kmail
KMCommand Class Reference
#include <kmcommands.h>
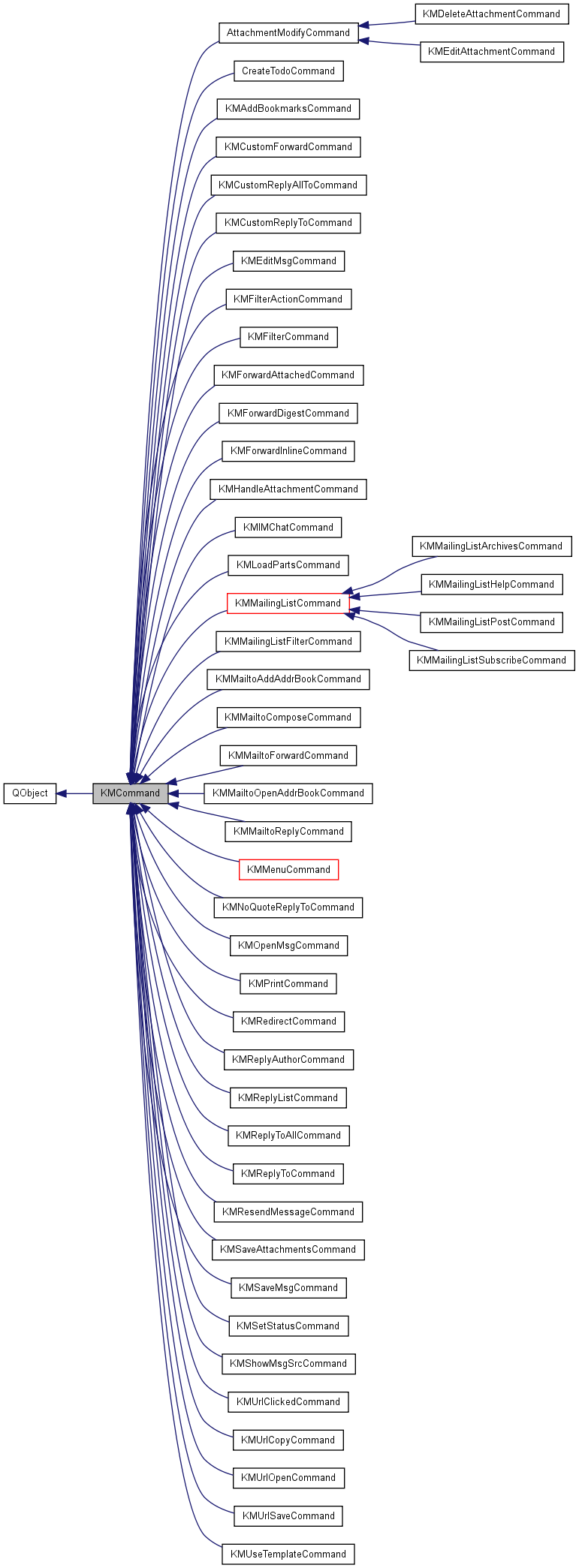
Public Types | |
enum | Result { Undefined, OK, Canceled, Failed } |
Public Slots | |
void | slotProgress (unsigned long done, unsigned long total) |
void | start () |
Signals | |
void | completed (KMCommand *command) |
void | messagesTransfered (KMCommand::Result result) |
Public Member Functions | |
void | keepFolderOpen (KMFolder *folder) |
KMCommand (QWidget *parent, KMMessage *message) | |
KMCommand (QWidget *parent, KMMsgBase *msgBase) | |
KMCommand (QWidget *parent, const QPtrList< KMMsgBase > &msgList) | |
KMCommand (QWidget *parent=0) | |
Result | result () |
virtual | ~KMCommand () |
Protected Member Functions | |
bool | deletesItself () |
bool | emitsCompletedItself () |
QWidget * | parentWidget () const |
KMMessage * | retrievedMessage () const |
const QPtrList< KMMessage > | retrievedMsgs () const |
void | setDeletesItself (bool deletesItself) |
void | setEmitsCompletedItself (bool emitsCompletedItself) |
void | setResult (Result result) |
Detailed Description
Definition at line 49 of file kmcommands.h.
Member Enumeration Documentation
enum KMCommand::Result |
Constructor & Destructor Documentation
KMCommand::KMCommand | ( | QWidget * | parent = 0 |
) |
Definition at line 158 of file kmcommands.cpp.
Definition at line 164 of file kmcommands.cpp.
Definition at line 170 of file kmcommands.cpp.
Definition at line 177 of file kmcommands.cpp.
KMCommand::~KMCommand | ( | ) | [virtual] |
Definition at line 185 of file kmcommands.cpp.
Member Function Documentation
void KMCommand::completed | ( | KMCommand * | command | ) | [signal] |
Emitted when the command has completed.
- Parameters:
-
result The status of the command.
bool KMCommand::deletesItself | ( | ) | [inline, protected] |
Definition at line 99 of file kmcommands.h.
bool KMCommand::emitsCompletedItself | ( | ) | [inline, protected] |
Definition at line 108 of file kmcommands.h.
void KMCommand::keepFolderOpen | ( | KMFolder * | folder | ) |
These folders will be closed by the dtor, handy, if you need to keep a folder open during the lifetime of the command, but don't want to care about closing it again.
Definition at line 447 of file kmcommands.cpp.
void KMCommand::messagesTransfered | ( | KMCommand::Result | result | ) | [signal] |
QWidget * KMCommand::parentWidget | ( | ) | const [protected] |
Definition at line 218 of file kmcommands.cpp.
KMCommand::Result KMCommand::result | ( | ) |
Returns the result of the command.
Only call this method from the slot connected to completed().
Definition at line 195 of file kmcommands.cpp.
KMMessage * KMCommand::retrievedMessage | ( | ) | const [protected] |
Definition at line 213 of file kmcommands.cpp.
Definition at line 208 of file kmcommands.cpp.
void KMCommand::setDeletesItself | ( | bool | deletesItself | ) | [inline, protected] |
Specify whether the subclass takes care of the deletion of the object.
By default the base class will delete the object.
- Parameters:
-
deletesItself true if the subclass takes care of deletion, false if the base class should take care of deletion
Definition at line 105 of file kmcommands.h.
void KMCommand::setEmitsCompletedItself | ( | bool | emitsCompletedItself | ) | [inline, protected] |
Specify whether the subclass takes care of emitting the completed() signal.
By default the base class will emit this signal.
- Parameters:
-
emitsCompletedItself true if the subclass emits the completed signal, false if the base class should emit the signal
Definition at line 115 of file kmcommands.h.
void KMCommand::setResult | ( | Result | result | ) | [inline, protected] |
Use this to set the result of the command.
- Parameters:
-
result The result of the command.
Definition at line 121 of file kmcommands.h.
void KMCommand::slotProgress | ( | unsigned long | done, | |
unsigned long | total | |||
) | [slot] |
Definition at line 380 of file kmcommands.cpp.
void KMCommand::start | ( | ) | [slot] |
Definition at line 202 of file kmcommands.cpp.
The documentation for this class was generated from the following files: