marble
#include <GeoDataFeature.h>
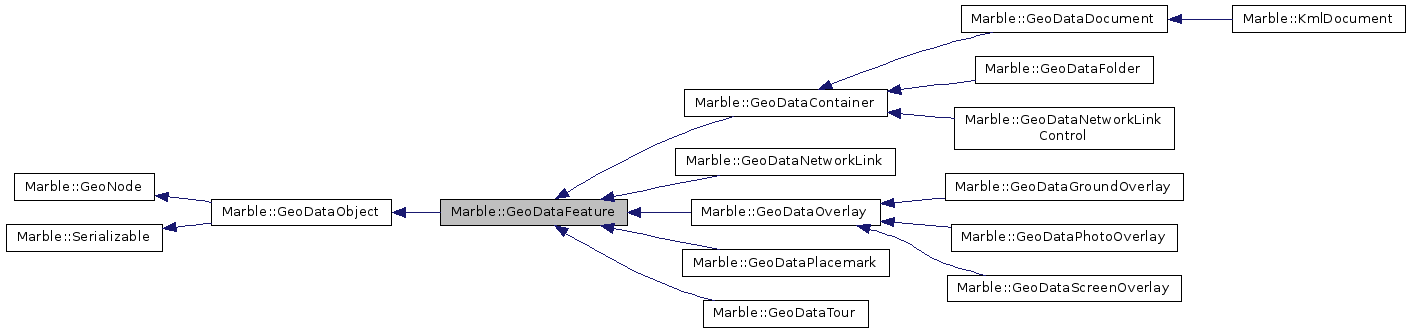
Public Member Functions | |
GeoDataFeature () | |
GeoDataFeature (const QString &name) | |
GeoDataFeature (const GeoDataFeature &other) | |
virtual | ~GeoDataFeature () |
const GeoDataAbstractView * | abstractView () const |
GeoDataAbstractView * | abstractView () |
QString | address () const |
QString | description () const |
bool | descriptionIsCDATA () const |
virtual void | detach () |
GeoDataExtendedData & | extendedData () const |
EnumFeatureId | featureId () const |
bool | isGloballyVisible () const |
bool | isVisible () const |
QString | name () const |
virtual const char * | nodeType () const |
GeoDataFeature & | operator= (const GeoDataFeature &other) |
virtual void | pack (QDataStream &stream) const |
QString | phoneNumber () const |
qint64 | popularity () const |
GeoDataRegion & | region () const |
const QString | role () const |
void | setAbstractView (GeoDataAbstractView *abstractView) |
void | setAddress (const QString &value) |
void | setDescription (const QString &value) |
void | setDescriptionCDATA (bool cdata) |
void | setExtendedData (const GeoDataExtendedData &extendedData) |
void | setName (const QString &value) |
void | setPhoneNumber (const QString &value) |
void | setPopularity (qint64 popularity) |
void | setRegion (const GeoDataRegion ®ion) |
void | setRole (const QString &role) |
void | setStyle (GeoDataStyle *style) |
void | setStyleMap (const GeoDataStyleMap *map) |
void | setStyleUrl (const QString &value) |
void | setTimeSpan (const GeoDataTimeSpan &timeSpan) |
void | setTimeStamp (const GeoDataTimeStamp &timeStamp) |
void | setVisible (bool value) |
void | setVisualCategory (GeoDataVisualCategory category) |
void | setZoomLevel (int index) |
const GeoDataStyle * | style () const |
const GeoDataStyleMap * | styleMap () const |
QString | styleUrl () const |
GeoDataTimeSpan & | timeSpan () const |
GeoDataTimeStamp & | timeStamp () const |
virtual void | unpack (QDataStream &stream) |
GeoDataVisualCategory | visualCategory () const |
int | zoomLevel () const |
![]() | |
GeoDataObject () | |
GeoDataObject (const GeoDataObject &) | |
virtual | ~GeoDataObject () |
int | id () const |
GeoDataObject & | operator= (const GeoDataObject &) |
virtual GeoDataObject * | parent () const |
QString | resolvePath (const QString &relativePath) const |
void | setId (int value) |
virtual void | setParent (GeoDataObject *parent) |
void | setTargetId (int value) |
int | targetId () const |
![]() | |
GeoNode () | |
virtual | ~GeoNode () |
![]() | |
virtual | ~Serializable () |
Static Public Member Functions | |
static QFont | defaultFont () |
static QColor | defaultLabelColor () |
static GeoDataVisualCategory | OsmVisualCategory (const QString &keyValue) |
static void | resetDefaultStyles () |
static void | setDefaultFont (const QFont &font) |
static void | setDefaultLabelColor (const QColor &color) |
Protected Member Functions | |
GeoDataFeature (GeoDataFeaturePrivate *priv) | |
Protected Attributes | |
GeoDataFeaturePrivate * | d |
Detailed Description
A base class for all geodata features.
