marble
#include <ElevationProfileFloatItem.h>
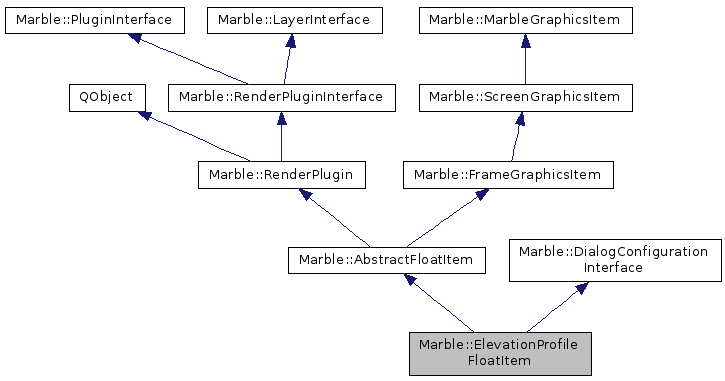
Signals | |
void | dataUpdated () |
![]() | |
void | actionGroupsChanged () |
void | enabledChanged (bool enable) |
void | repaintNeeded (QRegion dirtyRegion=QRegion()) |
void | settingsChanged (QString nameId) |
void | userCheckableChanged (bool isUserCheckable) |
void | visibilityChanged (bool visible, const QString &nameId) |
Public Member Functions | |
ElevationProfileFloatItem () | |
ElevationProfileFloatItem (const MarbleModel *marbleModel) | |
~ElevationProfileFloatItem () | |
virtual QStringList | backendTypes () const |
virtual void | changeViewport (ViewportParams *viewport) |
QDialog * | configDialog () |
virtual QString | copyrightYears () const |
virtual QString | description () const |
virtual QString | guiString () const |
virtual QIcon | icon () const |
virtual void | initialize () |
virtual bool | isInitialized () const |
virtual QString | name () const |
virtual QString | nameId () const |
virtual void | paintContent (QPainter *painter) |
virtual QList< PluginAuthor > | pluginAuthors () const |
virtual QString | version () const |
virtual qreal | zValue () const |
![]() | |
AbstractFloatItem (const MarbleModel *marbleModel, const QPointF &point=QPointF(10.0, 10.0), const QSizeF &size=QSizeF(150.0, 50.0)) | |
virtual | ~AbstractFloatItem () |
QFont | font () const |
QPen | pen () const |
bool | positionLocked () const |
bool | render (GeoPainter *painter, ViewportParams *viewport, const QString &renderPos="FLOAT_ITEM", GeoSceneLayer *layer=0) |
virtual QString | renderPolicy () const |
virtual QStringList | renderPosition () const |
virtual RenderType | renderType () const |
void | setFont (const QFont &font) |
void | setPen (const QPen &pen) |
virtual void | setSettings (const QHash< QString, QVariant > &settings) |
virtual QHash< QString, QVariant > | settings () const |
void | setVisible (bool visible) |
bool | visible () const |
![]() | |
RenderPlugin (const MarbleModel *marbleModel) | |
virtual | ~RenderPlugin () |
virtual const QList < QActionGroup * > * | actionGroups () const |
bool | enabled () const |
bool | isUserCheckable () const |
const MarbleModel * | marbleModel () const |
virtual RenderPlugin * | newInstance (const MarbleModel *marbleModel) const =0 |
virtual QString | runtimeTrace () const |
virtual const QList < QActionGroup * > * | toolbarActionGroups () const |
bool | visible () const |
![]() | |
virtual | ~RenderPluginInterface () |
![]() | |
virtual | ~PluginInterface () |
virtual QString | aboutDataText () const |
![]() | |
virtual | ~LayerInterface () |
![]() | |
FrameGraphicsItem (MarbleGraphicsItem *parent=0) | |
virtual | ~FrameGraphicsItem () |
QBrush | background () const |
QBrush | borderBrush () const |
Qt::PenStyle | borderStyle () const |
qreal | borderWidth () const |
QRectF | contentRect () const |
QSizeF | contentSize () const |
FrameType | frame () const |
qreal | margin () const |
qreal | marginBottom () const |
qreal | marginLeft () const |
qreal | marginRight () const |
qreal | marginTop () const |
qreal | padding () const |
QRectF | paintedRect () const |
void | setBackground (const QBrush &background) |
void | setBorderBrush (const QBrush &brush) |
void | setBorderStyle (Qt::PenStyle style) |
void | setBorderWidth (qreal width) |
void | setContentSize (const QSizeF &size) |
void | setFrame (FrameType type) |
void | setMargin (qreal margin) |
void | setMarginBottom (qreal marginBottom) |
void | setMarginLeft (qreal marginLeft) |
void | setMarginRight (qreal marginRight) |
void | setMarginTop (qreal marginTop) |
void | setPadding (qreal width) |
![]() | |
ScreenGraphicsItem (MarbleGraphicsItem *parent=0) | |
virtual | ~ScreenGraphicsItem () |
QList< QPointF > | absolutePositions () const |
GraphicsItemFlags | flags () const |
QPointF | position () const |
QPointF | positivePosition () const |
void | setFlags (GraphicsItemFlags flags) |
void | setPosition (const QPointF &position) |
![]() | |
virtual | ~MarbleGraphicsItem () |
CacheMode | cacheMode () const |
bool | contains (const QPointF &point) const |
void | hide () |
AbstractMarbleGraphicsLayout * | layout () const |
bool | paintEvent (QPainter *painter, const ViewportParams *viewport) |
void | setCacheMode (CacheMode mode) |
void | setLayout (AbstractMarbleGraphicsLayout *layout) |
virtual void | setProjection (const ViewportParams *viewport) |
void | setSize (const QSizeF &size) |
void | setVisible (bool visible) |
void | show () |
QSizeF | size () const |
bool | visible () const |
![]() | |
virtual | ~DialogConfigurationInterface () |
Protected Member Functions | |
virtual void | contextMenuEvent (QWidget *w, QContextMenuEvent *e) |
bool | eventFilter (QObject *object, QEvent *e) |
![]() | |
QMenu * | contextMenu () |
virtual void | toolTipEvent (QHelpEvent *e) |
![]() | |
bool | eventFilter (QObject *, QEvent *) |
![]() | |
virtual QPainterPath | backgroundShape () const |
virtual void | paint (QPainter *painter) |
virtual void | paintBackground (QPainter *painter) |
![]() | |
ScreenGraphicsItem (ScreenGraphicsItemPrivate *d_ptr) | |
![]() | |
MarbleGraphicsItem (MarbleGraphicsItemPrivate *d_ptr) | |
void | update () |
Detailed Description
The class that creates an interactive elvation profile.
