marble
#include <RouteRequest.h>
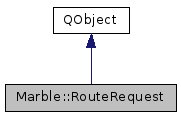
Signals | |
void | positionAdded (int index) |
void | positionChanged (int index, const GeoDataCoordinates &position) |
void | positionRemoved (int index) |
void | routingProfileChanged () |
Public Member Functions | |
RouteRequest (QObject *parent=0) | |
~RouteRequest () | |
void | addVia (const GeoDataCoordinates &position) |
void | append (const GeoDataCoordinates &coordinates, const QString &name=QString()) |
void | append (const GeoDataPlacemark &placemark) |
GeoDataCoordinates | at (int index) const |
void | clear () |
GeoDataCoordinates | destination () const |
void | insert (int index, const GeoDataCoordinates &coordinates, const QString &name=QString()) |
QString | name (int index) const |
GeoDataPlacemark & | operator[] (int index) |
GeoDataPlacemark const & | operator[] (int index) const |
QPixmap | pixmap (int index, int size=-1, int margin=2) const |
void | remove (int index) |
void | reverse () |
RoutingProfile | routingProfile () const |
void | setName (int index, const QString &name) |
void | setPosition (int index, const GeoDataCoordinates &position, const QString &name=QString()) |
void | setRoutingProfile (const RoutingProfile &profile) |
void | setVisited (int index, bool visited) |
int | size () const |
GeoDataCoordinates | source () const |
bool | visited (int index) const |
Detailed Description
Points to be included in a route.
An ordered list of GeoDataCoordinates with change notification and Pixmap access, similar to QAbstractItemModel.
Definition at line 31 of file RouteRequest.h.
Constructor & Destructor Documentation
|
explicit |
Constructor.
Definition at line 115 of file RouteRequest.cpp.
Marble::RouteRequest::~RouteRequest | ( | ) |
Destructor.
Definition at line 121 of file RouteRequest.cpp.
Member Function Documentation
void Marble::RouteRequest::addVia | ( | const GeoDataCoordinates & | position | ) |
Insert a via point.
Order will be chosen such that the via point is not before the start or after the destination. Furthermore the distance between neighboring route points is minimized
- Note
- : This does not trigger an update of the route. It becomes "dirty"
- Todo:
- : Minimizing the distance might not always be what the user wants
Definition at line 240 of file RouteRequest.cpp.
void Marble::RouteRequest::append | ( | const GeoDataCoordinates & | coordinates, |
const QString & | name = QString() |
||
) |
Add the given element to the end.
Definition at line 218 of file RouteRequest.cpp.
void Marble::RouteRequest::append | ( | const GeoDataPlacemark & | placemark | ) |
Definition at line 226 of file RouteRequest.cpp.
GeoDataCoordinates Marble::RouteRequest::at | ( | int | index | ) | const |
Accessor for the n-th position.
Definition at line 149 of file RouteRequest.cpp.
void Marble::RouteRequest::clear | ( | ) |
Remove all elements.
Definition at line 202 of file RouteRequest.cpp.
GeoDataCoordinates Marble::RouteRequest::destination | ( | ) | const |
The last point, or a default constructed if empty.
Definition at line 140 of file RouteRequest.cpp.
void Marble::RouteRequest::insert | ( | int | index, |
const GeoDataCoordinates & | coordinates, | ||
const QString & | name = QString() |
||
) |
Add the given element at the given position.
Definition at line 209 of file RouteRequest.cpp.
QString Marble::RouteRequest::name | ( | int | index | ) | const |
Definition at line 268 of file RouteRequest.cpp.
GeoDataPlacemark & Marble::RouteRequest::operator[] | ( | int | index | ) |
Definition at line 326 of file RouteRequest.cpp.
const GeoDataPlacemark & Marble::RouteRequest::operator[] | ( | int | index | ) | const |
Definition at line 331 of file RouteRequest.cpp.
QPixmap Marble::RouteRequest::pixmap | ( | int | index, |
int | size = -1 , |
||
int | margin = 2 |
||
) | const |
Returns a pixmap which indicates the position of the element.
Definition at line 154 of file RouteRequest.cpp.
|
signal |
An element was added at the given position.
|
signal |
The value of the n-th element was changed.
|
signal |
The element at the given position was removed.
void Marble::RouteRequest::remove | ( | int | index | ) |
Remove the element at the given position.
Definition at line 232 of file RouteRequest.cpp.
void Marble::RouteRequest::reverse | ( | ) |
Definition at line 304 of file RouteRequest.cpp.
RoutingProfile Marble::RouteRequest::routingProfile | ( | ) | const |
Definition at line 321 of file RouteRequest.cpp.
|
signal |
The routing profile was changed.
void Marble::RouteRequest::setName | ( | int | index, |
const QString & | name | ||
) |
Definition at line 261 of file RouteRequest.cpp.
void Marble::RouteRequest::setPosition | ( | int | index, |
const GeoDataCoordinates & | position, | ||
const QString & | name = QString() |
||
) |
Change the value of the element at the given position.
Definition at line 249 of file RouteRequest.cpp.
void Marble::RouteRequest::setRoutingProfile | ( | const RoutingProfile & | profile | ) |
Definition at line 315 of file RouteRequest.cpp.
void Marble::RouteRequest::setVisited | ( | int | index, |
bool | visited | ||
) |
Definition at line 277 of file RouteRequest.cpp.
int Marble::RouteRequest::size | ( | ) | const |
Number of points in the route.
Definition at line 126 of file RouteRequest.cpp.
GeoDataCoordinates Marble::RouteRequest::source | ( | ) | const |
The first point, or a default constructed if empty.
Definition at line 131 of file RouteRequest.cpp.
bool Marble::RouteRequest::visited | ( | int | index | ) | const |
Definition at line 293 of file RouteRequest.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:38:57 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.