QCPSelectionDecoratorBracket
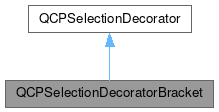
Public Types | |
enum | BracketStyle { bsSquareBracket , bsHalfEllipse , bsEllipse , bsPlus , bsUserStyle } |
Protected Member Functions | |
QPointF | getPixelCoordinates (const QCPPlottableInterface1D *interface1d, int dataIndex) const |
double | getTangentAngle (const QCPPlottableInterface1D *interface1d, int dataIndex, int direction) const |
![]() | |
virtual bool | registerWithPlottable (QCPAbstractPlottable *plottable) |
Protected Attributes | |
QBrush | mBracketBrush |
int | mBracketHeight |
QPen | mBracketPen |
BracketStyle | mBracketStyle |
int | mBracketWidth |
int | mTangentAverage |
bool | mTangentToData |
![]() | |
QBrush | mBrush |
QPen | mPen |
QCPAbstractPlottable * | mPlottable |
QCPScatterStyle | mScatterStyle |
QCPScatterStyle::ScatterProperties | mUsedScatterProperties |
Detailed Description
A selection decorator which draws brackets around each selected data segment.
Additionally to the regular highlighting of selected segments via color, fill and scatter style, this QCPSelectionDecorator subclass draws markers at the begin and end of each selected data segment of the plottable.
The shape of the markers can be controlled with setBracketStyle, setBracketWidth and setBracketHeight. The color/fill can be controlled with setBracketPen and setBracketBrush.
To introduce custom bracket styles, it is only necessary to sublcass QCPSelectionDecoratorBracket and reimplement drawBracket. The rest will be managed by the base class.
Definition at line 4855 of file qcustomplot.h.
Member Enumeration Documentation
◆ BracketStyle
Defines which shape is drawn at the boundaries of selected data ranges.
Some of the bracket styles further allow specifying a height and/or width, see setBracketHeight and setBracketWidth.
Enumerator | |
---|---|
bsSquareBracket | A square bracket is drawn. |
bsHalfEllipse | A half ellipse is drawn. The size of the ellipse is given by the bracket width/height properties. |
bsEllipse | An ellipse is drawn. The size of the ellipse is given by the bracket width/height properties. |
bsPlus | A plus is drawn. |
bsUserStyle | Start custom bracket styles at this index when subclassing and reimplementing drawBracket. |
Definition at line 4866 of file qcustomplot.h.
Constructor & Destructor Documentation
◆ QCPSelectionDecoratorBracket()
QCPSelectionDecoratorBracket::QCPSelectionDecoratorBracket | ( | ) |
Creates a new QCPSelectionDecoratorBracket instance with default values.
Definition at line 17164 of file qcustomplot.cpp.
◆ ~QCPSelectionDecoratorBracket()
|
overridevirtual |
Definition at line 17176 of file qcustomplot.cpp.
Member Function Documentation
◆ bracketBrush()
|
inline |
Definition at line 4879 of file qcustomplot.h.
◆ bracketHeight()
|
inline |
Definition at line 4881 of file qcustomplot.h.
◆ bracketPen()
|
inline |
Definition at line 4878 of file qcustomplot.h.
◆ bracketStyle()
|
inline |
Definition at line 4882 of file qcustomplot.h.
◆ bracketWidth()
|
inline |
Definition at line 4880 of file qcustomplot.h.
◆ drawBracket()
|
virtual |
Draws the bracket shape with painter. The parameter direction is either -1 or 1 and indicates whether the bracket shall point to the left or the right (i.e. is a closing or opening bracket, respectively).
The passed painter already contains all transformations that are necessary to position and rotate the bracket appropriately. Painting operations can be performed as if drawing upright brackets on flat data with horizontal key axis, with (0, 0) being the center of the bracket.
If you wish to sublcass QCPSelectionDecoratorBracket in order to provide custom bracket shapes (see QCPSelectionDecoratorBracket::bsUserStyle), this is the method you should reimplement.
Definition at line 17268 of file qcustomplot.cpp.
◆ drawDecoration()
|
overridevirtual |
Draws the bracket decoration on the data points at the begin and end of each selected data segment given in seletion.
