ISD::Mount
#include <indimount.h>
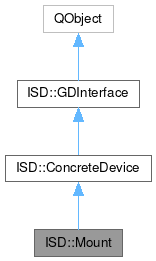
Signals | |
void | axisReversed (INDI_EQ_AXIS axis, bool reversed) |
void | newCoords (const SkyPoint &position, const PierSide pierside, const dms &ha) |
void | newParkStatus (ISD::ParkStatus status) |
void | newStatus (ISD::Mount::Status status) |
void | newTarget (SkyPoint ¤tCoords) |
void | newTargetName (const QString &name) |
void | pierSideChanged (PierSide side) |
void | slewRateChanged (int rate) |
![]() | |
void | Connected () |
void | Disconnected () |
void | propertyDefined (INDI::Property prop) |
void | propertyDeleted (INDI::Property prop) |
void | propertyUpdated (INDI::Property prop) |
void | ready () |
Public Slots | |
bool | abort () |
bool | park () |
bool | setCustomTrackRate (double raRate, double deRate) |
bool | setSlewRate (int index) |
bool | setTrackEnabled (bool enable) |
bool | setTrackMode (uint8_t index) |
bool | unpark () |
Public Member Functions | |
Mount (GenericDevice *parent) | |
bool | canAbort () |
bool | canControlTrack () const |
bool | canCustomPark () |
bool | canFlip () |
bool | canGoto () |
bool | canGuide () |
bool | canPark () |
bool | canSync () |
bool | canTrackSatellite () |
void | centerLock () |
void | centerUnlock () |
bool | clearAlignmentModel () |
bool | clearParking () |
const SkyPoint & | currentCoordinates () const |
bool | doPulse (GuideDirection dir, int msecs) |
bool | doPulse (GuideDirection ra_dir, int ra_msecs, GuideDirection dec_dir, int dec_msecs) |
void | find () |
bool | getCustomTrackRate (double &raRate, double &deRate) |
bool | getEqCoords (double *ra, double *dec) |
QString | getManualMotionString () const |
int | getSlewRate () const |
bool | getTrackMode (uint8_t &index) |
bool | hasAlignmentModel () |
bool | hasCustomTrackRate () const |
bool | hasSlewRates () |
bool | hasTrackModes () const |
const dms | hourAngle () const |
bool | isInMotion () |
bool | isJ2000 () |
bool | isParked () |
bool | isReversed (INDI_EQ_AXIS axis) |
bool | isSlewing () |
bool | isTracking () |
bool | MoveNS (VerticalMotion dir, MotionCommand cmd) |
bool | MoveWE (HorizontalMotion dir, MotionCommand cmd) |
ParkStatus | parkStatus () |
PierSide | pierSide () const |
void | processNumber (INDI::Property prop) override |
void | processSwitch (INDI::Property prop) override |
void | processText (INDI::Property prop) override |
void | registerProperty (INDI::Property prop) override |
bool | sendParkingOptionCommand (ParkOptionCommand command) |
bool | setAlignmentModelEnabled (bool enable) |
void | setAltLimits (double minAltitude, double maxAltitude, bool trackingOnly) |
void | setCustomParking (SkyPoint *coords=nullptr) |
bool | setReversedEnabled (INDI_EQ_AXIS axis, bool enabled) |
bool | setSatelliteTLEandTrack (QString tle, const KStarsDateTime satPassStart, const KStarsDateTime satPassEnd) |
bool | Slew (double ra, double dec, bool flip=false) |
bool | Slew (SkyPoint *ScopeTarget, bool flip=false) |
QStringList | slewRates () |
Status | status () |
Status | status (INumberVectorProperty *nvp) |
const QString | statusString (Status status, bool translated=true) const |
bool | StopNS () |
void | stopTimers () |
bool | StopWE () |
bool | Sync (double ra, double dec) |
bool | Sync (SkyPoint *ScopeTarget) |
![]() | |
ConcreteDevice (GenericDevice *parent) | |
void | Connect () |
void | Disconnect () |
GenericDevice * | genericDevice () const |
INDI::PropertyBlob | getBLOB (const QString &name) const |
const QString & | getDeviceName () const |
const QSharedPointer< DriverInfo > & | getDriverInfo () const |
uint32_t | getDriverInterface () |
const QString & | getDUBSObjectPath () const |
INDI::PropertyLight | getLight (const QString &name) const |
QString | getMessage (int id) const |
bool | getMinMaxStep (const QString &propName, const QString &elementName, double *min, double *max, double *step) const |
INDI::PropertyNumber | getNumber (const QString &name) const |
IPerm | getPermission (const QString &propName) const |
Properties | getProperties () const |
INDI::Property | getProperty (const QString &name) const |
IPState | getState (const QString &propName) const |
INDI::PropertySwitch | getSwitch (const QString &name) const |
INDI::PropertyText | getText (const QString &name) const |
bool | isConnected () const |
bool | isReady () const |
virtual bool | processBLOB (INDI::Property) override |
virtual void | processLight (INDI::Property) override |
virtual void | processMessage (int) override |
void | processProperties () |
void | registeProperties () |
virtual void | removeProperty (INDI::Property) override |
void | sendNewProperty (INDI::Property prop) |
bool | setConfig (INDIConfig tConfig) |
virtual void | updateProperty (INDI::Property prop) override |
![]() | |
GDInterface (QObject *parent) | |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static QString | pierSideStateString (PierSide ps) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Static Public Attributes | |
static const QList< KLocalizedString > | mountStates |
Protected Member Functions | |
bool | sendCoords (SkyPoint *ScopeTarget) |
bool | slewDefined () |
void | updateJ2000Coordinates (SkyPoint *coords) |
void | updateParkStatus () |
void | updateTarget () |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
bool | connected |
QString | name |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
![]() | |
static uint8_t | getID () |
![]() | |
QString | m_DBusObjectPath |
QString | m_Name |
GenericDevice * | m_Parent |
QScopedPointer< QTimer > | m_ReadyTimer |
![]() | |
static uint8_t | m_ID = 1 |
Detailed Description
device handle controlling Mounts.
It can slew and sync to a specific sky point and supports all standard properties with INDI telescope device.
Definition at line 28 of file indimount.h.
Member Enumeration Documentation
◆ HorizontalMotion
enum ISD::Mount::HorizontalMotion |
Definition at line 37 of file indimount.h.
◆ MotionCommand
enum ISD::Mount::MotionCommand |
Definition at line 38 of file indimount.h.
◆ ParkOptionCommand
enum ISD::Mount::ParkOptionCommand |
Definition at line 63 of file indimount.h.
◆ PierSide
enum ISD::Mount::PierSide |
Definition at line 39 of file indimount.h.
◆ Status
enum ISD::Mount::Status |
Definition at line 53 of file indimount.h.
◆ TrackModes
enum ISD::Mount::TrackModes |
Definition at line 64 of file indimount.h.
◆ VerticalMotion
enum ISD::Mount::VerticalMotion |
Definition at line 36 of file indimount.h.
Constructor & Destructor Documentation
◆ Mount()
|
explicit |
Definition at line 35 of file indimount.cpp.
Member Function Documentation
◆ abort
|
slot |
Definition at line 970 of file indimount.cpp.
◆ canAbort()
|
inline |
Definition at line 131 of file indimount.h.
◆ canControlTrack()
|
inline |
Definition at line 102 of file indimount.h.
◆ canCustomPark()
|
inline |
Definition at line 148 of file indimount.h.
◆ canFlip()
|
inline |
Definition at line 88 of file indimount.h.
◆ canGoto()
|
inline |
Definition at line 84 of file indimount.h.
◆ canGuide()
bool ISD::Mount::canGuide | ( | ) |
Definition at line 509 of file indimount.cpp.
◆ canPark()
bool ISD::Mount::canPark | ( | ) |
Definition at line 517 of file indimount.cpp.
◆ canSync()
|
inline |
Definition at line 96 of file indimount.h.
◆ canTrackSatellite()
|
inline |
Definition at line 194 of file indimount.h.
◆ centerLock()
void ISD::Mount::centerLock | ( | ) |
Definition at line 621 of file indimount.cpp.
◆ centerUnlock()
void ISD::Mount::centerUnlock | ( | ) |
Definition at line 635 of file indimount.cpp.
◆ clearAlignmentModel()
bool ISD::Mount::clearAlignmentModel | ( | ) |
Definition at line 1269 of file indimount.cpp.
◆ clearParking()
bool ISD::Mount::clearParking | ( | ) |
Definition at line 1252 of file indimount.cpp.
◆ currentCoordinates()
|
inline |
Definition at line 217 of file indimount.h.
◆ doPulse() [1/2]
bool ISD::Mount::doPulse | ( | GuideDirection | dir, |
int | msecs ) |
Definition at line 555 of file indimount.cpp.
◆ doPulse() [2/2]
bool ISD::Mount::doPulse | ( | GuideDirection | ra_dir, |
int | ra_msecs, | ||
GuideDirection | dec_dir, | ||
int | dec_msecs ) |
Definition at line 544 of file indimount.cpp.
◆ find()
void ISD::Mount::find | ( | ) |
Definition at line 613 of file indimount.cpp.
