RootNode
#include <rootnode.h>
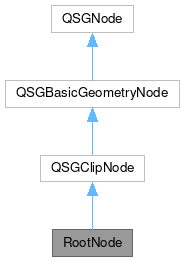
Public Member Functions | |
CometsItem * | cometsItem () |
void | genCachedTextures () |
QSGTexture * | getCachedTexture (int size, char spType) |
LabelsItem * | labelsItem () |
StarItem * | starItem () |
TelescopeSymbolsItem * | telescopeSymbolsItem () |
void | testLeakAdd () |
void | testLeakDelete () |
void | update (bool clearTextures=false) |
void | updateClipPoly () |
![]() | |
QRectF | clipRect () const const |
bool | isRectangular () const const |
void | setClipRect (const QRectF &rect) |
void | setIsRectangular (bool rectHint) |
![]() | |
QSGGeometry * | geometry () |
const QSGGeometry * | geometry () const const |
void | setGeometry (QSGGeometry *geometry) |
![]() | |
void | appendChildNode (QSGNode *node) |
QSGNode * | childAtIndex (int i) const const |
int | childCount () const const |
QSGNode * | firstChild () const const |
Flags | flags () const const |
void | insertChildNodeAfter (QSGNode *node, QSGNode *after) |
void | insertChildNodeBefore (QSGNode *node, QSGNode *before) |
virtual bool | isSubtreeBlocked () const const |
QSGNode * | lastChild () const const |
void | markDirty (DirtyState bits) |
QSGNode * | nextSibling () const const |
QSGNode * | parent () const const |
void | prependChildNode (QSGNode *node) |
virtual void | preprocess () |
QSGNode * | previousSibling () const const |
void | removeAllChildNodes () |
void | removeChildNode (QSGNode *node) |
void | setFlag (Flag f, bool enabled) |
void | setFlags (Flags f, bool enabled) |
NodeType | type () const const |
Additional Inherited Members | |
![]() | |
enum | DirtyStateBit |
enum | Flag |
enum | NodeType |
![]() | |
BasicNodeType | |
ClipNodeType | |
DirtyForceUpdate | |
DirtyGeometry | |
DirtyMaterial | |
DirtyMatrix | |
DirtyNodeAdded | |
DirtyNodeRemoved | |
DirtyOpacity | |
DirtyPropagationMask | |
typedef | DirtyState |
DirtySubtreeBlocked | |
DirtyUsePreprocess | |
typedef | Flags |
GeometryNodeType | |
InternalReserved | |
IsVisitableNode | |
OpacityNodeType | |
OwnedByParent | |
OwnsGeometry | |
OwnsMaterial | |
OwnsOpaqueMaterial | |
RenderNodeType | |
RootNodeType | |
TransformNodeType | |
UsePreprocess | |
Detailed Description
A QSGClipNode derived class used as a container for holding pointers to nodes and for clipping.
Upon construction RootNode generates all textures that are used by PointNode.
KStars Lite has the following hierarchy:
- RootNode - the parent of all nodes that also acts as a clipping node
- SkyItem derived nodes that acts like SkyComponent in regular KStars
- SkyNode derived nodes that represent SkyObjects (similar to SkyComponent::draw())
- Simple nodes like EllipseNode or PointNode that draw basic geometry and stars
A container for nodes that holds collection of textures for stars and provides clipping
- Version
- 1.0
Definition at line 59 of file rootnode.h.
Constructor & Destructor Documentation
◆ RootNode()
RootNode::RootNode | ( | ) |
Definition at line 47 of file rootnode.cpp.
◆ ~RootNode()
|
virtual |
Definition at line 154 of file rootnode.cpp.
Member Function Documentation
◆ cometsItem()
|
inline |
Definition at line 87 of file rootnode.h.
◆ genCachedTextures()
void RootNode::genCachedTextures | ( | ) |
initializes textureCache with cached images of stars from SkyMapLite
Definition at line 165 of file rootnode.cpp.
◆ getCachedTexture()
QSGTexture * RootNode::getCachedTexture | ( | int | size, |
char | spType ) |
returns cached texture from textureCache
- Parameters
-
size size of the star spType spectral class
- Returns
- cached QSGTexture from textureCache
Definition at line 185 of file rootnode.cpp.
◆ labelsItem()
|
inline |
Definition at line 89 of file rootnode.h.
◆ starItem()
|
inline |
Definition at line 91 of file rootnode.h.
◆ telescopeSymbolsItem()
|
inline |
Definition at line 95 of file rootnode.h.
◆ testLeakAdd()
void RootNode::testLeakAdd | ( | ) |
Definition at line 102 of file rootnode.cpp.
◆ testLeakDelete()
void RootNode::testLeakDelete | ( | ) |
Debug functions.
Definition at line 127 of file rootnode.cpp.
◆ update()
void RootNode::update | ( | bool | clearTextures = false | ) |
update positions of all child SkyItems
- Parameters
-
clearTextures true if textures for PointNodes should recreated (required when color scheme is changed)
Definition at line 216 of file rootnode.cpp.
◆ updateClipPoly()
void RootNode::updateClipPoly | ( | ) |
triangulates and sets new clipping polygon provided by Projection system
Definition at line 190 of file rootnode.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Mar 28 2025 11:57:27 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.