marble
#include <GeoDataPlacemark.h>
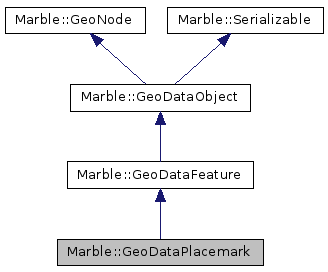
Public Member Functions | |
GeoDataPlacemark () | |
GeoDataPlacemark (const GeoDataPlacemark &placemark) | |
GeoDataPlacemark (const QString &name) | |
~GeoDataPlacemark () | |
qreal | area () const |
GeoDataCoordinates | coordinate (const QDateTime &dateTime=QDateTime(), bool *iconAtCoordinates=0) const |
void | coordinate (qreal &longitude, qreal &latitude, qreal &altitude) const |
const QString | countryCode () const |
GeoDataGeometry * | geometry () const |
const GeoDataLookAt * | lookAt () const |
GeoDataLookAt * | lookAt () |
MARBLE_DEPRECATED (void setCoordinate(const GeoDataPoint &point)) | |
virtual QXmlStreamWriter & | operator<< (QXmlStreamWriter &stream) const |
bool | operator== (const GeoDataPlacemark &other) const |
virtual void | pack (QDataStream &stream) const |
virtual QXmlStreamWriter & | pack (QXmlStreamWriter &stream) const |
qint64 | population () const |
void | setArea (qreal area) |
void | setCoordinate (qreal longitude, qreal latitude, qreal altitude=0, GeoDataCoordinates::Unit _unit=GeoDataCoordinates::Radian) |
void | setCoordinate (const GeoDataCoordinates &coordinate) |
void | setCountryCode (const QString &code) |
void | setGeometry (GeoDataGeometry *entry) |
void | setPopulation (qint64 population) |
void | setState (const QString &state) |
const QString | state () const |
virtual void | unpack (QDataStream &stream) |
![]() | |
GeoDataFeature () | |
GeoDataFeature (const QString &name) | |
GeoDataFeature (const GeoDataFeature &other) | |
virtual | ~GeoDataFeature () |
const GeoDataAbstractView * | abstractView () const |
GeoDataAbstractView * | abstractView () |
QString | address () const |
QString | description () const |
bool | descriptionIsCDATA () const |
virtual void | detach () |
GeoDataExtendedData & | extendedData () const |
EnumFeatureId | featureId () const |
bool | isGloballyVisible () const |
bool | isVisible () const |
QString | name () const |
virtual const char * | nodeType () const |
GeoDataFeature & | operator= (const GeoDataFeature &other) |
QString | phoneNumber () const |
qint64 | popularity () const |
GeoDataRegion & | region () const |
const QString | role () const |
void | setAbstractView (GeoDataAbstractView *abstractView) |
void | setAddress (const QString &value) |
void | setDescription (const QString &value) |
void | setDescriptionCDATA (bool cdata) |
void | setExtendedData (const GeoDataExtendedData &extendedData) |
void | setName (const QString &value) |
void | setPhoneNumber (const QString &value) |
void | setPopularity (qint64 popularity) |
void | setRegion (const GeoDataRegion ®ion) |
void | setRole (const QString &role) |
void | setStyle (GeoDataStyle *style) |
void | setStyleMap (const GeoDataStyleMap *map) |
void | setStyleUrl (const QString &value) |
void | setTimeSpan (const GeoDataTimeSpan &timeSpan) |
void | setTimeStamp (const GeoDataTimeStamp &timeStamp) |
void | setVisible (bool value) |
void | setVisualCategory (GeoDataVisualCategory category) |
void | setZoomLevel (int index) |
const GeoDataStyle * | style () const |
const GeoDataStyleMap * | styleMap () const |
QString | styleUrl () const |
GeoDataTimeSpan & | timeSpan () const |
GeoDataTimeStamp & | timeStamp () const |
GeoDataVisualCategory | visualCategory () const |
int | zoomLevel () const |
![]() | |
GeoDataObject () | |
GeoDataObject (const GeoDataObject &) | |
virtual | ~GeoDataObject () |
int | id () const |
GeoDataObject & | operator= (const GeoDataObject &) |
virtual GeoDataObject * | parent () const |
QString | resolvePath (const QString &relativePath) const |
void | setId (int value) |
virtual void | setParent (GeoDataObject *parent) |
void | setTargetId (int value) |
int | targetId () const |
![]() | |
GeoNode () | |
virtual | ~GeoNode () |
![]() | |
virtual | ~Serializable () |
Detailed Description
a class representing a point of interest on the map
This class represents a point of interest, e.g. a city or a mountain. It is filled with data by the KML or GPX loader and the PlacemarkModel makes use of it.
