knode
#include <kncollectionview.h>
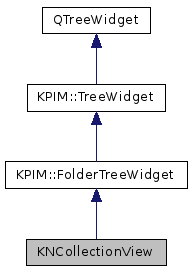
Public Slots | |
void | activateFolder (KNFolder::Ptr f) |
void | addAccount (KNNntpAccount::Ptr a) |
void | addFolder (KNFolder::Ptr f) |
void | addGroup (KNGroup::Ptr g) |
void | addPendingFolders () |
void | decCurrentFolder () |
void | incCurrentFolder () |
void | nextGroup () |
void | prevGroup () |
void | reload () |
void | reloadAccounts () |
void | reloadFolders () |
void | removeAccount (KNNntpAccount::Ptr a) |
void | removeFolder (KNFolder::Ptr f) |
void | removeGroup (KNGroup::Ptr g) |
void | selectCurrentFolder () |
void | updateAccount (KNNntpAccount::Ptr a) |
void | updateFolder (KNFolder::Ptr f) |
void | updateGroup (KNGroup::Ptr g) |
Signals | |
void | contextMenu (QTreeWidgetItem *item, const QPoint &position) |
![]() | |
void | renamed (QTreeWidgetItem *item) |
![]() | |
void | columnVisibilityChanged (int logicalIndex) |
Public Member Functions | |
KNCollectionView (QWidget *parent) | |
~KNCollectionView () | |
void | readConfig () |
void | setActive (QTreeWidgetItem *item) |
void | writeConfig () |
![]() | |
FolderTreeWidget (QWidget *parent, const char *name=0) | |
int | addDataSizeColumn (const QString &headerLabel) |
int | addLabelColumn (const QString &headerLabel) |
int | addTotalColumn (const QString &headerLabel) |
int | addUnreadColumn (const QString &headerLabel) |
const QColor & | closeToQuotaWarningColor () const |
int | dataSizeColumnIndex () const |
bool | dataSizeColumnVisible () const |
int | labelColumnIndex () const |
bool | labelColumnVisible () const |
void | setCloseToQuotaWarningColor (const QColor &clr) |
void | setUnreadCountColor (const QColor &clr) |
int | totalColumnIndex () const |
bool | totalColumnVisible () const |
int | unreadColumnIndex () const |
bool | unreadColumnVisible () const |
const QColor & | unreadCountColor () const |
void | updateColumnForItem (FolderTreeWidgetItem *item, int columnIndex) |
![]() | |
TreeWidget (QWidget *parent, const char *name=0) | |
int | addColumn (const QString &label, int headerAlignment=Qt::AlignLeft|Qt::AlignVCenter) |
virtual bool | fillHeaderContextMenu (KMenu *menu, const QPoint &clickPoint) |
QTreeWidgetItem * | firstItem () const |
bool | isColumnHidden (int logicalIndex) const |
QTreeWidgetItem * | lastItem () const |
bool | manualColumnHidingEnabled () const |
bool | restoreLayout (KConfigGroup &group, const QString &keyName=QString()) |
bool | restoreLayout (KConfig *config, const QString &groupName, const QString &keyName=QString()) |
bool | saveLayout (KConfigGroup &group, const QString &keyName=QString()) const |
bool | saveLayout (KConfig *config, const QString &groupName, const QString &keyName=QString()) const |
void | setColumnHidden (int logicalIndex, bool hide) |
bool | setColumnText (int columnIndex, const QString &label) |
void | setManualColumnHidingEnabled (bool enable) |
void | toggleColumn (int logicalIndex) |
Protected Member Functions | |
virtual void | contextMenuEvent (QContextMenuEvent *event) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
void | handleDragNDropEvent (QDropEvent *event, bool enforceDrop) |
virtual QStringList | mimeTypes () const |
virtual void | paintEvent (QPaintEvent *event) |
virtual void | startDrag (Qt::DropActions supportedActions) |
![]() | |
virtual void | changeEvent (QEvent *e) |
Detailed Description
The group/folder tree.
Definition at line 30 of file kncollectionview.h.
Constructor & Destructor Documentation
|
explicit |
Definition at line 36 of file kncollectionview.cpp.
KNCollectionView::~KNCollectionView | ( | ) |
Definition at line 76 of file kncollectionview.cpp.
Member Function Documentation
|
slot |
Definition at line 257 of file kncollectionview.cpp.
|
slot |
Definition at line 109 of file kncollectionview.cpp.
|
slot |
Definition at line 188 of file kncollectionview.cpp.
|
slot |
Definition at line 158 of file kncollectionview.cpp.
|
slot |
Definition at line 242 of file kncollectionview.cpp.
|
signal |
This signal is emitted when a context menu should be displayed for item
as global position position
.
|
protectedvirtual |
Emits the signal contextMenu() to display a context menu on items of the view.
Reimplemented from QAbstractScrollArea.
Definition at line 333 of file kncollectionview.cpp.
|
slot |
Definition at line 301 of file kncollectionview.cpp.
|
protectedvirtual |
Reimplement to only accept MIME types which are accepted in dropEvent.
Definition at line 359 of file kncollectionview.cpp.
|
protectedvirtual |
Reimplemented to hide the drop indicator when the drag leave the view.
Definition at line 368 of file kncollectionview.cpp.
|
protectedvirtual |
Reimplemented to accept the event
only when the target folder can be dropped articles or folders.
Definition at line 376 of file kncollectionview.cpp.
|
protectedvirtual |
Handles actual droping of articles or folder.
Definition at line 384 of file kncollectionview.cpp.
|
protected |
Called by dragMoveEvent() and dropEvent().
- Parameters
-
event The drop/drag-move event. enforceDrop If true the actual move of folder or move/copy of articles happens.
Definition at line 392 of file kncollectionview.cpp.
|
slot |
Definition at line 313 of file kncollectionview.cpp.
|
protectedvirtual |
Returns the "x-knode-drag/folder" mime-type for drag&droping of folders within this view.
Definition at line 450 of file kncollectionview.cpp.
|
slot |
Definition at line 287 of file kncollectionview.cpp.
|
protectedvirtual |
Definition at line 343 of file kncollectionview.cpp.
|
slot |
Definition at line 294 of file kncollectionview.cpp.
void KNCollectionView::readConfig | ( | ) |
Definition at line 95 of file kncollectionview.cpp.
|
slot |
Definition at line 270 of file kncollectionview.cpp.
|
slot |
Definition at line 147 of file kncollectionview.cpp.
|
slot |
Definition at line 232 of file kncollectionview.cpp.
|
slot |
Definition at line 126 of file kncollectionview.cpp.
|
slot |
Definition at line 216 of file kncollectionview.cpp.
|
slot |
Definition at line 170 of file kncollectionview.cpp.
|
slot |
Definition at line 325 of file kncollectionview.cpp.
void KNCollectionView::setActive | ( | QTreeWidgetItem * | item | ) |
Selects item
and set it the current item.
Definition at line 276 of file kncollectionview.cpp.
|
protectedvirtual |
Reimplemented to perform the actual drag operations of folders.
This takes care of showing a nice drag icon/cursor instead of the default that consists of the whole item widget (cells of label & counts).
- Parameters
-
supportedActions unused parameter.
Definition at line 457 of file kncollectionview.cpp.
|
slot |
Definition at line 141 of file kncollectionview.cpp.
|
slot |
Definition at line 264 of file kncollectionview.cpp.
|
slot |
Definition at line 181 of file kncollectionview.cpp.
void KNCollectionView::writeConfig | ( | ) |
Definition at line 102 of file kncollectionview.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:58:37 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.