QCA::KeyStoreEntry
#include <QtCrypto>
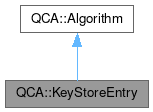
Public Types | |
enum | Type { TypeKeyBundle , TypeCertificate , TypeCRL , TypePGPSecretKey , TypePGPPublicKey } |
Public Member Functions | |
KeyStoreEntry () | |
KeyStoreEntry (const KeyStoreEntry &from) | |
KeyStoreEntry (const QString &serialized) | |
Certificate | certificate () const |
CRL | crl () const |
bool | ensureAccess () |
bool | ensureAvailable () |
QString | id () const |
bool | isAccessible () const |
bool | isAvailable () const |
bool | isNull () const |
KeyBundle | keyBundle () const |
QString | name () const |
KeyStoreEntry & | operator= (const KeyStoreEntry &from) |
PGPKey | pgpPublicKey () const |
PGPKey | pgpSecretKey () const |
QString | storeId () const |
QString | storeName () const |
QString | toString () const |
Type | type () const |
![]() | |
Algorithm (const Algorithm &from) | |
void | change (const QString &type, const QString &provider) |
void | change (Provider::Context *c) |
Provider::Context * | context () |
const Provider::Context * | context () const |
Algorithm & | operator= (const Algorithm &from) |
Provider * | provider () const |
Provider::Context * | takeContext () |
QString | type () const |
Static Public Member Functions | |
static KeyStoreEntry | fromString (const QString &serialized) |
Additional Inherited Members | |
![]() | |
Algorithm () | |
Algorithm (const QString &type, const QString &provider) | |
Detailed Description
Single entry in a KeyStore.
This is a container for any kind of object in a KeyStore (such as PGP keys, or X.509 certificates / private keys).
KeyStoreEntry objects are obtained through KeyStore or loaded from a serialized string format. The latter method requires a KeyStoreEntry obtained through KeyStore to be serialized for future loading. For example:
KeyStoreEntry objects may or may not be available. An entry is unavailable if it has a private content that is not present. The private content might exist on external hardware. To determine if an entry is available, call isAvailable(). To ensure an entry is available before performing a private key operation, call ensureAvailable. For example:
ensureAvailable() blocks and may cause hardware access, but if it completes successfully then you may use the entry's private content. It also means, in the case of a Smart Card token, that it is probably inserted.
To watch this entry asynchronously, you would do:
Unlike private content, public content is always usable even if the entry is not available. Serialized entry data contains all of the metadata necessary to reconstruct the public content.
Now, even though an entry may be available, it does not mean you have access to use it for operations. For example, even though a KeyBundle entry offered by a Smart Card may be available, as soon as you try to use the PrivateKey object for a signing operation, a PIN might be asked for. You can call ensureAccess() if you want to synchronously provide the PIN early on:
Note that you don't have to call ensureAvailable() before ensureAccess(). Calling the latter is enough to imply both.
After an application is configured to use a particular key, it is expected that its usual running procedure will be:
1) Construct KeyStoreEntry from the serialized data. 2) If the content object is not available, wait for it (with either ensureAvailable() or KeyStoreEntryWatcher). 3) Pass the content object(s) to a high level operation like TLS.
In this case, any PIN prompting and private key operations would be caused/handled from the TLS object. Omit step 2 and the private key operations might cause token prompting.
- Examples
- eventhandlerdemo.cpp.
Definition at line 140 of file qca_keystore.h.
Member Enumeration Documentation
◆ Type
The type of entry in the KeyStore.
Definition at line 146 of file qca_keystore.h.
Constructor & Destructor Documentation
◆ KeyStoreEntry() [1/3]
QCA::KeyStoreEntry::KeyStoreEntry | ( | ) |
Create an empty KeyStoreEntry.
◆ KeyStoreEntry() [2/3]
QCA::KeyStoreEntry::KeyStoreEntry | ( | const QString & | serialized | ) |
Create a passive KeyStoreEntry based on a serialized string.
