SkyMapLite
#include <skymaplite.h>
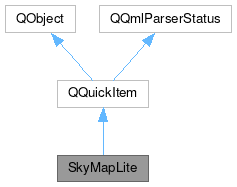
Properties | |
bool | automaticMode |
bool | centerLocked |
SkyObjectLite * | clickedObjectLite |
SkyPointLite * | clickedPointLite |
QStringList | FOVSymbols |
double | magLim |
double | skyRotation |
bool | slewing |
![]() | |
activeFocus | |
activeFocusOnTab | |
antialiasing | |
baselineOffset | |
childrenRect | |
clip | |
containmentMask | |
enabled | |
focus | |
height | |
implicitHeight | |
implicitWidth | |
opacity | |
parent | |
rotation | |
scale | |
smooth | |
state | |
transformOrigin | |
visible | |
width | |
x | |
y | |
z | |
![]() | |
objectName | |
Signals | |
void | automaticModeChanged (bool) |
void | centerLockedChanged (bool) |
void | destinationChanged () |
void | magLimChanged (double magLim) |
void | mousePointChanged (SkyPoint *) |
void | objectChanged () |
void | objectLiteChanged () |
void | pointLiteChanged () |
void | posClicked (QPointF pos) |
void | positionChanged () |
void | positionClicked (SkyPoint *) |
void | skyRotationChanged (double skyRotation) |
void | slewingChanged (bool) |
void | symbolsFOVChanged (QStringList) |
void | zoomChanged () |
Public Slots | |
void | forceUpdate () |
void | forceUpdateNow () |
void | resizeItem () |
void | setSkyRotation (double skyRotation) |
void | slewFocus () |
void | slotCenter () |
void | slotClockSlewing () |
void | slotSelectObject (SkyObject *skyObj) |
void | slotUpdateSky (bool now) |
void | slotZoomDefault () |
void | slotZoomIn () |
void | slotZoomOut () |
void | updateAutomaticMode () |
Public Member Functions | |
~SkyMapLite () | |
Q_INVOKABLE void | addFOVSymbol (const QString &FOVName, bool initialState=false) |
SkyObject * | clickedObject () const |
SkyPoint * | clickedPoint () |
void | deleteSkyNode (SkyNode *skyNode) |
SkyPoint * | destination () |
SkyPoint * | focus () |
SkyObject * | focusObject () const |
SkyPoint * | focusPoint () |
bool | getAutomaticMode () const |
QSGTexture * | getCachedTexture (int size, char spType) |
bool | getCenterLocked () |
SkyObjectLite * | getClickedObjectLite () |
SkyPointLite * | getClickedPointLite () |
Q_INVOKABLE QStringList | getFOVSymbols () |
QVector< QVector< QPixmap * > > | getImageCache () |
double | getMagLim () |
double | getSkyRotation () const |
bool | getSlewing () const |
int | harvardToIndex (char c) |
void | initialize (QQuickItem *parent) |
void | initStarImages () |
bool | isFOVVisible (int index) |
bool | isSlewing () const |
void | loadingFinished () |
const Projector * | projector () const |
Q_INVOKABLE uint | projType () const |
Q_INVOKABLE void | setAutomaticMode (bool automaticMode) |
void | setCenterLocked (bool centerLocked) |
void | setClickedObject (SkyObject *o) |
void | setClickedPoint (SkyPoint *f) |
void | setDestination (const dms &ra, const dms &dec) |
void | setDestination (const SkyPoint &f) |
void | setDestinationAltAz (const dms &alt, const dms &az, bool altIsRefracted) |
void | setFocus (const dms &ra, const dms &dec) |
void | setFocus (SkyPoint *f) |
void | setFocusAltAz (const dms &alt, const dms &az) |
void | setFocusObject (SkyObject *o) |
void | setFocusPoint (SkyPoint *f) |
Q_INVOKABLE void | setFOVVisible (int index, bool visible) |
void | setMagLim (double magLim) |
void | setSizeMagLim (float sizeMagLim) |
void | setSlewing (bool newSlewing) |
void | setupProjector () |
void | setZoomFactor (double factor) |
float | sizeMagLim () const |
void | stopTracking () |
QSGTexture * | textToTexture (QString text, QColor color=QColor(255, 255, 255), bool zoomFont=false) |
void | updateFocus () |
![]() | |
QQuickItem (QQuickItem *parent) | |
Qt::MouseButtons | acceptedMouseButtons () const const |
bool | acceptHoverEvents () const const |
bool | acceptTouchEvents () const const |
void | activeFocusChanged (bool) |
bool | activeFocusOnTab () const const |
void | activeFocusOnTabChanged (bool) |
