marble
#include <GeoDataTrack.h>
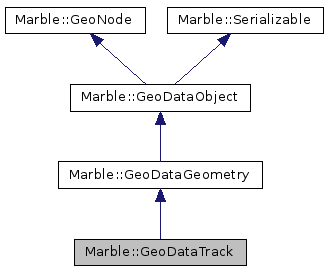
Public Member Functions | |
GeoDataTrack () | |
GeoDataTrack (const GeoDataGeometry &other) | |
void | addPoint (const QDateTime &when, const GeoDataCoordinates &coord) |
void | appendAltitude (qreal altitude) |
void | appendCoordinates (const GeoDataCoordinates &coord) |
void | appendWhen (const QDateTime &when) |
void | clear () |
GeoDataCoordinates | coordinatesAt (const QDateTime &when) const |
GeoDataCoordinates | coordinatesAt (int index) const |
QList< GeoDataCoordinates > | coordinatesList () const |
GeoDataExtendedData & | extendedData () const |
QDateTime | firstWhen () const |
virtual EnumGeometryId | geometryId () const |
bool | interpolate () const |
QDateTime | lastWhen () const |
virtual const GeoDataLatLonAltBox & | latLonAltBox () const |
const GeoDataLineString * | lineString () const |
virtual const char * | nodeType () const |
virtual void | pack (QDataStream &stream) const |
void | removeAfter (const QDateTime &when) |
void | removeBefore (const QDateTime &when) |
void | setExtendedData (const GeoDataExtendedData &extendedData) |
void | setInterpolate (bool on) |
int | size () const |
virtual void | unpack (QDataStream &stream) |
QList< QDateTime > | whenList () const |
![]() | |
GeoDataGeometry () | |
GeoDataGeometry (const GeoDataGeometry &other) | |
virtual | ~GeoDataGeometry () |
AltitudeMode | altitudeMode () const |
void | detach () |
bool | extrude () const |
GeoDataGeometry & | operator= (const GeoDataGeometry &other) |
void | setAltitudeMode (const AltitudeMode altitudeMode) |
void | setExtrude (bool extrude) |
![]() | |
GeoDataObject () | |
GeoDataObject (const GeoDataObject &) | |
virtual | ~GeoDataObject () |
int | id () const |
GeoDataObject & | operator= (const GeoDataObject &) |
virtual GeoDataObject * | parent () const |
QString | resolvePath (const QString &relativePath) const |
void | setId (int value) |
virtual void | setParent (GeoDataObject *parent) |
void | setTargetId (int value) |
int | targetId () const |
![]() | |
GeoNode () | |
virtual | ~GeoNode () |
![]() | |
virtual | ~Serializable () |
Detailed Description
A geometry for tracking objects made of (time, coordinates) pairs.
GeoDataTrack implements the Track tag defined in Google's extension of the Open Geospatial Consortium standard KML 2.2 at http://code.google.com/apis/kml/documentation/kmlreference.html#gxtrack .
A track is made of points, each point has a coordinates and a time value associated with the coordinates. New points can be added using the addPoint() method. The coordinates of the tracked object at a particular time can be found using coordinatesAt(), you can specify if interpolation should be used using the setInterpolate() function.
By default, a LineString that passes through every coordinates in the track is drawn. You can customize it by changing the GeoDataLineStyle, for example if the GeoDataTrack is the geometry of feature, you can disable the line drawing with:
For convenience, the methods appendCoordinates() and appendWhen() are provided. They let you add points by specifying their coordinates and time value separately. When N calls to one of these methods are followed by N calls to the other, the first coordinates will be matched with the first time value, the second coordinates with the second time value, etc. This follows the way "coord" and "when" tags inside the Track tag should be parsed.
Definition at line 54 of file GeoDataTrack.h.
Constructor & Destructor Documentation
Marble::GeoDataTrack::GeoDataTrack | ( | ) |
Definition at line 55 of file GeoDataTrack.cpp.
|
explicit |
Definition at line 61 of file GeoDataTrack.cpp.
Member Function Documentation
void Marble::GeoDataTrack::addPoint | ( | const QDateTime & | when, |
const GeoDataCoordinates & | coord | ||
) |
Add a new point with coordinates coord
associated with the time value when
.
Definition at line 175 of file GeoDataTrack.cpp.
void Marble::GeoDataTrack::appendAltitude | ( | qreal | altitude | ) |
Add altitude information to the last appended coordinates.
Definition at line 197 of file GeoDataTrack.cpp.
void Marble::GeoDataTrack::appendCoordinates | ( | const GeoDataCoordinates & | coord | ) |
Add the coordinates part for a new point.
