kmail
KMFilterMgr Class Reference
#include <kmfiltermgr.h>
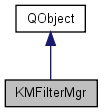
Public Types | |
enum | FilterSet { NoSet = 0x0, Inbound = 0x1, Outbound = 0x2, Explicit = 0x4, All = Inbound|Outbound|Explicit } |
Public Slots | |
void | slotFolderRemoved (KMFolder *aFolder) |
Signals | |
void | filterListUpdated () |
Public Member Functions | |
void | appendFilters (const QValueList< KMFilter * > &filters, bool replaceIfNameExists=false) |
bool | atLeastOneFilterAppliesTo (unsigned int accountID) const |
bool | atLeastOneIncomingFilterAppliesTo (unsigned int accountID) const |
bool | atLeastOneOnlineImapFolderTarget () |
bool | beginFiltering (KMMsgBase *msgBase) const |
void | beginUpdate () |
void | cleanup () |
void | clear () |
void | createFilter (const QCString &field, const QString &value) |
const QString | createUniqueName (const QString &name) |
void | deref (bool force=false) |
void | dump () const |
void | endFiltering (KMMsgBase *msgBase) const |
void | endUpdate () |
const QValueList< KMFilter * > & | filters () const |
void | folderCreated (KMFolder *) |
bool | folderRemoved (KMFolder *aFolder, KMFolder *aNewFolder) |
KMFilterMgr (bool popFilter=false) | |
int | moveMessage (KMMessage *msg) const |
void | openDialog (QWidget *parent, bool checkForEmptyFilterList=true) |
int | process (Q_UINT32 serNum, const KMFilter *filter) |
int | process (KMMessage *msg, const KMFilter *filter) |
int | process (KMMessage *msg, FilterSet aSet=Inbound, bool account=false, uint accountId=0) |
void | readConfig (void) |
void | ref () |
void | setFilters (const QValueList< KMFilter * > &filters) |
void | setShowLaterMsgs (bool show) |
bool | showLaterMsgs () const |
int | tempOpenFolder (KMFolder *aFolder) |
void | writeConfig (bool withSync=TRUE) |
virtual | ~KMFilterMgr () |
Detailed Description
Definition at line 34 of file kmfiltermgr.h.
Member Enumeration Documentation
Constructor & Destructor Documentation
KMFilterMgr::KMFilterMgr | ( | bool | popFilter = false |
) |
Definition at line 37 of file kmfiltermgr.cpp.
KMFilterMgr::~KMFilterMgr | ( | ) | [virtual] |
Definition at line 53 of file kmfiltermgr.cpp.
Member Function Documentation
void KMFilterMgr::appendFilters | ( | const QValueList< KMFilter * > & | filters, | |
bool | replaceIfNameExists = false | |||
) |
Append the list of filters to the current list of filters and write everything back into the configuration.
The filter manager takes ownership of the filters in the list.
Definition at line 441 of file kmfiltermgr.cpp.
bool KMFilterMgr::atLeastOneFilterAppliesTo | ( | unsigned int | accountID | ) | const |
Returns whether at least one filter applies to this account, which means that mail must be downloaded in order to be filtered, for example;.
Definition at line 307 of file kmfiltermgr.cpp.
bool KMFilterMgr::atLeastOneIncomingFilterAppliesTo | ( | unsigned int | accountID | ) | const |
Returns whether at least one incoming filter applies to this account, which means that mail must be downloaded in order to be filtered, for example;.
Definition at line 318 of file kmfiltermgr.cpp.
bool KMFilterMgr::atLeastOneOnlineImapFolderTarget | ( | ) |
Returns whether at least one filter targets a folder on an online IMAP account.
Definition at line 329 of file kmfiltermgr.cpp.
bool KMFilterMgr::beginFiltering | ( | KMMsgBase * | msgBase | ) | const |
Definition at line 104 of file kmfiltermgr.cpp.
void KMFilterMgr::beginUpdate | ( | ) | [inline] |
Called at the beginning of an filter list update.
Currently a no-op
Definition at line 147 of file kmfiltermgr.h.
void KMFilterMgr::cleanup | ( | ) |
void KMFilterMgr::clear | ( | ) |
Open an edit dialog, create a new filter and preset the first rule with "field equals value".
Definition at line 408 of file kmfiltermgr.cpp.
Check for existing filters with the &p name and extend the "name" to "name (i)" until no match is found for i=1.