bool | antialiasing () const const |
void | antialiasingChanged (bool) |
qreal | baselineOffset () const const |
void | baselineOffsetChanged (qreal) |
QBindable< qreal > | bindableHeight () |
QBindable< qreal > | bindableWidth () |
QBindable< qreal > | bindableX () |
QBindable< qreal > | bindableY () |
virtual QRectF | boundingRect () const const |
QQuickItem * | childAt (qreal x, qreal y) const const |
QList< QQuickItem * > | childItems () const const |
QRectF | childrenRect () |
void | childrenRectChanged (const QRectF &) |
bool | clip () const const |
void | clipChanged (bool) |
virtual QRectF | clipRect () const const |
QObject * | containmentMask () const const |
void | containmentMaskChanged () |
virtual bool | contains (const QPointF &point) const const |
QCursor | cursor () const const |
void | dumpItemTree () const const |
void | enabledChanged () |
void | ensurePolished () |
bool | filtersChildMouseEvents () const const |
Flags | flags () const const |
void | focusChanged (bool) |
void | forceActiveFocus () |
void | forceActiveFocus (Qt::FocusReason reason) |
void | grabMouse () |
QSharedPointer< QQuickItemGrabResult > | grabToImage (const QSize &targetSize) |
void | grabTouchPoints (const QList< int > &ids) |
bool | hasActiveFocus () const const |
bool | hasFocus () const const |
qreal | height () const const |
void | heightChanged () |
qreal | implicitHeight () const const |
void | implicitHeightChanged () |
qreal | implicitWidth () const const |
void | implicitWidthChanged () |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const const |
bool | isAncestorOf (const QQuickItem *child) const const |
bool | isEnabled () const const |
bool | isFocusScope () const const |
virtual bool | isTextureProvider () const const |
bool | isVisible () const const |
bool | keepMouseGrab () const const |
bool | keepTouchGrab () const const |
QPointF | mapFromGlobal (const QPointF &point) const const |
QPointF | mapFromItem (const QQuickItem *item, const QPointF &point) const const |
QPointF | mapFromScene (const QPointF &point) const const |
QRectF | mapRectFromItem (const QQuickItem *item, const QRectF &rect) const const |
QRectF | mapRectFromScene (const QRectF &rect) const const |
QRectF | mapRectToItem (const QQuickItem *item, const QRectF &rect) const const |
QRectF | mapRectToScene (const QRectF &rect) const const |
QPointF | mapToGlobal (const QPointF &point) const const |
QPointF | mapToItem (const QQuickItem *item, const QPointF &point) const const |
QPointF | mapToScene (const QPointF &point) const const |
QQuickItem * | nextItemInFocusChain (bool forward) |
qreal | opacity () const const |
void | opacityChanged () |
void | parentChanged (QQuickItem *) |
QQuickItem * | parentItem () const const |
void | polish () |
void | resetAntialiasing () |
void | resetHeight () |
void | resetWidth () |
qreal | rotation () const const |
void | rotationChanged () |
qreal | scale () const const |
void | scaleChanged () |
QQuickItem * | scopedFocusItem () const const |
void | setAcceptedMouseButtons (Qt::MouseButtons buttons) |
void | setAcceptHoverEvents (bool enabled) |
void | setAcceptTouchEvents (bool enabled) |
void | setActiveFocusOnTab (bool) |
void | setAntialiasing (bool) |
void | setBaselineOffset (qreal) |
void | setClip (bool) |
void | setContainmentMask (QObject *mask) |
void | setCursor (const QCursor &cursor) |
void | setEnabled (bool) |
void | setFiltersChildMouseEvents (bool filter) |
void | setFlag (Flag flag, bool enabled) |
void | setFlags (Flags flags) |
void | setFocus (bool focus, Qt::FocusReason reason) |
void | setFocus (bool) |
void | setHeight (qreal) |
void | setImplicitHeight (qreal) |
void | setImplicitWidth (qreal) |
void | setKeepMouseGrab (bool keep) |
void | setKeepTouchGrab (bool keep) |
void | setOpacity (qreal) |
void | setParentItem (QQuickItem *parent) |
void | setRotation (qreal) |
void | setScale (qreal) |
void | setSize (const QSizeF &size) |
void | setSmooth (bool) |
void | setState (const QString &) |
void | setTransformOrigin (TransformOrigin) |
void | setVisible (bool) |
void | setWidth (qreal) |
void | setX (qreal) |
void | setY (qreal) |
void | setZ (qreal) |
QSizeF | size () const const |
bool | smooth () const const |
void | smoothChanged (bool) |
void | stackAfter (const QQuickItem *sibling) |
void | stackBefore (const QQuickItem *sibling) |
QString | state () const const |
void | stateChanged (const QString &) |
virtual QSGTextureProvider * | textureProvider () const const |
TransformOrigin | transformOrigin () const const |
void | transformOriginChanged (TransformOrigin) |
void | ungrabMouse () |
void | ungrabTouchPoints () |
void | unsetCursor () |
void | update () |
QQuickItem * | viewportItem () const const |
void | visibleChanged () |
qreal | width () const const |
void | widthChanged () |
QQuickWindow * | window () const const |
void | windowChanged (QQuickWindow *window) |
qreal | x () const const |
void | xChanged () |
qreal | y () const const |
void | yChanged () |
qreal | z () const const |
void | zChanged () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static SkyMapLite * | createInstance () |
static double | deleteLimit () |
static SkyMapLite * | Instance () |
static bool | IsSlewing () |
static RootNode * | rootNode () |
static void | setRootNode (RootNode *root) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
virtual void | mouseDoubleClickEvent (QMouseEvent *e) override |
virtual void | mouseMoveEvent (QMouseEvent *e) override |
virtual void | mousePressEvent (QMouseEvent *e) override |
virtual void | mouseReleaseEvent (QMouseEvent *e) override |
virtual void | touchEvent (QTouchEvent *e) override |
virtual QSGNode * | updatePaintNode (QSGNode *oldNode, UpdatePaintNodeData *updatePaintNodeData) override |
virtual void | wheelEvent (QWheelEvent *e) override |
![]() | |
virtual bool | childMouseEventFilter (QQuickItem *item, QEvent *event) |
virtual void | classBegin () override |
virtual void | componentComplete () override |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual bool | event (QEvent *ev) override |
virtual void | focusInEvent (QFocusEvent *) |
virtual void | focusOutEvent (QFocusEvent *) |
virtual void | geometryChange (const QRectF &newGeometry, const QRectF &oldGeometry) |
bool | heightValid () const const |
virtual void | hoverEnterEvent (QHoverEvent *event) |
virtual void | hoverLeaveEvent (QHoverEvent *event) |
virtual void | hoverMoveEvent (QHoverEvent *event) |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
bool | isComponentComplete () const const |
virtual void | itemChange (ItemChange change, const ItemChangeData &value) |
virtual void | keyPressEvent (QKeyEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | mouseUngrabEvent () |
virtual void | releaseResources () |
virtual void | touchUngrabEvent () |
void | updateInputMethod (Qt::InputMethodQueries queries) |
virtual void | updatePolish () |
bool | widthValid () const const |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
enum | Flag |
enum | ItemChange |
enum | TransformOrigin |
![]() | |
Bottom | |
BottomLeft | |
BottomRight | |
Center | |
typedef | Flags |
ItemAcceptsDrops | |
ItemAcceptsInputMethod | |
ItemActiveFocusHasChanged | |
ItemAntialiasingHasChanged | |
ItemChildAddedChange | |
ItemChildRemovedChange | |
ItemClipsChildrenToShape | |
ItemDevicePixelRatioHasChanged | |
ItemEnabledHasChanged | |
ItemHasContents | |
ItemIsFocusScope | |
ItemIsViewport | |
ItemObservesViewport | |
ItemOpacityHasChanged | |
ItemParentHasChanged | |
ItemRotationHasChanged | |
ItemSceneChange | |
ItemVisibleHasChanged | |
Left | |
Right | |
Top | |
TopLeft | |
TopRight | |
![]() | |
typedef | QObjectList |
Detailed Description
This is the main item that displays all SkyItems.
After its instantiation it is reparanted to an object with objectName SkyMapLiteWrapper in main.qml. To display SkyItems they are reparanted to instance of SkyMapLite.
SkyMapLite handles most user interaction events (both mouse and keyboard).
Item for displaying sky objects; also handles user interaction events.
- Version
- 1.0
Definition at line 58 of file skymaplite.h.
Property Documentation
◆ automaticMode
|
readwrite |
Definition at line 75 of file skymaplite.h.
◆ centerLocked
|
readwrite |
true if SkyMapLite is centered on an object and only pinch-to-zoom needs to be available
Definition at line 74 of file skymaplite.h.
◆ clickedObjectLite
|
read |
Definition at line 66 of file skymaplite.h.
◆ clickedPointLite
|
read |
wrappers for clickedPoint and clickedObject.
Used to set clicked object and point from QML
Definition at line 65 of file skymaplite.h.
◆ FOVSymbols
|
read |
list of FOVSymbols that are currently available
Definition at line 68 of file skymaplite.h.
◆ magLim
|
readwrite |
◆ skyRotation
|
readwrite |
Definition at line 76 of file skymaplite.h.
◆ slewing
|
readwrite |
true if SkyMapLite is being panned
Definition at line 70 of file skymaplite.h.
Constructor & Destructor Documentation
◆ SkyMapLite()
|
protected |
Definition at line 91 of file skymaplite.cpp.
◆ ~SkyMapLite()
SkyMapLite::~SkyMapLite | ( | ) |
Member Function Documentation
◆ addFOVSymbol()
void SkyMapLite::addFOVSymbol | ( | const QString & | FOVName, |
bool | initialState = false ) |
adds FOV symbol to m_FOVSymbols
- Parameters
-
FOVName name of a FOV symbol initialState defines whether the state is initial
Definition at line 919 of file skymaplite.cpp.
◆ clickedObject()
|
inline |
Retrieve the object nearest to a mouse click event.
If the user clicks on the sky map, a pointer to the nearest SkyObject is stored in the private member ClickedObject. This function returns the ClickedObject pointer, or nullptr if there is no CLickedObject.
- Returns
- a pointer to the object nearest to a user mouse click.
Definition at line 234 of file skymaplite.h.
◆ clickedPoint()
|
inline |
Retrieve the ClickedPoint position.
When the user clicks on a point in the sky map, the sky coordinates of the mouse cursor are stored in the private member ClickedPoint. This function retrieves a pointer to ClickedPoint.
- Returns
- a pointer to ClickedPoint, the sky coordinates where the user clicked.
Definition at line 217 of file skymaplite.h.
◆ createInstance()
|
static |
Creates instance of SkyMapLite (delete the old one if any)
Definition at line 215 of file skymaplite.cpp.
◆ deleteLimit()
|
static |
return limit of hides for the node to delete it
Definition at line 199 of file skymaplite.cpp.
◆ deleteSkyNode()
void SkyMapLite::deleteSkyNode | ( | SkyNode * | skyNode | ) |
skyNode will be deleted on the next call to updatePaintNode (currently used only in StarNode(struct in StarBlock))
Definition at line 205 of file skymaplite.cpp.
◆ destination()
|
inline |
retrieve the Destination position.
The Destination is the point on the sky to which the focus will be moved.
- Returns
- a pointer to the destination point of the sky map
Definition at line 135 of file skymaplite.h.
◆ destinationChanged
|
signal |
Emitted by setDestination(), and connected to slewFocus().
Whenever the Destination point is changed, slewFocus() will iteratively step the Focus toward Destination until it is reached.
