Akonadi::EntityTreeModel
#include <entitytreemodel.h>
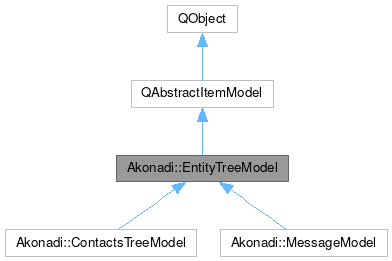
Signals | |
void | collectionFetched (int collectionId) |
void | collectionPopulated (Akonadi::Collection::Id collectionId) |
void | collectionTreeFetched (const Akonadi::Collection::List &collections) |
void | errorOccurred (const QString &message) |
Public Member Functions | |
EntityTreeModel (Monitor *monitor, QObject *parent=nullptr) | |
~EntityTreeModel () override | |
bool | canFetchMore (const QModelIndex &parent) const override |
CollectionFetchStrategy | collectionFetchStrategy () const |
int | columnCount (const QModelIndex &parent=QModelIndex()) const override |
QVariant | data (const QModelIndex &index, int role=Qt::DisplayRole) const override |
bool | dropMimeData (const QMimeData *data, Qt::DropAction action, int row, int column, const QModelIndex &parent) override |
void | fetchMore (const QModelIndex &parent) override |
Qt::ItemFlags | flags (const QModelIndex &index) const override |
bool | hasChildren (const QModelIndex &parent=QModelIndex()) const override |
QVariant | headerData (int section, Qt::Orientation orientation, int role=Qt::DisplayRole) const override |
bool | includeRootCollection () const |
QModelIndex | index (int row, int column, const QModelIndex &parent=QModelIndex()) const override |
bool | isCollectionPopulated (Akonadi::Collection::Id) const |
bool | isCollectionTreeFetched () const |
bool | isFullyPopulated () const |
ItemPopulationStrategy | itemPopulationStrategy () const |
Akonadi::CollectionFetchScope::ListFilter | listFilter () const |
QModelIndexList | match (const QModelIndex &start, int role, const QVariant &value, int hits=1, Qt::MatchFlags flags=Qt::MatchFlags(Qt::MatchStartsWith|Qt::MatchWrap)) const override |
QMimeData * | mimeData (const QModelIndexList &indexes) const override |
QStringList | mimeTypes () const override |
QModelIndex | parent (const QModelIndex &index) const override |
Q_ENUM (Roles) | |
QHash< int, QByteArray > | roleNames () const override |
QString | rootCollectionDisplayName () const |
int | rowCount (const QModelIndex &parent=QModelIndex()) const override |
void | setCollectionFetchStrategy (CollectionFetchStrategy strategy) |
void | setCollectionMonitored (const Akonadi::Collection &col, bool monitored=true) |
void | setCollectionsMonitored (const Akonadi::Collection::List &collections) |
bool | setData (const QModelIndex &index, const QVariant &value, int role=Qt::EditRole) override |
void | setIncludeRootCollection (bool include) |
void | setItemPopulationStrategy (ItemPopulationStrategy strategy) |
void | setListFilter (Akonadi::CollectionFetchScope::ListFilter filter) |
void | setRootCollectionDisplayName (const QString &name) |
void | setShowSystemEntities (bool show) |
Qt::DropActions | supportedDropActions () const override |
bool | systemEntitiesShown () const |
![]() | |
QAbstractItemModel (QObject *parent) | |
virtual QModelIndex | buddy (const QModelIndex &index) const const |
virtual bool | canDropMimeData (const QMimeData *data, Qt::DropAction action, int row, int column, const QModelIndex &parent) const const |
bool | checkIndex (const QModelIndex &index, CheckIndexOptions options) const const |
virtual bool | clearItemData (const QModelIndex &index) |
void | columnsAboutToBeInserted (const QModelIndex &parent, int first, int last) |
void | columnsAboutToBeMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationColumn) |
void | columnsAboutToBeRemoved (const QModelIndex &parent, int first, int last) |
void | columnsInserted (const QModelIndex &parent, int first, int last) |
void | columnsMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationColumn) |
void | columnsRemoved (const QModelIndex &parent, int first, int last) |
void | dataChanged (const QModelIndex &topLeft, const QModelIndex &bottomRight, const QList< int > &roles) |
bool | hasIndex (int row, int column, const QModelIndex &parent) const const |
void | headerDataChanged (Qt::Orientation orientation, int first, int last) |
bool | insertColumn (int column, const QModelIndex &parent) |
virtual bool | insertColumns (int column, int count, const QModelIndex &parent) |
bool | insertRow (int row, const QModelIndex &parent) |
virtual bool | insertRows (int row, int count, const QModelIndex &parent) |
virtual QMap< int, QVariant > | itemData (const QModelIndex &index) const const |
void | layoutAboutToBeChanged (const QList< QPersistentModelIndex > &parents, QAbstractItemModel::LayoutChangeHint hint) |
