KRunner::AbstractRunner
#include <KRunner/AbstractRunner>
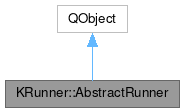
Signals | |
void | prepare () |
void | teardown () |
Public Member Functions | |
bool | hasMatchRegex () const |
QString | id () const |
bool | isMatchingSuspended () const |
virtual void | match (KRunner::RunnerContext &context)=0 |
QRegularExpression | matchRegex () const |
KPluginMetaData | metadata () const |
int | minLetterCount () const |
QString | name () const |
virtual void | reloadConfiguration () |
virtual void | run (const KRunner::RunnerContext &context, const KRunner::QueryMatch &match) |
void | setMatchRegex (const QRegularExpression ®ex) |
void | setMinLetterCount (int count) |
void | setTriggerWords (const QStringList &triggerWords) |
QList< RunnerSyntax > | syntaxes () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
AbstractRunner (QObject *parent, const KPluginMetaData &pluginMetaData) | |
void | addSyntax (const QString &exampleQuery, const QString &description) |
void | addSyntax (const QStringList &exampleQueries, const QString &description) |
void | addSyntax (const RunnerSyntax &syntax) |
KConfigGroup | config () const |
virtual void | init () |
virtual QMimeData * | mimeDataForMatch (const KRunner::QueryMatch &match) |
void | setSyntaxes (const QList< RunnerSyntax > &syntaxes) |
void | suspendMatching (bool suspend) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
An abstract base class for Plasma Runner plugins.
Be aware that runners will be moved to their own thread after being instantiated. This means that except for AbstractRunner::run and the constructor, all methods will be non-blocking for the UI. Consider doing heavy resource initialization in the init method instead of the constructor.
Definition at line 44 of file abstractrunner.h.
Constructor & Destructor Documentation
◆ AbstractRunner()
|
explicitprotected |
Constructor for a KRunner plugin.
- Note
- You should connect here to the prepare/teardown signals. However, avoid doing heavy initialization here in favor of doing it in AbstractRunner::init
- Parameters
-
parent parent object for this runner pluginMetaData metadata that was embedded in the runner args for compatibility with KPluginFactory, since 6.0 this can be omitted
- Since
- 5.72
Definition at line 23 of file abstractrunner.cpp.
Member Function Documentation
◆ addSyntax() [1/3]
|
inlineprotected |
Utility overload for creating a syntax based on the given parameters.
- See also
- RunnerSyntax
- Since
- 5.106
Definition at line 251 of file abstractrunner.h.
◆ addSyntax() [2/3]
|
inlineprotected |
Utility overload for creating a syntax based on the given parameters.
- See also
- RunnerSyntax
- Since
- 5.106
Definition at line 257 of file abstractrunner.h.
◆ addSyntax() [3/3]
|
protected |
Adds a registered syntax that this runner understands.
This is used to display to the user what this runner can understand and how it can be used.
- Parameters
-
syntax the syntax to register
Definition at line 59 of file abstractrunner.cpp.
◆ config()
|
protected |
Provides access to the runner's configuration object.
Definition at line 49 of file abstractrunner.cpp.
◆ hasMatchRegex()
bool KRunner::AbstractRunner::hasMatchRegex | ( | ) | const |
If the runner has a valid regex and non empty regex.
- Since
- 5.75
Definition at line 167 of file abstractrunner.cpp.
◆ id()
QString KRunner::AbstractRunner::id | ( | ) | const |
- Returns
- an id from the runner's metadata'
Definition at line 93 of file abstractrunner.cpp.
◆ init()
|
protectedvirtual |
Reimplement this to run any initialization routines on first load.
Because it is executed in the runner's thread, it will not block the UI and is thus preferred. By default, it calls reloadConfiguration();
Until the runner is initialized, it will not be queried by the RunnerManager.
Definition at line 103 of file abstractrunner.cpp.
◆ isMatchingSuspended()
bool KRunner::AbstractRunner::isMatchingSuspended | ( | ) | const |
- Returns
- true if the runner is currently busy with non-interuptable work, signaling that the RunnerManager may not query it or read it's config properties
Definition at line 108 of file abstractrunner.cpp.
◆ match()
|
pure virtual |
This is the main query method.
It should trigger creation of QueryMatch instances through RunnerContext::addMatch and RunnerContext::addMatches.
If the runner can run precisely the requested term (RunnerContext::query()), it should create an exact match by setting the type to RunnerContext::ExactMatch. The first runner that creates a QueryMatch will be the default runner. Other runner's matches will be suggested in the interface. Non-exact matches should be offered via RunnerContext::PossibleMatch.
The match will be activated via run() if the user selects it.
All matches need to be reported once this method returns. Asynchronous runners therefore need to make use of a local event loop to wait for all matches.
