marble
#include <AnnotatePlugin.h>
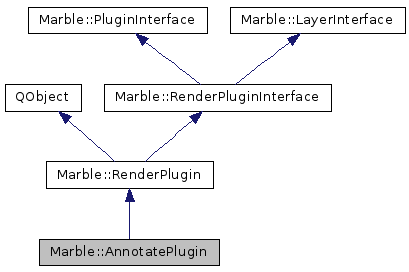
Public Slots | |
void | clearAnnotations () |
void | enableModel (bool enabled) |
void | loadAnnotationFile () |
void | saveAnnotationFile () |
void | setAddingPlacemark (bool) |
void | setDrawingPolygon (bool) |
void | setRemovingItems (bool) |
![]() | |
QAction * | action () const |
void | restoreDefaultSettings () |
void | setEnabled (bool enabled) |
bool | setSetting (const QString &key, const QVariant &value) |
QVariant | setting (const QString &key) |
QStringList | settingKeys () |
void | setUserCheckable (bool isUserCheckable) |
void | setVisible (bool visible) |
Signals | |
void | itemRemoved () |
void | placemarkAdded () |
![]() | |
void | actionGroupsChanged () |
void | enabledChanged (bool enable) |
void | repaintNeeded (QRegion dirtyRegion=QRegion()) |
void | settingsChanged (QString nameId) |
void | userCheckableChanged (bool isUserCheckable) |
void | visibilityChanged (bool visible, const QString &nameId) |
Public Member Functions | |
AnnotatePlugin (const MarbleModel *model=0) | |
virtual | ~AnnotatePlugin () |
virtual const QList < QActionGroup * > * | actionGroups () const |
QStringList | backendTypes () const |
QString | copyrightYears () const |
QString | description () const |
QString | guiString () const |
QIcon | icon () const |
void | initialize () |
bool | isInitialized () const |
QString | name () const |
QString | nameId () const |
QList< PluginAuthor > | pluginAuthors () const |
bool | render (GeoPainter *painter, ViewportParams *viewport, const QString &renderPos, GeoSceneLayer *layer=0) |
QString | renderPolicy () const |
QStringList | renderPosition () const |
virtual QString | runtimeTrace () const |
virtual const QList < QActionGroup * > * | toolbarActionGroups () const |
QString | version () const |
![]() | |
RenderPlugin (const MarbleModel *marbleModel) | |
virtual | ~RenderPlugin () |
bool | enabled () const |
bool | isUserCheckable () const |
const MarbleModel * | marbleModel () const |
virtual RenderPlugin * | newInstance (const MarbleModel *marbleModel) const =0 |
virtual RenderType | renderType () const |
virtual void | setSettings (const QHash< QString, QVariant > &settings) |
virtual QHash< QString, QVariant > | settings () const |
bool | visible () const |
![]() | |
virtual | ~RenderPluginInterface () |
![]() | |
virtual | ~PluginInterface () |
virtual QString | aboutDataText () const |
![]() | |
virtual | ~LayerInterface () |
virtual qreal | zValue () const |
Protected Member Functions | |
bool | eventFilter (QObject *watched, QEvent *event) |
![]() | |
bool | eventFilter (QObject *, QEvent *) |
Additional Inherited Members | |
![]() | |
enum | RenderType { UnknownRenderType, TopLevelRenderType, PanelRenderType, OnlineRenderType, ThemeRenderType } |
![]() | |
QString | description |
bool | enabled |
QString | name |
QString | nameId |
bool | userCheckable |
QString | version |
bool | visible |
Detailed Description
The class that specifies the Marble layer interface of a plugin.
Definition at line 43 of file AnnotatePlugin.h.
Constructor & Destructor Documentation
|
explicit |
Definition at line 42 of file AnnotatePlugin.cpp.
|
virtual |
Definition at line 73 of file AnnotatePlugin.cpp.
Member Function Documentation
|
virtual |
Getting all actions.
This method is used by the main window to get all of the actions that this plugin defines. There is no guarantee where the main window will place the actions but it will generally be in a Menu. The returned QList should also contain all of the actions returned by
- See also
- toolbarActions().
- Returns
- a list of grouped actions
Reimplemented from Marble::RenderPlugin.
Definition at line 164 of file AnnotatePlugin.cpp.
|
virtual |
Returns the name(s) of the backend that the plugin can render This method should return the name of the backend that the plugin can render.
The string has to be the same one that is given for the attribute in the layer element of the DGML file that backend is able to process. Examples to replace available default backends would be "vector" or "texture". To provide a completely new kind of functionality please choose your own unique string.