void | layoutChanged (const QList< QPersistentModelIndex > &parents, QAbstractItemModel::LayoutChangeHint hint) |
void | modelAboutToBeReset () |
void | modelReset () |
bool | moveColumn (const QModelIndex &sourceParent, int sourceColumn, const QModelIndex &destinationParent, int destinationChild) |
virtual bool | moveColumns (const QModelIndex &sourceParent, int sourceColumn, int count, const QModelIndex &destinationParent, int destinationChild) |
bool | moveRow (const QModelIndex &sourceParent, int sourceRow, const QModelIndex &destinationParent, int destinationChild) |
virtual bool | moveRows (const QModelIndex &sourceParent, int sourceRow, int count, const QModelIndex &destinationParent, int destinationChild) |
virtual void | multiData (const QModelIndex &index, QModelRoleDataSpan roleDataSpan) const const |
bool | removeColumn (int column, const QModelIndex &parent) |
virtual bool | removeColumns (int column, int count, const QModelIndex &parent) |
bool | removeRow (int row, const QModelIndex &parent) |
virtual bool | removeRows (int row, int count, const QModelIndex &parent) |
virtual void | revert () |
void | rowsAboutToBeInserted (const QModelIndex &parent, int start, int end) |
void | rowsAboutToBeMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationRow) |
void | rowsAboutToBeRemoved (const QModelIndex &parent, int first, int last) |
void | rowsInserted (const QModelIndex &parent, int first, int last) |
void | rowsMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationRow) |
void | rowsRemoved (const QModelIndex &parent, int first, int last) |
virtual bool | setHeaderData (int section, Qt::Orientation orientation, const QVariant &value, int role) |
virtual bool | setItemData (const QModelIndex &index, const QMap< int, QVariant > &roles) |
virtual QModelIndex | sibling (int row, int column, const QModelIndex &index) const const |
virtual void | sort (int column, Qt::SortOrder order) |
virtual QSize | span (const QModelIndex &index) const const |
virtual bool | submit () |
virtual Qt::DropActions | supportedDragActions () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static QModelIndexList | modelIndexesForItem (const QAbstractItemModel *model, const Item &item) |
static QModelIndex | modelIndexForCollection (const QAbstractItemModel *model, const Collection &collection) |
static Collection | updatedCollection (const QAbstractItemModel *model, const Collection &col) |
static Collection | updatedCollection (const QAbstractItemModel *model, qint64 collectionId) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
void | clearAndReset () |
virtual int | entityColumnCount (HeaderGroup headerGroup) const |
virtual QVariant | entityData (const Collection &collection, int column, int role=Qt::DisplayRole) const |
virtual QVariant | entityData (const Item &item, int column, int role=Qt::DisplayRole) const |
virtual QVariant | entityHeaderData (int section, Qt::Orientation orientation, int role, HeaderGroup headerGroup) const |
![]() | |
void | beginInsertColumns (const QModelIndex &parent, int first, int last) |
void | beginInsertRows (const QModelIndex &parent, int first, int last) |
bool | beginMoveColumns (const QModelIndex &sourceParent, int sourceFirst, int sourceLast, const QModelIndex &destinationParent, int destinationChild) |
bool | beginMoveRows (const QModelIndex &sourceParent, int sourceFirst, int sourceLast, const QModelIndex &destinationParent, int destinationChild) |
void | beginRemoveColumns (const QModelIndex &parent, int first, int last) |
void | beginRemoveRows (const QModelIndex &parent, int first, int last) |
void | beginResetModel () |
void | changePersistentIndex (const QModelIndex &from, const QModelIndex &to) |
void | changePersistentIndexList (const QModelIndexList &from, const QModelIndexList &to) |
QModelIndex | createIndex (int row, int column, const void *ptr) const const |
QModelIndex | createIndex (int row, int column, quintptr id) const const |
void | endInsertColumns () |
void | endInsertRows () |
void | endMoveColumns () |
void | endMoveRows () |
void | endRemoveColumns () |
void | endRemoveRows () |
void | endResetModel () |
QModelIndexList | persistentIndexList () const const |
virtual void | resetInternalData () |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | CheckIndexOptions |
DoNotUseParent | |
HorizontalSortHint | |
IndexIsValid | |
NoLayoutChangeHint | |
NoOption | |
ParentIsInvalid | |
VerticalSortHint | |
![]() | |
typedef | QObjectList |
Detailed Description
A model for collections and items together.