GeoDataFeature is the base class for most geodata classes that correspond to places on a map. It is never instantiated by itself, but is always used as part of a derived class.
- See also
- GeoDataPlacemark
- GeoDataContainer
Definition at line 55 of file GeoDataFeature.h.
Member Enumeration Documentation
A categorization of a placemark as defined by ...FIXME.
Definition at line 75 of file GeoDataFeature.h.
Constructor & Destructor Documentation
Marble::GeoDataFeature::GeoDataFeature | ( | ) |
Definition at line 42 of file GeoDataFeature.cpp.
|
explicit |
Create a new GeoDataFeature with name
as its name.
Definition at line 55 of file GeoDataFeature.cpp.
Marble::GeoDataFeature::GeoDataFeature | ( | const GeoDataFeature & | other | ) |
Definition at line 48 of file GeoDataFeature.cpp.
|
virtual |
Definition at line 68 of file GeoDataFeature.cpp.
|
protected |
Definition at line 62 of file GeoDataFeature.cpp.
Member Function Documentation
const GeoDataAbstractView * Marble::GeoDataFeature::abstractView | ( | ) | const |
Get the Abstract view of the feature.
Definition at line 535 of file GeoDataFeature.cpp.
GeoDataAbstractView * Marble::GeoDataFeature::abstractView | ( | ) |
Definition at line 540 of file GeoDataFeature.cpp.
QString Marble::GeoDataFeature::address | ( | ) | const |
Return the address of the feature.
Definition at line 491 of file GeoDataFeature.cpp.
|
static |
Definition at line 458 of file GeoDataFeature.cpp.
|
static |
Definition at line 469 of file GeoDataFeature.cpp.
QString Marble::GeoDataFeature::description | ( | ) | const |
Return the text description of the feature.
Definition at line 513 of file GeoDataFeature.cpp.
bool Marble::GeoDataFeature::descriptionIsCDATA | ( | ) | const |
test if the description is CDATA or not CDATA allows for special characters to be included in XML and also allows for other XML formats to be embedded in the XML without interfering with parser namespace.
- Returns
- if the description should be treated as CDATA if the description is a plain string
Definition at line 524 of file GeoDataFeature.cpp.
|
virtual |
Definition at line 734 of file GeoDataFeature.cpp.
GeoDataExtendedData & Marble::GeoDataFeature::extendedData | ( | ) | const |
Return the ExtendedData assigned to the feature.
Definition at line 653 of file GeoDataFeature.cpp.
EnumFeatureId Marble::GeoDataFeature::featureId | ( | ) | const |
Definition at line 99 of file GeoDataFeature.cpp.
bool Marble::GeoDataFeature::isGloballyVisible | ( | ) | const |
Return whether this feature is visible or not in the context of its parenting.
Definition at line 592 of file GeoDataFeature.cpp.
bool Marble::GeoDataFeature::isVisible | ( | ) | const |
Return whether this feature is visible or not.
Definition at line 581 of file GeoDataFeature.cpp.
QString Marble::GeoDataFeature::name | ( | ) | const |
The name of the feature.
The name of the feature should be a short string. It is often shown directly on the map and need therefore not take up much space.
- Returns
- The name of this feature
Definition at line 480 of file GeoDataFeature.cpp.
|
virtual |
Provides type information for downcasting a GeoData.
Implements Marble::GeoDataObject.
Reimplemented in Marble::GeoDataNetworkLinkControl, Marble::GeoDataPhotoOverlay, Marble::GeoDataGroundOverlay, Marble::GeoDataNetworkLink, and Marble::GeoDataScreenOverlay.
Definition at line 94 of file GeoDataFeature.cpp.
GeoDataFeature & Marble::GeoDataFeature::operator= | ( | const GeoDataFeature & | other | ) |
Definition at line 80 of file GeoDataFeature.cpp.
|
static |
Convenience categorization of placemarks for Osm key=value pairs.
Definition at line 785 of file GeoDataFeature.cpp.
|
virtual |
Serialize the contents of the feature to stream
.
Reimplemented from Marble::GeoDataObject.
Reimplemented in Marble::GeoDataPlacemark, Marble::GeoDataDocument, and Marble::GeoDataContainer.
Definition at line 754 of file GeoDataFeature.cpp.
QString Marble::GeoDataFeature::phoneNumber | ( | ) | const |
Return the phone number of the feature.
Definition at line 502 of file GeoDataFeature.cpp.
qint64 Marble::GeoDataFeature::popularity | ( | ) | const |
Return the popularity of the feature.
Definition at line 718 of file GeoDataFeature.cpp.
GeoDataRegion & Marble::GeoDataFeature::region | ( | ) | const |
Return the region assigned to the placemark.
Definition at line 664 of file GeoDataFeature.cpp.
|
static |
Return the label font of the placemark.
Definition at line 729 of file GeoDataFeature.cpp.
const QString Marble::GeoDataFeature::role | ( | ) | const |
Return the role of the placemark.
FIXME: describe roles here!
Definition at line 686 of file GeoDataFeature.cpp.
void Marble::GeoDataFeature::setAbstractView | ( | GeoDataAbstractView * | abstractView | ) |
Set the abstract view of the feature.
Definition at line 545 of file GeoDataFeature.cpp.
void Marble::GeoDataFeature::setAddress | ( | const QString & | value | ) |
Set the address of this feature to value
.
Definition at line 496 of file GeoDataFeature.cpp.
|
static |
Definition at line 463 of file GeoDataFeature.cpp.
|
static |
Definition at line 474 of file GeoDataFeature.cpp.
void Marble::GeoDataFeature::setDescription | ( | const QString & | value | ) |
Set the description of this feature to value
.
Definition at line 518 of file GeoDataFeature.cpp.
void Marble::GeoDataFeature::setDescriptionCDATA | ( | bool | cdata | ) |
Set the description to be CDATA See:
- See also
- descriptionIsCDATA()
Definition at line 529 of file GeoDataFeature.cpp.
void Marble::GeoDataFeature::setExtendedData | ( | const GeoDataExtendedData & | extendedData | ) |
Sets the ExtendedData of the feature.
- Parameters
-
extendedData the new ExtendedData to be used.
Definition at line 658 of file GeoDataFeature.cpp.
void Marble::GeoDataFeature::setName | ( | const QString & | value | ) |
Set a new name for this feature.
- Parameters
-
value the new name
Definition at line 485 of file GeoDataFeature.cpp.
void Marble::GeoDataFeature::setPhoneNumber | ( | const QString & | value | ) |
Set the phone number of this feature to value
.
Definition at line 507 of file GeoDataFeature.cpp.
void Marble::GeoDataFeature::setPopularity | ( | qint64 | popularity | ) |
Sets the popularity
of the feature.
- Parameters
-
popularity the new popularity value
Definition at line 723 of file GeoDataFeature.cpp.
void Marble::GeoDataFeature::setRegion | ( | const GeoDataRegion & | region | ) |
Sets the region of the placemark.
- Parameters
-
region new value for the region
The feature is only shown when the region if active.
Definition at line 669 of file GeoDataFeature.cpp.
void Marble::GeoDataFeature::setRole | ( | const QString & | role | ) |
Sets the role of the placemark.
- Parameters
-
role the new role to be used.