◆ focus()
|
inline |
Retrieve the Focus point; the position on the sky at the center of the skymap.
- Returns
- a pointer to the central focus point of the sky map
Definition at line 125 of file skymaplite.h.
◆ focusObject()
|
inline |
Retrieve the object which is centered in the sky map.
If the user centers the sky map on an object (by double-clicking or using the Find Object dialog), a pointer to the "focused" object is stored in the private member FocusObject. This function returns a pointer to the FocusObject, or nullptr if there is not FocusObject.
- Returns
- a pointer to the object at the center of the sky map.
Definition at line 252 of file skymaplite.h.
◆ focusPoint()
|
inline |
retrieve the FocusPoint position.
The FocusPoint stores the position on the sky that is to be focused next. This is not exactly the same as the Destination point, because when the Destination is set, it will begin slewing immediately.
- Returns
- a pointer to the sky point which is to be focused next.
Definition at line 149 of file skymaplite.h.
◆ forceUpdate
|
slot |
Recalculates the positions of objects in the sky, and then repaints the sky map.
Definition at line 639 of file skymaplite.cpp.
◆ forceUpdateNow
|
inlineslot |
◆ getAutomaticMode()
|
inline |
True if automatic mode is on (SkyMapLite is controlled by smartphones accelerometer magnetometer)
Definition at line 438 of file skymaplite.h.
◆ getCachedTexture()
QSGTexture * SkyMapLite::getCachedTexture | ( | int | size, |
char | spType ) |
returns cached texture from textureCache.
Use outside of scene graph rendering thread (e.g. not during call to updatePaintNode) is prohibited!
- Parameters
-
size size of the star spType spectral class
- Returns
- cached QSGTexture from textureCache
Definition at line 210 of file skymaplite.cpp.
◆ getCenterLocked()
|
inline |
getter for centerLocked
Definition at line 409 of file skymaplite.h.
◆ getClickedObjectLite()
|
inline |
getter for clickedObjectLite
Definition at line 401 of file skymaplite.h.
◆ getClickedPointLite()
|
inline |
getter for clickedPointLite
Definition at line 393 of file skymaplite.h.
◆ getFOVSymbols()
|
inline |
this QList should be used as a model in QML to switch on/off FOV symbols
Definition at line 382 of file skymaplite.h.
◆ getImageCache()
returns cache of star images
- Returns
- star images cache
Definition at line 844 of file skymaplite.cpp.
◆ getMagLim()
|
inline |
- Returns
- current magnitude limit
Definition at line 310 of file skymaplite.h.
◆ getSkyRotation()
|
inline |
Definition at line 448 of file skymaplite.h.
◆ getSlewing()
|
inline |
Proxy method for SkyMapDrawAbstract::drawObjectLabels()
- Returns
- true if SkyMapLite is being slewed
Definition at line 422 of file skymaplite.h.
◆ harvardToIndex()
int SkyMapLite::harvardToIndex | ( | char | c | ) |
Returns index for a Harvard spectral classification.
Definition at line 810 of file skymaplite.cpp.
◆ initialize()
void SkyMapLite::initialize | ( | QQuickItem * | parent | ) |
Bind size to parent's size and initialize star images.
Definition at line 222 of file skymaplite.cpp.
◆ initStarImages()
void SkyMapLite::initStarImages | ( | ) |
Initializes images of Stars and puts them in cache (copied from SkyQPainter)
Definition at line 996 of file skymaplite.cpp.
◆ Instance()
|
inlinestatic |
Definition at line 99 of file skymaplite.h.
◆ isFOVVisible()
bool SkyMapLite::isFOVVisible | ( | int | index | ) |
- Parameters
-
index of FOVSymbol in m_FOVSymbols
- Returns
- true if FOV symbol with name FOVName should be drawn.
Definition at line 928 of file skymaplite.cpp.
◆ IsSlewing()
|
inlinestatic |
Definition at line 104 of file skymaplite.h.
◆ isSlewing()
bool SkyMapLite::isSlewing | ( | ) | const |
- Returns
- true if the map is in slewing mode or clock is active
Definition at line 805 of file skymaplite.cpp.
