marble
#include <WeatherItem.h>
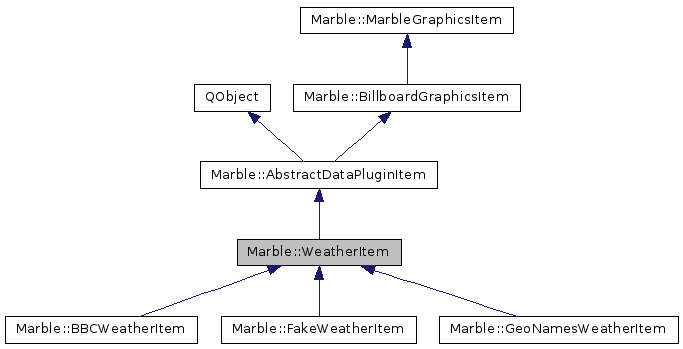
Public Slots | |
void | openBrowser () |
![]() | |
void | toggleFavorite () |
Signals | |
void | descriptionChanged () |
void | imageChanged () |
void | stationNameChanged () |
void | temperatureChanged () |
![]() | |
void | favoriteChanged (const QString &id, bool favorite) |
void | idChanged () |
void | stickyChanged () |
void | updated () |
Public Member Functions | |
WeatherItem (QObject *parent=0) | |
WeatherItem (MarbleWidget *widget, QObject *parent=0) | |
~WeatherItem () | |
QAction * | action () |
virtual QList< QAction * > | actions () |
virtual void | addDownloadedFile (const QString &url, const QString &type)=0 |
void | addForecastWeather (const QList< WeatherData > &forecasts) |
WeatherData | currentWeather () const |
QString | description () const |
QMap< QDate, WeatherData > | forecastWeather () const |
QString | image () const |
bool | initialized () const |
QString | itemType () const |
bool | operator< (const AbstractDataPluginItem *other) const |
quint8 | priority () const |
virtual bool | request (const QString &type) |
virtual QString | service () const =0 |
void | setCurrentWeather (const WeatherData &weather) |
void | setForecastWeather (const QMap< QDate, WeatherData > &forecasts) |
void | setMarbleWidget (MarbleWidget *widget) |
void | setPriority (quint8 priority) |
void | setSettings (const QHash< QString, QVariant > &settings) |
void | setStationName (const QString &name) |
QString | stationName () const |
double | temperature () const |
![]() | |
AbstractDataPluginItem (QObject *parent=0) | |
virtual | ~AbstractDataPluginItem () |
qreal | addedAngularResolution () const |
QString | id () const |
bool | isFavorite () const |
bool | isSticky () const |
void | setAddedAngularResolution (qreal resolution) |
virtual void | setFavorite (bool favorite) |
void | setId (const QString &id) |
void | setSticky (bool sticky) |
void | setTarget (const QString &target) |
void | setToolTip (const QString &toolTip) |
QString | target () |
QString | toolTip () const |
![]() | |
BillboardGraphicsItem () | |
Qt::Alignment | alignment () const |
QList< QRectF > | boundingRects () const |
QRectF | containsRect (const QPointF &point) const |
GeoDataCoordinates | coordinate () const |
QList< QPointF > | positions () const |
void | setAlignment (Qt::Alignment alignment) |
void | setCoordinate (const GeoDataCoordinates &coordinates) |
![]() | |
virtual | ~MarbleGraphicsItem () |
CacheMode | cacheMode () const |
bool | contains (const QPointF &point) const |
virtual QRectF | contentRect () const |
virtual QSizeF | contentSize () const |
void | hide () |
AbstractMarbleGraphicsLayout * | layout () const |
bool | paintEvent (QPainter *painter, const ViewportParams *viewport) |
void | setCacheMode (CacheMode mode) |
virtual void | setContentSize (const QSizeF &size) |
void | setLayout (AbstractMarbleGraphicsLayout *layout) |
virtual void | setProjection (const ViewportParams *viewport) |
void | setSize (const QSizeF &size) |
void | setVisible (bool visible) |
void | show () |
QSizeF | size () const |
bool | visible () const |
Properties | |
QString | description |
QString | image |
QString | station |
double | temperature |
![]() | |
bool | favorite |
QString | identifier |
bool | sticky |
Additional Inherited Members | |
![]() | |
enum | CacheMode { NoCache, ItemCoordinateCache, DeviceCoordinateCache } |
![]() | |
MarbleGraphicsItem (MarbleGraphicsItemPrivate *d_ptr) | |
virtual bool | eventFilter (QObject *object, QEvent *e) |
virtual void | paint (QPainter *painter) |
void | update () |
![]() | |
MarbleGraphicsItemPrivate *const | d |
Detailed Description
This is the class painting a weather item on the screen.
