QCPLayoutInset
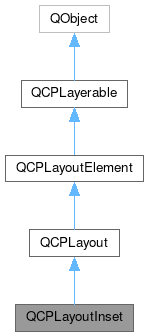
Public Types | |
enum | InsetPlacement { ipFree , ipBorderAligned } |
![]() | |
enum | SizeConstraintRect { scrInnerRect , scrOuterRect } |
enum | UpdatePhase { upPreparation , upMargins , upLayout } |
![]() | |
typedef | QObjectList |
Public Member Functions | |
QCPLayoutInset () | |
void | addElement (QCPLayoutElement *element, const QRectF &rect) |
void | addElement (QCPLayoutElement *element, Qt::Alignment alignment) |
virtual QCPLayoutElement * | elementAt (int index) const override |
virtual int | elementCount () const override |
Qt::Alignment | insetAlignment (int index) const |
InsetPlacement | insetPlacement (int index) const |
QRectF | insetRect (int index) const |
virtual double | selectTest (const QPointF &pos, bool onlySelectable, QVariant *details=nullptr) const override |
void | setInsetAlignment (int index, Qt::Alignment alignment) |
void | setInsetPlacement (int index, InsetPlacement placement) |
void | setInsetRect (int index, const QRectF &rect) |
virtual void | simplify () override |
virtual bool | take (QCPLayoutElement *element) override |
virtual QCPLayoutElement * | takeAt (int index) override |
virtual void | updateLayout () override |
![]() | |
QCPLayout () | |
void | clear () |
virtual QList< QCPLayoutElement * > | elements (bool recursive) const override |
bool | remove (QCPLayoutElement *element) |
bool | removeAt (int index) |
virtual void | update (UpdatePhase phase) override |
![]() | |
QCPLayoutElement (QCustomPlot *parentPlot=nullptr) | |
QCP::MarginSides | autoMargins () const |
QCPLayout * | layout () const |
QCPMarginGroup * | marginGroup (QCP::MarginSide side) const |
QHash< QCP::MarginSide, QCPMarginGroup * > | marginGroups () const |
QMargins | margins () const |
virtual QSize | maximumOuterSizeHint () const |
QSize | maximumSize () const |
QMargins | minimumMargins () const |
virtual QSize | minimumOuterSizeHint () const |
QSize | minimumSize () const |
QRect | outerRect () const |
QRect | rect () const |
void | setAutoMargins (QCP::MarginSides sides) |
void | setMarginGroup (QCP::MarginSides sides, QCPMarginGroup *group) |
void | setMargins (const QMargins &margins) |
void | setMaximumSize (const QSize &size) |
void | setMaximumSize (int width, int height) |
void | setMinimumMargins (const QMargins &margins) |
void | setMinimumSize (const QSize &size) |
void | setMinimumSize (int width, int height) |
void | setOuterRect (const QRect &rect) |
void | setSizeConstraintRect (SizeConstraintRect constraintRect) |
SizeConstraintRect | sizeConstraintRect () const |
![]() | |
QCPLayerable (QCustomPlot *plot, QString targetLayer=QString(), QCPLayerable *parentLayerable=nullptr) | |
bool | antialiased () const |
QCPLayer * | layer () const |
QCPLayerable * | parentLayerable () const |
QCustomPlot * | parentPlot () const |
bool | realVisibility () const |
void | setAntialiased (bool enabled) |
bool | setLayer (const QString &layerName) |
Q_SLOT bool | setLayer (QCPLayer *layer) |
void | setVisible (bool on) |
bool | visible () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Attributes | |
QList< QCPLayoutElement * > | mElements |
QList< Qt::Alignment > | mInsetAlignment |
QList< InsetPlacement > | mInsetPlacement |
QList< QRectF > | mInsetRect |
![]() | |
QCP::MarginSides | mAutoMargins |
QHash< QCP::MarginSide, QCPMarginGroup * > | mMarginGroups |
QMargins | mMargins |
QSize | mMaximumSize |
QMargins | mMinimumMargins |
QSize | mMinimumSize |
QRect | mOuterRect |
QCPLayout * | mParentLayout |
QRect | mRect |
SizeConstraintRect | mSizeConstraintRect |
![]() | |
bool | mAntialiased |
QCPLayer * | mLayer |
QPointer< QCPLayerable > | mParentLayerable |
QCustomPlot * | mParentPlot |
bool | mVisible |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
void | layerChanged (QCPLayer *newLayer) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
void | adoptElement (QCPLayoutElement *el) |
QVector< int > | getSectionSizes (QVector< int > maxSizes, QVector< int > minSizes, QVector< double > stretchFactors, int totalSize) const |
void | releaseElement (QCPLayoutElement *el) |
void | sizeConstraintsChanged () const |
![]() | |
virtual void | applyDefaultAntialiasingHint (QCPPainter *painter) const override |
virtual int | calculateAutoMargin (QCP::MarginSide side) |
virtual void | draw (QCPPainter *painter) override |
virtual void | layoutChanged () |
virtual void | parentPlotInitialized (QCustomPlot *parentPlot) override |
![]() | |
