marble
#include <RenderPlugin.h>
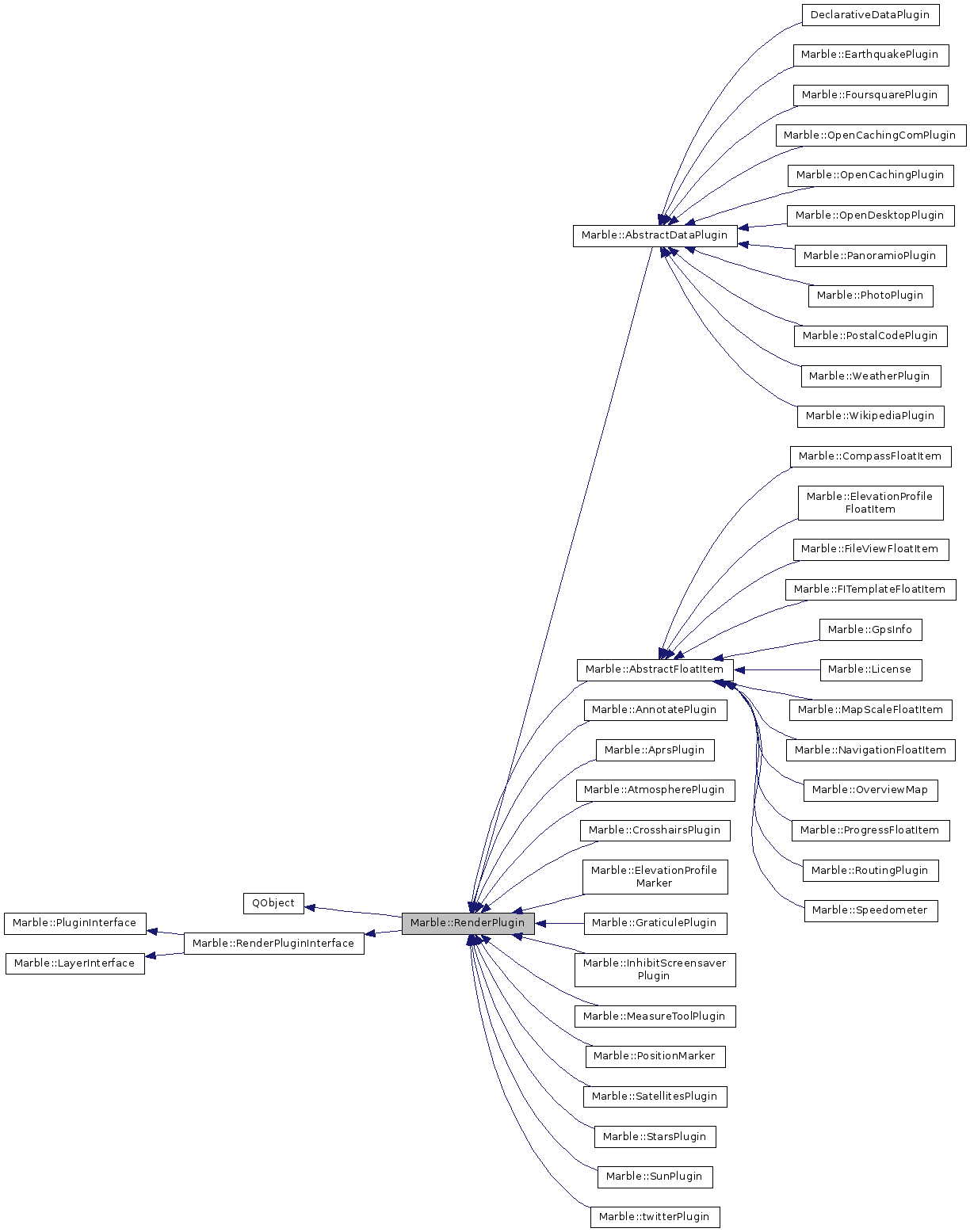
Public Types | |
enum | RenderType { UnknownRenderType, TopLevelRenderType, PanelRenderType, OnlineRenderType, ThemeRenderType } |
Public Slots | |
QAction * | action () const |
void | restoreDefaultSettings () |
void | setEnabled (bool enabled) |
bool | setSetting (const QString &key, const QVariant &value) |
QVariant | setting (const QString &key) |
QStringList | settingKeys () |
void | setUserCheckable (bool isUserCheckable) |
void | setVisible (bool visible) |
Signals | |
void | actionGroupsChanged () |
void | enabledChanged (bool enable) |
void | repaintNeeded (QRegion dirtyRegion=QRegion()) |
void | settingsChanged (QString nameId) |
void | userCheckableChanged (bool isUserCheckable) |
void | visibilityChanged (bool visible, const QString &nameId) |
Public Member Functions | |
RenderPlugin (const MarbleModel *marbleModel) | |
virtual | ~RenderPlugin () |
virtual const QList < QActionGroup * > * | actionGroups () const |
bool | enabled () const |
virtual QString | guiString () const =0 |
bool | isUserCheckable () const |
const MarbleModel * | marbleModel () const |
virtual RenderPlugin * | newInstance (const MarbleModel *marbleModel) const =0 |
virtual RenderType | renderType () const |
virtual QString | runtimeTrace () const |
virtual void | setSettings (const QHash< QString, QVariant > &settings) |
virtual QHash< QString, QVariant > | settings () const |
virtual const QList < QActionGroup * > * | toolbarActionGroups () const |
bool | visible () const |
![]() | |
virtual | ~RenderPluginInterface () |
virtual QStringList | backendTypes () const =0 |
virtual void | initialize ()=0 |
virtual bool | isInitialized () const =0 |
virtual QString | renderPolicy () const =0 |
![]() | |
virtual | ~PluginInterface () |
virtual QString | aboutDataText () const |
virtual QString | copyrightYears () const =0 |
virtual QString | description () const =0 |
virtual QIcon | icon () const =0 |
virtual QString | name () const =0 |
virtual QString | nameId () const =0 |
virtual QList< PluginAuthor > | pluginAuthors () const =0 |
virtual QString | version () const =0 |
![]() | |
virtual | ~LayerInterface () |