It uses the method drawBracket to actually draw the shapes.
\seebaseclassmethod
Reimplemented from QCPSelectionDecorator.
Definition at line 17311 of file qcustomplot.cpp.
◆ getPixelCoordinates()
|
protected |
Returns the pixel coordinates of the data point at dataIndex, using interface1d to access the data points.
Definition at line 17410 of file qcustomplot.cpp.
◆ getTangentAngle()
|
protected |
If setTangentToData is enabled, brackets need to be rotated according to the data slope. This method returns the angle in radians by which a bracket at the given dataIndex must be rotated.
The parameter direction must be set to either -1 or 1, representing whether it is an opening or closing bracket. Since for slope calculation multiple data points are required, this defines the direction in which the algorithm walks, starting at dataIndex, to average those data points. (see setTangentToData and setTangentAverage)
interface1d is the interface to the plottable's data which is used to query data coordinates.
Definition at line 17363 of file qcustomplot.cpp.
◆ setBracketBrush()
void QCPSelectionDecoratorBracket::setBracketBrush | ( | const QBrush & | brush | ) |
Sets the brush that will be used to draw the brackets at the beginning and end of each selected data segment.
Definition at line 17193 of file qcustomplot.cpp.
◆ setBracketHeight()
void QCPSelectionDecoratorBracket::setBracketHeight | ( | int | height | ) |
Sets the height of the drawn bracket. The height dimension is always perpendicular to the key axis of the data, or the tangent direction of the current data slope, if setTangentToData is enabled.
Definition at line 17213 of file qcustomplot.cpp.
◆ setBracketPen()
void QCPSelectionDecoratorBracket::setBracketPen | ( | const QPen & | pen | ) |
Sets the pen that will be used to draw the brackets at the beginning and end of each selected data segment.
Definition at line 17184 of file qcustomplot.cpp.
◆ setBracketStyle()
void QCPSelectionDecoratorBracket::setBracketStyle | ( | QCPSelectionDecoratorBracket::BracketStyle | style | ) |
Sets the shape that the bracket/marker will have.
- See also
- setBracketWidth, setBracketHeight
Definition at line 17223 of file qcustomplot.cpp.
◆ setBracketWidth()
void QCPSelectionDecoratorBracket::setBracketWidth | ( | int | width | ) |
Sets the width of the drawn bracket. The width dimension is always parallel to the key axis of the data, or the tangent direction of the current data slope, if setTangentToData is enabled.
Definition at line 17203 of file qcustomplot.cpp.
◆ setTangentAverage()
void QCPSelectionDecoratorBracket::setTangentAverage | ( | int | pointCount | ) |
Controls over how many data points the slope shall be averaged, when brackets shall be aligned with the data (if setTangentToData is true).
From the position of the bracket, pointCount points towards the selected data range will be taken into account. The smallest value of pointCount is 1, which is effectively equivalent to disabling setTangentToData.
Definition at line 17248 of file qcustomplot.cpp.
◆ setTangentToData()
void QCPSelectionDecoratorBracket::setTangentToData | ( | bool | enabled | ) |
Sets whether the brackets will be rotated such that they align with the slope of the data at the position that they appear in.
For noisy data, it might be more visually appealing to average the slope over multiple data points. This can be configured via setTangentAverage.
Definition at line 17235 of file qcustomplot.cpp.
◆ tangentAverage()
|
inline |
Definition at line 4884 of file qcustomplot.h.
◆ tangentToData()
|
inline |
Definition at line 4883 of file qcustomplot.h.
Member Data Documentation
◆ mBracketBrush
|
protected |
Definition at line 4904 of file qcustomplot.h.
◆ mBracketHeight
|
protected |
Definition at line 4906 of file qcustomplot.h.
◆ mBracketPen
|
protected |
Definition at line 4903 of file qcustomplot.h.
◆ mBracketStyle
|
protected |
Definition at line 4907 of file qcustomplot.h.
◆ mBracketWidth
|
protected |
Definition at line 4905 of file qcustomplot.h.
◆ mTangentAverage
|
protected |
Definition at line 4909 of file qcustomplot.h.
◆ mTangentToData
|
protected |
Definition at line 4908 of file qcustomplot.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:47:17 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.