◆ loadingFinished()
|
inline |
called when SkyMapComposite finished loading all SkyComponents
Definition at line 301 of file skymaplite.h.
◆ magLimChanged
|
signal |
Emitted when magnitude limit is changed.
◆ mouseDoubleClickEvent()
|
overrideprotectedvirtual |
Center SkyMap at double-clicked location
Reimplemented from QQuickItem.
Definition at line 138 of file skymapliteevents.cpp.
◆ mouseMoveEvent()
|
overrideprotectedvirtual |
This function does several different things depending on the state of the program:
- If Angle-measurement mode is active, update the end-ruler point to the mouse cursor, and continue this function.
- If we are defining a ZoomBox, update the ZoomBox rectangle, redraw the screen, and return.
- If dragging the mouse in the map, update focus such that RA, Dec under the mouse cursor remains constant.
- If just moving the mouse, simply update the curso coordinates in the status bar.
Reimplemented from QQuickItem.
Definition at line 148 of file skymapliteevents.cpp.
◆ mousePointChanged
|
signal |
Emitted when position under mouse changed.
◆ mousePressEvent()
|
overrideprotectedvirtual |
Process keystrokes:
- arrow keys Slew the map
- +/- keys Zoom in and out
- Space Toggle between Horizontal and Equatorial coordinate systems
- 0-9 Go to a major Solar System body (0=Sun; 1-9 are the major planets, except 3=Moon)
- [ Place starting point for measuring an angular distance
- ] End point for Angular Distance; display measurement.
- Escape Cancel Angular measurement
- ,/< Step backward one time step
- ./> Step forward one time step When keyRelease is triggered, just set the "slewing" flag to false, and update the display (to draw objects that are hidden when slewing==true). Determine RA, Dec coordinates of clicked location. Find the SkyObject which is nearest to the clicked location.
If left-clicked: Set set mouseButtonDown==true, slewing==true; display nearest object name in status bar. If right-clicked: display popup menu appropriate for nearest object.
Reimplemented from QQuickItem.
Definition at line 21 of file skymapliteevents.cpp.
◆ mouseReleaseEvent()
|
overrideprotectedvirtual |
set mouseButtonDown==false, slewing==false
Reimplemented from QQuickItem.
Definition at line 100 of file skymapliteevents.cpp.
◆ objectChanged
|
signal |
Emitted when current object changed.
◆ objectLiteChanged
|
signal |
Wrapper of ClickedObject for QML.
◆ pointLiteChanged
|
signal |
Wrapper of ClickedPoint for QML.
◆ posClicked
|
signal |
Emitted when user clicks on SkyMapLite (analogous to positionClicked but sends QPoint)
◆ positionChanged
|
signal |
Emitted when pointing changed.
(At least should)
◆ positionClicked
|
signal |
Emitted when a position is clicked.
◆ projector()
|
inline |
Get the current projector.
- Returns
- a pointer to the current projector.
Definition at line 323 of file skymaplite.h.
◆ projType()
uint SkyMapLite::projType | ( | ) | const |
used in QML
- Returns
- type of current projection system
Definition at line 524 of file skymapliteevents.cpp.
◆ resizeItem
|
slot |
Called whenever wrappers' width or height are changed.
Probably will be used to update positions of items.
Definition at line 565 of file skymaplite.cpp.
◆ rootNode()
|
inlinestatic |
Definition at line 346 of file skymaplite.h.
◆ setAutomaticMode()
void SkyMapLite::setAutomaticMode | ( | bool | automaticMode | ) |
switch automatic mode on/off according to isOn parameter
Definition at line 957 of file skymaplite.cpp.
◆ setCenterLocked()
void SkyMapLite::setCenterLocked | ( | bool | centerLocked | ) |
sets whether SkyMapLite is centered on an object and locked(olny pinch-to-zoom is available)
Definition at line 951 of file skymaplite.cpp.
◆ setClickedObject()
void SkyMapLite::setClickedObject | ( | SkyObject * | o | ) |
Set the ClickedObject pointer to the argument.
- Parameters
-
o pointer to the SkyObject to be assigned as the ClickedObject
Definition at line 312 of file skymaplite.cpp.
◆ setClickedPoint()
void SkyMapLite::setClickedPoint | ( | SkyPoint * | f | ) |
Set the ClickedPoint to the skypoint given as an argument.
- Parameters
-
f pointer to the new ClickedPoint.
Definition at line 306 of file skymaplite.cpp.
◆ setDestination() [1/2]
sets the destination point of the skymap, using ra/dec coordinates.
- Note
- This function behaves essentially like the above function. It differs only in the data types of its arguments.
- Parameters
-
ra the new right ascension dec the new declination
Definition at line 283 of file skymaplite.cpp.
◆ setDestination() [2/2]
void SkyMapLite::setDestination | ( | const SkyPoint & | f | ) |
sets the destination point of the sky map.
- Note
- setDestination() emits the destinationChanged() SIGNAL, which triggers the SLOT function SkyMap::slewFocus(). This function iteratively steps the Focus point toward Destination, repainting the sky at each step (if Options::useAnimatedSlewing()==true).
- Parameters
-
f a pointer to the SkyPoint the map should slew to
Definition at line 278 of file skymaplite.cpp.
◆ setDestinationAltAz()
sets the destination point of the sky map, using its alt/az coordinates.
- Parameters
-
alt the new altitude az the new azimuth altIsRefracted set to true if the altitude supplied is apparent
Definition at line 290 of file skymaplite.cpp.
◆ setFocus() [1/2]
sets the focus point of the skymap, using ra/dec coordinates
- Note
- This function behaves essentially like the above function. It differs only in the data types of its arguments.
- Parameters
-
ra the new right ascension dec the new declination
Definition at line 257 of file skymaplite.cpp.
◆ setFocus() [2/2]
void SkyMapLite::setFocus | ( | SkyPoint * | f | ) |
sets the central focus point of the sky map.
- Parameters
-
f a pointer to the SkyPoint the map should be centered on
Definition at line 252 of file skymaplite.cpp.
◆ setFocusAltAz()
sets the focus point of the sky map, using its alt/az coordinates
- Parameters
-
alt the new altitude (actual, i.e. without refraction correction) az the new azimuth
Definition at line 266 of file skymaplite.cpp.
◆ setFocusObject()
void SkyMapLite::setFocusObject | ( | SkyObject * | o | ) |
Set the FocusObject pointer to the argument.
- Parameters
-
o pointer to the SkyObject to be assigned as the FocusObject
Definition at line 318 of file skymaplite.cpp.
◆ setFocusPoint()
|
inline |
set the FocusPoint; the position that is to be the next Destination.
- Parameters
-
f a pointer to the FocusPoint SkyPoint.
Definition at line 204 of file skymaplite.h.
◆ setFOVVisible()
void SkyMapLite::setFOVVisible | ( | int | index, |
bool | visible ) |
updates visibility of FOV symbol according to visible
- Parameters
-
index of FOVSymbol in m_FOVSymbols visible defines whether the FOV symbol should be visible
Definition at line 933 of file skymaplite.cpp.
◆ setMagLim()
void SkyMapLite::setMagLim | ( | double | magLim | ) |
set magnitude limit
Definition at line 499 of file skymapliteevents.cpp.
◆ setRootNode()
|
inlinestatic |
Definition at line 351 of file skymaplite.h.
◆ setSizeMagLim()
|
inline |
Set magnitude limit for size of stars.
Used in StarItem
Definition at line 335 of file skymaplite.h.
◆ setSkyRotation
|
slot |
Definition at line 605 of file skymaplite.cpp.
◆ setSlewing()
void SkyMapLite::setSlewing | ( | bool | newSlewing | ) |
sets whether SkyMapLite is being slewed
Definition at line 942 of file skymaplite.cpp.
◆ setupProjector()
void SkyMapLite::setupProjector | ( | ) |
Call to set up the projector before update of SkyItems positions begins.
Definition at line 735 of file skymaplite.cpp.
◆ setZoomFactor()
void SkyMapLite::setZoomFactor | ( | double | factor | ) |
◆ sizeMagLim()
|
inline |
Used in PointSourceNode.
