step/stepcore
#include <rigidbody.h>
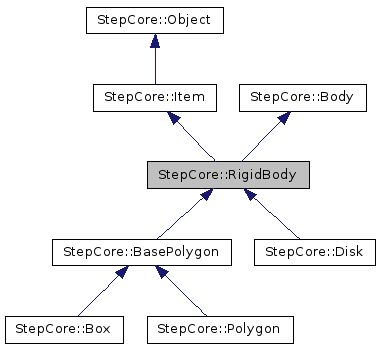
Public Types | |
enum | { PositionOffset = 0, AngleOffset = 2 } |
Public Member Functions | |
RigidBody (Vector2d position=Vector2d::Zero(), double angle=0, Vector2d velocity=Vector2d::Zero(), double angularVelocity=0, double mass=1, double inertia=1) | |
Vector2d | acceleration () const |
void | addForce (const double *force, const double *forceVariance) |
double | angle () const |
double | angularAcceleration () const |
double | angularMomentum () const |
double | angularVelocity () const |
void | applyForce (const Vector2d &force, const Vector2d &position) |
void | applyTorque (double torque) |
const Vector2d & | force () const |
void | getAccelerations (double *acceleration, double *accelerationVariance) |
void | getInverseMass (VectorXd *inverseMass, DynSparseRowMatrix *variance, int offset) |
void | getVariables (double *position, double *velocity, double *positionVariance, double *velocityVariance) |
double | inertia () const |
double | kineticEnergy () const |
double | mass () const |
Vector2d | momentum () const |
Vector2d | pointLocalToWorld (const Vector2d &p) const |
Vector2d | pointWorldToLocal (const Vector2d &p) const |
const Vector2d & | position () const |
void | resetForce (bool resetVariance) |
RigidBodyErrors * | rigidBodyErrors () |
void | setAngle (double angle) |
void | setAngularMomentum (double angularMomentum) |
void | setAngularVelocity (double angularVelocity) |
void | setForce (const Vector2d &force) |
void | setInertia (double inertia) |
void | setKineticEnergy (double kineticEnergy) |
void | setMass (double mass) |
void | setMomentum (const Vector2d &momentum) |
void | setPosition (const Vector2d &position) |
void | setTorque (double torque) |
void | setVariables (const double *position, const double *velocity, const double *positionVariance, const double *velocityVariance) |
void | setVelocity (const Vector2d &velocity) |
double | torque () const |
int | variablesCount () |
Vector2d | vectorLocalToWorld (const Vector2d &v) const |
Vector2d | vectorWorldToLocal (const Vector2d &v) const |
const Vector2d & | velocity () const |
Vector2d | velocityLocal (const Vector2d &localPoint) const |
Vector2d | velocityWorld (const Vector2d &worldPoint) const |
![]() | |
Item (const QString &name=QString()) | |
Item (const Item &item) | |
virtual | ~Item () |
Color | color () const |
void | deleteObjectErrors () |
ItemGroup * | group () const |
ObjectErrors * | objectErrors () |
Item & | operator= (const Item &item) |
void | setColor (Color color) |
virtual void | setGroup (ItemGroup *group) |
virtual void | setWorld (World *world) |
ObjectErrors * | tryGetObjectErrors () const |
World * | world () const |
virtual void | worldItemRemoved (Item *item STEPCORE_UNUSED) |
![]() | |
Object (const QString &name=QString()) | |
virtual | ~Object () |
const QString & | name () const |
void | setName (const QString &name) |
![]() | |
Body () | |
virtual | ~Body () |
int | variablesOffset () const |
Protected Member Functions | |
ObjectErrors * | createObjectErrors () |
Protected Attributes | |
double | _angle |
double | _angularVelocity |
Vector2d | _force |
double | _inertia |
double | _mass |
Vector2d | _position |
double | _torque |
Vector2d | _velocity |
![]() | |
QString | _name |
Detailed Description
Rigid body.
Definition at line 144 of file rigidbody.h.
Member Enumeration Documentation
anonymous enum |
Enumerator | |
---|---|
PositionOffset |
Offset of body position in variables array. |
AngleOffset |
Offset of body angle in variables array. |
Definition at line 149 of file rigidbody.h.
Constructor & Destructor Documentation
|
explicit |
Constructs RigidBody.
Definition at line 163 of file rigidbody.cc.
Member Function Documentation
|
inline |
Get acceleration of the center of mass of the body.
Definition at line 184 of file rigidbody.h.
|
virtual |
Add force and (possibly) its variance to force accomulator.
- Note
- This function is used only by generic constraints handling code, force objects should use body-specific functions.
Implements StepCore::Body.
Definition at line 289 of file rigidbody.cc.
|
inline |
Get angle of the body.
Definition at line 165 of file rigidbody.h.
|
inline |
Get angular acceleration of the body.
Definition at line 187 of file rigidbody.h.
|
inline |
Get angular momentum of the body.
