marble
#include <FrameGraphicsItem.h>
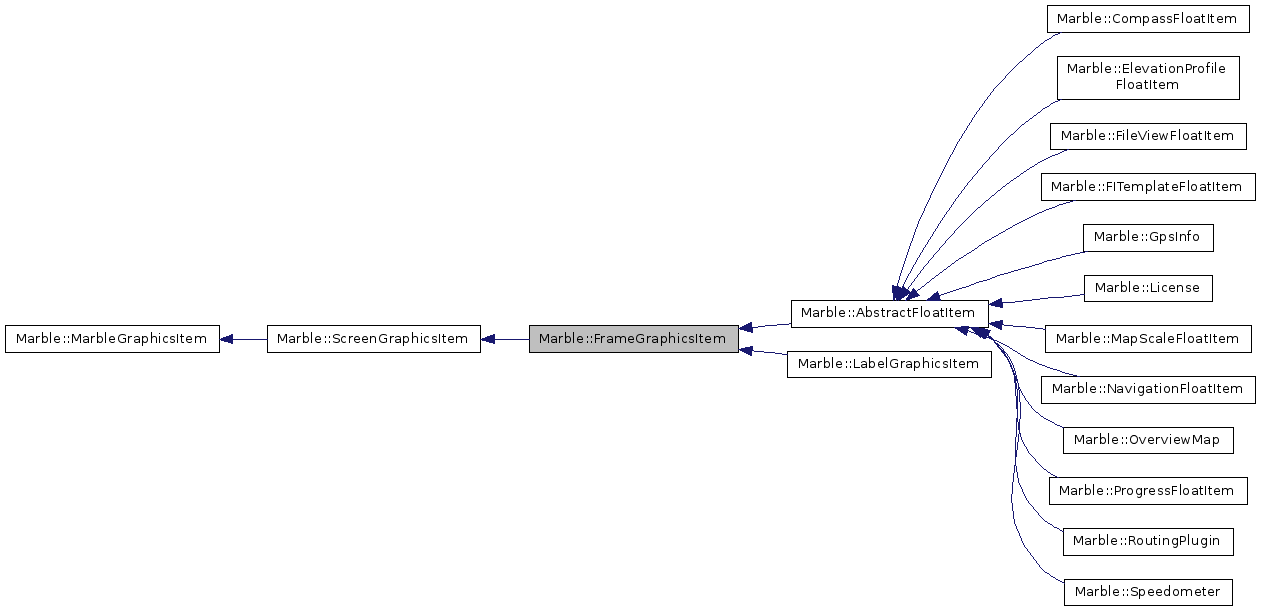
Public Types | |
enum | FrameType { NoFrame, RectFrame, RoundedRectFrame, ShadowFrame } |
![]() | |
enum | GraphicsItemFlag { ItemIsMovable = 0x1, ItemIsHideable = 0x2 } |
![]() | |
enum | CacheMode { NoCache, ItemCoordinateCache, DeviceCoordinateCache } |
Public Member Functions | |
FrameGraphicsItem (MarbleGraphicsItem *parent=0) | |
virtual | ~FrameGraphicsItem () |
QBrush | background () const |
QBrush | borderBrush () const |
Qt::PenStyle | borderStyle () const |
qreal | borderWidth () const |
QRectF | contentRect () const |
QSizeF | contentSize () const |
FrameType | frame () const |
qreal | margin () const |
qreal | marginBottom () const |
qreal | marginLeft () const |
qreal | marginRight () const |
qreal | marginTop () const |
qreal | padding () const |
QRectF | paintedRect () const |
void | setBackground (const QBrush &background) |
void | setBorderBrush (const QBrush &brush) |
void | setBorderStyle (Qt::PenStyle style) |
void | setBorderWidth (qreal width) |
void | setContentSize (const QSizeF &size) |
void | setFrame (FrameType type) |
void | setMargin (qreal margin) |
void | setMarginBottom (qreal marginBottom) |
void | setMarginLeft (qreal marginLeft) |
void | setMarginRight (qreal marginRight) |
void | setMarginTop (qreal marginTop) |
void | setPadding (qreal width) |
![]() | |
ScreenGraphicsItem (MarbleGraphicsItem *parent=0) | |
virtual | ~ScreenGraphicsItem () |
QList< QPointF > | absolutePositions () const |
GraphicsItemFlags | flags () const |
QPointF | position () const |
QPointF | positivePosition () const |
void | setFlags (GraphicsItemFlags flags) |
void | setPosition (const QPointF &position) |
![]() | |
virtual | ~MarbleGraphicsItem () |
CacheMode | cacheMode () const |
bool | contains (const QPointF &point) const |
void | hide () |
AbstractMarbleGraphicsLayout * | layout () const |
bool | paintEvent (QPainter *painter, const ViewportParams *viewport) |
void | setCacheMode (CacheMode mode) |
void | setLayout (AbstractMarbleGraphicsLayout *layout) |
virtual void | setProjection (const ViewportParams *viewport) |
void | setSize (const QSizeF &size) |
void | setVisible (bool visible) |
void | show () |
QSizeF | size () const |
bool | visible () const |
Protected Member Functions | |
virtual QPainterPath | backgroundShape () const |
virtual void | paint (QPainter *painter) |