Definition at line 691 of file GeoDataFeature.cpp.
void Marble::GeoDataFeature::setStyle | ( | GeoDataStyle * | style | ) |
Sets the style of the placemark.
- Parameters
-
style the new style to be used.
Definition at line 645 of file GeoDataFeature.cpp.
void Marble::GeoDataFeature::setStyleMap | ( | const GeoDataStyleMap * | map | ) |
Sets the styleMap of the feature.
Definition at line 702 of file GeoDataFeature.cpp.
void Marble::GeoDataFeature::setStyleUrl | ( | const QString & | value | ) |
Set the styleUrl of this feature to value
.
Definition at line 556 of file GeoDataFeature.cpp.
void Marble::GeoDataFeature::setTimeSpan | ( | const GeoDataTimeSpan & | timeSpan | ) |
Set the timespan of the feature.
- Parameters
-
timeSpan new of timespan.
Definition at line 607 of file GeoDataFeature.cpp.
void Marble::GeoDataFeature::setTimeStamp | ( | const GeoDataTimeStamp & | timeStamp | ) |
Set the timestamp of the feature.
- Parameters
-
timeStamp new of the timestamp.
Definition at line 618 of file GeoDataFeature.cpp.
void Marble::GeoDataFeature::setVisible | ( | bool | value | ) |
Set a new value for visibility.
- Parameters
-
value new value for the visibility
This function sets the visibility, i.e. whether this feature should be shown or not. This can be changed either from a GUI or through some action of the program.
Definition at line 586 of file GeoDataFeature.cpp.
void Marble::GeoDataFeature::setVisualCategory | ( | GeoDataFeature::GeoDataVisualCategory | index | ) |
Sets the symbol index
of the placemark.
- Parameters
-
category the new category to be used.
Definition at line 680 of file GeoDataFeature.cpp.
void Marble::GeoDataFeature::setZoomLevel | ( | int | index | ) |
Sets the popularity index
of the placemark.
- Parameters
-
index the new index to be used.
Definition at line 712 of file GeoDataFeature.cpp.
const GeoDataStyle * Marble::GeoDataFeature::style | ( | ) | const |
Return the style assigned to the placemark.
Definition at line 624 of file GeoDataFeature.cpp.
const GeoDataStyleMap * Marble::GeoDataFeature::styleMap | ( | ) | const |
Return a pointer to a GeoDataStyleMap object which represents the styleMap of this feature.
A styleMap is simply a QMap<QString,QString> which can connect two styles with a keyword. This can be used to have a highlighted and a normal style.
- See also
- GeoDataStyleMap
Definition at line 697 of file GeoDataFeature.cpp.
QString Marble::GeoDataFeature::styleUrl | ( | ) | const |
Return the styleUrl of the feature.
Definition at line 551 of file GeoDataFeature.cpp.
GeoDataTimeSpan & Marble::GeoDataFeature::timeSpan | ( | ) | const |
Return the timespan of the feature.
Definition at line 602 of file GeoDataFeature.cpp.
GeoDataTimeStamp & Marble::GeoDataFeature::timeStamp | ( | ) | const |
Return the timestamp of the feature.
Definition at line 613 of file GeoDataFeature.cpp.
|
virtual |
Unserialize the contents of the feature from stream
.
Reimplemented from Marble::GeoDataObject.
Reimplemented in Marble::GeoDataPlacemark, Marble::GeoDataDocument, and Marble::GeoDataContainer.
Definition at line 769 of file GeoDataFeature.cpp.
GeoDataFeature::GeoDataVisualCategory Marble::GeoDataFeature::visualCategory | ( | ) | const |
Return the symbol index of the placemark.
Definition at line 675 of file GeoDataFeature.cpp.
int Marble::GeoDataFeature::zoomLevel | ( | ) | const |
Return the popularity index of the placemark.
The popularity index is a value which describes at which zoom level the placemark will be shown.
Definition at line 707 of file GeoDataFeature.cpp.
Member Data Documentation
|
protected |
Definition at line 484 of file GeoDataFeature.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:38:55 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.