Definition at line 223 of file rigidbody.h.
|
inline |
Get angular velocity of the body.
Definition at line 179 of file rigidbody.h.
Apply force (and torque) to the body at given position (in World coordinates)
Definition at line 170 of file rigidbody.cc.
|
inline |
Apply torque (but no force) to the body.
Definition at line 205 of file rigidbody.h.
|
inlineprotectedvirtual |
Creates specific ObjectErrors-derived class (to be reimplemented in derived classes)
Reimplemented from StepCore::Item.
Definition at line 265 of file rigidbody.h.
|
inline |
Get force that acts upon the body.
Definition at line 190 of file rigidbody.h.
|
virtual |
Copy acceleration (forces left-multiplied by inverse mass) and (possibly) its variances to arrays.
Variances should only be copied if accelerationVariance != NULL.
Implements StepCore::Body.
Definition at line 273 of file rigidbody.cc.
|
virtual |
Get inverse mass and (possibly) its variance matrixes.
Variance should only be copied of variance != NULL.
Implements StepCore::Body.
Definition at line 313 of file rigidbody.cc.
|
virtual |
Copy positions, velocities and (possibly) its variances to arrays.
Variances should only be copied if positionVariance != NULL.
Implements StepCore::Body.
Definition at line 233 of file rigidbody.cc.
|
inline |
Get inertia "tensor" of the body.
Definition at line 213 of file rigidbody.h.
|
inline |
Get kinetic energy of the body.
Definition at line 229 of file rigidbody.h.
|
inline |
Get mass of the body.
Definition at line 208 of file rigidbody.h.
|
inline |
Get momentum of the body.
Definition at line 218 of file rigidbody.h.
Translate local coordinates on body to world coordinates.
Definition at line 201 of file rigidbody.cc.
Translate world coordinates to local coordinates on body.
Definition at line 209 of file rigidbody.cc.
|
inline |
Get position of the center of mass of the body.
Definition at line 160 of file rigidbody.h.
|
virtual |
Reset force accomulator and (possibly) its variance to zero.
Variance should only be reseted if resetVariance == true.
Implements StepCore::Body.
Definition at line 302 of file rigidbody.cc.
|
inline |
Get (and possibly create) RigidBodyErrors object.
Definition at line 261 of file rigidbody.h.
|
inline |
Set angle of the body.
Definition at line 167 of file rigidbody.h.
|
inline |
Set angular momentum of the body (will modify only angularVelocity)
Definition at line 225 of file rigidbody.h.
|
inline |
Set angular velocity of the body.
Definition at line 181 of file rigidbody.h.
|
inline |
Set force that acts upon the body.
Definition at line 192 of file rigidbody.h.
|
inline |
Set inertia "tensor" of the body.
Definition at line 215 of file rigidbody.h.
void StepCore::RigidBody::setKineticEnergy | ( | double | kineticEnergy | ) |
Set kinetic energy of the body (will modify only velocity and (possibly) angularVelocity)
Definition at line 329 of file rigidbody.cc.
|
inline |
Set mass of the body.
Definition at line 210 of file rigidbody.h.
|
inline |
Set momentum of the body (will modify only velocity)
Definition at line 220 of file rigidbody.h.
|
inline |
Set position of the center of mass of the body.
Definition at line 162 of file rigidbody.h.
|
inline |
Set torque that acts upon the body.
Definition at line 197 of file rigidbody.h.
|
virtual |
Set positions, velocities and (possibly) its variances using values in arrays and also reset accelerations and its variances.
Variances should only be copied and reseted if positionVariance != NULL.
Implements StepCore::Body.
Definition at line 250 of file rigidbody.cc.
|
inline |
Set velocity of the particle.
Definition at line 172 of file rigidbody.h.
|
inline |
Get torque that acts upon the body.
Definition at line 195 of file rigidbody.h.
|
inlinevirtual |
Get count of dynamic variables (not including velocities)
Implements StepCore::Body.
Definition at line 250 of file rigidbody.h.
Translate local vector on body to world vector.
Definition at line 217 of file rigidbody.cc.
Translate world vector to local vector on body.
Definition at line 225 of file rigidbody.cc.
|
inline |
Get velocity of the center of mass of the body.
Definition at line 170 of file rigidbody.h.
Get velocity of given (local) point on the body.
Definition at line 195 of file rigidbody.cc.
Get velocity of given (world) point on the body.
Definition at line 189 of file rigidbody.cc.
Member Data Documentation
|
protected |
Definition at line 268 of file rigidbody.h.
|
protected |
Definition at line 271 of file rigidbody.h.
|
protected |
Definition at line 273 of file rigidbody.h.
|
protected |
Definition at line 277 of file rigidbody.h.
|
protected |
Definition at line 276 of file rigidbody.h.
|
protected |
Definition at line 267 of file rigidbody.h.
|
protected |
Definition at line 274 of file rigidbody.h.
|
protected |
Definition at line 270 of file rigidbody.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:43:06 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.