Akonadi models and views provide a high level way to interact with the akonadi server. Most applications will use these classes.
Models provide an interface for viewing, updating, deleting and moving Items and Collections. Additionally, the models are updated automatically if another application changes the data or inserts of deletes items etc.
- Note
- The EntityTreeModel should be used with the EntityTreeView or the EntityListView class either directly or indirectly via proxy models.
Retrieving Collections and Items from the model
If you want to retrieve and Item or Collection from the model, and already have a valid QModelIndex for the correct row, the Collection can be retrieved like this:
And similarly for Items. This works even if there is a proxy model between the calling code and the EntityTreeModel.
If you want to retrieve a Collection for a particular Collection::Id and you do not yet have a valid QModelIndex, use modelIndexForCollection.
Using EntityTreeModel in your application
The responsibilities which fall to the application developer are
- Configuring the Monitor and EntityTreeModel
- Making use of this class via proxy models
- Subclassing for type specific display information
Creating and configuring the EntityTreeModel
This class is a wrapper around a Akonadi::Monitor object. The model represents a part of the collection and item tree configured in the Monitor. The structure of the model mirrors the structure of Collections and Items on the Akonadi server.
The following code creates a model which fetches items and collections relevant to addressees (contacts), and automatically manages keeping the items up to date.
The EntityTreeModel will show items of a different type by changing the line
to a different mimetype. KContacts::Addressee::mimeType() is an alias for "text/directory". If changed to KMime::Message::mimeType() (an alias for "message/rfc822") the model would instead contain emails. The model can be configured to contain items of any mimetype known to Akonadi.
- Note
- The EntityTreeModel does some extra configuration on the Monitor, such as setting itemFetchScope() and collectionFetchScope() to retrieve all ancestors. This is necessary for proper function of the model.
- See also
- Akonadi::ItemFetchScope::AncestorRetrieval.
- akonadi-mimetypes.
The EntityTreeModel can be further configured for certain behaviours such as fetching of collections and items.
The model can be configured to not fetch items into the model (ie, fetch collections only) by setting
The items may be fetched lazily, i.e. not inserted into the model until request by the user for performance reasons.
The Collection tree is always built immediately if Collections are to be fetched.
This will typically be used with a EntityMimeTypeFilterModel in a configuration such as KMail or AkonadiConsole.
The CollectionFetchStrategy determines how the model will be populated with Collections. That is, if FetchNoCollections is set, no collections beyond the root of the model will be fetched. This can be used in combination with setting a particular Collection to monitor.
This has the effect of creating a model of only a list of Items, and not collections. This is similar in behaviour and aims to the ItemModel. By using FetchFirstLevelCollections instead, a mixed list of entities can be created.
- Note
- It is important that you set only one Collection to be monitored in the monitor object. This one collection will be the root of the tree. If you need a model with a more complex structure, consider monitoring a common ancestor and using a SelectionProxyModel.
- See also
- lazy-model-population
It is also possible to show the root Collection as part of the selectable model:
By default the displayed name of the root collection is '[*]', because it doesn't require i18n, and is generic. It can be changed too.
This feature is used in KAddressBook.
If items are to be fetched by the model, it is necessary to specify which parts of the items are to be fetched, using the ItemFetchScope class. By default, only the basic metadata is fetched. To fetch all item data, including all attributes:
Using EntityTreeModel with Proxy models
An Akonadi::SelectionProxyModel can be used to simplify managing selection in one view through multiple proxy models to a representation in another view. The selectionModel of the initial view is used to create a proxied model which filters out anything not related to the current selection.
