Marble::GeoDataPolygon
#include <GeoDataPolygon.h>
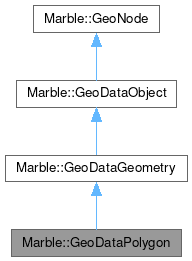
Public Member Functions | |
GeoDataPolygon (const GeoDataGeometry &other) | |
GeoDataPolygon (TessellationFlags f=NoTessellation) | |
~GeoDataPolygon () override | |
void | appendInnerBoundary (const GeoDataLinearRing &boundary) |
virtual bool | contains (const GeoDataCoordinates &coordinates) const |
GeoDataGeometry * | copy () const override |
EnumGeometryId | geometryId () const override |
QList< GeoDataLinearRing > & | innerBoundaries () |
const QList< GeoDataLinearRing > & | innerBoundaries () const |
virtual bool | isClosed () const |
const GeoDataLatLonAltBox & | latLonAltBox () const override |
const char * | nodeType () const override |
bool | operator!= (const GeoDataPolygon &other) const |
bool | operator== (const GeoDataPolygon &other) const |
GeoDataLinearRing & | outerBoundary () |
const GeoDataLinearRing & | outerBoundary () const |
void | pack (QDataStream &stream) const override |
int | renderOrder () const |
void | setOuterBoundary (const GeoDataLinearRing &boundary) |
void | setRenderOrder (int) |
void | setTessellate (bool tessellate) |
void | setTessellationFlags (TessellationFlags f) |
bool | tessellate () const |
TessellationFlags | tessellationFlags () const |
void | unpack (QDataStream &stream) override |
![]() | |
AltitudeMode | altitudeMode () const |
void | detach () |
bool | extrude () const |
bool | operator!= (const GeoDataGeometry &other) const |
bool | operator== (const GeoDataGeometry &other) const |
void | pack (QDataStream &stream) const override |
void | setAltitudeMode (const AltitudeMode altitudeMode) |
void | setExtrude (bool extrude) |
void | unpack (QDataStream &stream) override |
![]() | |
GeoDataObject (const GeoDataObject &) | |
QString | id () const |
GeoDataObject & | operator= (const GeoDataObject &) |
void | pack (QDataStream &stream) const override |
GeoDataObject * | parent () |
const GeoDataObject * | parent () const |
QString | resolvePath (const QString &relativePath) const |
void | setId (const QString &value) |
void | setParent (GeoDataObject *parent) |
void | setTargetId (const QString &value) |
QString | targetId () const |
void | unpack (QDataStream &steam) override |
![]() |
Additional Inherited Members | |
![]() | |
GeoDataGeometry (const GeoDataGeometry &other) | |
GeoDataGeometry (GeoDataGeometryPrivate *priv) | |
bool | equals (const GeoDataGeometry &other) const |
virtual bool | equals (const GeoDataObject &other) const |
GeoDataGeometry & | operator= (const GeoDataGeometry &other) |
![]() | |
![]() | |
GeoDataGeometryPrivate * | d_ptr |
Detailed Description
A polygon that can have "holes".
GeoDataPolygon is a tool class that implements the Polygon tag/class of the Open Geospatial Consortium standard KML 2.2.
GeoDataPolygon extends GeoDataGeometry to store and edit Polygons.
In the QPainter API "pure" Polygons would represent polygons with "holes" inside. However QPolygon doesn't provide this feature directly.
Whenever a Polygon is painted GeoDataLineStyle should be used to assign a color and line width.
The polygon consists of
- a single outer boundary and
- optionally a set of inner boundaries.
All boundaries are LinearRings.
The boundaries of a GeoDataPolygon consist of several (geodetic) nodes which are each connected through line segments. The nodes are stored as GeoDataCoordinates objects.
The API which provides access to the nodes is similar to the API of QList.
GeoDataPolygon allows Polygons to be tessellated in order to make them follow the terrain and the curvature of the earth. The tessellation options allow for different ways of visualization:
- Not tessellated: A Polygon that connects each two nodes directly and straight in screen coordinate space.
- A tessellated line: Each line segment is bent so that the Polygon follows the curvature of the earth and its terrain. A tessellated line segment connects two nodes at the shortest possible distance ("along great circles").
- A tessellated line that follows latitude circles whenever possible: In this case Latitude circles are followed as soon as two subsequent nodes have exactly the same amount of latitude. In all other places the line segments follow great circles.
Some convenience methods have been added that allow to calculate the geodesic bounding box or the length of a Polygon.
- See also
- GeoDataLinearRing
Definition at line 75 of file GeoDataPolygon.h.
Constructor & Destructor Documentation
◆ GeoDataPolygon() [1/2]
|
explicit |
Creates a new Polygon.
Definition at line 19 of file GeoDataPolygon.cpp.
◆ GeoDataPolygon() [2/2]
|
explicit |
Creates a Polygon from an existing geometry object.
Definition at line 25 of file GeoDataPolygon.cpp.
◆ ~GeoDataPolygon()
|
override |
Destroys a Polygon.
Definition at line 31 of file GeoDataPolygon.cpp.
Member Function Documentation
◆ appendInnerBoundary()
void Marble::GeoDataPolygon::appendInnerBoundary | ( | const GeoDataLinearRing & | boundary | ) |
Appends a given LinearRing as an inner boundary of the Polygon.
- See also
- GeoDataLinearRing
Definition at line 166 of file GeoDataPolygon.cpp.
◆ contains()
|
virtual |
Returns whether the given coordinates lie within the polygon.
- Returns
true
if the coordinates lie within the polygon (and not in its holes), false otherwise.
Definition at line 233 of file GeoDataPolygon.cpp.
◆ copy()
|
overridevirtual |
Implements Marble::GeoDataGeometry.
Definition at line 48 of file GeoDataPolygon.cpp.
◆ geometryId()
|
overridevirtual |
Implements Marble::GeoDataGeometry.
Definition at line 43 of file GeoDataPolygon.cpp.
◆ innerBoundaries() [1/2]
QList< GeoDataLinearRing > & Marble::GeoDataPolygon::innerBoundaries | ( | ) |
Returns a set of inner boundaries which are represented as LinearRings.
- See also
- GeoDataLinearRing
Definition at line 152 of file GeoDataPolygon.cpp.
◆ innerBoundaries() [2/2]
const QList< GeoDataLinearRing > & Marble::GeoDataPolygon::innerBoundaries | ( | ) | const |
Returns a set of inner boundaries which are represented as LinearRings.
- See also
- GeoDataLinearRing
Definition at line 160 of file GeoDataPolygon.cpp.
◆ isClosed()
|
virtual |
Returns whether a Polygon is a closed polygon.
- Returns
true
for a Polygon.
Definition at line 83 of file GeoDataPolygon.cpp.
◆ latLonAltBox()
|
overridevirtual |
Returns the smallest latLonAltBox that contains the Polygon.
- See also
- GeoDataLatLonAltBox
Reimplemented from Marble::GeoDataGeometry.
Definition at line 124 of file GeoDataPolygon.cpp.
◆ nodeType()
|
overridevirtual |
Provides type information for downcasting a GeoNode.
Implements Marble::GeoNode.
Definition at line 38 of file GeoDataPolygon.cpp.
◆ operator!=()
bool Marble::GeoDataPolygon::operator!= | ( | const GeoDataPolygon & | other | ) | const |
Definition at line 78 of file GeoDataPolygon.cpp.
◆ operator==()
bool Marble::GeoDataPolygon::operator== | ( | const GeoDataPolygon & | other | ) | const |
Returns true/false depending on whether this and other are/are not equal.
Definition at line 53 of file GeoDataPolygon.cpp.
◆ outerBoundary() [1/2]
GeoDataLinearRing & Marble::GeoDataPolygon::outerBoundary | ( | ) |
Returns the outer boundary that is represented as a LinearRing.
- See also
- GeoDataLinearRing
Definition at line 130 of file GeoDataPolygon.cpp.
◆ outerBoundary() [2/2]
const GeoDataLinearRing & Marble::GeoDataPolygon::outerBoundary | ( | ) | const |
Returns the outer boundary that is represented as a LinearRing.
- See also
- GeoDataLinearRing
Definition at line 138 of file GeoDataPolygon.cpp.
◆ pack()
|
override |
Serialize the Polygon to a stream.
- Parameters
-
stream the stream.
Definition at line 188 of file GeoDataPolygon.cpp.
◆ renderOrder()
int Marble::GeoDataPolygon::renderOrder | ( | ) | const |
Definition at line 182 of file GeoDataPolygon.cpp.
◆ setOuterBoundary()
void Marble::GeoDataPolygon::setOuterBoundary | ( | const GeoDataLinearRing & | boundary | ) |
Sets the given LinearRing as an outer boundary of the Polygon.
- See also
- GeoDataLinearRing
Definition at line 144 of file GeoDataPolygon.cpp.
◆ setRenderOrder()
void Marble::GeoDataPolygon::setRenderOrder | ( | int | renderOrder | ) |
Definition at line 174 of file GeoDataPolygon.cpp.
◆ setTessellate()
void Marble::GeoDataPolygon::setTessellate | ( | bool | tessellate | ) |
Sets the tessellation property for the Polygon.
If tessellate is true
then the Polygon's line segments are bent and follow the earth's surface and terrain along great circles. If tessellate is false
then the Polygon's line segments are rendered as straight lines in screen coordinate space.
Definition at line 94 of file GeoDataPolygon.cpp.
◆ setTessellationFlags()
void Marble::GeoDataPolygon::setTessellationFlags | ( | TessellationFlags | f | ) |
Sets the given tessellation flags for a Polygon.
Definition at line 116 of file GeoDataPolygon.cpp.
◆ tessellate()
bool Marble::GeoDataPolygon::tessellate | ( | ) | const |
Returns whether the Polygon follows the earth's surface.
- Returns
true
if the Polygon's line segments follow the earth's surface and terrain along great circles.
Definition at line 88 of file GeoDataPolygon.cpp.
◆ tessellationFlags()
TessellationFlags Marble::GeoDataPolygon::tessellationFlags | ( | ) | const |
Returns the tessellation flags for a Polygon.
Definition at line 110 of file GeoDataPolygon.cpp.
◆ unpack()
|
override |
Unserialize the Polygon from a stream.
- Parameters
-
stream the stream.
Definition at line 206 of file GeoDataPolygon.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Mon Nov 4 2024 16:37:04 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.