Marble::GeoDataBuilding
#include <GeoDataBuilding.h>
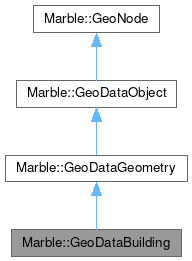
Public Member Functions | |
GeoDataBuilding (const GeoDataBuilding &other) | |
GeoDataBuilding (const GeoDataGeometry &other) | |
~GeoDataBuilding () override | |
GeoDataGeometry * | copy () const override |
QVector< NamedEntry > | entries () const |
EnumGeometryId | geometryId () const override |
double | height () const |
const GeoDataLatLonAltBox & | latLonAltBox () const override |
int | maxLevel () const |
int | minLevel () const |
GeoDataMultiGeometry * | multiGeometry () const |
QString | name () const |
const char * | nodeType () const override |
QVector< int > | nonExistentLevels () const |
GeoDataBuilding & | operator= (const GeoDataBuilding &other) |
void | setEntries (const QVector< NamedEntry > &entries) |
void | setHeight (double height) |
void | setMaxLevel (int maxLevel) |
void | setMinLevel (int minLevel) |
void | setName (const QString &name) |
void | setNonExistentLevels (const QVector< int > &nonExistentLevels) |
![]() | |
AltitudeMode | altitudeMode () const |
void | detach () |
bool | extrude () const |
bool | operator!= (const GeoDataGeometry &other) const |
bool | operator== (const GeoDataGeometry &other) const |
void | pack (QDataStream &stream) const override |
void | setAltitudeMode (const AltitudeMode altitudeMode) |
void | setExtrude (bool extrude) |
void | unpack (QDataStream &stream) override |
![]() | |
GeoDataObject (const GeoDataObject &) | |
QString | id () const |
GeoDataObject & | operator= (const GeoDataObject &) |
void | pack (QDataStream &stream) const override |
GeoDataObject * | parent () |
const GeoDataObject * | parent () const |
QString | resolvePath (const QString &relativePath) const |
void | setId (const QString &value) |
void | setParent (GeoDataObject *parent) |
void | setTargetId (const QString &value) |
QString | targetId () const |
void | unpack (QDataStream &steam) override |
Static Public Member Functions | |
static double | parseBuildingHeight (const QString &buildingHeight) |
Additional Inherited Members | |
![]() | |
GeoDataGeometry (const GeoDataGeometry &other) | |
GeoDataGeometry (GeoDataGeometryPrivate *priv) | |
bool | equals (const GeoDataGeometry &other) const |
virtual bool | equals (const GeoDataObject &other) const |
GeoDataGeometry & | operator= (const GeoDataGeometry &other) |
![]() | |
GeoDataGeometryPrivate * | d_ptr |
Detailed Description
Contains important information about a building and its floors (levels)
GeoDataBuilding holds information such as minimum floor, maximum floor, floor data and their respective MultiGeometry and other possible metadata such as the total height of the building, type etc.
Definition at line 28 of file GeoDataBuilding.h.
Constructor & Destructor Documentation
◆ GeoDataBuilding() [1/3]
|
explicit |
Definition at line 12 of file GeoDataBuilding.cpp.
◆ GeoDataBuilding() [2/3]
|
explicit |
Definition at line 18 of file GeoDataBuilding.cpp.
◆ GeoDataBuilding() [3/3]
|
explicit |
Definition at line 24 of file GeoDataBuilding.cpp.
◆ ~GeoDataBuilding()
|
override |
Destroys the GeoDataBuilding
Definition at line 30 of file GeoDataBuilding.cpp.
Member Function Documentation
◆ copy()
|
overridevirtual |
Implements Marble::GeoDataGeometry.
Definition at line 52 of file GeoDataBuilding.cpp.
◆ entries()
QVector< GeoDataBuilding::NamedEntry > Marble::GeoDataBuilding::entries | ( | ) | const |
Definition at line 119 of file GeoDataBuilding.cpp.
◆ geometryId()
|
overridevirtual |
Implements Marble::GeoDataGeometry.
Definition at line 47 of file GeoDataBuilding.cpp.
◆ height()
double Marble::GeoDataBuilding::height | ( | ) | const |
- Returns
- the height of the building
Definition at line 57 of file GeoDataBuilding.cpp.
◆ latLonAltBox()
|
overridevirtual |
- Returns
- the latlonaltbox for the contained multigeometry
Reimplemented from Marble::GeoDataGeometry.
Definition at line 102 of file GeoDataBuilding.cpp.
◆ maxLevel()
int Marble::GeoDataBuilding::maxLevel | ( | ) | const |
- Returns
- the maximum level of the building
Definition at line 77 of file GeoDataBuilding.cpp.
◆ minLevel()
int Marble::GeoDataBuilding::minLevel | ( | ) | const |
- Returns
- the minimum level
Definition at line 67 of file GeoDataBuilding.cpp.
◆ multiGeometry()
GeoDataMultiGeometry * Marble::GeoDataBuilding::multiGeometry | ( | ) | const |
- Returns
- the multigeometry associated with the building
Definition at line 97 of file GeoDataBuilding.cpp.
◆ name()
QString Marble::GeoDataBuilding::name | ( | ) | const |
- Returns
- the name of the building
Definition at line 109 of file GeoDataBuilding.cpp.
◆ nodeType()
|
overridevirtual |
Provides type information for downcasting a GeoNode.
Implements Marble::GeoNode.
Definition at line 42 of file GeoDataBuilding.cpp.
◆ nonExistentLevels()
QVector< int > Marble::GeoDataBuilding::nonExistentLevels | ( | ) | const |
- Returns
- the non existent levels in the building
Definition at line 87 of file GeoDataBuilding.cpp.
◆ operator=()
GeoDataBuilding & Marble::GeoDataBuilding::operator= | ( | const GeoDataBuilding & | other | ) |
Definition at line 35 of file GeoDataBuilding.cpp.
◆ parseBuildingHeight()
|
static |
Definition at line 129 of file GeoDataBuilding.cpp.
◆ setEntries()
void Marble::GeoDataBuilding::setEntries | ( | const QVector< NamedEntry > & | entries | ) |
Definition at line 124 of file GeoDataBuilding.cpp.
◆ setHeight()
void Marble::GeoDataBuilding::setHeight | ( | double | height | ) |
Sets the height of the building
- Parameters
-
height
Definition at line 62 of file GeoDataBuilding.cpp.
◆ setMaxLevel()
void Marble::GeoDataBuilding::setMaxLevel | ( | int | maxLevel | ) |
Sets the maximum level of the building
- Parameters
-
maxLevel
Definition at line 82 of file GeoDataBuilding.cpp.
◆ setMinLevel()
void Marble::GeoDataBuilding::setMinLevel | ( | int | minLevel | ) |
Sets the minimum level of the building
- Parameters
-
minLevel
Definition at line 72 of file GeoDataBuilding.cpp.
◆ setName()
void Marble::GeoDataBuilding::setName | ( | const QString & | name | ) |
◆ setNonExistentLevels()
void Marble::GeoDataBuilding::setNonExistentLevels | ( | const QVector< int > & | nonExistentLevels | ) |
Sets the non existent levels of the building
- Parameters
-
nonExistentLevels
Definition at line 92 of file GeoDataBuilding.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Jul 26 2024 11:57:58 by doxygen 1.11.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.