See this class description for more information.
- See also
- appendWhen
Definition at line 190 of file GeoDataTrack.cpp.
void Marble::GeoDataTrack::appendWhen | ( | const QDateTime & | when | ) |
Add the time value part for a new point.
See this class description for more information.
- See also
- appendCoordinates
Definition at line 207 of file GeoDataTrack.cpp.
void Marble::GeoDataTrack::clear | ( | ) |
Remove all the points contained in the track.
Definition at line 212 of file GeoDataTrack.cpp.
GeoDataCoordinates Marble::GeoDataTrack::coordinatesAt | ( | const QDateTime & | when | ) | const |
If interpolate() is true, return the coordinates interpolated from the time values before and after when
, otherwise return the coordinates of the point with the closest time value less than or equal to when
.
- See also
- interpolate
Definition at line 110 of file GeoDataTrack.cpp.
GeoDataCoordinates Marble::GeoDataTrack::coordinatesAt | ( | int | index | ) | const |
Return coordinates at specified index.
This is useful when the track contains coordinates without time information.
Definition at line 170 of file GeoDataTrack.cpp.
QList< GeoDataCoordinates > Marble::GeoDataTrack::coordinatesList | ( | ) | const |
Returns the coordinates of all the points in the map, sorted by their time value.
Definition at line 100 of file GeoDataTrack.cpp.
GeoDataExtendedData & Marble::GeoDataTrack::extendedData | ( | ) | const |
Return the ExtendedData assigned to the feature.
Definition at line 260 of file GeoDataTrack.cpp.
QDateTime Marble::GeoDataTrack::firstWhen | ( | ) | const |
Return the time value of the first point in the track, or an invalid QDateTime if the track is empty.
Definition at line 82 of file GeoDataTrack.cpp.
|
virtual |
Reimplemented from Marble::GeoDataGeometry.
Definition at line 275 of file GeoDataTrack.cpp.
bool Marble::GeoDataTrack::interpolate | ( | ) | const |
Returns true if coordinatesAt() should use interpolation, false otherwise.
The default is false.
- See also
- setInterpolate, coordinatesAt
Definition at line 72 of file GeoDataTrack.cpp.
QDateTime Marble::GeoDataTrack::lastWhen | ( | ) | const |
Return the time value of the last point in the track, or an invalid QDateTime if the track is empty.
Definition at line 91 of file GeoDataTrack.cpp.
|
virtual |
Reimplemented from Marble::GeoDataGeometry.
Definition at line 280 of file GeoDataTrack.cpp.
const GeoDataLineString * Marble::GeoDataTrack::lineString | ( | ) | const |
Return the GeoDataLineString representing the current track.
Definition at line 247 of file GeoDataTrack.cpp.
|
virtual |
Provides type information for downcasting a GeoData.
Reimplemented from Marble::GeoDataGeometry.
Definition at line 270 of file GeoDataTrack.cpp.
|
virtual |
Serialize the contents of the feature to stream
.
Reimplemented from Marble::GeoDataGeometry.
Definition at line 286 of file GeoDataTrack.cpp.
void Marble::GeoDataTrack::removeAfter | ( | const QDateTime & | when | ) |
Remove all points from the track whose time value is greater than when
.
Definition at line 233 of file GeoDataTrack.cpp.
void Marble::GeoDataTrack::removeBefore | ( | const QDateTime & | when | ) |
Remove all points from the track whose time value is less than when
.
Definition at line 219 of file GeoDataTrack.cpp.
void Marble::GeoDataTrack::setExtendedData | ( | const GeoDataExtendedData & | extendedData | ) |
Sets the ExtendedData of the feature.
- Parameters
-
extendedData the new ExtendedData to be used.
Definition at line 265 of file GeoDataTrack.cpp.
void Marble::GeoDataTrack::setInterpolate | ( | bool | on | ) |
Set whether coordinatesAt() should use interpolation.
- See also
- interpolate, coordinatesAt
Definition at line 77 of file GeoDataTrack.cpp.
int Marble::GeoDataTrack::size | ( | ) | const |
Returns the number of points in the track.
Definition at line 67 of file GeoDataTrack.cpp.
|
virtual |
Unserialize the contents of the feature from stream
.
Reimplemented from Marble::GeoDataGeometry.
Definition at line 291 of file GeoDataTrack.cpp.
QList< QDateTime > Marble::GeoDataTrack::whenList | ( | ) | const |
Returns the time value of all the points in the map, in chronological order.
Definition at line 105 of file GeoDataTrack.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:38:56 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.