LabelsItem
#include <labelsitem.h>
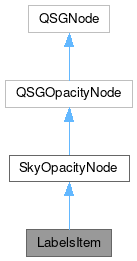
Public Types | |
enum | label_t { STAR_LABEL , ASTEROID_LABEL , COMET_LABEL , PLANET_LABEL , JUPITER_MOON_LABEL , SATURN_MOON_LABEL , DEEP_SKY_LABEL , DSO_MESSIER_LABEL , DSO_OTHER_LABEL , CONSTEL_NAME_LABEL , SATELLITE_LABEL , RUDE_LABEL , NUM_LABEL_TYPES , HORIZON_LABEL , EQUATOR_LABEL , ECLIPTIC_LABEL , TELESCOPE_SYMBOL , CATALOG_STAR_LABEL , CATALOG_DSO_LABEL , NO_LABEL } |
![]() | |
enum | DirtyStateBit |
enum | Flag |
enum | NodeType |
Public Member Functions | |
LabelsItem () | |
GuideLabelNode * | addGuideLabel (QString name, label_t labelType) |
LabelNode * | addLabel (QString name, label_t labelType) |
LabelNode * | addLabel (SkyObject *skyObject, label_t labelType) |
LabelNode * | addLabel (SkyObject *skyObject, label_t labelType, Trixel trixel) |
TrixelNode * | addTrixel (label_t labelType, Trixel trixel) |
void | deleteLabel (LabelNode *label) |
void | deleteLabels (label_t labelType) |
LabelTypeNode * | getLabelNode (label_t labelType) |
void | hideLabels (label_t labelType) |
RootNode * | rootNode () |
void | setRootNode (RootNode *rootNode) |
void | showLabels (label_t labelType) |
void | update () |
void | updateChildLabels (label_t labelType) |
![]() | |
virtual void | hide () |
virtual void | show () |
bool | visible () |
![]() | |
qreal | opacity () const const |
void | setOpacity (qreal opacity) |
![]() | |
void | appendChildNode (QSGNode *node) |
QSGNode * | childAtIndex (int i) const const |
int | childCount () const const |
QSGNode * | firstChild () const const |
Flags | flags () const const |
void | insertChildNodeAfter (QSGNode *node, QSGNode *after) |
void | insertChildNodeBefore (QSGNode *node, QSGNode *before) |
virtual bool | isSubtreeBlocked () const const |
QSGNode * | lastChild () const const |
void | markDirty (DirtyState bits) |
QSGNode * | nextSibling () const const |
QSGNode * | parent () const const |
void | prependChildNode (QSGNode *node) |
virtual void | preprocess () |
QSGNode * | previousSibling () const const |
void | removeAllChildNodes () |
void | removeChildNode (QSGNode *node) |
void | setFlag (Flag f, bool enabled) |
void | setFlags (Flags f, bool enabled) |
NodeType | type () const const |
Additional Inherited Members | |
![]() | |
BasicNodeType | |
ClipNodeType | |
DirtyForceUpdate | |
DirtyGeometry | |
DirtyMaterial | |
DirtyMatrix | |
DirtyNodeAdded | |
DirtyNodeRemoved | |
DirtyOpacity | |
DirtyPropagationMask | |
typedef | DirtyState |
DirtySubtreeBlocked | |
DirtyUsePreprocess | |
typedef | Flags |
GeometryNodeType | |
InternalReserved | |
IsVisitableNode | |
OpacityNodeType | |
OwnedByParent | |
OwnsGeometry | |
OwnsMaterial | |
OwnsOpaqueMaterial | |
RenderNodeType | |
RootNodeType | |
TransformNodeType | |
UsePreprocess | |
Detailed Description
This class is in charge of labels in SkyMapLite.
Labels can be instantiated by calling addLabel with either SkyObject or plain QString as a name. There are two types of label nodes available - LabelNode that can't be rotated and GuideLabelNode that supports rotation (but it is not used anywhere yet).
To prevent labels from overlapping this class uses SkyLabeler. We check LabelNode for overlapping by calling SkyLabeler::markText() (SkyLabeler::markRegion() for GuideLabelNode) and update().
Each of SkyItems that uses labels has its own label type in enum label_t (copied from SkyLabeler but was extended). Labels of particular type are reparented to LabelTypeNode(QSGOpacityNode) so to hide all labels of some type you just need to set opacity of LabelTypeNode that corresponds to this type to 0.