A Placemark can have an associated geometry which will be rendered to the map along with the placemark's point icon. If you would like to render more than one geometry for any one placemark than use
- See also
- setGeometry() to set add a
- MultiGeometry.
This is more or less only a GeoDataFeature with a geographic position and a country code attached to it. The country code is not provided in a KML file.
Definition at line 54 of file GeoDataPlacemark.h.
Constructor & Destructor Documentation
Marble::GeoDataPlacemark::GeoDataPlacemark | ( | ) |
Create a new placemark.
Definition at line 30 of file GeoDataPlacemark.cpp.
Marble::GeoDataPlacemark::GeoDataPlacemark | ( | const GeoDataPlacemark & | placemark | ) |
Create a new placemark from existing placemark placemark
.
Definition at line 36 of file GeoDataPlacemark.cpp.
|
explicit |
Create a new placemark with the given name
.
Definition at line 42 of file GeoDataPlacemark.cpp.
Marble::GeoDataPlacemark::~GeoDataPlacemark | ( | ) |
Delete the placemark.
Definition at line 49 of file GeoDataPlacemark.cpp.
Member Function Documentation
qreal Marble::GeoDataPlacemark::area | ( | ) | const |
Return the area size of the feature in square km.
FIXME: Once we make Marble more area-aware we need to move this into the GeoDataArea class which will get inherited from GeoDataPlacemark (or GeoDataFeature).
Definition at line 144 of file GeoDataPlacemark.cpp.
GeoDataCoordinates Marble::GeoDataPlacemark::coordinate | ( | const QDateTime & | dateTime = QDateTime() , |
bool * | iconAtCoordinates = 0 |
||
) | const |
Return the coordinates of the placemark at time dateTime
as a GeoDataCoordinates.
The dateTime
parameter should be used if the placemark geometry() is a GeoDataTrack and thus contains several coordinates associated with a date and time.
The iconAtCoordinates
boolean is set to true if an icon should be drawn to represent the placemark at these coordinates as described in https://code.google.com/apis/kml/documentation/kmlreference.html#placemark, it is set to false otherwise.
- See also
- GeoDataTrack::GeoDataTrack
Definition at line 78 of file GeoDataPlacemark.cpp.
void Marble::GeoDataPlacemark::coordinate | ( | qreal & | longitude, |
qreal & | latitude, | ||
qreal & | altitude | ||
) | const |
Return the coordinates of the placemark as longitude
, latitude
and altitude
.
Definition at line 116 of file GeoDataPlacemark.cpp.
const QString Marble::GeoDataPlacemark::countryCode | ( | ) | const |
Return the country code of the placemark.
Definition at line 177 of file GeoDataPlacemark.cpp.
GeoDataGeometry * Marble::GeoDataPlacemark::geometry | ( | ) | const |
The geometry of the GeoDataPlacemark is to be rendered to the marble map along with the icon at the coordinate associated with this Placemark.
- Returns
- a pointer to the current Geometry object
Definition at line 63 of file GeoDataPlacemark.cpp.
const GeoDataLookAt * Marble::GeoDataPlacemark::lookAt | ( | ) | const |
Returns GeoDataLookAt object if lookAt is setup earlier otherwise It will convert GeoDataCoordinates of Placemark to GeoDataLookAt with range equals to altitude of GeoDataCoordinate.