The SelectionProxyModel can handle complex selections.
See the KSelectionProxyModel documentation for the valid configurations of a Akonadi::SelectionProxyModel.
Obviously, the SelectionProxyModel may be used in a view, or further processed with other proxy models. Typically, the result from this model will be further filtered to remove collections from the item list as in the above example.
There are several advantages of using EntityTreeModel with the SelectionProxyModel, namely the items can be fetched and cached instead of being fetched many times, and the chain of proxies from the core model to the view is automatically handled. There is no need to manage all the mapToSource and mapFromSource calls manually.
A KDescendantsProxyModel can be used to represent all descendants of a model as a flat list. For example, to show all descendant items in a selected Collection in a list:
Note that it is important in this case to use the KDescendantsProxyModel before the EntityMimeTypeFilterModel. Otherwise, by filtering out the collections first, you would also be filtering out their child items.
This pattern is used in KAddressBook.
It would not make sense to use a KDescendantsProxyModel with LazyPopulation.
Subclassing EntityTreeModel
Usually an application will create a subclass of an EntityTreeModel and use that in several views via proxy models.
The subclassing is necessary in order for the data in the model to have type-specific representation in applications
For example, the headerData for an EntityTreeModel will be different depending on whether it is in a view showing only Collections in which case the header data should be "AddressBooks" for example, or only Items, in which case the headerData would be for example "Family Name", "Given Name" and "Email address" for contacts or "Subject", "Sender", "Date" in the case of emails.
Additionally, the actual data shown in the rows of the model should be type specific.
In summary, it must be possible to have different numbers of columns, different data in the rows of those columns, and different titles for each column depending on the contents of the view.
The way this is accomplished is by using the EntityMimeTypeFilterModel for splitting the model into a "CollectionTree" and an "Item List" as in the above example, and using a type-specific EntityTreeModel subclass to return the type-specific data, typically for only one type (for example, contacts or emails).
The following protected virtual methods should be implemented in the subclass:
int entityColumnCount( HeaderGroup headerGroup ) const;
– Implement to return the number of columns for a HeaderGroup. If the HeaderGroup is CollectionTreeHeaders, return the number of columns to display for the Collection tree, and if it is ItemListHeaders, return the number of columns to display for the item. In the case of addressee, this could be for example, two (for given name and family name) or for emails it could be three (for subject, sender, date). This is a decision of the subclass implementor.QVariant entityHeaderData( int section, Qt::Orientation orientation, int role, HeaderGroup headerGroup ) const;
– Implement to return the data for each section for a HeaderGroup. For example, if the header group is CollectionTreeHeaders in a contacts model, the string "Address books" might be returned for column 0, whereas if the headerGroup is ItemListHeaders, the strings "Given Name", "Family Name", "Email Address" might be returned for the columns 0, 1, and 2.QVariant entityData( const Collection &collection, int column, int role = Qt::DisplayRole ) const;
– Implement to return data for a particular Collection. Typically this will be the name of the collection or the EntityDisplayAttribute.QVariant entityData(const Item &item, int column, int role = Qt::DisplayRole) const;
– Implement to return the data for a particular item and column. In the case of email for example, this would be the actual subject, sender and date of the email.
- Note
- The entityData methods are just for convenience. the QAbstractItemModel::data method can be overridden if required.
The application writer must then properly configure proxy models for the views, so that the correct data is shown in the correct view. That is the purpose of these lines in the above example
Progress reporting
The EntityTreeModel uses asynchronous Akonadi::Job instances to fill and update itself. For example, a job is run to fetch the contents of collections (that is, list the items in it). Additionally, individual Akonadi::Items can be fetched in different parts at different times.
To indicate that such a job is underway, the EntityTreeModel makes the FetchState available. The FetchState returned from a QModelIndex representing a Akonadi::Collection will be FetchingState if a listing of the items in that collection is underway, otherwise the state is IdleState.
- Since
- 4.4
Definition at line 305 of file entitytreemodel.h.
Member Enumeration Documentation
◆ CollectionFetchStrategy
Describes what collections shall be fetched by and represent in the model.