◆ getCustomTrackRate()
bool ISD::Mount::getCustomTrackRate | ( | double & | raRate, |
double & | deRate ) |
Definition at line 1424 of file indimount.cpp.
◆ getEqCoords()
bool ISD::Mount::getEqCoords | ( | double * | ra, |
double * | dec ) |
Definition at line 1034 of file indimount.cpp.
◆ getManualMotionString()
QString ISD::Mount::getManualMotionString | ( | ) | const |
Definition at line 1326 of file indimount.cpp.
◆ getSlewRate()
int ISD::Mount::getSlewRate | ( | ) | const |
Definition at line 1179 of file indimount.cpp.
◆ getTrackMode()
bool ISD::Mount::getTrackMode | ( | uint8_t & | index | ) |
Definition at line 1393 of file indimount.cpp.
◆ hasAlignmentModel()
|
inline |
Definition at line 171 of file indimount.h.
◆ hasCustomTrackRate()
|
inline |
Definition at line 116 of file indimount.h.
◆ hasSlewRates()
|
inline |
Definition at line 177 of file indimount.h.
◆ hasTrackModes()
|
inline |
Definition at line 109 of file indimount.h.
◆ hourAngle()
const dms ISD::Mount::hourAngle | ( | ) | const |
Hour angle of the current coordinates.
Definition at line 1512 of file indimount.cpp.
◆ isInMotion()
bool ISD::Mount::isInMotion | ( | ) |
Definition at line 539 of file indimount.cpp.
◆ isJ2000()
|
inline |
Definition at line 76 of file indimount.h.
◆ isParked()
|
inline |
Definition at line 144 of file indimount.h.
◆ isReversed()
bool ISD::Mount::isReversed | ( | INDI_EQ_AXIS | axis | ) |
Definition at line 1518 of file indimount.cpp.
◆ isSlewing()
bool ISD::Mount::isSlewing | ( | ) |
Definition at line 529 of file indimount.cpp.
◆ isTracking()
bool ISD::Mount::isTracking | ( | ) |
Definition at line 1371 of file indimount.cpp.
◆ MoveNS()
bool ISD::Mount::MoveNS | ( | VerticalMotion | dir, |
MotionCommand | cmd ) |
Definition at line 1058 of file indimount.cpp.
◆ MoveWE()
bool ISD::Mount::MoveWE | ( | HorizontalMotion | dir, |
MotionCommand | cmd ) |
Definition at line 1121 of file indimount.cpp.
◆ newCoords
|
signal |
Update event with the current telescope position.
- Parameters
-
position mount position. Independent from the mount type, the EQ coordinates(both JNow and J2000) as well as the alt/az values are filled. pierside for GEMs report the pier side the scope is currently (PierSide::PIER_WEST means the mount is on the western side of the pier pointing east of the meridian). ha current hour angle
◆ newStatus
|
signal |
Change in the mount status.
◆ newTarget
|
signal |
The mount has finished the slew to a new target.
- Parameters
-
currentCoords exact position where the mount is positioned
◆ newTargetName
|
signal |
The mount has finished the slew to a new target.
- Parameters
-
Name Name of object, if any, the mount is positioned at.
◆ park
|
slot |
Definition at line 992 of file indimount.cpp.
◆ parkStatus()
|
inline |
Definition at line 155 of file indimount.h.
◆ pierSide()
|
inline |
Definition at line 188 of file indimount.h.
◆ pierSideStateString()
|
inlinestatic |
Definition at line 40 of file indimount.h.
◆ processNumber()
|
overridevirtual |
Reimplemented from ISD::ConcreteDevice.
Definition at line 207 of file indimount.cpp.
◆ processSwitch()
|
overridevirtual |
Reimplemented from ISD::ConcreteDevice.
Definition at line 305 of file indimount.cpp.
◆ processText()
|
overridevirtual |
Reimplemented from ISD::ConcreteDevice.
Definition at line 381 of file indimount.cpp.
◆ registerProperty()
|
overridevirtual |
Reimplemented from ISD::ConcreteDevice.
Definition at line 70 of file indimount.cpp.
◆ sendCoords()
|
protected |
Send the coordinates to the mount's INDI driver.
Due to the INDI implementation, this function is shared for syncing, slewing and other (partly scope specific) functions like the setting parking position. The interpretation of the coordinates depends in the setting of other INDI switches for slewing, synching, tracking etc.
- Parameters
-
ScopeTarget target coordinates
- Returns
- true if sending the coordinates succeeded
Definition at line 641 of file indimount.cpp.