Implements Marble::RenderPluginInterface.
Definition at line 82 of file AnnotatePlugin.cpp.
|
slot |
Definition at line 307 of file AnnotatePlugin.cpp.
|
virtual |
Implements Marble::PluginInterface.
Definition at line 122 of file AnnotatePlugin.cpp.
|
virtual |
Returns a user description of the plugin.
Implements Marble::PluginInterface.
Definition at line 112 of file AnnotatePlugin.cpp.
|
slot |
Definition at line 187 of file AnnotatePlugin.cpp.
|
protected |
Definition at line 393 of file AnnotatePlugin.cpp.
|
virtual |
String that should be displayed in GUI.
Using a "&" you can suggest key shortcuts
Example: "&Stars"
- Returns
- string for gui usage
Implements Marble::RenderPlugin.
Definition at line 102 of file AnnotatePlugin.cpp.
|
virtual |
Returns an icon for the plugin.
Implements Marble::PluginInterface.
Definition at line 134 of file AnnotatePlugin.cpp.
|
virtual |
Implements Marble::RenderPluginInterface.
Definition at line 140 of file AnnotatePlugin.cpp.
|
virtual |
Implements Marble::RenderPluginInterface.
Definition at line 155 of file AnnotatePlugin.cpp.
|
signal |
|
slot |
Definition at line 344 of file AnnotatePlugin.cpp.
|
virtual |
Returns the user-visible name of the plugin.
The user-visible name should be context free, i.e. the name should provide enough information as to what the plugin is about in the context of Marble.
Example: "Starry Sky Background", "OpenRouteService Routing"
Implements Marble::PluginInterface.
Definition at line 97 of file AnnotatePlugin.cpp.
|
virtual |
Returns the unique name of the plugin.
Examples: "starrysky", "openrouteservice"
Implements Marble::PluginInterface.
Definition at line 107 of file AnnotatePlugin.cpp.
|
signal |
|
virtual |
Implements Marble::PluginInterface.
Definition at line 127 of file AnnotatePlugin.cpp.
|
virtual |
Renders the content provided by the layer on the viewport.
- Parameters
-
painter painter object allowing to paint on the map viewport metadata, such as current projection, screen dimension, etc. renderPos current render position layer deprecated, always zero (NULL)
- Returns
true
Returns whether the rendering has been successful
Implements Marble::LayerInterface.
Definition at line 174 of file AnnotatePlugin.cpp.
|
virtual |
Return how the plugin settings should be used.
FIXME: Document this Possible Values: "ALWAYS" – the plugin renders at the preferred position no matter what got specified in the DGML file. "SPECIFIED" – renders only in case it got specified in the DGML file. "SPECIFIED_ALWAYS" – In case it got specified in the DGML file render according to the specification otherwise choose the preferred position
Implements Marble::RenderPluginInterface.
Definition at line 87 of file AnnotatePlugin.cpp.
|
virtual |
Preferred level in the layer stack for the rendering.
Gives a preferred level in the existing layer stack where the render() method of this plugin should get executed. Possible Values: "NONE" "STARS" "BEHIND_TARGET" "SURFACE" "HOVERS_ABOVE_SURFACE" "ATMOSPHERE" "ORBIT" "ALWAYS_ON_TOP" "FLOAT_ITEM" "USER_TOOLS"
Implements Marble::LayerInterface.
Definition at line 92 of file AnnotatePlugin.cpp.
|
virtual |
Returns a debug line for perfo/tracing issues.
Reimplemented from Marble::RenderPlugin.
Definition at line 160 of file AnnotatePlugin.cpp.
|
slot |
Definition at line 327 of file AnnotatePlugin.cpp.
|
slot |
Definition at line 202 of file AnnotatePlugin.cpp.
|
slot |
Definition at line 207 of file AnnotatePlugin.cpp.
|
slot |
Definition at line 233 of file AnnotatePlugin.cpp.
|
virtual |
Getting all actions which should be placed in the toolbar.
This method returns a subset of the actions returned by
- See also
- actions() which are intended to be placed in a more prominent place such as a toolbar above the Marble Widget. You are not guaranteed that they will be in an actual toolbar but they will be visible and discoverable
- Returns
- a list of grouped toolbar actions
Reimplemented from Marble::RenderPlugin.
Definition at line 169 of file AnnotatePlugin.cpp.
|
virtual |
Implements Marble::PluginInterface.
Definition at line 117 of file AnnotatePlugin.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:38:55 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.