Definition at line 68 of file GeoDataPlacemark.cpp.
GeoDataLookAt * Marble::GeoDataPlacemark::lookAt | ( | ) |
Definition at line 73 of file GeoDataPlacemark.cpp.
Marble::GeoDataPlacemark::MARBLE_DEPRECATED | ( | void | setCoordinateconst GeoDataPoint &point | ) |
|
virtual |
Definition at line 214 of file GeoDataPlacemark.cpp.
bool Marble::GeoDataPlacemark::operator== | ( | const GeoDataPlacemark & | other | ) | const |
comparison operator is implemented slightly different than one would expect.
Only Placemarks that are copies of each other are assumed to be equal.
Definition at line 53 of file GeoDataPlacemark.cpp.
|
virtual |
Serialize the Placemark to a data stream.
This is a binary serialisation and is deserialised using
- See also
- unpack()
- Parameters
-
stream the QDataStream to serialise object to.
Reimplemented from Marble::GeoDataFeature.
Definition at line 188 of file GeoDataPlacemark.cpp.
|
virtual |
Serialise this Placemark to a XML stream writer.
- See also
- QXmlStreamWriter in the Qt documentation for more info. This will output the XML representation of this Placemark. The default XML format is KML, to have other formats supported you need to create a subclass and override this method.
- Parameters
-
stream the XML Stream Reader to output to.
Definition at line 206 of file GeoDataPlacemark.cpp.
qint64 Marble::GeoDataPlacemark::population | ( | ) | const |
Return the population of the placemark.
Definition at line 155 of file GeoDataPlacemark.cpp.
void Marble::GeoDataPlacemark::setArea | ( | qreal | area | ) |
Set the area size of the feature in square km.
Definition at line 149 of file GeoDataPlacemark.cpp.
void Marble::GeoDataPlacemark::setCoordinate | ( | qreal | longitude, |
qreal | latitude, | ||
qreal | altitude = 0 , |
||
GeoDataCoordinates::Unit | _unit = GeoDataCoordinates::Radian |
||
) |
Set the coordinate of the placemark in longitude
and latitude
.
Definition at line 121 of file GeoDataPlacemark.cpp.
void Marble::GeoDataPlacemark::setCoordinate | ( | const GeoDataCoordinates & | coordinate | ) |
Set the coordinate of the placemark with an GeoDataPoint
.
Definition at line 126 of file GeoDataPlacemark.cpp.
void Marble::GeoDataPlacemark::setCountryCode | ( | const QString & | code | ) |
Set the country code
of the placemark.
Definition at line 182 of file GeoDataPlacemark.cpp.
void Marble::GeoDataPlacemark::setGeometry | ( | GeoDataGeometry * | entry | ) |
Sets the current Geometry of this Placemark.
- See also
- geometry() and the class overview for description of the geometry concept. The geometry can be set to any
- GeoDataGeometry like
- GeoDataPoint,
- GeoDataLineString,
- GeoDataLinearRing and
- GeoDataMultiGeometry
Definition at line 136 of file GeoDataPlacemark.cpp.
void Marble::GeoDataPlacemark::setPopulation | ( | qint64 | population | ) |
Sets the population
of the placemark.
- Parameters
-
population the new population value
Definition at line 160 of file GeoDataPlacemark.cpp.
void Marble::GeoDataPlacemark::setState | ( | const QString & | state | ) |
Set the state state
of the placemark.
Definition at line 171 of file GeoDataPlacemark.cpp.
const QString Marble::GeoDataPlacemark::state | ( | ) | const |
Return the state of the placemark.
Definition at line 166 of file GeoDataPlacemark.cpp.
|
virtual |
Deserialize the Placemark from a data stream.
This has the opposite effect from
- See also
- pack()
- Parameters
-
stream the QDataStream to deserialise from.
Reimplemented from Marble::GeoDataFeature.
Definition at line 220 of file GeoDataPlacemark.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:38:56 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.