KAutoSaveFile
#include <KAutoSaveFile>
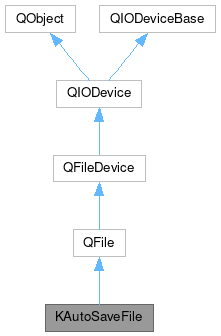
Public Member Functions | |
KAutoSaveFile (const QUrl &filename, QObject *parent=nullptr) | |
KAutoSaveFile (QObject *parent=nullptr) | |
~KAutoSaveFile () override | |
QUrl | managedFile () const |
bool | open (OpenMode openmode) override |
virtual void | releaseLock () |
void | setManagedFile (const QUrl &filename) |
![]() | |
QFile (const QString &name) | |
QFile (const QString &name, QObject *parent) | |
QFile (const std::filesystem::path &name) | |
QFile (const std::filesystem::path &name, QObject *parent) | |
QFile (QObject *parent) | |
bool | copy (const QString &newName) |
bool | copy (const std::filesystem::path &newName) |
bool | exists () const const |
virtual QString | fileName () const const override |
std::filesystem::path | filesystemFileName () const const |
std::filesystem::path | filesystemSymLinkTarget () const const |
bool | link (const QString &linkName) |
bool | link (const std::filesystem::path &newName) |
bool | moveToTrash () |
bool | open (FILE *fh, OpenMode mode, FileHandleFlags handleFlags) |
bool | open (int fd, OpenMode mode, FileHandleFlags handleFlags) |
bool | open (OpenMode mode, Permissions permissions) |
virtual Permissions | permissions () const const override |
bool | remove () |
bool | rename (const QString &newName) |
bool | rename (const std::filesystem::path &newName) |
virtual bool | resize (qint64 sz) override |
void | setFileName (const QString &name) |
void | setFileName (const std::filesystem::path &name) |
virtual bool | setPermissions (Permissions permissions) override |
virtual qint64 | size () const const override |
QString | symLinkTarget () const const |
![]() | |
virtual bool | atEnd () const const override |
virtual void | close () override |
FileError | error () const const |
QDateTime | fileTime (QFileDevice::FileTime time) const const |
bool | flush () |
int | handle () const const |
virtual bool | isSequential () const const override |
uchar * | map (qint64 offset, qint64 size, MemoryMapFlags flags) |
virtual qint64 | pos () const const override |
virtual bool | seek (qint64 pos) override |
bool | setFileTime (const QDateTime &newDate, QFileDevice::FileTime fileTime) |
bool | unmap (uchar *address) |
void | unsetError () |
![]() | |
QIODevice (QObject *parent) | |
void | aboutToClose () |
virtual qint64 | bytesAvailable () const const |
virtual qint64 | bytesToWrite () const const |
void | bytesWritten (qint64 bytes) |
virtual bool | canReadLine () const const |
void | channelBytesWritten (int channel, qint64 bytes) |
void | channelReadyRead (int channel) |
void | commitTransaction () |
int | currentReadChannel () const const |
int | currentWriteChannel () const const |
QString | errorString () const const |
bool | getChar (char *c) |
bool | isOpen () const const |
bool | isReadable () const const |
bool | isTextModeEnabled () const const |
bool | isTransactionStarted () const const |
bool | isWritable () const const |
virtual bool | open (QIODeviceBase::OpenMode mode) |
QIODeviceBase::OpenMode | openMode () const const |
qint64 | peek (char *data, qint64 maxSize) |
QByteArray | peek (qint64 maxSize) |
bool | putChar (char c) |
qint64 | read (char *data, qint64 maxSize) |
QByteArray | read (qint64 maxSize) |
QByteArray | readAll () |
int | readChannelCount () const const |
void | readChannelFinished () |
qint64 | readLine (char *data, qint64 maxSize) |
QByteArray | readLine (qint64 maxSize) |
void | readyRead () |
virtual bool | reset () |
void | rollbackTransaction () |
void | setCurrentReadChannel (int channel) |
void | setCurrentWriteChannel (int channel) |
void | setTextModeEnabled (bool enabled) |
qint64 | skip (qint64 maxSize) |
void | startTransaction () |
void | ungetChar (char c) |
virtual bool | waitForBytesWritten (int msecs) |
virtual bool | waitForReadyRead (int msecs) |
qint64 | write (const char *data) |