- Parameters
-
serialized the string containing the keystore entry information
- See also
- fromString
◆ KeyStoreEntry() [3/3]
QCA::KeyStoreEntry::KeyStoreEntry | ( | const KeyStoreEntry & | from | ) |
Standard copy constructor.
- Parameters
-
from the source entry
Member Function Documentation
◆ certificate()
Certificate QCA::KeyStoreEntry::certificate | ( | ) | const |
If a Certificate is stored in this object, return that certificate.
◆ crl()
CRL QCA::KeyStoreEntry::crl | ( | ) | const |
◆ ensureAccess()
bool QCA::KeyStoreEntry::ensureAccess | ( | ) |
Like ensureAvailable, but will also ensure that the PIN is provided if needed.
- See also
- isAccessible
- ensureAvailable
- Note
- This synchronous operation may require event handling, and so it must not be called from the same thread as an EventHandler.
◆ ensureAvailable()
bool QCA::KeyStoreEntry::ensureAvailable | ( | ) |
Returns true if the entry is available, otherwise false.
Available means that any private content for this entry is present and ready for use. In the case of a smart card, this will ensure the card is inserted, and may invoke a token prompt.
Calling this function on an already available entry may cause the entry to be refreshed.
- See also
- isAvailable
- ensureAccess
- Note
- This function is blocking.
- This synchronous operation may require event handling, and so it must not be called from the same thread as an EventHandler.
◆ fromString()
|
static |
Load a passive entry by using a serialized string as input.
- Parameters
-
serialized the string containing the keystore entry information
- Returns
- the newly created KeyStoreEntry
◆ id()
QString QCA::KeyStoreEntry::id | ( | ) | const |
The ID associated with the key stored in this object.
◆ isAccessible()
bool QCA::KeyStoreEntry::isAccessible | ( | ) | const |
Test if the key is currently accessible.
This means that the private key part can be used at this time. For a smartcard, this means that all required operations (e.g. login / PIN entry) are completed.
If isAccessible() is true, then the key is necessarily available (i.e. isAvailable() is also true).
- See also
- ensureAccessible
- isAvailable
◆ isAvailable()
bool QCA::KeyStoreEntry::isAvailable | ( | ) | const |
Test if the key is available for use.
A key is considered available if the key's private content is present.
- See also
- ensureAvailable
- isAccessible
◆ isNull()
bool QCA::KeyStoreEntry::isNull | ( | ) | const |
Test if this key is empty (null)
◆ keyBundle()
KeyBundle QCA::KeyStoreEntry::keyBundle | ( | ) | const |
If a KeyBundle is stored in this object, return that bundle.
◆ name()
QString QCA::KeyStoreEntry::name | ( | ) | const |
The name associated with the key stored in this object.
◆ operator=()
KeyStoreEntry & QCA::KeyStoreEntry::operator= | ( | const KeyStoreEntry & | from | ) |
Standard assignment operator.
- Parameters
-
from the source entry
◆ pgpPublicKey()
PGPKey QCA::KeyStoreEntry::pgpPublicKey | ( | ) | const |
If the key stored in this object is either an public or private PGP key, extract the public key part of that PGP key.
◆ pgpSecretKey()
PGPKey QCA::KeyStoreEntry::pgpSecretKey | ( | ) | const |
If the key stored in this object is a private PGP key, return the contents of that key.
◆ storeId()
QString QCA::KeyStoreEntry::storeId | ( | ) | const |
The id of the KeyStore for this key object.
- See also
- KeyStore::id()
◆ storeName()
◆ toString()
QString QCA::KeyStoreEntry::toString | ( | ) | const |
Serialize into a string for use as a passive entry.
◆ type()
Type QCA::KeyStoreEntry::type | ( | ) | const |
Determine the type of key stored in this object.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:50:48 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.