virtual void | paintBackground (QPainter *painter) |
virtual void | paintContent (QPainter *painter) |
![]() | |
ScreenGraphicsItem (ScreenGraphicsItemPrivate *d_ptr) | |
virtual bool | eventFilter (QObject *, QEvent *) |
![]() | |
MarbleGraphicsItem (MarbleGraphicsItemPrivate *d_ptr) | |
void | update () |
Additional Inherited Members | |
![]() | |
MarbleGraphicsItemPrivate *const | d |
Detailed Description
Definition at line 25 of file FrameGraphicsItem.h.
Member Enumeration Documentation
Enumerator | |
---|---|
NoFrame | |
RectFrame | |
RoundedRectFrame | |
ShadowFrame |
Definition at line 28 of file FrameGraphicsItem.h.
Constructor & Destructor Documentation
|
explicit |
Definition at line 31 of file FrameGraphicsItem.cpp.
|
virtual |
Definition at line 37 of file FrameGraphicsItem.cpp.
Member Function Documentation
QBrush FrameGraphicsItem::background | ( | ) | const |
Returns the background brush of the item.
Definition at line 160 of file FrameGraphicsItem.cpp.
|
protectedvirtual |
Returns the shape of the background.
Reimplemented in Marble::FITemplateFloatItem, Marble::CompassFloatItem, Marble::ProgressFloatItem, and Marble::FileViewFloatItem.
Definition at line 210 of file FrameGraphicsItem.cpp.
QBrush FrameGraphicsItem::borderBrush | ( | ) | const |
Returns the brush of the border.
Definition at line 138 of file FrameGraphicsItem.cpp.
Qt::PenStyle FrameGraphicsItem::borderStyle | ( | ) | const |
Returns the style of the border.
Definition at line 149 of file FrameGraphicsItem.cpp.
qreal FrameGraphicsItem::borderWidth | ( | ) | const |
Returns the border width of the item.
Definition at line 113 of file FrameGraphicsItem.cpp.
|
virtual |
Returns the rect of the content in item coordinates.
Reimplemented from Marble::MarbleGraphicsItem.
Definition at line 171 of file FrameGraphicsItem.cpp.
|
virtual |
Returns the size of the content of the MarbleGraphicsItem.
This is identical to size() for default MarbleGraphicsItems.
Reimplemented from Marble::MarbleGraphicsItem.
Definition at line 184 of file FrameGraphicsItem.cpp.
FrameGraphicsItem::FrameType FrameGraphicsItem::frame | ( | ) | const |
Returns the type of the frame.
Definition at line 42 of file FrameGraphicsItem.cpp.
qreal FrameGraphicsItem::margin | ( | ) | const |
Returns the margin of the item.
This is used for all margins with the value 0.0. The padding is the space outside the painted space.