qint64 | write (const char *data, qint64 maxSize) |
qint64 | write (const QByteArray &data) |
int | writeChannelCount () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static QList< KAutoSaveFile * > | allStaleFiles (const QString &applicationName=QString()) |
static QList< KAutoSaveFile * > | staleFiles (const QUrl &url, const QString &applicationName=QString()) |
![]() | |
bool | copy (const QString &fileName, const QString &newName) |
QString | decodeName (const char *localFileName) |
QString | decodeName (const QByteArray &localFileName) |
QByteArray | encodeName (const QString &fileName) |
bool | exists (const QString &fileName) |
std::filesystem::path | filesystemSymLinkTarget (const std::filesystem::path &fileName) |
bool | link (const QString &fileName, const QString &linkName) |
bool | moveToTrash (const QString &fileName, QString *pathInTrash) |
Permissions | permissions (const QString &fileName) |
Permissions | permissions (const std::filesystem::path &filename) |
bool | remove (const QString &fileName) |
bool | rename (const QString &oldName, const QString &newName) |
bool | resize (const QString &fileName, qint64 sz) |
bool | setPermissions (const QString &fileName, Permissions permissions) |
bool | setPermissions (const std::filesystem::path &filename, Permissions permissionSpec) |
QString | symLinkTarget (const QString &fileName) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
enum | FileError |
enum | FileHandleFlag |
enum | FileTime |
enum | MemoryMapFlag |
enum | Permission |
![]() | |
enum | OpenModeFlag |
![]() | |
objectName | |
![]() | |
AbortError | |
AutoCloseHandle | |
CopyError | |
DontCloseHandle | |
ExeGroup | |
ExeOther | |
ExeOwner | |
ExeUser | |
FatalError | |
FileAccessTime | |
FileBirthTime | |
typedef | FileHandleFlags |
FileMetadataChangeTime | |
FileModificationTime | |
MapPrivateOption | |
typedef | MemoryMapFlags |
NoError | |
NoOptions | |
OpenError | |
typedef | Permissions |
PermissionsError | |
PositionError | |
ReadError | |
ReadGroup | |
ReadOther | |
ReadOwner | |
ReadUser | |
RemoveError | |
RenameError | |
ResizeError | |
ResourceError | |
TimeOutError | |
UnspecifiedError | |
WriteError | |
WriteGroup | |
WriteOther | |
WriteOwner | |
WriteUser | |
![]() | |
typedef | QObjectList |
![]() | |
Append | |
ExistingOnly | |
NewOnly | |
NotOpen | |
typedef | OpenMode |
ReadOnly | |
ReadWrite | |
Text | |
Truncate | |
Unbuffered | |
WriteOnly | |
![]() | |
virtual qint64 | readData (char *data, qint64 len) override |
virtual qint64 | readLineData (char *data, qint64 maxlen) override |
virtual qint64 | writeData (const char *data, qint64 len) override |
![]() | |
void | setErrorString (const QString &str) |
void | setOpenMode (QIODeviceBase::OpenMode openMode) |
virtual qint64 | skipData (qint64 maxSize) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Creates and manages a temporary "auto-save" file.
Autosave files are temporary files that applications use to store the unsaved data in a file they have open for editing. KAutoSaveFile allows you to easily create and manage such files, as well as to recover the unsaved data left over by a crashed or otherwise gone process.
Each KAutoSaveFile object is associated with one specific file that the application holds open. KAutoSaveFile is also a QObject, so it can be reparented to the actual opened file object, so as to manage the lifetime of the temporary file.
Typical use consists of:
- verifying whether stale autosave files exist for the opened file
- deciding whether to recover the old, autosaved data
- if not recovering, creating a KAutoSaveFile object for the opened file
- during normal execution of the program, periodically save unsaved data into the KAutoSaveFile file.
KAutoSaveFile holds a lock on the autosave file, so it's safe to delete the file and recreate it later. Because of that, disposing of stale autosave files should be done with releaseLock(). No lock is held on the managed file.
