marble
#include <MarbleDeclarativeWidget.h>
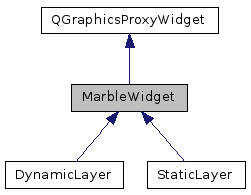
Public Slots | |
QStringList | activeFloatItems () const |
Coordinate * | center () |
void | centerOn (const Marble::GeoDataLatLonBox &bbox) |
void | centerOn (const GeoDataCoordinates &coordinates) |
bool | containsFloatItem (const QString &name) |
bool | containsRenderPlugin (const QString &name) |
Coordinate * | coordinate (int x, int y) |
void | downloadArea (int topTileLevel, int bottomTileLevel) |
void | downloadRoute (qreal offset, int topTileLevel, int bottomTileLevel) |
Marble::AbstractFloatItem * | floatItem (const QString &name) |
QList< QObject * > | floatItems () const |
bool | inputEnabled () const |
QString | mapThemeId () const |
QStandardItemModel * | mapThemeModel () |
QPoint | pixel (qreal longitude, qreal latitude) const |
QString | projection () const |
Marble::RenderPlugin * | renderPlugin (const QString &name) |
QList< QObject * > | renderPlugins () const |
void | setActiveFloatItems (const QStringList &items) |
void | setCenter (Coordinate *center) |
void | setDataPluginDelegate (const QString &plugin, QDeclarativeComponent *delegate) |
void | setGeoSceneProperty (const QString &key, bool value) |
void | setInputEnabled (bool enabled) |
void | setMapThemeId (const QString &mapThemeId) |
void | setProjection (const QString &projection) |
void | zoomIn () |
void | zoomOut () |
Signals | |
void | mapThemeChanged () |
void | mapThemeModelChanged () |
void | mouseClickGeoPosition (qreal longitude, qreal latitude) |
void | placemarkSelected (Placemark *placemark) |
void | projectionChanged () |
void | radiusChanged () |
void | visibleLatLonAltBoxChanged () |
void | workOfflineChanged () |
Public Member Functions | |
MarbleWidget (QGraphicsItem *parent=0, Qt::WindowFlags flags=0) | |
~MarbleWidget () | |
QStringList | activeRenderPlugins () const |
QDeclarativeListProperty< QObject > | childList () |
QDeclarativeListProperty < DeclarativeDataPlugin > | dataLayers () |
Marble::MarbleModel * | model () |
int | radius () const |
void | setActiveRenderPlugins (const QStringList &items) |
void | setRadius (int radius) |
void | setWorkOffline (bool workOffline) |
const Marble::ViewportParams * | viewport () const |
bool | workOffline () const |
Protected Member Functions | |
virtual bool | event (QEvent *event) |
virtual bool | sceneEvent (QEvent *event) |
Properties | |
QStringList | activeFloatItems |
QStringList | activeRenderPlugins |
Coordinate | center |
QDeclarativeListProperty< QObject > | children |
QDeclarativeListProperty < DeclarativeDataPlugin > | dataLayers |
QList< QObject * > | floatItems |
bool | inputEnabled |
QString | mapThemeId |
QStandardItemModel | mapThemeModel |
QString | projection |
int | radius |
QList< QObject * > | renderPlugins |
bool | workOffline |
Detailed Description
Wraps a Marble::MarbleWidget, providing access to important properties and methods.
- Todo:
- FIXME: Currently stuffed in a QGraphicsProxyWidget as otherwise it is only displayed in QML when it is the only widget. For performance reasons it would be nice to avoid this.
Definition at line 50 of file MarbleDeclarativeWidget.h.
Constructor & Destructor Documentation
|
explicit |
Constructor.
Definition at line 43 of file MarbleDeclarativeWidget.cpp.
MarbleWidget::~MarbleWidget | ( | ) |
Definition at line 78 of file MarbleDeclarativeWidget.cpp.
Member Function Documentation
|
slot |
Returns a list of active (!) float items.
QStringList MarbleWidget::activeRenderPlugins | ( | ) | const |
|
slot |
|
slot |
Definition at line 261 of file MarbleDeclarativeWidget.cpp.
|
slot |
Definition at line 266 of file MarbleDeclarativeWidget.cpp.
QDeclarativeListProperty< QObject > MarbleWidget::childList | ( | ) |
Definition at line 136 of file MarbleDeclarativeWidget.cpp.
|
slot |
Definition at line 435 of file MarbleDeclarativeWidget.cpp.
|
slot |
Definition at line 403 of file MarbleDeclarativeWidget.cpp.
|
slot |
Returns the coordinate at the given screen position.