So it is a subclass of AbstractDataItem.
Definition at line 37 of file WeatherItem.h.
Constructor & Destructor Documentation
|
explicit |
Definition at line 327 of file WeatherItem.cpp.
|
explicit |
Definition at line 334 of file WeatherItem.cpp.
Marble::WeatherItem::~WeatherItem | ( | ) |
Definition at line 342 of file WeatherItem.cpp.
Member Function Documentation
|
virtual |
Returns the action of this specific item.
Reimplemented from Marble::AbstractDataPluginItem.
Definition at line 347 of file WeatherItem.cpp.
|
virtual |
Reimplemented from Marble::AbstractDataPluginItem.
Definition at line 548 of file WeatherItem.cpp.
|
pure virtual |
Reimplemented from Marble::AbstractDataPluginItem.
Implemented in Marble::BBCWeatherItem, Marble::FakeWeatherItem, and Marble::GeoNamesWeatherItem.
void Marble::WeatherItem::addForecastWeather | ( | const QList< WeatherData > & | forecasts | ) |
Adds additional forecasts to the list.
If there are multiple forecasts for one day, it will choose the most recent (as of pubDate).
Definition at line 431 of file WeatherItem.cpp.
WeatherData Marble::WeatherItem::currentWeather | ( | ) | const |
Definition at line 402 of file WeatherItem.cpp.
QString Marble::WeatherItem::description | ( | ) | const |
|
signal |
QMap< QDate, WeatherData > Marble::WeatherItem::forecastWeather | ( | ) | const |
Definition at line 418 of file WeatherItem.cpp.
QString Marble::WeatherItem::image | ( | ) | const |
|
signal |
|
virtual |
Implements Marble::AbstractDataPluginItem.
Definition at line 367 of file WeatherItem.cpp.
|
virtual |
Returns the type of this specific item.
Implements Marble::AbstractDataPluginItem.
Definition at line 356 of file WeatherItem.cpp.
|
slot |
Definition at line 490 of file WeatherItem.cpp.
|
virtual |
Implements Marble::AbstractDataPluginItem.
Definition at line 375 of file WeatherItem.cpp.
quint8 Marble::WeatherItem::priority | ( | ) | const |
Definition at line 462 of file WeatherItem.cpp.
|
virtual |
Test if the item wants to request type
again.
Reimplemented in Marble::BBCWeatherItem.
Definition at line 361 of file WeatherItem.cpp.
|
pure virtual |
Returns the provider of the weather information.
Implemented in Marble::BBCWeatherItem, Marble::GeoNamesWeatherItem, and Marble::FakeWeatherItem.
void Marble::WeatherItem::setCurrentWeather | ( | const WeatherData & | weather | ) |
Definition at line 407 of file WeatherItem.cpp.
void Marble::WeatherItem::setForecastWeather | ( | const QMap< QDate, WeatherData > & | forecasts | ) |
Definition at line 423 of file WeatherItem.cpp.
void Marble::WeatherItem::setMarbleWidget | ( | MarbleWidget * | widget | ) |
Definition at line 485 of file WeatherItem.cpp.
void Marble::WeatherItem::setPriority | ( | quint8 | priority | ) |
Definition at line 467 of file WeatherItem.cpp.
|
virtual |
Set the settings of the item.
This is usually called automatically before painting. If you reimplement this it would be useful to check for changes before copying.
Reimplemented from Marble::AbstractDataPluginItem.
Definition at line 472 of file WeatherItem.cpp.
void Marble::WeatherItem::setStationName | ( | const QString & | name | ) |
Definition at line 391 of file WeatherItem.cpp.
QString Marble::WeatherItem::stationName | ( | ) | const |
Definition at line 386 of file WeatherItem.cpp.
|
signal |
double Marble::WeatherItem::temperature | ( | ) | const |
|
signal |
Property Documentation
|
read |
Definition at line 42 of file WeatherItem.h.
|
read |
Definition at line 43 of file WeatherItem.h.
|
readwrite |
Definition at line 41 of file WeatherItem.h.
|
read |
Definition at line 44 of file WeatherItem.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:38:57 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.