virtual bool | render (GeoPainter *painter, ViewportParams *viewport, const QString &renderPos, GeoSceneLayer *layer)=0 |
virtual QStringList | renderPosition () const =0 |
virtual qreal | zValue () const |
Protected Member Functions | |
bool | eventFilter (QObject *, QEvent *) |
Properties | |
QString | description |
bool | enabled |
QString | name |
QString | nameId |
bool | userCheckable |
QString | version |
bool | visible |
Detailed Description
The abstract class that creates a renderable item.
Renderable Plugins can be used to extend Marble's functionality: They allow to draw stuff on top of the map / globe
Definition at line 43 of file RenderPlugin.h.
Member Enumeration Documentation
A Type of plugin.
Enumerator | |
---|---|
UnknownRenderType | |
TopLevelRenderType | |
PanelRenderType | |
OnlineRenderType | |
ThemeRenderType |
Definition at line 59 of file RenderPlugin.h.
Constructor & Destructor Documentation
|
explicit |
Definition at line 60 of file RenderPlugin.cpp.
|
virtual |
Definition at line 76 of file RenderPlugin.cpp.
Member Function Documentation
|
slot |
Plugin's menu action.
The action is checkable and controls the visibility of the plugin.
- Returns
- action, displayed in menu
Definition at line 86 of file RenderPlugin.cpp.
|
virtual |
Getting all actions.
This method is used by the main window to get all of the actions that this plugin defines. There is no guarantee where the main window will place the actions but it will generally be in a Menu. The returned QList should also contain all of the actions returned by
- See also
- toolbarActions().
- Returns
- a list of grouped actions
Reimplemented in Marble::AnnotatePlugin.
Definition at line 96 of file RenderPlugin.cpp.
|
signal |
This signal is emitted if the actions that the plugin supports change in any way.
bool Marble::RenderPlugin::enabled | ( | ) | const |
is enabled
This method indicates enableability of the plugin
If plugin is enabled it going to be displayed in Marble Menu as active action which can be
- See also
- setUserCheckable
- Returns
- enableability of the plugin
- See also
- setEnabled
|
signal |
This signal is emitted if the enabled property is changed with.