Definition at line 341 of file skymaplite.h.
◆ skyRotationChanged
|
signal |
Emitted when skyRotation used to rotate coordinates of SkyPoints is changed.
◆ slewFocus
|
slot |
Step the Focus point toward the Destination point.
Do this iteratively, redrawing the Sky Map after each step, until the Focus point is within 1 step of the Destination point. For the final step, snap directly to Destination, and redraw the map.
Definition at line 401 of file skymaplite.cpp.
◆ slewingChanged
|
signal |
Emitted when SkyMapLite is being slewed or slewing is finished.
◆ slotCenter
|
slot |
Center the display at the point ClickedPoint.
The essential part of the function is to simply set the Destination point, which will emit the destinationChanged() SIGNAL, which triggers the slewFocus() SLOT. Additionally, this function performs some bookkeeping tasks, such updating whether we are tracking the new object/position, adding a Planet Trail if required, etc.
- See also
- destinationChanged()
- slewFocus()
Definition at line 327 of file skymaplite.cpp.
◆ slotClockSlewing
|
slot |
Checks whether the timestep exceeds a threshold value.
If so, sets ClockSlewing=true and sets the SimClock to ManualMode.
Definition at line 516 of file skymaplite.cpp.
◆ slotSelectObject
|
slot |
centres skyObj in SkyMap and opens context drawer with skyObj Used in FindDialogLite
Definition at line 590 of file skymaplite.cpp.
◆ slotUpdateSky
|
slot |
Update the focus point and call forceUpdate()
- Parameters
-
now is saved for compatibility reasons
Definition at line 683 of file skymaplite.cpp.
◆ slotZoomDefault
|
slot |
Set default zoom.
Definition at line 585 of file skymaplite.cpp.
◆ slotZoomIn
|
slot |
Zoom in one step.
Definition at line 575 of file skymaplite.cpp.
◆ slotZoomOut
|
slot |
Zoom out one step.
Definition at line 580 of file skymaplite.cpp.
◆ stopTracking()
void SkyMapLite::stopTracking | ( | ) |
Convenience function for shutting off tracking mode.
Just calls KStars::slotTrack()
Definition at line 516 of file skymapliteevents.cpp.
◆ symbolsFOVChanged
|
signal |
Emitted when FOVSymbols list was changed (new value appended)
◆ textToTexture()
QSGTexture * SkyMapLite::textToTexture | ( | QString | text, |
QColor | color = QColor(255, 255, 255), | ||
bool | zoomFont = false ) |
creates QImage from text and converts it to QSGTexture
- Parameters
-
text the text string color text color zoomFont if true zoom-dependent font from SkyLabeler will be used else standart font is used
- Returns
- QSGTexture with text
- Note
- font size is set in SkyLabeler::SkyLabeler() by initializing m_stdFont with default font
Definition at line 849 of file skymaplite.cpp.
◆ touchEvent()
|
overrideprotectedvirtual |
this function handles zooming in and out using "pinch to zoom" gesture
Reimplemented from QQuickItem.
Definition at line 268 of file skymapliteevents.cpp.
◆ updateAutomaticMode
|
slot |
updates focus of SkyMapLite according to data from DeviceOrientation (Smartphone's sensors)
Definition at line 979 of file skymaplite.cpp.
◆ updateFocus()
void SkyMapLite::updateFocus | ( | ) |
Update the focus position according to current options.
Definition at line 690 of file skymaplite.cpp.
◆ updatePaintNode()
|
overrideprotectedvirtual |
Updates SkyMapLite by calling RootNode::update(), which in turn initiates update of all child nodes.
Add or delete telescope crosshairs
Reimplemented from QQuickItem.
Definition at line 143 of file skymaplite.cpp.
◆ wheelEvent()
|
overrideprotectedvirtual |
Zoom in and out with the mouse wheel.
Reimplemented from QQuickItem.
Definition at line 254 of file skymapliteevents.cpp.
◆ zoomChanged
|
signal |
Emitted when zoom level is changed.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Jul 26 2024 11:59:54 by doxygen 1.11.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.