Order of drawing can be changed in LabelsItem's constructor. Order of labels update can be changed in update().
This class is not derived from SkyItem as it doesn't have label type and SkyItem's header needs an inclusion of this header to allow use of label_t enum. (Might be a good idea to fix this)
- Note
- font size is set in SkyLabeler::SkyLabeler() by initializing m_stdFont with default font
Handles labels in SkyMapLite
- Version
- 1.0
Definition at line 52 of file labelsitem.h.
Member Enumeration Documentation
◆ label_t
enum LabelsItem::label_t |
The label_t enum.
Holds types of labels
Enumerator | |
---|---|
NUM_LABEL_TYPES | Rude labels block other labels FIXME: find a better solution. |
Definition at line 61 of file labelsitem.h.
Constructor & Destructor Documentation
◆ LabelsItem()
LabelsItem::LabelsItem | ( | ) |
Constructor.
Definition at line 19 of file labelsitem.cpp.
Member Function Documentation
◆ addGuideLabel()
GuideLabelNode * LabelsItem::addGuideLabel | ( | QString | name, |
label_t | labelType ) |
does the same as above but instead creates GuideLabelNode
- Note
- currently GuideLabelNode is not used anywhere so it is not fully supported yet
Definition at line 145 of file labelsitem.cpp.
◆ addLabel() [1/3]
does the same as above but with QString instead of SkyObject
Definition at line 138 of file labelsitem.cpp.
◆ addLabel() [2/3]
Create LabelNode with given skyObject and append it to LabelTypeNode that corresponds to type.
- Parameters
-
skyObject for which the label is created labelType type of LabelTypeNode to which this label has to be reparented
- Returns
- pointer to newly created LabelNode
Definition at line 111 of file labelsitem.cpp.
◆ addLabel() [3/3]
Create LabelNode and append it to corresponding TrixelNode so that all labels can be hidden whenever Trixel is not displayed.
Use for sky objects that are indexed by SkyMesh
- Parameters
-
skyObject for which the label is created labelType type of LabelTypeNode to which this label has to be reparented trixel id of trixel
Definition at line 118 of file labelsitem.cpp.
◆ addTrixel()
TrixelNode * LabelsItem::addTrixel | ( | label_t | labelType, |
Trixel | trixel ) |
adds trixel to the node corresponding to labelType
Definition at line 152 of file labelsitem.cpp.
◆ deleteLabel()
void LabelsItem::deleteLabel | ( | LabelNode * | label | ) |
deletes particular label
Definition at line 241 of file labelsitem.cpp.
◆ deleteLabels()
void LabelsItem::deleteLabels | ( | label_t | labelType | ) |
deletes all labels of type labelType
Definition at line 214 of file labelsitem.cpp.
◆ getLabelNode()
|
inline |
- Returns
- LabelTypeNode that holds labels of labelType
Definition at line 126 of file labelsitem.h.
◆ hideLabels()
void LabelsItem::hideLabels | ( | label_t | labelType | ) |
hides all labels of type labelType
Definition at line 190 of file labelsitem.cpp.
◆ rootNode()
|
inline |
- Returns
- pointer to RootNode that instantiated this object
Definition at line 153 of file labelsitem.h.
◆ setRootNode()
void LabelsItem::setRootNode | ( | RootNode * | rootNode | ) |
sets m_rootNode and appends to it this node
- Parameters
-
rootNode parent RootNode that instantiates this object
Definition at line 202 of file labelsitem.cpp.
◆ showLabels()
void LabelsItem::showLabels | ( | label_t | labelType | ) |
shows all labels of type labelType
Definition at line 196 of file labelsitem.cpp.
◆ update()
void LabelsItem::update | ( | ) |
The order of labels update can be changed here.
updates all child labels
Definition at line 159 of file labelsitem.cpp.
◆ updateChildLabels()
void LabelsItem::updateChildLabels | ( | label_t | labelType | ) |
updates child labels of LabelTypeNode that corresponds to type in m_labelsLists Labels for stars and DSOs we update labels only if corresponding TrixelNode is visible.
- Parameters
-
labelType type of LabelTypeNode (see m_labelsLists)
Definition at line 289 of file labelsitem.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:40 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.