Marble::GeoDataTrack
#include <GeoDataTrack.h>
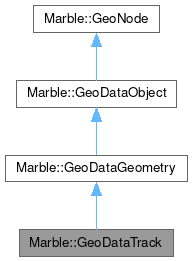
Public Member Functions | |
GeoDataTrack (const GeoDataTrack &other) | |
void | addPoint (const QDateTime &when, const GeoDataCoordinates &coord) |
void | appendAltitude (qreal altitude) |
void | appendCoordinates (const GeoDataCoordinates &coord) |
void | appendWhen (const QDateTime &when) |
void | clear () |
GeoDataCoordinates | coordinatesAt (const QDateTime &when) const |
GeoDataCoordinates | coordinatesAt (int index) const |
QList< GeoDataCoordinates > | coordinatesList () const |
GeoDataGeometry * | copy () const override |
GeoDataExtendedData & | extendedData () |
const GeoDataExtendedData & | extendedData () const |
QDateTime | firstWhen () const |
EnumGeometryId | geometryId () const override |
bool | interpolate () const |
QDateTime | lastWhen () const |
const GeoDataLatLonAltBox & | latLonAltBox () const override |
const GeoDataLineString * | lineString () const |
const char * | nodeType () const override |
bool | operator!= (const GeoDataTrack &other) const |
GeoDataTrack & | operator= (const GeoDataTrack &other) |
bool | operator== (const GeoDataTrack &other) const |
void | pack (QDataStream &stream) const override |
void | removeAfter (const QDateTime &when) |
void | removeBefore (const QDateTime &when) |
void | setExtendedData (const GeoDataExtendedData &extendedData) |
void | setInterpolate (bool on) |
int | size () const |
void | unpack (QDataStream &stream) override |
QList< QDateTime > | whenList () const |
![]() | |
AltitudeMode | altitudeMode () const |
void | detach () |
bool | extrude () const |
bool | operator!= (const GeoDataGeometry &other) const |
bool | operator== (const GeoDataGeometry &other) const |
void | pack (QDataStream &stream) const override |
void | setAltitudeMode (const AltitudeMode altitudeMode) |
void | setExtrude (bool extrude) |
void | unpack (QDataStream &stream) override |
![]() | |
GeoDataObject (const GeoDataObject &) | |
QString | id () const |
GeoDataObject & | operator= (const GeoDataObject &) |
void | pack (QDataStream &stream) const override |
GeoDataObject * | parent () |
const GeoDataObject * | parent () const |
QString | resolvePath (const QString &relativePath) const |
void | setId (const QString &value) |
void | setParent (GeoDataObject *parent) |
void | setTargetId (const QString &value) |
QString | targetId () const |
void | unpack (QDataStream &steam) override |
Additional Inherited Members | |
![]() | |
GeoDataGeometry (const GeoDataGeometry &other) | |
GeoDataGeometry (GeoDataGeometryPrivate *priv) | |
bool | equals (const GeoDataGeometry &other) const |
virtual bool | equals (const GeoDataObject &other) const |
GeoDataGeometry & | operator= (const GeoDataGeometry &other) |
![]() | |
GeoDataGeometryPrivate * | d_ptr |
Detailed Description
A geometry for tracking objects made of (time, coordinates) pairs.
GeoDataTrack implements the Track tag defined in Google's extension of the Open Geospatial Consortium standard KML 2.2 at https://developers.google.com/kml/documentation/kmlreference#gxtrack .
A track is made of points, each point has a coordinates and a time value associated with the coordinates. New points can be added using the addPoint() method. The coordinates of the tracked object at a particular time can be found using coordinatesAt(), you can specify if interpolation should be used using the setInterpolate() function.
By default, a LineString that passes through every coordinates in the track is drawn. You can customize it by changing the GeoDataLineStyle, for example if the GeoDataTrack is the geometry of feature, you can disable the line drawing with:
For convenience, the methods appendCoordinates() and appendWhen() are provided. They let you add points by specifying their coordinates and time value separately. When N calls to one of these methods are followed by N calls to the other, the first coordinates will be matched with the first time value, the second coordinates with the second time value, etc. This follows the way "coord" and "when" tags inside the Track tag should be parsed.
Definition at line 51 of file GeoDataTrack.h.
Constructor & Destructor Documentation
◆ GeoDataTrack()
Marble::GeoDataTrack::GeoDataTrack | ( | ) |
Definition at line 56 of file GeoDataTrack.cpp.
Member Function Documentation
◆ addPoint()
void Marble::GeoDataTrack::addPoint | ( | const QDateTime & | when, |
const GeoDataCoordinates & | coord ) |
Add a new point with coordinates coord
associated with the time value when
.
Definition at line 211 of file GeoDataTrack.cpp.
◆ appendAltitude()
void Marble::GeoDataTrack::appendAltitude | ( | qreal | altitude | ) |
Add altitude information to the last appended coordinates.
Definition at line 239 of file GeoDataTrack.cpp.
◆ appendCoordinates()
void Marble::GeoDataTrack::appendCoordinates | ( | const GeoDataCoordinates & | coord | ) |
Add the coordinates part for a new point.
See this class description for more information.
- See also
- appendWhen
Definition at line 229 of file GeoDataTrack.cpp.
◆ appendWhen()
void Marble::GeoDataTrack::appendWhen | ( | const QDateTime & | when | ) |
Add the time value part for a new point.