- See also
- setEnabled
|
protected |
Definition at line 209 of file RenderPlugin.cpp.
|
pure virtual |
String that should be displayed in GUI.
Using a "&" you can suggest key shortcuts
Example: "&Stars"
- Returns
- string for gui usage
Implemented in Marble::StarsPlugin, Marble::GraticulePlugin, Marble::FITemplateFloatItem, Marble::CrosshairsPlugin, Marble::ElevationProfileFloatItem, Marble::AnnotatePlugin, DeclarativeDataPlugin, Marble::twitterPlugin, Marble::NavigationFloatItem, Marble::OverviewMap, Marble::PositionMarker, Marble::AprsPlugin, Marble::MeasureToolPlugin, Marble::OpenCachingComPlugin, Marble::GpsInfo, Marble::Speedometer, Marble::PhotoPlugin, Marble::ElevationProfileMarker, Marble::OpenCachingPlugin, Marble::SatellitesPlugin, Marble::CompassFloatItem, Marble::InhibitScreensaverPlugin, Marble::WeatherPlugin, Marble::WikipediaPlugin, Marble::MapScaleFloatItem, Marble::RoutingPlugin, Marble::FileViewFloatItem, Marble::EarthquakePlugin, Marble::SunPlugin, Marble::License, Marble::OpenDesktopPlugin, Marble::ProgressFloatItem, Marble::AtmospherePlugin, Marble::PanoramioPlugin, Marble::FoursquarePlugin, and Marble::PostalCodePlugin.
bool Marble::RenderPlugin::isUserCheckable | ( | ) | const |
is user checkable
This method indicates user checkability of plugin's action displayed in application menu
Can control plugin visibility
- Warning
- User can do it only if
- See also
- enabled is true
- Returns
- checkability of the plugin
- See also
- setUserCheckable
Definition at line 178 of file RenderPlugin.cpp.
const MarbleModel * Marble::RenderPlugin::marbleModel | ( | ) | const |
Access to the MarbleModel.
Internal way to access the model of marble. Can be used to interact with the main application
- Returns
- marble model
- See also
- MarbleModel
Definition at line 81 of file RenderPlugin.cpp.
|
pure virtual |
Creation a new instance of the plugin.
This method is used to create a new object of the current plugin using the marbleModel
given.
- Parameters
-
marbleModel base model
- Returns
- new instance of current plugin
- Note
- Typically this method is implemented with the help of the MARBLE_PLUGIN() macro.
Implemented in DeclarativeDataPlugin.
|
virtual |
Render type of the plugin.
Function for returning the type of plugin this is for. This affects where in the menu tree the action() is placed.
- See also
- RenderType
- Returns
- : The type of render plugin this is
Reimplemented in Marble::StarsPlugin, Marble::AbstractDataPlugin, Marble::AbstractFloatItem, Marble::CrosshairsPlugin, Marble::SatellitesPlugin, and Marble::AtmospherePlugin.
Definition at line 199 of file RenderPlugin.cpp.
|
signal |
This signal is emitted if an update of the view is needed.
If available with the dirtyRegion
which is the region the view will change in. If dirtyRegion.isEmpty() returns true, the whole viewport has to be repainted.
|
slot |
Passes an empty set of settings to the plugin.
Well behaving plugins restore their settings to default values as a result of calling this method.
Definition at line 214 of file RenderPlugin.cpp.
|
virtual |
Returns a debug line for perfo/tracing issues.
Reimplemented from Marble::LayerInterface.
Reimplemented in Marble::AnnotatePlugin.
Definition at line 204 of file RenderPlugin.cpp.
|
slot |
settting enabled
If enabled
= true, plugin will be enabled
If plugin is enabled it will be possible to show/hide it from menu (access from UI)
- Parameters
-
enabled plugin's enabled state
- See also
- enabled
Definition at line 137 of file RenderPlugin.cpp.
|
slot |
Change setting key's values.