◆ sendParkingOptionCommand()
bool ISD::Mount::sendParkingOptionCommand | ( | ParkOptionCommand | command | ) |
Definition at line 1443 of file indimount.cpp.
◆ setAlignmentModelEnabled()
bool ISD::Mount::setAlignmentModelEnabled | ( | bool | enable | ) |
Definition at line 1196 of file indimount.cpp.
◆ setAltLimits()
void ISD::Mount::setAltLimits | ( | double | minAltitude, |
double | maxAltitude, | ||
bool | trackingOnly ) |
Definition at line 1189 of file indimount.cpp.
◆ setCustomParking()
void ISD::Mount::setCustomParking | ( | SkyPoint * | coords = nullptr | ) |
Definition at line 602 of file indimount.cpp.
◆ setCustomTrackRate
|
slot |
Definition at line 1404 of file indimount.cpp.
◆ setReversedEnabled()
bool ISD::Mount::setReversedEnabled | ( | INDI_EQ_AXIS | axis, |
bool | enabled ) |
Definition at line 1527 of file indimount.cpp.
◆ setSatelliteTLEandTrack()
bool ISD::Mount::setSatelliteTLEandTrack | ( | QString | tle, |
const KStarsDateTime | satPassStart, | ||
const KStarsDateTime | satPassEnd ) |
Tracks satellite on provided TLE, initial epoch for trajectory calculation and window in minutes.
This function needs a Two-Line-Element and a time window in the form of an initial point and a number of minutes on which the trajectory should start. The function was developed wiht the lx200 in mind. If the trajectory has already started, the current time and a window of 1min are sufficient.
- Parameters
-
tle Two-line-element. satPassStart Start time of the trajectory calculation satPassEnd End time of the trajectory calculation
Definition at line 1228 of file indimount.cpp.
◆ setSlewRate
|
slot |
Definition at line 1156 of file indimount.cpp.
◆ setTrackEnabled
|
slot |
Definition at line 1351 of file indimount.cpp.
◆ setTrackMode
|
slot |
Definition at line 1376 of file indimount.cpp.
◆ Slew() [1/2]
bool ISD::Mount::Slew | ( | double | ra, |
double | dec, | ||
bool | flip = false ) |
Definition at line 888 of file indimount.cpp.
◆ Slew() [2/2]
bool ISD::Mount::Slew | ( | SkyPoint * | ScopeTarget, |
bool | flip = false ) |
Definition at line 906 of file indimount.cpp.
◆ slewDefined()
|
protected |
Check whether sending new coordinates will result into a slew.
Definition at line 866 of file indimount.cpp.
◆ slewRates()
|
inline |
Definition at line 181 of file indimount.h.
◆ status() [1/2]
Mount::Status ISD::Mount::status | ( | ) |
Definition at line 1301 of file indimount.cpp.
◆ status() [2/2]
Mount::Status ISD::Mount::status | ( | INumberVectorProperty * | nvp | ) |
Definition at line 1456 of file indimount.cpp.
◆ statusString()
const QString ISD::Mount::statusString | ( | Mount::Status | status, |
bool | translated = true ) const |
Definition at line 1314 of file indimount.cpp.
◆ StopNS()
bool ISD::Mount::StopNS | ( | ) |
Definition at line 1107 of file indimount.cpp.
◆ stopTimers()
void ISD::Mount::stopTimers | ( | ) |
stopTimers Stop timers to prevent timing race condition when device is unavailable and timer is still invoked.
Definition at line 1538 of file indimount.cpp.
◆ StopWE()
bool ISD::Mount::StopWE | ( | ) |
Definition at line 1093 of file indimount.cpp.
◆ Sync() [1/2]
bool ISD::Mount::Sync | ( | double | ra, |
double | dec ) |
Definition at line 936 of file indimount.cpp.
◆ Sync() [2/2]
bool ISD::Mount::Sync | ( | SkyPoint * | ScopeTarget | ) |
Definition at line 946 of file indimount.cpp.
◆ unpark
|
slot |
Definition at line 1013 of file indimount.cpp.
◆ updateJ2000Coordinates()
|
protected |
Helper function to update the J2000 coordinates of a sky point from its JNow coordinates.
- Parameters
-
coords sky point with correct JNow values in RA and DEC
Definition at line 186 of file indimount.cpp.
◆ updateParkStatus()
|
protected |
updateParkStatus Updating parking status by checking the TELESCOPE_PARK property.
Definition at line 439 of file indimount.cpp.
◆ updateTarget()
|
protected |
updateTarget update target position from {
- See also
- currentPosition} and (if not pointing into the empty sky) also the target name.
Definition at line 194 of file indimount.cpp.
Member Data Documentation
◆ mountStates
|
static |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:41 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.