void | applyAntialiasingHint (QCPPainter *painter, bool localAntialiased, QCP::AntialiasedElement overrideElement) const |
virtual QRect | clipRect () const |
virtual void | deselectEvent (bool *selectionStateChanged) |
void | initializeParentPlot (QCustomPlot *parentPlot) |
virtual void | mouseDoubleClickEvent (QMouseEvent *event, const QVariant &details) |
virtual void | mouseMoveEvent (QMouseEvent *event, const QPointF &startPos) |
virtual void | mousePressEvent (QMouseEvent *event, const QVariant &details) |
virtual void | mouseReleaseEvent (QMouseEvent *event, const QPointF &startPos) |
bool | moveToLayer (QCPLayer *layer, bool prepend) |
virtual void | selectEvent (QMouseEvent *event, bool additive, const QVariant &details, bool *selectionStateChanged) |
virtual QCP::Interaction | selectionCategory () const |
void | setParentLayerable (QCPLayerable *parentLayerable) |
virtual void | wheelEvent (QWheelEvent *event) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
static QSize | getFinalMaximumOuterSize (const QCPLayoutElement *el) |
static QSize | getFinalMinimumOuterSize (const QCPLayoutElement *el) |
Detailed Description
A layout that places child elements aligned to the border or arbitrarily positioned.
Elements are placed either aligned to the border or at arbitrary position in the area of the layout. Which placement applies is controlled with the InsetPlacement (setInsetPlacement).
Elements are added via addElement(QCPLayoutElement *element, Qt::Alignment alignment) or addElement(QCPLayoutElement *element, const QRectF &rect). If the first method is used, the inset placement will default to ipBorderAligned and the element will be aligned according to the alignment parameter. The second method defaults to ipFree and allows placing elements at arbitrary position and size, defined by rect.
The alignment or rect can be set via setInsetAlignment or setInsetRect, respectively.
This is the layout that every QCPAxisRect has as QCPAxisRect::insetLayout.
Definition at line 1472 of file qcustomplot.h.
Member Enumeration Documentation
◆ InsetPlacement
Defines how the placement and sizing is handled for a certain element in a QCPLayoutInset.
Enumerator | |
---|---|
ipFree | The element may be positioned/sized arbitrarily, see setInsetRect. |
ipBorderAligned | The element is aligned to one of the layout sides, see setInsetAlignment. |
Definition at line 1479 of file qcustomplot.h.
Constructor & Destructor Documentation
◆ QCPLayoutInset()
|
explicit |
Creates an instance of QCPLayoutInset and sets default values.
Definition at line 4887 of file qcustomplot.cpp.
◆ ~QCPLayoutInset()
|
overridevirtual |
Definition at line 4891 of file qcustomplot.cpp.
Member Function Documentation
◆ addElement() [1/2]
void QCPLayoutInset::addElement | ( | QCPLayoutElement * | element, |
const QRectF & | rect ) |
Adds the specified element to the layout as an inset with free positioning/sizing (setInsetAlignment is initialized with ipFree). The position and size is set to rect.
rect is given in fractions of the whole inset layout rect. So an inset with rect (0, 0, 1, 1) will span the entire layout. An inset with rect (0.6, 0.1, 0.35, 0.35) will be in the top right corner of the layout, with 35% width and height of the parent layout.
Definition at line 5146 of file qcustomplot.cpp.
◆ addElement() [2/2]
void QCPLayoutInset::addElement | ( | QCPLayoutElement * | element, |
Qt::Alignment | alignment ) |
Adds the specified element to the layout as an inset aligned at the border (setInsetAlignment is initialized with ipBorderAligned). The alignment is set to alignment.
alignment is an or combination of the following alignment flags: Qt::AlignLeft, Qt::AlignHCenter, Qt::AlighRight, Qt::AlignTop, Qt::AlignVCenter, Qt::AlignBottom. Any other alignment flags will be ignored.
Definition at line 5120 of file qcustomplot.cpp.
◆ elementAt()
|
overridevirtual |
Returns the element in the cell with the given index. If index is invalid, returns nullptr
.
Note that even if index is valid, the respective cell may be empty in some layouts (e.g. QCPLayoutGrid), so this function may return nullptr
in those cases. You may use this function to check whether a cell is empty or not.
- See also
- elements, elementCount, takeAt
Implements QCPLayout.
Definition at line 5039 of file qcustomplot.cpp.
◆ elementCount()
|
overridevirtual |
Returns the number of elements/cells in the layout.
Implements QCPLayout.
Definition at line 5033 of file qcustomplot.cpp.