- Parameters
-
key setting key value new value
This method applies value
for the key
- Returns
- successfully changed or not
Definition at line 224 of file RenderPlugin.cpp.
|
virtual |
Set the settings of the plugin.
Usually this is called at startup to restore saved settings.
- Parameters
-
new plugin's settings
- See also
- settings
Reimplemented in Marble::StarsPlugin, Marble::GraticulePlugin, Marble::PositionMarker, Marble::CrosshairsPlugin, Marble::OverviewMap, Marble::CompassFloatItem, Marble::PhotoPlugin, Marble::AprsPlugin, Marble::MeasureToolPlugin, Marble::WikipediaPlugin, Marble::EarthquakePlugin, Marble::WeatherPlugin, Marble::OpenCachingPlugin, Marble::SatellitesPlugin, Marble::RoutingPlugin, Marble::OpenDesktopPlugin, and Marble::AbstractFloatItem.
Definition at line 193 of file RenderPlugin.cpp.
|
slot |
Getting setting value from the settings.
- Parameters
-
key setting's key index
This method should be used to get current value of key
in settings hash table
- Returns
- setting value
Definition at line 237 of file RenderPlugin.cpp.
|
slot |
Full list of the settings keys.
This method should be used to get all possible settings' keys for the plugin's settings
- Returns
- list with the keys of settings
Definition at line 219 of file RenderPlugin.cpp.
|
virtual |
Settings of the plugin.
Settings is the map (hash table) of plugin's settings This method is called to determine the current settings of the plugin for serialization, e.g. when closing the application.
- Returns
- plugin's settings
- See also
- setSettings
Reimplemented in Marble::StarsPlugin, Marble::GraticulePlugin, Marble::CrosshairsPlugin, Marble::PositionMarker, Marble::OverviewMap, Marble::CompassFloatItem, Marble::AprsPlugin, Marble::MeasureToolPlugin, Marble::PhotoPlugin, Marble::WikipediaPlugin, Marble::WeatherPlugin, Marble::SatellitesPlugin, Marble::EarthquakePlugin, Marble::RoutingPlugin, Marble::OpenCachingPlugin, Marble::OpenDesktopPlugin, and Marble::AbstractFloatItem.
Definition at line 183 of file RenderPlugin.cpp.
|
signal |
This signal is emitted if the settings of the RenderPlugin changed.
|
slot |
settting user checkable
If isUserCheckable
= true, user will get an option to control visibility in application menu
- Parameters
-
isUserCheckable user checkability of the plugin
- See also
- isUserCheckable
Definition at line 159 of file RenderPlugin.cpp.
|
slot |
settting visible
If visible
= true, plugin will be visible
- Parameters
-
visible visibility of the plugin
- See also
- visible
Definition at line 149 of file RenderPlugin.cpp.
|
virtual |
Getting all actions which should be placed in the toolbar.
This method returns a subset of the actions returned by
- See also
- actions() which are intended to be placed in a more prominent place such as a toolbar above the Marble Widget. You are not guaranteed that they will be in an actual toolbar but they will be visible and discoverable
- Returns
- a list of grouped toolbar actions
Reimplemented in Marble::AnnotatePlugin.
Definition at line 101 of file RenderPlugin.cpp.
|
signal |
This signal is emitted if the user checkable property is changed with.
- See also
- setUserCheckable
|
signal |
This signal is emitted if the visibility is changed with.
- See also
- setVisible
bool Marble::RenderPlugin::visible | ( | ) | const |
is visible
This method indicates visibility of the plugin
If plugin is visible you can see it on the map/globe
- Returns
- visibility of the plugin
- See also
- setVisible
Property Documentation
|
read |
Definition at line 50 of file RenderPlugin.h.
|
readwrite |
Definition at line 51 of file RenderPlugin.h.
|
read |
Definition at line 47 of file RenderPlugin.h.
|
read |
Definition at line 48 of file RenderPlugin.h.
|
readwrite |
Definition at line 53 of file RenderPlugin.h.
|
read |
Definition at line 49 of file RenderPlugin.h.
|
readwrite |
Definition at line 52 of file RenderPlugin.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:38:57 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.