LabelNode
#include <labelnode.h>
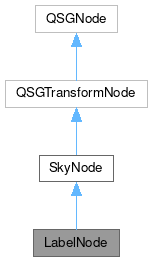
Public Member Functions | |
LabelNode (QString name, LabelsItem::label_t type) | |
LabelNode (SkyObject *skyObject, LabelsItem::label_t type) | |
virtual | ~LabelNode () |
virtual void | changePos (QPointF pos) override |
void | createTexture (QColor color=QColor()) |
void | initialize () |
LabelsItem::label_t | labelType () |
QString | name () |
void | setLabelPos (QPointF pos) |
virtual void | update () override |
bool | zoomFont () |
![]() | |
SkyNode (SkyObject *skyObject) | |
void | addChildNode (QSGNode *node) |
virtual void | hide () |
int | hideCount () |
SkyMapLite * | map () const |
const Projector * | projector () |
virtual void | show () |
SkyObject * | skyObject () const |
void | update (bool drawLabel) |
bool | visible () |
![]() | |
const QMatrix4x4 & | matrix () const const |
void | setMatrix (const QMatrix4x4 &matrix) |
![]() | |
void | appendChildNode (QSGNode *node) |
QSGNode * | childAtIndex (int i) const const |
int | childCount () const const |
QSGNode * | firstChild () const const |
Flags | flags () const const |
void | insertChildNodeAfter (QSGNode *node, QSGNode *after) |
void | insertChildNodeBefore (QSGNode *node, QSGNode *before) |
virtual bool | isSubtreeBlocked () const const |
QSGNode * | lastChild () const const |
void | markDirty (DirtyState bits) |
QSGNode * | nextSibling () const const |
QSGNode * | parent () const const |
void | prependChildNode (QSGNode *node) |
virtual void | preprocess () |
QSGNode * | previousSibling () const const |
void | removeAllChildNodes () |
void | removeChildNode (QSGNode *node) |
void | setFlag (Flag f, bool enabled) |
void | setFlags (Flags f, bool enabled) |
NodeType | type () const const |
Public Attributes | |
QPointF | labelPos |
![]() | |
SkyOpacityNode * | m_opacity { nullptr } |
![]() | |
BasicNodeType | |
ClipNodeType | |
DirtyForceUpdate | |
DirtyGeometry | |
DirtyMaterial | |
DirtyMatrix | |
DirtyNodeAdded | |
DirtyNodeRemoved | |
DirtyOpacity | |
DirtyPropagationMask | |
typedef | DirtyState |
DirtySubtreeBlocked | |
DirtyUsePreprocess | |
typedef | Flags |
GeometryNodeType | |
InternalReserved | |
IsVisitableNode | |
OpacityNodeType | |
OwnedByParent | |
OwnsGeometry | |
OwnsMaterial | |
OwnsOpaqueMaterial | |
RenderNodeType | |
RootNodeType | |
TransformNodeType | |
UsePreprocess | |
Additional Inherited Members | |
![]() | |
enum | DirtyStateBit |
enum | Flag |
enum | NodeType |
![]() | |
bool | m_drawLabel { false } |
int | m_hideCount { 0 } |
SkyObject * | m_skyObject { nullptr } |
Detailed Description
A SkyNode derived class used for displaying labels.
- Version
- 1.0
Definition at line 26 of file labelnode.h.
Constructor & Destructor Documentation
◆ LabelNode() [1/2]
LabelNode::LabelNode | ( | SkyObject * | skyObject, |
LabelsItem::label_t | type ) |
Constructor.
Use name of skyObject as a text
- Parameters
-
skyObject - target object, for which this label is created. type - type of label (corresponds to type of SkyObject)
Definition at line 15 of file labelnode.cpp.
◆ LabelNode() [2/2]
LabelNode::LabelNode | ( | QString | name, |
LabelsItem::label_t | type ) |
Constructor.
Use string parameter name as a text
- Parameters
-
name - text of label type - type of label (corresponds to type of SkyObject)
Definition at line 22 of file labelnode.cpp.
◆ ~LabelNode()
|
virtual |
Destructor.
Definition at line 28 of file labelnode.cpp.
Member Function Documentation
◆ changePos()
|
overridevirtual |
Changes position of the label.
- Parameters
-
pos - new position
Reimplemented from SkyNode.
Definition at line 114 of file labelnode.cpp.
◆ createTexture()
Create texture from label's name.
- Parameters
-
color - color of the label
Definition at line 74 of file labelnode.cpp.
◆ initialize()
void LabelNode::initialize | ( | ) |
Convenience function to not to repeat the same code in 2 constructors.
Set parameters of label based on its type
Definition at line 35 of file labelnode.cpp.
◆ labelType()
|
inline |
Definition at line 62 of file labelnode.h.
◆ name()
|
inline |
Definition at line 60 of file labelnode.h.
◆ setLabelPos()
void LabelNode::setLabelPos | ( | QPointF | pos | ) |
set the position of label with the given offset from SkyObject's position and makes the label visible if it was hidden
- Warning
- Keep mind that to update labels position, you should first set it with setLabelPos() and then call update()
- Parameters
-
pos position of label
Definition at line 123 of file labelnode.cpp.
◆ update()
|
overridevirtual |
Update position of label according to labelPos and recreate texture if label's size depends on zoom level.
Reimplemented from SkyNode.
Definition at line 135 of file labelnode.cpp.
◆ zoomFont()
|
inline |
- Returns
- true if the size of text depends on zoom
Definition at line 73 of file labelnode.h.
Member Data Documentation
◆ labelPos
QPointF LabelNode::labelPos |
Definition at line 90 of file labelnode.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Jul 26 2024 11:59:53 by doxygen 1.11.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.