marble
#include <GeoDataContainer.h>
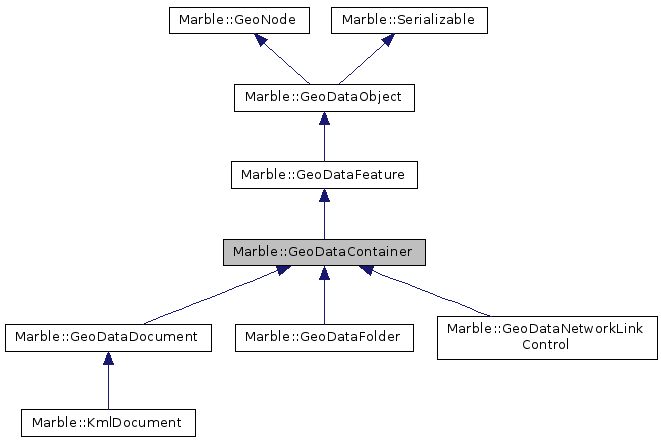
Public Member Functions | |
GeoDataContainer () | |
GeoDataContainer (const GeoDataContainer &other) | |
virtual | ~GeoDataContainer () |
void | append (GeoDataFeature *other) |
GeoDataFeature & | at (int pos) |
const GeoDataFeature & | at (int pos) const |
QVector< GeoDataFeature * > ::Iterator | begin () |
GeoDataFeature * | child (int) |
const GeoDataFeature * | child (int) const |
int | childPosition (const GeoDataFeature *child) const |
void | clear () |
QVector< GeoDataFeature * > ::ConstIterator | constBegin () const |
QVector< GeoDataFeature * > ::ConstIterator | constEnd () const |
QVector< GeoDataFeature * > ::Iterator | end () |
QVector< GeoDataFeature * > | featureList () const |
GeoDataFeature & | first () |
const GeoDataFeature & | first () const |
QVector< GeoDataFolder * > | folderList () const |
void | insert (GeoDataFeature *other, int index) |
GeoDataFeature & | last () |
const GeoDataFeature & | last () const |
GeoDataLatLonAltBox | latLonAltBox () const |
GeoDataContainerPrivate * | p () const |
virtual void | pack (QDataStream &stream) const |
QVector< GeoDataPlacemark * > | placemarkList () const |
void | remove (int index) |
int | size () const |
virtual void | unpack (QDataStream &stream) |
![]() | |
GeoDataFeature () | |
GeoDataFeature (const QString &name) | |
GeoDataFeature (const GeoDataFeature &other) | |
virtual | ~GeoDataFeature () |
const GeoDataAbstractView * | abstractView () const |
GeoDataAbstractView * | abstractView () |
QString | address () const |
QString | description () const |
bool | descriptionIsCDATA () const |
virtual void | detach () |
GeoDataExtendedData & | extendedData () const |
EnumFeatureId | featureId () const |
bool | isGloballyVisible () const |
bool | isVisible () const |
QString | name () const |
virtual const char * | nodeType () const |
GeoDataFeature & | operator= (const GeoDataFeature &other) |
QString | phoneNumber () const |
qint64 | popularity () const |
GeoDataRegion & | region () const |
const QString | role () const |
void | setAbstractView (GeoDataAbstractView *abstractView) |
void | setAddress (const QString &value) |
void | setDescription (const QString &value) |
void | setDescriptionCDATA (bool cdata) |
void | setExtendedData (const GeoDataExtendedData &extendedData) |
void | setName (const QString &value) |
void | setPhoneNumber (const QString &value) |
void | setPopularity (qint64 popularity) |
void | setRegion (const GeoDataRegion ®ion) |
void | setRole (const QString &role) |
void | setStyle (GeoDataStyle *style) |
void | setStyleMap (const GeoDataStyleMap *map) |
void | setStyleUrl (const QString &value) |
void | setTimeSpan (const GeoDataTimeSpan &timeSpan) |
void | setTimeStamp (const GeoDataTimeStamp &timeStamp) |
void | setVisible (bool value) |
void | setVisualCategory (GeoDataVisualCategory category) |
void | setZoomLevel (int index) |
const GeoDataStyle * | style () const |
const GeoDataStyleMap * | styleMap () const |
QString | styleUrl () const |
GeoDataTimeSpan & | timeSpan () const |
GeoDataTimeStamp & | timeStamp () const |
GeoDataVisualCategory | visualCategory () const |
int | zoomLevel () const |
![]() | |
GeoDataObject () | |
GeoDataObject (const GeoDataObject &) | |
virtual | ~GeoDataObject () |
int | id () const |
GeoDataObject & | operator= (const GeoDataObject &) |
virtual GeoDataObject * | parent () const |
QString | resolvePath (const QString &relativePath) const |
void | setId (int value) |
virtual void | setParent (GeoDataObject *parent) |
void | setTargetId (int value) |
int | targetId () const |
![]() | |
GeoNode () | |
virtual | ~GeoNode () |
![]() | |
virtual | ~Serializable () |
Detailed Description
A base class that can hold GeoDataFeatures.
GeoDataContainer is the base class for the GeoData container classes GeoDataFolder and GeoDataDocument. It is never instantiated by itself, but is always used as part of a derived class.
It is based on GeoDataFeature, and it only adds a QVector<GeodataFeature *> to it, making it a Feature that can hold other Features.