Definition at line 53 of file FrameGraphicsItem.cpp.
qreal FrameGraphicsItem::marginBottom | ( | ) | const |
Returns the bottom margin of the item.
Definition at line 77 of file FrameGraphicsItem.cpp.
qreal FrameGraphicsItem::marginLeft | ( | ) | const |
Returns the left margin of the item.
Definition at line 89 of file FrameGraphicsItem.cpp.
qreal FrameGraphicsItem::marginRight | ( | ) | const |
Returns the right margin of the item.
Definition at line 101 of file FrameGraphicsItem.cpp.
qreal FrameGraphicsItem::marginTop | ( | ) | const |
Returns the top margin of the item.
Definition at line 65 of file FrameGraphicsItem.cpp.
qreal FrameGraphicsItem::padding | ( | ) | const |
Returns the padding of the item.
The padding is the empty space inside the border.
Definition at line 125 of file FrameGraphicsItem.cpp.
|
protectedvirtual |
This function won't be reimplemented in most cases.
Reimplemented from Marble::MarbleGraphicsItem.
Definition at line 235 of file FrameGraphicsItem.cpp.
|
protectedvirtual |
Paints the background.
This function won't be reimplemented in most cases.
Definition at line 225 of file FrameGraphicsItem.cpp.
|
protectedvirtual |
Here the items paint their content.
Reimplemented in Marble::ElevationProfileFloatItem, Marble::NavigationFloatItem, Marble::OverviewMap, Marble::CompassFloatItem, Marble::MapScaleFloatItem, Marble::ProgressFloatItem, and Marble::LabelGraphicsItem.
Definition at line 258 of file FrameGraphicsItem.cpp.
QRectF FrameGraphicsItem::paintedRect | ( | ) | const |
Definition at line 189 of file FrameGraphicsItem.cpp.
void FrameGraphicsItem::setBackground | ( | const QBrush & | background | ) |
Changes the background brush of the item.
Definition at line 165 of file FrameGraphicsItem.cpp.
void FrameGraphicsItem::setBorderBrush | ( | const QBrush & | brush | ) |
Change the brush of the border.
Definition at line 143 of file FrameGraphicsItem.cpp.
void FrameGraphicsItem::setBorderStyle | ( | Qt::PenStyle | style | ) |
Change the style of the border.
Definition at line 154 of file FrameGraphicsItem.cpp.
void FrameGraphicsItem::setBorderWidth | ( | qreal | width | ) |
Set the border width of the item.
Definition at line 118 of file FrameGraphicsItem.cpp.
|
virtual |
Sets the size of the content of the item.
size
is the size required for contents.
Reimplemented from Marble::MarbleGraphicsItem.
Reimplemented in Marble::LabelGraphicsItem.
Definition at line 204 of file FrameGraphicsItem.cpp.
void FrameGraphicsItem::setFrame | ( | FrameType | type | ) |
Sets the type of the Frame.
Standard is NoFrame.
Definition at line 47 of file FrameGraphicsItem.cpp.
void FrameGraphicsItem::setMargin | ( | qreal | margin | ) |
Sets the margin of the item.
This is used for all margins with the value 0.0.
Definition at line 58 of file FrameGraphicsItem.cpp.
void FrameGraphicsItem::setMarginBottom | ( | qreal | marginBottom | ) |
Set the bottom margin of the item.
Definition at line 82 of file FrameGraphicsItem.cpp.
void FrameGraphicsItem::setMarginLeft | ( | qreal | marginLeft | ) |
Set the left margin of the item.
Definition at line 94 of file FrameGraphicsItem.cpp.
void FrameGraphicsItem::setMarginRight | ( | qreal | marginRight | ) |
Set the right margin of the item.
Definition at line 106 of file FrameGraphicsItem.cpp.
void FrameGraphicsItem::setMarginTop | ( | qreal | marginTop | ) |
Set the top margin of the item.
Definition at line 70 of file FrameGraphicsItem.cpp.
void FrameGraphicsItem::setPadding | ( | qreal | width | ) |
Set the padding of the item.
Definition at line 130 of file FrameGraphicsItem.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:38:55 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.