See this class description for more information.
- See also
- appendCoordinates
Definition at line 254 of file GeoDataTrack.cpp.
◆ clear()
void Marble::GeoDataTrack::clear | ( | ) |
Remove all the points contained in the track.
Definition at line 262 of file GeoDataTrack.cpp.
◆ coordinatesAt() [1/2]
GeoDataCoordinates Marble::GeoDataTrack::coordinatesAt | ( | const QDateTime & | when | ) | const |
If interpolate() is true, return the coordinates interpolated from the time values before and after when
, otherwise return the coordinates of the point with the closest time value less than or equal to when
.
- See also
- interpolate
Definition at line 150 of file GeoDataTrack.cpp.
◆ coordinatesAt() [2/2]
GeoDataCoordinates Marble::GeoDataTrack::coordinatesAt | ( | int | index | ) | const |
Return coordinates at specified index.
This is useful when the track contains coordinates without time information.
Definition at line 205 of file GeoDataTrack.cpp.
◆ coordinatesList()
QList< GeoDataCoordinates > Marble::GeoDataTrack::coordinatesList | ( | ) | const |
Returns the coordinates of all the points in the map, sorted by their time value.
Definition at line 138 of file GeoDataTrack.cpp.
◆ copy()
|
overridevirtual |
Implements Marble::GeoDataGeometry.
Definition at line 77 of file GeoDataTrack.cpp.
◆ extendedData() [1/2]
GeoDataExtendedData & Marble::GeoDataTrack::extendedData | ( | ) |
Definition at line 316 of file GeoDataTrack.cpp.
◆ extendedData() [2/2]
const GeoDataExtendedData & Marble::GeoDataTrack::extendedData | ( | ) | const |
Return the ExtendedData assigned to the feature.
Definition at line 324 of file GeoDataTrack.cpp.
◆ firstWhen()
QDateTime Marble::GeoDataTrack::firstWhen | ( | ) | const |
Return the time value of the first point in the track, or an invalid QDateTime if the track is empty.
Definition at line 116 of file GeoDataTrack.cpp.
◆ geometryId()
|
overridevirtual |
Implements Marble::GeoDataGeometry.
Definition at line 72 of file GeoDataTrack.cpp.
◆ interpolate()
bool Marble::GeoDataTrack::interpolate | ( | ) | const |
Returns true if coordinatesAt() should use interpolation, false otherwise.
The default is false.
- See also
- setInterpolate, coordinatesAt
Definition at line 102 of file GeoDataTrack.cpp.
◆ lastWhen()
QDateTime Marble::GeoDataTrack::lastWhen | ( | ) | const |
Return the time value of the last point in the track, or an invalid QDateTime if the track is empty.
Definition at line 127 of file GeoDataTrack.cpp.
◆ latLonAltBox()
|
overridevirtual |
Reimplemented from Marble::GeoDataGeometry.
Definition at line 338 of file GeoDataTrack.cpp.
◆ lineString()
const GeoDataLineString * Marble::GeoDataTrack::lineString | ( | ) | const |
Return the GeoDataLineString representing the current track.
Definition at line 305 of file GeoDataTrack.cpp.
◆ nodeType()
|
overridevirtual |
Provides type information for downcasting a GeoNode.
Implements Marble::GeoNode.
Definition at line 67 of file GeoDataTrack.cpp.
◆ operator!=()
bool Marble::GeoDataTrack::operator!= | ( | const GeoDataTrack & | other | ) | const |
Definition at line 91 of file GeoDataTrack.cpp.
◆ operator==()
bool Marble::GeoDataTrack::operator== | ( | const GeoDataTrack & | other | ) | const |
: Equality operators.
Definition at line 82 of file GeoDataTrack.cpp.
◆ pack()
|
override |
Definition at line 344 of file GeoDataTrack.cpp.
◆ removeAfter()
void Marble::GeoDataTrack::removeAfter | ( | const QDateTime & | when | ) |
Remove all points from the track whose time value is greater than when
.
Definition at line 289 of file GeoDataTrack.cpp.
◆ removeBefore()
void Marble::GeoDataTrack::removeBefore | ( | const QDateTime & | when | ) |
Remove all points from the track whose time value is less than when
.
Definition at line 272 of file GeoDataTrack.cpp.
◆ setExtendedData()
void Marble::GeoDataTrack::setExtendedData | ( | const GeoDataExtendedData & | extendedData | ) |
Sets the ExtendedData of the feature.
- Parameters
-
extendedData the new ExtendedData to be used.
Definition at line 330 of file GeoDataTrack.cpp.
◆ setInterpolate()
void Marble::GeoDataTrack::setInterpolate | ( | bool | on | ) |
Set whether coordinatesAt() should use interpolation.
- See also
- interpolate, coordinatesAt
Definition at line 108 of file GeoDataTrack.cpp.
◆ size()
int Marble::GeoDataTrack::size | ( | ) | const |
Returns the number of points in the track.
Definition at line 96 of file GeoDataTrack.cpp.
◆ unpack()
|
override |
Definition at line 349 of file GeoDataTrack.cpp.
◆ whenList()
Returns the time value of all the points in the map, in chronological order.
- Since
- 0.26.0
Definition at line 144 of file GeoDataTrack.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 24 2025 11:52:10 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.