- See also
- GeoDataFolder
- GeoDataDocument
Definition at line 47 of file GeoDataContainer.h.
Constructor & Destructor Documentation
Marble::GeoDataContainer::GeoDataContainer | ( | ) |
Default constructor.
Definition at line 29 of file GeoDataContainer.cpp.
Marble::GeoDataContainer::GeoDataContainer | ( | const GeoDataContainer & | other | ) |
Definition at line 39 of file GeoDataContainer.cpp.
|
virtual |
Destruct the GeoDataContainer.
Definition at line 44 of file GeoDataContainer.cpp.
Member Function Documentation
void Marble::GeoDataContainer::append | ( | GeoDataFeature * | other | ) |
add an element
Definition at line 165 of file GeoDataContainer.cpp.
GeoDataFeature & Marble::GeoDataContainer::at | ( | int | pos | ) |
return the reference of the element at a specific position
Definition at line 184 of file GeoDataContainer.cpp.
const GeoDataFeature & Marble::GeoDataContainer::at | ( | int | pos | ) | const |
Definition at line 190 of file GeoDataContainer.cpp.
QVector< GeoDataFeature * >::Iterator Marble::GeoDataContainer::begin | ( | ) |
Definition at line 224 of file GeoDataContainer.cpp.
GeoDataFeature * Marble::GeoDataContainer::child | ( | int | i | ) |
returns the requested child item
Definition at line 132 of file GeoDataContainer.cpp.
const GeoDataFeature * Marble::GeoDataContainer::child | ( | int | i | ) | const |
returns the requested child item
Definition at line 137 of file GeoDataContainer.cpp.
int Marble::GeoDataContainer::childPosition | ( | const GeoDataFeature * | child | ) | const |
returns the position of an item in the list
Definition at line 145 of file GeoDataContainer.cpp.
void Marble::GeoDataContainer::clear | ( | ) |
Definition at line 217 of file GeoDataContainer.cpp.
QVector< GeoDataFeature * >::ConstIterator Marble::GeoDataContainer::constBegin | ( | ) | const |
Definition at line 234 of file GeoDataContainer.cpp.
QVector< GeoDataFeature * >::ConstIterator Marble::GeoDataContainer::constEnd | ( | ) | const |
Definition at line 239 of file GeoDataContainer.cpp.
QVector< GeoDataFeature * >::Iterator Marble::GeoDataContainer::end | ( | ) |
Definition at line 229 of file GeoDataContainer.cpp.
QVector< GeoDataFeature * > Marble::GeoDataContainer::featureList | ( | ) | const |
A convenience function that returns all features in this container.
- Returns
- A QVector of GeoDataFeature
- See also
- GeoDataFeature
Definition at line 124 of file GeoDataContainer.cpp.
GeoDataFeature & Marble::GeoDataContainer::first | ( | ) |
return the reference of the last element for convenience
Definition at line 206 of file GeoDataContainer.cpp.
const GeoDataFeature & Marble::GeoDataContainer::first | ( | ) | const |
Definition at line 212 of file GeoDataContainer.cpp.
QVector< GeoDataFolder * > Marble::GeoDataContainer::folderList | ( | ) | const |
A convenience function that returns all folders in this container.
- Returns
- A QVector of GeoDataFolder
- See also
- GeoDataFolder
Definition at line 90 of file GeoDataContainer.cpp.
void Marble::GeoDataContainer::insert | ( | GeoDataFeature * | other, |
int | index | ||
) |
Definition at line 158 of file GeoDataContainer.cpp.
GeoDataFeature & Marble::GeoDataContainer::last | ( | ) |
return the reference of the last element for convenience
Definition at line 195 of file GeoDataContainer.cpp.
const GeoDataFeature & Marble::GeoDataContainer::last | ( | ) | const |
Definition at line 201 of file GeoDataContainer.cpp.
GeoDataLatLonAltBox Marble::GeoDataContainer::latLonAltBox | ( | ) | const |
A convenience function that returns the LatLonAltBox of all placemarks in this container.
- Returns
- The GeoDataLatLonAltBox
- See also
- GeoDataLatLonAltBox
Definition at line 53 of file GeoDataContainer.cpp.
GeoDataContainerPrivate * Marble::GeoDataContainer::p | ( | ) | const |
Definition at line 48 of file GeoDataContainer.cpp.
|
virtual |
Serialize the container to a stream.
- Parameters
-
stream the stream
Reimplemented from Marble::GeoDataFeature.
Reimplemented in Marble::GeoDataDocument.
Definition at line 244 of file GeoDataContainer.cpp.
QVector< GeoDataPlacemark * > Marble::GeoDataContainer::placemarkList | ( | ) | const |
A convenience function that returns all placemarks in this container.
- Returns
- A QVector of GeoDataPlacemark
- See also
- GeoDataPlacemark
Definition at line 107 of file GeoDataContainer.cpp.
void Marble::GeoDataContainer::remove | ( | int | index | ) |
Definition at line 173 of file GeoDataContainer.cpp.
int Marble::GeoDataContainer::size | ( | ) | const |
size of the container
Definition at line 179 of file GeoDataContainer.cpp.
|
virtual |
Unserialize the container from a stream.
- Parameters
-
stream the stream
Reimplemented from Marble::GeoDataFeature.
Reimplemented in Marble::GeoDataDocument.
Definition at line 260 of file GeoDataContainer.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:38:55 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.