.n
Definition at line 416 of file kmfiltermgr.cpp.
void KMFilterMgr::deref | ( | bool | force = false |
) |
Decrement the reference count for the filter manager.
Call this method after processing messages with process(). Shall be called after all messages are processed. If the reference count is zero then this method closes all folders that have been temporarily opened with tempOpenFolder().
Definition at line 363 of file kmfiltermgr.cpp.
void KMFilterMgr::dump | ( | void | ) | const |
void KMFilterMgr::endFiltering | ( | KMMsgBase * | msgBase | ) | const |
Definition at line 128 of file kmfiltermgr.cpp.
void KMFilterMgr::endUpdate | ( | void | ) |
void KMFilterMgr::filterListUpdated | ( | ) | [signal] |
const QValueList<KMFilter*>& KMFilterMgr::filters | ( | ) | const [inline] |
void KMFilterMgr::folderCreated | ( | KMFolder * | ) | [inline] |
Called from the folder manager when a new folder has been created.
Forwards this to the filter dialog if that is open.
Definition at line 165 of file kmfiltermgr.h.
Called from the folder manager when a folder is removed.
Tests if the folder aFolder is used in any action. Changes to aNewFolder folder in this case. Returns TRUE if a change occurred.
Definition at line 478 of file kmfiltermgr.cpp.
int KMFilterMgr::moveMessage | ( | KMMessage * | msg | ) | const |
Definition at line 116 of file kmfiltermgr.cpp.
void KMFilterMgr::openDialog | ( | QWidget * | parent, | |
bool | checkForEmptyFilterList = true | |||
) |
Open an edit dialog.
If checkForEmptyFilterList is true, an empty filter is created to improve the visibility of the dialog in case no filter has been defined so far.
Definition at line 392 of file kmfiltermgr.cpp.
int KMFilterMgr::process | ( | Q_UINT32 | serNum, | |
const KMFilter * | filter | |||
) |
For ad-hoc filters.
Applies filter
to message with serNum
. Return codes are as with the above method.
Definition at line 179 of file kmfiltermgr.cpp.
For ad-hoc filters.
Applies filter
to msg
. Return codes are as with the above method.
- Deprecated:
- Use int process( quint32, const KMFilter * )
Definition at line 145 of file kmfiltermgr.cpp.
int KMFilterMgr::process | ( | KMMessage * | msg, | |
FilterSet | aSet = Inbound , |
|||
bool | account = false , |
|||
uint | accountId = 0 | |||
) |
Process given message by applying the filter rules one by one.
You can select which set of filters (incoming or outgoing) should be used.
- Parameters:
-
msg The message to process. aSet Select the filter set to use. account true if an account id is specified else false accountId The id of the KMAccount that the message was retrieved from
- Returns:
- 2 if a critical error occurred (eg out of disk space) 1 if the caller is still owner of the message and 0 otherwise. If the caller does not any longer own the message he *must* not delete the message or do similar stupid things. ;-)
Definition at line 228 of file kmfiltermgr.cpp.
void KMFilterMgr::readConfig | ( | void | ) |
void KMFilterMgr::ref | ( | void | ) |
Increment the reference count for the filter manager.
Call this method before processing messages with process()
Definition at line 357 of file kmfiltermgr.cpp.
void KMFilterMgr::setFilters | ( | const QValueList< KMFilter * > & | filters | ) |
Replace the list of filters under control of the filter manager.
The manager takes ownershipt of the filters.
Definition at line 463 of file kmfiltermgr.cpp.
void KMFilterMgr::setShowLaterMsgs | ( | bool | show | ) | [inline] |
bool KMFilterMgr::showLaterMsgs | ( | ) | const [inline] |
void KMFilterMgr::slotFolderRemoved | ( | KMFolder * | aFolder | ) | [slot] |
Definition at line 472 of file kmfiltermgr.cpp.
int KMFilterMgr::tempOpenFolder | ( | KMFolder * | aFolder | ) |
Open given folder and mark it as temporarily open.
The folder will be closed upon next call of cleanip(). This method is usually only called from within filter actions during process(). Returns returncode from KMFolder::open() call.
Definition at line 379 of file kmfiltermgr.cpp.
void KMFilterMgr::writeConfig | ( | bool | withSync = TRUE |
) |
The documentation for this class was generated from the following files: