QCA::KeyStoreListContext
#include <QtCrypto>
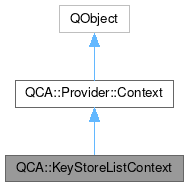
Signals | |
void | busyEnd () |
void | busyStart () |
void | diagnosticText (const QString &str) |
void | storeUpdated (int id) |
void | updated () |
Public Member Functions | |
KeyStoreListContext (Provider *p) | |
virtual KeyStoreEntryContext * | entry (int id, const QString &entryId) |
virtual QList< KeyStoreEntryContext * > | entryList (int id)=0 |
virtual KeyStoreEntryContext * | entryPassive (const QString &serialized) |
virtual QList< KeyStoreEntry::Type > | entryTypes (int id) const =0 |
virtual bool | isReadOnly (int id) const |
virtual QList< int > | keyStores ()=0 |
virtual QString | name (int id) const =0 |
virtual bool | removeEntry (int id, const QString &entryId) |
virtual void | setUpdatesEnabled (bool enabled) |
virtual void | start () |
virtual QString | storeId (int id) const =0 |
virtual KeyStore::Type | type (int id) const =0 |
virtual QString | writeEntry (int id, const Certificate &cert) |
virtual QString | writeEntry (int id, const CRL &crl) |
virtual QString | writeEntry (int id, const KeyBundle &kb) |
virtual QString | writeEntry (int id, const PGPKey &key) |
![]() | |
virtual Context * | clone () const =0 |
Provider * | provider () const |
bool | sameProvider (const Context *c) const |
QString | type () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
Context (const Context &from) | |
Context (Provider *parent, const QString &type) | |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
KeyStore provider.
- Note
- This class is part of the provider plugin interface and should not be used directly by applications. You probably want KeyStore instead.
Definition at line 1973 of file qcaprovider.h.
Constructor & Destructor Documentation
◆ KeyStoreListContext()
|
inline |
Standard constructor.
- Parameters
-
p the Provider associated with this context
Definition at line 1982 of file qcaprovider.h.
Member Function Documentation
◆ busyEnd
|
signal |
◆ busyStart
|
signal |
Emit this when the provider is busy looking for keystores.
The provider goes into a busy state when it has reason to believe there are keystores present, but it still needs to check or query some devices to see for sure.
For example, if a smart card is inserted, then the provider may immediately go into a busy state upon detecting the insert. However, it may take some seconds before the smart card information can be queried and reported by the provider. Once the card is queried successfully, the provider would leave the busy state and report the new keystore.
When this object is first started with start(), it is assumed to be in the busy state, so there is no need to emit this signal at the beginning.
◆ diagnosticText
|
signal |
Emitted when there is diagnostic text to report.
- Parameters
-
str the diagnostic text
◆ entry()
|
virtual |
Returns a single entry in the store, if the entry id is already known.
If the entry does not exist, the function returns 0.
The caller is responsible for deleting the returned entry object.
- Parameters
-
id the id for the store context entryId the entry to retrieve
◆ entryList()
|
pure virtual |
Returns the entries of the store, or an empty list if the integer context id is invalid.
The caller is responsible for deleting the returned entry objects.
- Parameters
-
id the id for the store context
◆ entryPassive()
|
virtual |
Returns a single entry, created from the serialization string of a previous entry (using KeyStoreEntryContext::serialize()).
If the serialization string cannot be parsed by this provider, or the entry cannot otherwise be created, the function returns 0.
The caller is responsible for deleting the returned entry object.
This function must be thread-safe.
- Parameters
-
serialized the serialized data to create the entry from
◆ entryTypes()
|
pure virtual |
Returns the types supported by the store, or an empty list if the integer context id is invalid.
This function should return all supported types, even if the store doesn't actually contain entries for all of the types.
- Parameters
-
id the id for the store context
◆ isReadOnly()
|
virtual |
Returns true if the store is read-only.
If the integer context id is invalid, this function should return true.
- Parameters
-
id the id for the store context
◆ keyStores()
|
pure virtual |
Returns a list of integer context ids, each representing a keystore instance.
If a keystore becomes unavailable and then later becomes available again (for example, if a smart card is removed and then the same one is inserted again), the integer context id must be different than last time.
◆ name()
|
pure virtual |
Returns the friendly name of the store, or an empty string if the integer context id is invalid.
- Parameters
-
id the id for the store context
◆ removeEntry()
|
virtual |
Remove an entry from the store.
Returns true if the entry is successfully removed, otherwise false.
- Parameters
-
id the id for the store context entryId the entry to remove from the store
◆ setUpdatesEnabled()
|
virtual |
Enables or disables update events.
The updated() and storeUpdated() signals might not be emitted if updates are not enabled.
- Parameters
-
enabled whether update notifications are enabled (true) or disabled (false)
◆ start()
|
virtual |
Starts the keystore provider.
◆ storeId()
|
pure virtual |
Returns the string id of the store, or an empty string if the integer context id is invalid.
The string id of the store should be unique to a single store, and it should persist between availability/unavailability. For example, a smart card that is removed and inserted again should have the same string id (despite having a new integer context id).
- Parameters
-
id the id for the store context
◆ storeUpdated
|
signal |
Indicates that the entry list of a keystore has changed (entries added, removed, or modified)
- Parameters
-
id the id of the key store that has changed
◆ type()
|
pure virtual |
Returns the type of the specified store, or -1 if the integer context id is invalid.
- Parameters
-
id the id for the store context
◆ updated
|
signal |
Indicates the list of keystores has changed, and that QCA should call keyStores() to obtain the latest list.
◆ writeEntry() [1/4]
|
virtual |
Write a Certificate to the store.
Returns the entry id of the new item, or an empty string if there was an error writing the item.
- Parameters
-
id the id for the store context cert the certificate to add to the store
◆ writeEntry() [2/4]
Write a CRL to the store.
Returns the entry id of the new item, or an empty string if there was an error writing the item.
- Parameters
-
id the id for the store context crl the revocation list to add to the store
◆ writeEntry() [3/4]
Write a KeyBundle to the store.
Returns the entry id of the new item, or an empty string if there was an error writing the item.
- Parameters
-
id the id for the store context kb the key bundle to add to the store
◆ writeEntry() [4/4]
Write a PGPKey to the store.
Returns the entry id of the new item, or an empty string if there was an error writing the item.
- Parameters
-
id the id for the store context key the PGP key to add to the store
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:01:48 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.