EclipseHandler
#include <eclipsehandler.h>
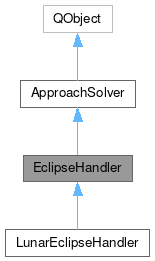
Public Types | |
typedef QVector< EclipseEvent_s > | EclipseVector |
Signals | |
void | signalComputationFinished () |
void | signalEventFound (EclipseEvent_s event) |
void | signalProgress (int) |
![]() | |
void | solverMadeProgress (int progress) |
Public Member Functions | |
EclipseHandler (QObject *parent=nullptr) | |
virtual EclipseVector | computeEclipses (long double startJD, long double endJD)=0 |
QVector< EclipseEvent_s > | getEvents () |
![]() | |
ApproachSolver (QObject *parent=nullptr) | |
QMap< long double, dms > | findClosestApproach (long double startJD, long double stopJD, const std::function< void(long double, dms)> &callback={}) |
GeoLocation * | getGeoLocation () |
void | setGeoLocation (GeoLocation *geo) |
void | setMaxSeparation (dms sep) |
void | setMaxSeparation (double sep) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
virtual double | findInitialStep (long double startJD, long double stopJD) override |
![]() | |
virtual dms | findDistance ()=0 |
bool | findPrecise (QPair< long double, dms > *out, long double jd, double step, int prevSign) |
dms | findSkyPointDistance (SkyPoint *obj1, SkyPoint *obj2) |
virtual double | getMaxSeparation () |
virtual void | updatePositions (long double jd)=0 |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
KSPlanet | m_Earth |
Detailed Description
The EclipseHandler class.
This is a base class for providing a common interface for eclipse events which can be quite different in nature. It is meant to be subclassed. (Check LunarEclipseHandler as an example)
- Todo
- remove uglieness from KSConjunct (export m_object... functionality to separate class!, that findinitialstepsize isn't nice either)
- Note
- I've integrated the
findDetails
stuff in the eclipse handler because it already has thebeef
it takes (instances and methods). OOP is not always the way.
Definition at line 118 of file eclipsehandler.h.
Member Typedef Documentation
◆ EclipseVector
typedef QVector<EclipseEvent_s> EclipseHandler::EclipseVector |
Definition at line 122 of file eclipsehandler.h.
Constructor & Destructor Documentation
◆ EclipseHandler()
|
explicit |
Definition at line 20 of file eclipsehandler.cpp.
◆ ~EclipseHandler()
|
overridevirtual |
Definition at line 24 of file eclipsehandler.cpp.
Member Function Documentation
◆ computeEclipses()
|
pure virtual |
compute
Implements the details for finding all the eclipses in a given time-frame. Should call findEclipse intelligently. e.g. only if the moon is full for lunar eclipses et-cetera
- Returns
- A vector of shared pointers to eclipse events.
Implemented in LunarEclipseHandler.
◆ findInitialStep()
|
inlineoverrideprotectedvirtual |
findStep
- Returns
- the step size used by findClosestApproach (in Julian Days)
Make this as big as possible. The bigger it is, the more likely is a skip over...
Implements ApproachSolver.
Reimplemented in LunarEclipseHandler.
Definition at line 168 of file eclipsehandler.h.
◆ getEvents()
|
inline |
getEvents
May be used if the return value of computeEclipses is being ignored.
- Note
- The underlying vector changes after every call to computeEclipses.
- Returns
- A vector of shared pointers to eclipse events.
Definition at line 144 of file eclipsehandler.h.
◆ signalComputationFinished
|
signal |
signalComputationFinished
signals the end of the computation
◆ signalEventFound
|
signal |
signalEventFound
A signal to be dispatched as soon as a new Event is found.
- Note
- Has to emitted by a subclass!
- Parameters
-
event
◆ signalProgress
|
signal |
signalProgress
gives the progress of the computation in percent
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Mar 7 2025 11:55:45 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.