It is recommended to use local status data in async runners. The simplest way is to have a separate class doing all the work like so:
Here MyAsyncWorker creates all the matches and calls RunnerContext::addMatch in some internal slot. It emits the finished() signal once done which will quit the loop and make the match() method return.
Execution of the correct action should be handled in the run method.
- Warning
- Returning from this method means to end execution of the runner.
◆ matchRegex()
QRegularExpression KRunner::AbstractRunner::matchRegex | ( | ) | const |
If this regex is set with a non empty pattern it must match the query in order for match being called.
Just like the minLetterCount property this check is ignored when the runner is in the singleRunnerMode. In case both the regex and the letter count is set the letter count is checked first.
- Returns
- matchRegex property
- See also
- hasMatchRegex
- Since
- 5.75
Definition at line 137 of file abstractrunner.cpp.
◆ metadata()
KPluginMetaData KRunner::AbstractRunner::metadata | ( | ) | const |
- Returns
- the plugin metadata for this runner that was passed in the constructor
Definition at line 98 of file abstractrunner.cpp.
◆ mimeDataForMatch()
|
protectedvirtual |
Reimplement this if you want your runner to support serialization and drag and drop.
By default, this sets the QMimeData urls to the ones specified in QueryMatch::urls
Definition at line 74 of file abstractrunner.cpp.
◆ minLetterCount()
int KRunner::AbstractRunner::minLetterCount | ( | ) | const |
This is the minimum letter count for the query.
If the query is shorter than this value and KRunner is not in the singleRunnerMode, match method is not called. This can be set using the X-Plasma-Runner-Min-Letter-Count property or the setMinLetterCount method. The default value is 0.
- See also
- setMinLetterCount
- match
- Since
- 5.75
Definition at line 127 of file abstractrunner.cpp.
◆ name()
QString KRunner::AbstractRunner::name | ( | ) | const |
Returns the translated name from the runner's metadata.
Definition at line 88 of file abstractrunner.cpp.
◆ prepare
|
signal |
This signal is emitted when matching is about to commence, giving runners an opportunity to prepare themselves, e.g.
loading data sets or preparing IPC or network connections. Things that should be loaded once and remain extant for the lifespan of the AbstractRunner should be done in init().
- See also
- init()
◆ reloadConfiguration()
|
virtual |
Reloads the runner's configuration.
This is called when it's KCM in the PluginSelector is applied. This function may be used to set for example using setMatchRegex, setMinLetterCount or setTriggerWords. Also, syntaxes should be updated when this method is called. While reloading the config, matching is suspended.
Definition at line 55 of file abstractrunner.cpp.
◆ run()
|
virtual |
Called whenever an exact or possible match associated with this runner is triggered.
- Parameters
-
context The context in which the match is triggered, i.e. for which the match was created. match The actual match to run/execute.
Definition at line 84 of file abstractrunner.cpp.
◆ setMatchRegex()
void KRunner::AbstractRunner::setMatchRegex | ( | const QRegularExpression & | regex | ) |
Set the matchRegex property.
- Parameters
-
regex
- Since
- 5.75
Definition at line 142 of file abstractrunner.cpp.
◆ setMinLetterCount()
void KRunner::AbstractRunner::setMinLetterCount | ( | int | count | ) |
Set the minLetterCount property.
- Parameters
-
count
- Since
- 5.75
Definition at line 132 of file abstractrunner.cpp.
◆ setSyntaxes()
|
protected |
Sets the list of syntaxes; passing in an empty list effectively clears the syntaxes.
- Parameters
-
the syntaxes to register for this runner
Definition at line 64 of file abstractrunner.cpp.
◆ setTriggerWords()
void KRunner::AbstractRunner::setTriggerWords | ( | const QStringList & | triggerWords | ) |
Constructs internally a regex which requires the query to start with the trigger words.
Multiple words are concatenated with or, for instance: "^word1|word2|word3". The trigger words are internally escaped. Also the minLetterCount is set to the shortest word in the list.
- Since
- 5.75
- See also
- matchRegex
Definition at line 148 of file abstractrunner.cpp.
◆ suspendMatching()
|
protected |
Sets whether or not the runner is available for match requests.
Useful to prevent queries when the runner is in a busy state.
- Note
- Do not permanently suspend the runner. This is only intended as a temporary measure to avoid useless queries being launched or async fetching of config/data being interfered with.
Definition at line 114 of file abstractrunner.cpp.
◆ syntaxes()
QList< RunnerSyntax > KRunner::AbstractRunner::syntaxes | ( | ) | const |
- Returns
- the syntaxes the runner has registered that it accepts and understands
Definition at line 69 of file abstractrunner.cpp.
◆ teardown
|
signal |
This signal is emitted when a session of matches is complete, giving runners the opportunity to tear down anything set up as a result of the prepare() method.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Apr 27 2024 22:11:43 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.