store has not actually been altered). writeEntry() will always return an empty string, and the entryWritten() signal indicates the result of a write.
◆ type()
◆ unavailable
|
signal |
Emitted when the KeyStore becomes unavailable.
◆ updated
|
signal |
◆ writeEntry() [1/4]
QString QCA::KeyStore::writeEntry | ( | const Certificate & | cert | ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters
-
cert the Certificate to add to the KeyStore
◆ writeEntry() [2/4]
◆ writeEntry() [3/4]
Add a entry to the KeyStore.
Returns the entryId of the written entry or an empty string on failure.
- Note
- This synchronous operation may require event handling, and so it must not be called from the same thread as an EventHandler (this is not a concern if asynchronous mode is enabled).
- See also
- startAsynchronousMode
◆ writeEntry() [4/4]
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:01:48 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.