◆ insetAlignment()
Qt::Alignment QCPLayoutInset::insetAlignment | ( | int | index | ) | const |
Returns the alignment of the element with the specified index. The alignment only has a meaning, if the inset placement (setInsetPlacement) is ipBorderAligned.
Definition at line 4916 of file qcustomplot.cpp.
◆ insetPlacement()
QCPLayoutInset::InsetPlacement QCPLayoutInset::insetPlacement | ( | int | index | ) | const |
Returns the placement type of the element with the specified index.
Definition at line 4901 of file qcustomplot.cpp.
◆ insetRect()
QRectF QCPLayoutInset::insetRect | ( | int | index | ) | const |
Returns the rect of the element with the specified index. The rect only has a meaning, if the inset placement (setInsetPlacement) is ipFree.
Definition at line 4935 of file qcustomplot.cpp.
◆ selectTest()
|
overridevirtual |
The inset layout is sensitive to events only at areas where its (visible) child elements are sensitive. If the selectTest method of any of the child elements returns a positive number for pos, this method returns a value corresponding to 0.99 times the parent plot's selection tolerance. The inset layout is not selectable itself by default. So if onlySelectable is true, -1.0 is returned.
See QCPLayerable::selectTest for a general explanation of this virtual method.
Reimplemented from QCPLayoutElement.
Definition at line 5093 of file qcustomplot.cpp.
◆ setInsetAlignment()
void QCPLayoutInset::setInsetAlignment | ( | int | index, |
Qt::Alignment | alignment ) |
If the inset placement (setInsetPlacement) is ipBorderAligned, this function is used to set the alignment of the element with the specified index to alignment.
alignment is an or combination of the following alignment flags: Qt::AlignLeft, Qt::AlignHCenter, Qt::AlighRight, Qt::AlignTop, Qt::AlignVCenter, Qt::AlignBottom. Any other alignment flags will be ignored.
Definition at line 4967 of file qcustomplot.cpp.
◆ setInsetPlacement()
void QCPLayoutInset::setInsetPlacement | ( | int | index, |
QCPLayoutInset::InsetPlacement | placement ) |
Sets the inset placement type of the element with the specified index to placement.
- See also
- InsetPlacement
Definition at line 4951 of file qcustomplot.cpp.
◆ setInsetRect()
void QCPLayoutInset::setInsetRect | ( | int | index, |
const QRectF & | rect ) |
If the inset placement (setInsetPlacement) is ipFree, this function is used to set the position and size of the element with the specified index to rect.
rect is given in fractions of the whole inset layout rect. So an inset with rect (0, 0, 1, 1) will span the entire layout. An inset with rect (0.6, 0.1, 0.35, 0.35) will be in the top right corner of the layout, with 35% width and height of the parent layout.
Note that the minimum and maximum sizes of the embedded element (QCPLayoutElement::setMinimumSize, QCPLayoutElement::setMaximumSize) are enforced.
Definition at line 4986 of file qcustomplot.cpp.
◆ simplify()
|
inlineoverridevirtual |
The QCPInsetLayout does not need simplification since it can never have empty cells due to its linear index structure. This method does nothing.
Reimplemented from QCPLayout.
Definition at line 1503 of file qcustomplot.h.
◆ take()
|
overridevirtual |
Removes the specified element from the layout and returns true on success.
If the element isn't in this layout, returns false.
Note that some layouts don't remove the respective cell right away but leave an empty cell after successful removal of the layout element. To collapse empty cells, use simplify.
- See also
- takeAt
Implements QCPLayout.
Definition at line 5066 of file qcustomplot.cpp.
◆ takeAt()
|
overridevirtual |
Removes the element with the given index from the layout and returns it.
If the index is invalid or the cell with that index is empty, returns nullptr
.
Note that some layouts don't remove the respective cell right away but leave an empty cell after successful removal of the layout element. To collapse empty cells, use simplify.
Implements QCPLayout.
Definition at line 5048 of file qcustomplot.cpp.
◆ updateLayout()
|
overridevirtual |
Subclasses reimplement this method to update the position and sizes of the child elements/cells via calling their QCPLayoutElement::setOuterRect. The default implementation does nothing.
The geometry used as a reference is the inner rect of this layout. Child elements should stay within that rect.
getSectionSizes may help with the reimplementation of this function.
- See also
- update
Reimplemented from QCPLayout.
Definition at line 4995 of file qcustomplot.cpp.
Member Data Documentation
◆ mElements
|
protected |
Definition at line 1512 of file qcustomplot.h.
◆ mInsetAlignment
|
protected |
Definition at line 1514 of file qcustomplot.h.
◆ mInsetPlacement
|
protected |
Definition at line 1513 of file qcustomplot.h.
◆ mInsetRect
Definition at line 1515 of file qcustomplot.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:47:17 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.