Enumerator | |
---|---|
FetchNoCollections | Fetches nothing. This creates an empty model. |
FetchFirstLevelChildCollections | Fetches first level collections in the root collection. |
FetchCollectionsRecursive | Fetches collections in the root collection recursively. This is the default. |
InvisibleCollectionFetch | Fetches collections, but does not put them in the model. This can be used to create a list of items in all collections. The ParentCollectionRole can still be used to retrieve the parent collection of an Item.
|
Definition at line 485 of file entitytreemodel.h.
◆ FetchState
Describes the state of fetch jobs related to particular collections.
- Since
- 4.5
Enumerator | |
---|---|
IdleState | There is no fetch of items in this collection in progress. |
FetchingState | There is a fetch of items in this collection in progress. |
Definition at line 361 of file entitytreemodel.h.
◆ HeaderGroup
Describes what header information the model shall return.
Definition at line 371 of file entitytreemodel.h.
◆ ItemPopulationStrategy
Describes how the model should populated its items.
Definition at line 396 of file entitytreemodel.h.
◆ Roles
Describes the roles for items.
Roles for collections are defined by the superclass.
Enumerator | |
---|---|
ItemIdRole | The item id. |
ItemRole | The Item. |
MimeTypeRole | The mimetype of the entity. |
CollectionIdRole | The collection id. |
CollectionRole | The collection. |
RemoteIdRole | The remoteId of the entity. |
CollectionChildOrderRole | Ordered list of child items if available. |
ParentCollectionRole | The parent collection of the entity. |
ColumnCountRole | Used by proxies to determine the number of columns for a header group. |
LoadedPartsRole | Parts available in the model for the item. |
AvailablePartsRole | Parts available in the Akonadi server for the item. |
SessionRole | The Session used by this model |
CollectionRefRole | Used to increase the reference count on a Collection |
CollectionDerefRole | Used to decrease the reference count on a Collection |
PendingCutRole | Used to indicate items which are to be cut. |
EntityUrlRole | The akonadi:/ Url of the entity as a string. Item urls will contain the mimetype. |
UnreadCountRole | Returns the number of unread items in a collection.
|
FetchStateRole | Returns the FetchState of a particular item.
|
IsPopulatedRole | Returns whether a Collection has been populated, i.e. whether its items have been fetched.
|
OriginalCollectionNameRole | Returns original name for collection.
|
DisplayNameRole | Returns the same as Qt::DisplayRole. |
UserRole | First role for user extensions. |
TerminalUserRole | Last role for user extensions. Don't use a role beyond this or headerData will break. |
Definition at line 313 of file entitytreemodel.h.
Constructor & Destructor Documentation
◆ EntityTreeModel()
Creates a new entity tree model.
- Parameters
-
monitor The Monitor whose entities should be represented in the model. parent The parent object.
Definition at line 36 of file entitytreemodel.cpp.
◆ ~EntityTreeModel()
|
override |
Destroys the entity tree model.
Definition at line 51 of file entitytreemodel.cpp.
Member Function Documentation
◆ canFetchMore()
|
nodiscardoverridevirtual |
Reimplemented from QAbstractItemModel.
Definition at line 813 of file entitytreemodel.cpp.
◆ clearAndReset()
|
protected |
Clears and resets the model.
Always call this instead of the reset method in the superclass. Using the reset method will not reliably clear or refill the model.
Definition at line 107 of file entitytreemodel.cpp.
◆ collectionFetched
|
signal |
Emitted once a collection has been fetched for the very first time.
This is like a dataChanged(), but specific to the initial loading, in order to update the GUI (window caption, state of actions). Usually, the GUI uses Akonadi::Monitor to be notified of further changes to the collections.
- Parameters
-
collectionId the identifier of the fetched collection
- Since
- 4.9.3
◆ collectionFetchStrategy()
|
nodiscard |
Returns the collection fetch strategy of the model.
Definition at line 1036 of file entitytreemodel.cpp.
◆ collectionPopulated
|
signal |
Signal emitted when a collection has been populated, i.e.
its items have been fetched.
- Parameters
-
collectionId id of the collection which has been populated
- See also
- collectionTreeFetched
- Since
- 4.10
◆ collectionTreeFetched
|
signal |
Signal emitted when the collection tree has been fetched for the first time.
