QCA::SASLContext
#include <QtCrypto>
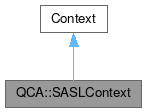
Classes | |
class | HostPort |
Public Types | |
enum | Result { Success , Error , Params , AuthCheck , Continue } |
Signals | |
void | resultsReady () |
Public Member Functions | |
SASLContext (Provider *p) | |
virtual SASL::AuthCondition | authCondition () const =0 |
virtual QString | authzid () const =0 |
virtual SASL::Params | clientParams () const =0 |
virtual int | encoded () const =0 |
virtual bool | haveClientInit () const =0 |
virtual QString | mech () const =0 |
virtual QStringList | mechlist () const =0 |
virtual void | nextStep (const QByteArray &from_net)=0 |
virtual QStringList | realmlist () const =0 |
virtual void | reset ()=0 |
virtual Result | result () const =0 |
virtual void | serverFirstStep (const QString &mech, const QByteArray *clientInit)=0 |
virtual void | setClientParams (const QString *user, const QString *authzid, const SecureArray *pass, const QString *realm)=0 |
virtual void | setConstraints (SASL::AuthFlags f, int minSSF, int maxSSF)=0 |
virtual void | setup (const QString &service, const QString &host, const HostPort *local, const HostPort *remote, const QString &ext_id, int ext_ssf)=0 |
virtual int | ssf () const =0 |
virtual void | startClient (const QStringList &mechlist, bool allowClientSendFirst)=0 |
virtual void | startServer (const QString &realm, bool disableServerSendLast)=0 |
virtual QByteArray | stepData () const =0 |
virtual QByteArray | to_app ()=0 |
virtual QByteArray | to_net ()=0 |
virtual void | tryAgain ()=0 |
virtual void | update (const QByteArray &from_net, const QByteArray &from_app)=0 |
virtual QString | username () const =0 |
virtual bool | waitForResultsReady (int msecs)=0 |
![]() | |
virtual Context * | clone () const =0 |
Provider * | provider () const |
bool | sameProvider (const Context *c) const |
QString | type () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
Context (const Context &from) | |
Context (Provider *parent, const QString &type) | |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
SASL provider.
- Note
- This class is part of the provider plugin interface and should not be used directly by applications. You probably want SASL instead.
Definition at line 2601 of file qcaprovider.h.
Member Enumeration Documentation
◆ Result
Definition at line 2631 of file qcaprovider.h.
Constructor & Destructor Documentation
◆ SASLContext()
|
inline |
Standard constructor.
- Parameters
-
p the Provider associated with this context
Definition at line 2645 of file qcaprovider.h.
Member Function Documentation
◆ authCondition()
|
pure virtual |
Returns the reason for failure, if the authentication was not successful.
This is only valid after authentication failure.
◆ authzid()
|
pure virtual |
Returns the authzid attempting to authorize (server mode only)
This is only valid after receiving the AuthCheck result code.
◆ clientParams()
|
pure virtual |
Returns the needed/optional client parameters.
This is only valid after receiving the Params result code.
◆ encoded()
|
pure virtual |
Returns the number of bytes of plaintext data that is encoded inside of to_net()
◆ haveClientInit()
|
pure virtual |
Returns true if the client has initialization data.
◆ mech()
|
pure virtual |
Returns the mechanism selected.
◆ mechlist()
|
pure virtual |
Returns the mechanism list (server mode only)
◆ nextStep()
|
pure virtual |
Perform another step of the SASL authentication.
This function returns immediately, and completion is signaled with the resultsReady() signal.
On completion, result() and stepData() will be valid.
- Parameters
-
from_net the data from the "other side" of the protocol to be used for the next step.
◆ realmlist()
|
pure virtual |
Returns the realm list (client mode only)
This is only valid after receiving the Params result code and SASL::Params::canSendRealm is set to true.
◆ reset()
|
pure virtual |
Reset the object to its initial state.
◆ result()
|
pure virtual |
Returns the result code of an operation.
◆ resultsReady
|
signal |
Emit this when a startClient(), startServer(), serverFirstStep(), nextStep(), tryAgain(), or update() operation has completed.
◆ serverFirstStep()
|
pure virtual |
Finishes server startup.
This function returns immediately, and completion is signaled with the resultsReady() signal.
On completion, result() and stepData() will be valid. If result() is Success, then the session is now in the connected state.
- Parameters
-
mech the mechanism to use clientInit initial data from the client, or 0 if there is no such data
◆ setClientParams()
|
pure virtual |
Set some of the client parameters (pass 0 to not set a field)
- Parameters
-
user the user name authzid the authorization name / role pass the password realm the realm to authenticate in
◆ setConstraints()
|
pure virtual |
Set the constraints of the session using SSF values.
This function will be called before startClient() or startServer().
- Parameters
-
f the flags to use minSSF the minimum strength factor that is acceptable maxSSF the maximum strength factor that is acceptable
◆ setup()
|
pure virtual |
Configure a new session.
This function will be called before any other configuration functions.
- Parameters
-
service the name of the network service being provided by this application, which can be used by the SASL system for policy control. Examples: "imap", "xmpp" host the hostname that the application is interacting with or as local pointer to a HostPort representing the local end of a network socket, or 0 if this information is unknown or not available remote pointer to a HostPort representing the peer end of a network socket, or 0 if this information is unknown or not available ext_id the id to be used for SASL EXTERNAL (client only) ext_ssf the SSF of the external authentication channel (client only)
◆ ssf()
|
pure virtual |
Returns the SSF of the active SASL session.
This is only valid after authentication success.
◆ startClient()
|
pure virtual |
Begins the session in client mode, starting with the authentication.
This function returns immediately, and completion is signaled with the resultsReady() signal.
On completion, result(), mech(), haveClientInit(), and stepData() will be valid. If result() is Success, then the session is now in the connected state.
- Parameters
-
mechlist the list of mechanisms allowClientSendFirst whether the client sends first (true) or the server sends first (false)
◆ startServer()
|
pure virtual |
Begins the session in server mode, starting with the authentication.
This function returns immediately, and completion is signaled with the resultsReady() signal.
On completion, result() and mechlist() will be valid. The result() function will return Success or Error. If the result is Success, then serverFirstStep() will be called next.
- Parameters
-
realm the realm to authenticate in disableServerSendLast whether the client sends first (true) or the server sends first (false)
◆ stepData()
|
pure virtual |
Returns an authentication payload for to be transmitted over the network.
◆ to_app()
|
pure virtual |
Returns data that is decoded from the network and should be processed by the application.
◆ to_net()
|
pure virtual |
Returns data that should be sent across the network (for the security layer)
◆ tryAgain()
|
pure virtual |
Attempt the most recent operation again.
This is used if the result() of an operation is Params or AuthCheck.
This function returns immediately, and completion is signaled with the resultsReady() signal.
On completion, result() and stepData() will be valid.
◆ update()
|
pure virtual |
Performs one iteration of the SASL security layer processing.
This function returns immediately, and completion is signaled with the resultsReady() signal.
On completion, result(), to_net(), encoded(), and to_app() will be valid. The result() function will return Success or Error.
- Parameters
-
from_net the data from the "other side" of the protocol from_app the data from the application of the protocol
◆ username()
|
pure virtual |
Returns the username attempting to authenticate (server mode only)
This is only valid after receiving the AuthCheck result code.
◆ waitForResultsReady()
|
pure virtual |
Waits for a startClient(), startServer(), serverFirstStep(), nextStep(), tryAgain(), or update() operation to complete.
In this case, the resultsReady() signal is not emitted. Returns true if the operation completed or false if this function times out.
This function is blocking.
- Parameters
-
msecs number of milliseconds to wait (-1 to wait forever)
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:01:48 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.