KStarsLite
#include <kstarslite.h>
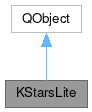
Properties | |
bool | runTutorial |
![]() | |
objectName | |
Signals | |
void | dataLoadFinished () |
void | notificationMessage (QString msg) |
void | runTutorialChanged () |
void | scaleChanged (float) |
void | showSplash () |
Public Slots | |
void | loadColorScheme (const QString &name) |
void | slotSetTime (QDateTime time) |
void | slotStepBackward () |
void | slotStepForward () |
void | slotToggleTimer () |
void | slotTrack () |
void | updateTime (const bool automaticDSTchange=true) |
bool | writeConfig () |
Public Member Functions | |
Q_INVOKABLE void | applyConfig (bool doApplyFocus=true) |
ClientManagerLite * | clientManagerLite () const |
KStarsData * | data () const |
Q_INVOKABLE void | fullUpdate () |
Q_INVOKABLE QColor | getColor (QString name) |
Q_INVOKABLE QString | getConfigCScheme () |
QQuickWindow * | getMainWindow () |
bool | getRunTutorial () |
ImageProvider * | imageProvider () const |
Q_INVOKABLE bool | isToggled (ObjectsToToggle toToggle) |
SkyMapLite * | map () const |
QQmlApplicationEngine * | qmlEngine () |
Q_INVOKABLE void | setProjection (uint proj) |
void | setRunTutorial (bool runTutorial) |
Q_INVOKABLE void | toggleObjects (ObjectsToToggle toToggle, bool toggle) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static KStarsLite * | createInstance (bool doSplash, bool clockrunning=true, const QString &startDateString=QString()) |
static KStarsLite * | Instance () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
This class loads QML files and connects SkyMapLite and KStarsData Unlike KStars class it is not a main window (see KStarsLite::m_Engine) but a root object that contains the program clock and holds pointers to SkyMapLite and KStarsData objects.
KStarsLite is a singleton, use KStarsLite::createInstance() to create an instance and KStarsLite::Instance() to get a pointer to the instance
- Version
- 1.0
Definition at line 46 of file kstarslite.h.
Property Documentation
◆ runTutorial
|
readwrite |
Definition at line 50 of file kstarslite.h.
Member Function Documentation
◆ applyConfig()
void KStarsLite::applyConfig | ( | bool | doApplyFocus = true | ) |
currently sets color scheme from config
Definition at line 390 of file kstarslite.cpp.
◆ clientManagerLite()
|
inline |
- Returns
- pointer to KStarsData object which handles connection to INDI server.
Definition at line 107 of file kstarslite.h.
◆ createInstance()
|
static |
Create an instance of this class.
Destroy any previous instance
- Parameters
-
doSplash clockrunning startDateString
- Note
- See KStarsLite::KStarsLite for details on parameters
- Returns
- a pointer to the instance
Definition at line 217 of file kstarslite.cpp.
◆ data()
|
inline |
- Returns
- pointer to KStarsData object which contains application data.
Definition at line 86 of file kstarslite.h.
◆ dataLoadFinished
|
signal |
Sent when KStarsData finishes loading data.
◆ fullUpdate()
void KStarsLite::fullUpdate | ( | ) |
used from QML to update positions of sky objects and update SkyMapLite
Definition at line 226 of file kstarslite.cpp.
◆ getMainWindow()
QQuickWindow * KStarsLite::getMainWindow | ( | ) |
- Returns
- pointer to the main window.
Definition at line 177 of file kstarslite.cpp.
◆ getRunTutorial()
bool KStarsLite::getRunTutorial | ( | ) |
- Returns
- true if tutorial should be shown
Definition at line 517 of file kstarslite.cpp.
◆ imageProvider()
|
inline |
- Returns
- pointer to ImageProvider that is used in QML to display image fetched from CCD
Definition at line 89 of file kstarslite.h.
◆ Instance()
|
inlinestatic |
- Returns
- a pointer to the instance of this class
Definition at line 77 of file kstarslite.h.
◆ loadColorScheme
|
slot |
Load a color scheme.
- Parameters
-
name the name of the color scheme to load (e.g., "Moonless Night")
Definition at line 297 of file kstarslite.cpp.
◆ map()
|
inline |
- Returns
- pointer to SkyMapLite object which draws SkyMap.
Definition at line 80 of file kstarslite.h.
◆ notificationMessage
|
signal |
Once this signal is emitted, notification with text msg will appear on the screen.
Use this signal to output messages to user (warnings, info etc.)
◆ qmlEngine()
|
inline |
- Returns
- pointer to QQmlApplicationEngine that runs QML
Definition at line 92 of file kstarslite.h.
◆ scaleChanged
|
signal |
Emitted whenever TimeSpinBox in QML changes the scale.
◆ setRunTutorial()
void KStarsLite::setRunTutorial | ( | bool | runTutorial | ) |
set whether tutorial should be shown on next startup
Definition at line 508 of file kstarslite.cpp.
◆ showSplash
|
signal |
Makes splash (Splash.qml) visible on startup.
◆ slotSetTime
|
slot |
sets time and date according to parameter time
Definition at line 326 of file kstarslite.cpp.
◆ slotStepBackward
|
slot |
action slot: advance one step backward in time
Definition at line 380 of file kstarslite.cpp.
◆ slotStepForward
|
slot |
action slot: advance one step forward in time
Definition at line 372 of file kstarslite.cpp.
◆ slotToggleTimer
|
slot |
action slot: toggle whether kstars clock is running or not
Definition at line 352 of file kstarslite.cpp.
◆ slotTrack
|
slot |
start tracking clickedPoint or stop tracking if we are already tracking some object
Definition at line 185 of file kstarslite.cpp.
◆ updateTime
|
slot |
Update time-dependent data and (possibly) repaint the sky map.
- Parameters
-
automaticDSTchange change DST status automatically?
Definition at line 234 of file kstarslite.cpp.
◆ writeConfig
|
slot |
Write current settings to config file.
Used to save config file upon exit
Definition at line 264 of file kstarslite.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Jul 26 2024 11:59:53 by doxygen 1.11.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.