Definition at line 233 of file MarbleDeclarativeWidget.cpp.
QDeclarativeListProperty<DeclarativeDataPlugin> MarbleWidget::dataLayers | ( | ) |
|
slot |
Definition at line 330 of file MarbleDeclarativeWidget.cpp.
|
slot |
Definition at line 318 of file MarbleDeclarativeWidget.cpp.
|
protectedvirtual |
Definition at line 373 of file MarbleDeclarativeWidget.cpp.
|
slot |
Definition at line 424 of file MarbleDeclarativeWidget.cpp.
|
slot |
|
slot |
Returns true if the map accepts keyboard/mouse input.
|
signal |
|
slot |
Returns the currently active map theme id, if any, in the form of e.g.
"earth/openstreetmap/openstreetmap.dgml"
|
slot |
|
signal |
Marble::MarbleModel * MarbleWidget::model | ( | ) |
Definition at line 85 of file MarbleDeclarativeWidget.cpp.
|
signal |
|
slot |
Returns the screen position of the given coordinate (can be out of the screen borders)
Definition at line 223 of file MarbleDeclarativeWidget.cpp.
|
signal |
|
slot |
Returns the active projection which can be either "Equirectangular", "Mercator" or "Spherical".
|
signal |
int MarbleWidget::radius | ( | ) | const |
|
signal |
|
slot |
Definition at line 392 of file MarbleDeclarativeWidget.cpp.
|
slot |
|
protectedvirtual |
Definition at line 382 of file MarbleDeclarativeWidget.cpp.
|
slot |
Activates all of the given float items and deactivates any others.
Definition at line 116 of file MarbleDeclarativeWidget.cpp.
void MarbleWidget::setActiveRenderPlugins | ( | const QStringList & | items | ) |
Definition at line 158 of file MarbleDeclarativeWidget.cpp.
|
slot |
Definition at line 249 of file MarbleDeclarativeWidget.cpp.
|
slot |
Definition at line 342 of file MarbleDeclarativeWidget.cpp.
|
slot |
Definition at line 313 of file MarbleDeclarativeWidget.cpp.
|
slot |
Toggle keyboard/mouse input.
Definition at line 171 of file MarbleDeclarativeWidget.cpp.
|
slot |
Change the currently active map theme id.
Ignored if the given map theme id is invalid (not installed).
- See also
- DeclarativeMapThemeManager
Definition at line 182 of file MarbleDeclarativeWidget.cpp.
|
slot |
Change the active projection.
Accepted values are "Equirectangular", "Mercator" and "Spherical"
Definition at line 202 of file MarbleDeclarativeWidget.cpp.
void MarbleWidget::setRadius | ( | int | radius | ) |
Definition at line 368 of file MarbleDeclarativeWidget.cpp.
void MarbleWidget::setWorkOffline | ( | bool | workOffline | ) |
Definition at line 358 of file MarbleDeclarativeWidget.cpp.
const Marble::ViewportParams * MarbleWidget::viewport | ( | ) | const |
Definition at line 90 of file MarbleDeclarativeWidget.cpp.
|
signal |
Forwarded from MarbleWidget.
Zoom value and/or center position have changed
bool MarbleWidget::workOffline | ( | ) | const |
|
signal |
|
slot |
Zoom in by a fixed amount.
Definition at line 213 of file MarbleDeclarativeWidget.cpp.
|
slot |
Zoom out by a fixed amount.
Definition at line 218 of file MarbleDeclarativeWidget.cpp.
Property Documentation
|
readwrite |
Definition at line 60 of file MarbleDeclarativeWidget.h.
|
readwrite |
Definition at line 61 of file MarbleDeclarativeWidget.h.
|
readwrite |
Definition at line 54 of file MarbleDeclarativeWidget.h.
|
read |
Definition at line 68 of file MarbleDeclarativeWidget.h.
|
read |
Definition at line 67 of file MarbleDeclarativeWidget.h.
|
read |
Definition at line 64 of file MarbleDeclarativeWidget.h.
|
readwrite |
Definition at line 58 of file MarbleDeclarativeWidget.h.
|
readwrite |
Definition at line 56 of file MarbleDeclarativeWidget.h.
|
read |
Definition at line 62 of file MarbleDeclarativeWidget.h.
|
readwrite |
Definition at line 57 of file MarbleDeclarativeWidget.h.
|
readwrite |
Definition at line 55 of file MarbleDeclarativeWidget.h.
|
read |
Definition at line 63 of file MarbleDeclarativeWidget.h.
|
readwrite |
Definition at line 59 of file MarbleDeclarativeWidget.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:38:55 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.