- Parameters
-
collections list of collections which have been fetched
- Since
- 4.10
◆ columnCount()
|
nodiscardoverridevirtual |
Implements QAbstractItemModel.
Definition at line 143 of file entitytreemodel.cpp.
◆ data()
|
nodiscardoverridevirtual |
Implements QAbstractItemModel.
Definition at line 222 of file entitytreemodel.cpp.
◆ dropMimeData()
|
overridevirtual |
Reimplemented from QAbstractItemModel.
Definition at line 415 of file entitytreemodel.cpp.
◆ entityColumnCount()
|
protectedvirtual |
Definition at line 650 of file entitytreemodel.cpp.
◆ entityData() [1/2]
|
protectedvirtual |
Provided for convenience of subclasses.
Definition at line 181 of file entitytreemodel.cpp.
◆ entityData() [2/2]
|
protectedvirtual |
Provided for convenience of subclasses.
Definition at line 153 of file entitytreemodel.cpp.
◆ entityHeaderData()
|
protectedvirtual |
Reimplement this to provide different header data.
This is needed when using one model with multiple proxies and views, and each should show different header data.
Definition at line 658 of file entitytreemodel.cpp.
◆ errorOccurred
|
signal |
Emitted when an error occurred.
- Since
- 6.4.0
◆ fetchMore()
|
overridevirtual |
Reimplemented from QAbstractItemModel.
Definition at line 819 of file entitytreemodel.cpp.
◆ flags()
|
nodiscardoverridevirtual |
Reimplemented from QAbstractItemModel.
Definition at line 334 of file entitytreemodel.cpp.
◆ hasChildren()
|
nodiscardoverridevirtual |
Reimplemented from QAbstractItemModel.
Definition at line 845 of file entitytreemodel.cpp.
◆ headerData()
|
nodiscardoverridevirtual |
Reimplemented from QAbstractItemModel.
Definition at line 674 of file entitytreemodel.cpp.
◆ includeRootCollection()
|
nodiscard |
Returns whether the root collection is provided by the model.
Definition at line 991 of file entitytreemodel.cpp.
◆ index()
|
nodiscardoverridevirtual |
Implements QAbstractItemModel.
Definition at line 536 of file entitytreemodel.cpp.
◆ isCollectionPopulated()
|
nodiscard |
Returns whether the collection has been populated.
- See also
- collectionPopulated
- Since
- 4.12
Definition at line 866 of file entitytreemodel.cpp.
◆ isCollectionTreeFetched()
|
nodiscard |
Returns whether the collection tree has been fetched at initialisation.
- See also
- collectionTreeFetched
- Since
- 4.10
Definition at line 860 of file entitytreemodel.cpp.
◆ isFullyPopulated()
|
nodiscard |
Returns whether the model is fully populated.
Returns true once the collection tree has been fetched and all collections have been populated.
- Since
- 4.14
Definition at line 872 of file entitytreemodel.cpp.
◆ itemPopulationStrategy()
|
nodiscard |
Returns the item population strategy of the model.
Definition at line 977 of file entitytreemodel.cpp.
◆ listFilter()
|
nodiscard |
◆ match()
|
nodiscardoverridevirtual |
Reimplemented to handle the AmazingCompletionRole.
Reimplemented from QAbstractItemModel.
Definition at line 878 of file entitytreemodel.cpp.
◆ mimeData()
|
nodiscardoverridevirtual |
Reimplemented from QAbstractItemModel.
Definition at line 682 of file entitytreemodel.cpp.
◆ mimeTypes()
|
nodiscardoverridevirtual |
Reimplemented from QAbstractItemModel.
Definition at line 409 of file entitytreemodel.cpp.
◆ modelIndexesForItem()
|
static |
Returns a QModelIndex in model
which points to item
.
This method can be used through proxy models if model
is a proxy model.
- Parameters
-
model the model to query for the item item the item to look for
- See also
- modelIndexForCollection
- Since
- 4.5
Definition at line 1078 of file entitytreemodel.cpp.
◆ modelIndexForCollection()
|
static |
Returns a QModelIndex in model
which points to collection
.
This method can be used through proxy models if model
is a proxy model.
This can be useful for example if an id is stored in a config file and needs to be used in the application.
