KRunner::RunnerManager
#include <KRunner/RunnerManager>
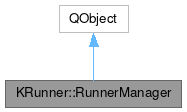
Properties | |
QStringList | history |
bool | historyEnabled |
![]() | |
objectName | |
Signals | |
void | historyEnabledChanged () |
void | matchesChanged (const QList< KRunner::QueryMatch > &matches) |
void | queryFinished () |
void | requestUpdateQueryString (const QString &term, int cursorPosition) |
Public Slots | |
void | launchQuery (const QString &term, const QString &runnerId=QString()) |
void | matchSessionComplete () |
void | reset () |
Q_INVOKABLE void | setHistoryEnvironmentIdentifier (const QString &identifier) |
void | setupMatchSession () |
Public Member Functions | |
RunnerManager (const KConfigGroup &pluginConfigGroup, const KConfigGroup &stateGroup, QObject *parent) | |
RunnerManager (QObject *parent=nullptr) | |
Q_INVOKABLE QString | getHistorySuggestion (const QString &typedQuery) const |
QStringList | history () const |
bool | historyEnabled () |
AbstractRunner * | loadRunner (const KPluginMetaData &pluginMetaData) |
QList< QueryMatch > | matches () const |
QMimeData * | mimeDataForMatch (const QueryMatch &match) const |
QString | query () const |
void | reloadConfiguration () |
Q_INVOKABLE void | removeFromHistory (int index) |
bool | run (const QueryMatch &match, const KRunner::Action &action={}) |
AbstractRunner * | runner (const QString &pluginId) const |
QList< AbstractRunner * > | runners () const |
RunnerContext * | searchContext () const |
void | setAllowedRunners (const QStringList &runners) |
void | setHistoryEnabled (bool enabled) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static QList< KPluginMetaData > | runnerMetaDataList () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The RunnerManager class decides what installed runners are runnable, and their ratings.
It is the main proxy to the runners.
Definition at line 42 of file runnermanager.h.
Property Documentation
◆ history
|
read |
Definition at line 45 of file runnermanager.h.
◆ historyEnabled
|
readwrite |
Definition at line 46 of file runnermanager.h.
Constructor & Destructor Documentation
◆ RunnerManager() [1/2]
|
explicit |
Constructs a RunnerManager with the given parameters.
- Parameters
-
configurationGroup Config group used for reading enabled plugins stateGroup Config group used for storing history
- Since
- 6.0
Definition at line 378 of file runnermanager.cpp.
◆ RunnerManager() [2/2]
|
explicit |
Constructs a RunnerManager using the default locations for state/plugin config.
Definition at line 386 of file runnermanager.cpp.
◆ ~RunnerManager()
|
override |
Definition at line 396 of file runnermanager.cpp.
Member Function Documentation
◆ getHistorySuggestion()
Get the suggested history entry for the typed query.
If no entry is found an empty string is returned.
- Parameters
-
typedQuery
- Returns
- completion for typedQuery
- Since
- 5.78
Definition at line 649 of file runnermanager.cpp.
◆ history()
QStringList KRunner::RunnerManager::history | ( | ) | const |
- Returns
- History of this runner for the current activity. If the RunnerManager is not history aware the global entries will be returned.
- Since
- 5.78
Definition at line 635 of file runnermanager.cpp.
◆ historyEnabled()
bool KRunner::RunnerManager::historyEnabled | ( | ) |
If history completion is enabled, the default value is true.
- Since
- 5.78
◆ historyEnabledChanged
|
signal |
- See also
historyEnabled
- Since
- 5.78
◆ launchQuery
|
slot |
Launch a query, this will create threads and return immediately.
When the information will be available can be known using the matchesChanged signal.
- Parameters
-
term the term we want to find matches for runnerId optional, if only one specific runner is to be used; providing an id will put the manager into single runner mode
Definition at line 548 of file runnermanager.cpp.
◆ loadRunner()
AbstractRunner * KRunner::RunnerManager::loadRunner | ( | const KPluginMetaData & | pluginMetaData | ) |
Attempts to add the AbstractRunner plugin represented by the plugin info passed in.
Usually one can simply let the configuration of plugins handle loading Runner plugins, but in cases where specific runners should be loaded this allows for that to take place
- Note
- Consider using setAllowedRunners in case you want to only allow specific runners
- Parameters
-
pluginMetaData the metaData to use to load the plugin
- Returns
- the loaded runner or nullptr
Definition at line 419 of file runnermanager.cpp.