Examples: Opening a new file:
The function recoverFiles could loop over the list of files and do this:
If the file is unsaved, periodically write the contents to the save file:
When the user saves the file, the autosaved file is no longer necessary and can be removed or emptied.
Definition at line 120 of file kautosavefile.h.
Constructor & Destructor Documentation
◆ KAutoSaveFile() [1/2]
Constructs a KAutoSaveFile for file filename
.
The temporary file is not opened or created until actually needed. The file filename
does not have to exist for KAutoSaveFile to be constructed (if it exists, it will not be touched).
- Parameters
-
filename the filename that this KAutoSaveFile refers to parent the parent object
Definition at line 85 of file kautosavefile.cpp.
◆ KAutoSaveFile() [2/2]
|
explicit |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Constructs a KAutoSaveFile object.
Note that you need to call setManagedFile() before calling open().
- Parameters
-
parent the parent object
Definition at line 92 of file kautosavefile.cpp.
◆ ~KAutoSaveFile()
|
override |
Destroys the KAutoSaveFile object, removes the autosave file and drops the lock being held (if any).
Definition at line 98 of file kautosavefile.cpp.
Member Function Documentation
◆ allStaleFiles()
|
static |
Returns all stale autosave files left behind by crashed or otherwise gone instances of this application.
If not given, the application name is obtained from QCoreApplication, so be sure to have set it correctly before calling this function.
See staleFiles() for information on the returned objects.
The application owns all returned KAutoSaveFile objects and is responsible for deleting them when no longer needed. Remember that deleting the KAutoSaveFile will release the file lock and remove the stale autosave file.
Definition at line 227 of file kautosavefile.cpp.
◆ managedFile()
QUrl KAutoSaveFile::managedFile | ( | ) | const |
Retrieves the URL of the file managed by KAutoSaveFile.
This is the same URL that was given to setManagedFile() or the KAutoSaveFile constructor.
This is the name of the real file being edited by the application. To get the name of the temporary file where data can be saved, use fileName() (after you have called open()).
Definition at line 104 of file kautosavefile.cpp.
◆ open()
|
overridevirtual |
Opens the autosave file and locks it if it wasn't already locked.
The name of the temporary file where data can be saved to will be set by this function and can be retrieved with fileName(). It will not change unless releaseLock() is called. No other application will attempt to edit such a file either while the lock is held.
- Parameters
-
openmode the mode that should be used to open the file, probably QIODevice::ReadWrite
- Returns
- true if the file could be opened (= locked and created), false if the operation failed
Reimplemented from QFile.
Definition at line 128 of file kautosavefile.cpp.
◆ releaseLock()
|
virtual |
Closes the autosave file resource and removes the lock file.
The file name returned by fileName() will no longer be protected and can be overwritten by another application at any time. To obtain a new lock, call open() again.
This function calls remove(), so the autosave temporary file will be removed too.
Definition at line 117 of file kautosavefile.cpp.
◆ setManagedFile()
void KAutoSaveFile::setManagedFile | ( | const QUrl & | filename | ) |
Sets the URL of the file managed by KAutoSaveFile.
This should be the name of the real file being edited by the application. If the file was previously set, this function calls releaseLock().
- Parameters
-
filename the filename that this KAutoSaveFile refers to
Definition at line 109 of file kautosavefile.cpp.
◆ staleFiles()
|
static |
Checks for stale autosave files for the file url
.
Returns a list of autosave files that contain autosaved data left behind by other instances of the application, due to crashing or otherwise uncleanly exiting.
It is the application's job to determine what to do with such unsaved data. Generally, this is done by asking the user if he wants to see the recovered data, and then allowing the user to save if he wants to.
If not given, the application name is obtained from QCoreApplication, so be sure to have set it correctly before calling this function.
This function returns a list of unopened KAutoSaveFile objects. By calling open() on them, the application will steal the lock. Subsequent releaseLock() or deleting of the object will then erase the stale autosave file.
The application owns all returned KAutoSaveFile objects and is responsible for deleting them when no longer needed. Remember that deleting the KAutoSaveFile will release the file lock and remove the stale autosave file.
Definition at line 199 of file kautosavefile.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 18 2025 12:05:24 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.