Definition at line 43 of file ElevationProfileFloatItem.h.
Constructor & Destructor Documentation
Marble::ElevationProfileFloatItem::ElevationProfileFloatItem | ( | ) |
Definition at line 36 of file ElevationProfileFloatItem.cpp.
|
explicit |
Definition at line 44 of file ElevationProfileFloatItem.cpp.
Marble::ElevationProfileFloatItem::~ElevationProfileFloatItem | ( | ) |
Definition at line 83 of file ElevationProfileFloatItem.cpp.
Member Function Documentation
|
virtual |
Returns the name(s) of the backend that the plugin can render This method should return the name of the backend that the plugin can render.
The string has to be the same one that is given for the attribute in the layer element of the DGML file that backend is able to process. Examples to replace available default backends would be "vector" or "texture". To provide a completely new kind of functionality please choose your own unique string.
Implements Marble::RenderPluginInterface.
Definition at line 87 of file ElevationProfileFloatItem.cpp.
|
virtual |
Reimplemented from Marble::AbstractFloatItem.
Definition at line 160 of file ElevationProfileFloatItem.cpp.
|
virtual |
Returns a pointer to the configuration dialog of the plugin.
- Returns
- : Pointer to the configuration dialog, which must be non-zero.
Implements Marble::DialogConfigurationInterface.
Definition at line 387 of file ElevationProfileFloatItem.cpp.
|
protectedvirtual |
Reimplemented from Marble::AbstractFloatItem.
Definition at line 405 of file ElevationProfileFloatItem.cpp.
|
virtual |
Implements Marble::PluginInterface.
Definition at line 122 of file ElevationProfileFloatItem.cpp.
|
signal |
|
virtual |
Returns a user description of the plugin.
Implements Marble::PluginInterface.
Definition at line 117 of file ElevationProfileFloatItem.cpp.
|
protectedvirtual |
Reimplemented from Marble::AbstractFloatItem.
Definition at line 427 of file ElevationProfileFloatItem.cpp.
|
virtual |
String that should be displayed in GUI.
Using a "&" you can suggest key shortcuts
Example: "&Stars"
- Returns
- string for gui usage
Implements Marble::RenderPlugin.
Definition at line 102 of file ElevationProfileFloatItem.cpp.
|
virtual |
Returns an icon for the plugin.
Implements Marble::PluginInterface.
Definition at line 134 of file ElevationProfileFloatItem.cpp.
|
virtual |
Implements Marble::RenderPluginInterface.
Definition at line 139 of file ElevationProfileFloatItem.cpp.
|
virtual |
Implements Marble::RenderPluginInterface.
Definition at line 155 of file ElevationProfileFloatItem.cpp.
|
virtual |
Returns the user-visible name of the plugin.
The user-visible name should be context free, i.e. the name should provide enough information as to what the plugin is about in the context of Marble.
Example: "Starry Sky Background", "OpenRouteService Routing"
Implements Marble::PluginInterface.
Definition at line 97 of file ElevationProfileFloatItem.cpp.
|
virtual |
Returns the unique name of the plugin.
Examples: "starrysky", "openrouteservice"
Implements Marble::PluginInterface.
Definition at line 107 of file ElevationProfileFloatItem.cpp.
|
virtual |
Here the items paint their content.
Reimplemented from Marble::FrameGraphicsItem.
Definition at line 182 of file ElevationProfileFloatItem.cpp.
|
virtual |
Implements Marble::PluginInterface.
Definition at line 127 of file ElevationProfileFloatItem.cpp.
|
virtual |
Implements Marble::PluginInterface.
Definition at line 112 of file ElevationProfileFloatItem.cpp.
|
virtual |
Returns the z value of the layer (default: 0.0).
If two layers are painted at the same render position, the one with the higher z value is painted on top. If both have the same z value, their paint order is undefined.
Reimplemented from Marble::LayerInterface.
Definition at line 92 of file ElevationProfileFloatItem.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:38:55 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.