◆ matches()
QList< QueryMatch > KRunner::RunnerManager::matches | ( | ) | const |
Retrieves all available matches found so far for the previously launched query.
- Returns
- List of matches
Definition at line 456 of file runnermanager.cpp.
◆ matchesChanged
|
signal |
Emitted each time a new match is added to the list.
◆ matchSessionComplete
|
slot |
Call this method when the query session is finished for the time being.
- See also
- prepareForMatchSession
Definition at line 536 of file runnermanager.cpp.
◆ mimeDataForMatch()
QMimeData * KRunner::RunnerManager::mimeDataForMatch | ( | const QueryMatch & | match | ) | const |
- Returns
- mime data of the specified match
Definition at line 485 of file runnermanager.cpp.
◆ query()
QString KRunner::RunnerManager::query | ( | ) | const |
- Returns
- the current query term set in launchQuery
Definition at line 630 of file runnermanager.cpp.
◆ queryFinished
|
signal |
Emitted when the launchQuery finish.
◆ reloadConfiguration()
void KRunner::RunnerManager::reloadConfiguration | ( | ) |
Causes a reload of the current configuration This gets called automatically when the config in the KCM is saved.
Definition at line 402 of file runnermanager.cpp.
◆ removeFromHistory()
void KRunner::RunnerManager::removeFromHistory | ( | int | index | ) |
Delete the given index from the history.
- Parameters
-
historyEntry
- Since
- 5.78
Definition at line 640 of file runnermanager.cpp.
◆ requestUpdateQueryString
|
signal |
Put the given search term in the KRunner search field.
- Parameters
-
term The term that should be displayed cursorPosition Where the cursor should be positioned
- Since
- 6.0
◆ reset
|
slot |
Reset the current data and stops the query.
Definition at line 660 of file runnermanager.cpp.
◆ run()
bool KRunner::RunnerManager::run | ( | const QueryMatch & | match, |
const KRunner::Action & | action = {} ) |
Runs a given match.
This also respects the extra handling for the InformationalMatch. This also handles the history automatically
- Parameters
-
match the match to be executed selectedAction the action returned by QueryMatch::actions that has been selected by the user, nullptr if none
- Returns
- if the RunnerWindow should close
- Since
- 6.0
Definition at line 461 of file runnermanager.cpp.
◆ runner()
AbstractRunner * KRunner::RunnerManager::runner | ( | const QString & | pluginId | ) | const |
Finds and returns a loaded runner or a nullptr.
- Parameters
-
pluginId the name of the runner plugin
- Returns
- Pointer to the runner
Definition at line 434 of file runnermanager.cpp.
◆ runnerMetaDataList()
|
static |
- Returns
- metadata list of all known Runner plugins
- Since
- 5.72
Definition at line 490 of file runnermanager.cpp.
◆ runners()
QList< AbstractRunner * > KRunner::RunnerManager::runners | ( | ) | const |
- Returns
- the list of all currently loaded runners
Definition at line 443 of file runnermanager.cpp.
◆ searchContext()
RunnerContext * KRunner::RunnerManager::searchContext | ( | ) | const |
Retrieves the current context.
- Returns
- pointer to the current context
Definition at line 451 of file runnermanager.cpp.
◆ setAllowedRunners()
void KRunner::RunnerManager::setAllowedRunners | ( | const QStringList & | runners | ) |
Sets a whitelist for the plugins that can be loaded by this manager.
Runners that are disabled through the config will not be loaded.
- Parameters
-
plugins the plugin names of allowed runners
Definition at line 410 of file runnermanager.cpp.
◆ setHistoryEnabled()
void KRunner::RunnerManager::setHistoryEnabled | ( | bool | enabled | ) |
Enables/disabled the history feature for the RunnerManager instance.
The value will not be persisted and is only kept during the object's lifetime.
- Since
- 6.0
Definition at line 679 of file runnermanager.cpp.
◆ setHistoryEnvironmentIdentifier
|
slot |
Set the environment identifier for recording history and launch counts.
- Since
- 6.0
Definition at line 690 of file runnermanager.cpp.
◆ setupMatchSession
|
slot |
Call this method when the runners should be prepared for a query session.
Call matchSessionComplete when the query session is finished for the time being.
- See also
- matchSessionComplete
Definition at line 513 of file runnermanager.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Apr 27 2024 22:11:43 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.