Note however, that to restore view state such as scrolling, selection and expansion of items in trees, the ETMViewStateSaver can be used for convenience.
- See also
- modelIndexesForItem
- Since
- 4.5
Definition at line 1066 of file entitytreemodel.cpp.
◆ parent()
|
nodiscardoverridevirtual |
Implements QAbstractItemModel.
Definition at line 571 of file entitytreemodel.cpp.
◆ roleNames()
|
nodiscardoverridevirtual |
Reimplemented from QAbstractItemModel.
Definition at line 114 of file entitytreemodel.cpp.
◆ rootCollectionDisplayName()
|
nodiscard |
Returns the display name of the root collection.
Definition at line 1005 of file entitytreemodel.cpp.
◆ rowCount()
|
nodiscardoverridevirtual |
Implements QAbstractItemModel.
Definition at line 612 of file entitytreemodel.cpp.
◆ setCollectionFetchStrategy()
void EntityTreeModel::setCollectionFetchStrategy | ( | CollectionFetchStrategy | strategy | ) |
Sets the collection fetch strategy
of the model.
Definition at line 1011 of file entitytreemodel.cpp.
◆ setCollectionMonitored()
void EntityTreeModel::setCollectionMonitored | ( | const Akonadi::Collection & | col, |
bool | monitored = true ) |
Adds or removes a specific collection from the monitored set without resetting the model.
Only call this if you're monitoring specific collections (not mimetype/resources/items).
- Since
- 4.14
- See also
- setCollectionsMonitored()
Definition at line 89 of file entitytreemodel.cpp.
◆ setCollectionsMonitored()
void EntityTreeModel::setCollectionsMonitored | ( | const Akonadi::Collection::List & | collections | ) |
Monitors the specified collections and resets the model.
- Since
- 4.14
Definition at line 75 of file entitytreemodel.cpp.
◆ setData()
|
overridevirtual |
Reimplemented from QAbstractItemModel.
Definition at line 718 of file entitytreemodel.cpp.
◆ setIncludeRootCollection()
void EntityTreeModel::setIncludeRootCollection | ( | bool | include | ) |
Sets whether the root collection shall be provided by the model.
- Parameters
-
include enables root collection if set as true
- See also
- setRootCollectionDisplayName()
Definition at line 983 of file entitytreemodel.cpp.
◆ setItemPopulationStrategy()
void EntityTreeModel::setItemPopulationStrategy | ( | ItemPopulationStrategy | strategy | ) |
Sets the item population strategy
of the model.
Definition at line 950 of file entitytreemodel.cpp.
◆ setListFilter()
void EntityTreeModel::setListFilter | ( | Akonadi::CollectionFetchScope::ListFilter | filter | ) |
◆ setRootCollectionDisplayName()
void EntityTreeModel::setRootCollectionDisplayName | ( | const QString & | name | ) |
Sets the display name
of the root collection of the model.
The default display name is "[*]".
- Parameters
-
name the name to display for the root collection
- Note
- The display name for the root collection is only used if the root collection has been included with setIncludeRootCollection().
Definition at line 997 of file entitytreemodel.cpp.
◆ setShowSystemEntities()
void EntityTreeModel::setShowSystemEntities | ( | bool | show | ) |
Some Entities are hidden in the model, but exist for internal purposes, for example, custom object directories in groupware resources.
They are hidden by default, but can be shown by setting show
to true.
- Parameters
-
show enabled displaying of hidden entities if set as true
Most applications will not need to use this feature.
Definition at line 101 of file entitytreemodel.cpp.
◆ supportedDropActions()
|
nodiscardoverridevirtual |
Reimplemented from QAbstractItemModel.
Definition at line 404 of file entitytreemodel.cpp.
◆ systemEntitiesShown()
|
nodiscard |
Returns true
if internal system entities are shown, and false
otherwise.
Definition at line 95 of file entitytreemodel.cpp.
◆ updatedCollection() [1/2]
|
static |
Definition at line 1115 of file entitytreemodel.cpp.
◆ updatedCollection() [2/2]
|
static |
Returns an Akonadi::Collection from the model
based on given collectionId
.
This is faster and simpler than retrieving a full Collection from the ETM by using modelIndexForCollection() and then querying for the index data.
Definition at line 1098 of file entitytreemodel.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 25 2025 11:47:47 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.