KFileWidget
#include <KFileWidget>
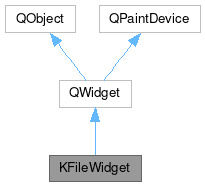
Public Types | |
enum | OperationMode { Other = 0 , Opening , Saving } |
![]() | |
enum | RenderFlag |
![]() | |
enum | PaintDeviceMetric |
Signals | |
void | accepted () |
void | fileHighlighted (const QUrl &) |
void | fileSelected (const QUrl &) |
void | filterChanged (const KFileFilter &filter) |
void | selectionChanged () |
Public Slots | |
void | accept () |
void | slotCancel () |
void | slotOk () |
Public Member Functions | |
KFileWidget (const QUrl &startDir, QWidget *parent=nullptr) | |
~KFileWidget () override | |
QUrl | baseUrl () const |
QPushButton * | cancelButton () const |
void | clearFilter () |
KFileFilter | currentFilter () const |
QSize | dialogSizeHint () const |
KDirOperator * | dirOperator () |
KFileFilterCombo * | filterWidget () const |
bool | keepsLocation () const |
KUrlComboBox * | locationEdit () const |
KFile::Modes | mode () const |
QPushButton * | okButton () const |
OperationMode | operationMode () const |
void | readConfig (KConfigGroup &group) |
QString | selectedFile () const |
QStringList | selectedFiles () const |
QUrl | selectedUrl () const |
QList< QUrl > | selectedUrls () const |
void | setConfirmOverwrite (bool enable) |
void | setCustomWidget (const QString &text, QWidget *widget) |
void | setCustomWidget (QWidget *widget) |
void | setFilters (const QList< KFileFilter > &filters, const KFileFilter &activeFilter=KFileFilter()) |
void | setInlinePreviewShown (bool show) |
void | setKeepLocation (bool keep) |
void | setLocationLabel (const QString &text) |
void | setMode (KFile::Modes m) |
void | setOperationMode (OperationMode) |
void | setPreviewWidget (KPreviewWidgetBase *w) |
void | setSelectedUrl (const QUrl &url) |
void | setSelectedUrls (const QList< QUrl > &urls) |
void | setSupportedSchemes (const QStringList &schemes) |
void | setUrl (const QUrl &url, bool clearforward=true) |
void | setViewMode (KFile::FileView mode) |
QSize | sizeHint () const override |
QStringList | supportedSchemes () const |
![]() | |
QWidget (QWidget *parent, Qt::WindowFlags f) | |
bool | acceptDrops () const const |
QString | accessibleDescription () const const |
QString | accessibleName () const const |
QList< QAction * > | actions () const const |
void | activateWindow () |
QAction * | addAction (const QIcon &icon, const QString &text) |
QAction * | addAction (const QIcon &icon, const QString &text, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QIcon &icon, const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text) |
QAction * | addAction (const QString &text, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
void | addAction (QAction *action) |
void | addActions (const QList< QAction * > &actions) |
void | adjustSize () |
bool | autoFillBackground () const const |
QPalette::ColorRole | backgroundRole () const const |
QBackingStore * | backingStore () const const |
QSize | baseSize () const const |
QWidget * | childAt (const QPoint &p) const const |
QWidget * | childAt (int x, int y) const const |
QRect | childrenRect () const const |
QRegion | childrenRegion () const const |
void | clearFocus () |
void | clearMask () |
bool | close () |
QMargins | contentsMargins () const const |
QRect | contentsRect () const const |
Qt::ContextMenuPolicy | contextMenuPolicy () const const |
QCursor | cursor () const const |
void | customContextMenuRequested (const QPoint &pos) |
WId | effectiveWinId () const const |
void | ensurePolished () const const |
Qt::FocusPolicy | focusPolicy () const const |
QWidget * | focusProxy () const const |
QWidget * | focusWidget () const const |
const QFont & | font () const const |
QFontInfo | fontInfo () const const |
QFontMetrics | fontMetrics () const const |
QPalette::ColorRole | foregroundRole () const const |
QRect | frameGeometry () const const |
QSize | frameSize () const const |
const QRect & | geometry () const const |
QPixmap | grab (const QRect &rectangle) |
void | grabGesture (Qt::GestureType gesture, Qt::GestureFlags flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const const |
QGraphicsProxyWidget * | graphicsProxyWidget () const const |
bool | hasEditFocus () const const |
bool | hasFocus () const const |
virtual bool | hasHeightForWidth () const const |
bool | hasMouseTracking () const const |
bool | hasTabletTracking () const const |
int | height () const const |
virtual int | heightForWidth (int w) const const |
void | hide () |
Qt::InputMethodHints | inputMethodHints () const const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, const QList< QAction * > &actions) |
bool | isActiveWindow () const const |
bool | isAncestorOf (const QWidget *child) const const |
bool | isEnabled () const const |
bool | isEnabledTo (const QWidget *ancestor) const const |
bool | isFullScreen () const const |
bool | isHidden () const const |
bool | isMaximized () const const |
bool | isMinimized () const const |
bool | isModal () const const |
bool | isTopLevel () const const |
bool | isVisible () const const |
bool | isVisibleTo (const QWidget *ancestor) const const |
bool | isWindow () const const |
bool | isWindowModified () const const |
QLayout * | layout () const const |
Qt::LayoutDirection | layoutDirection () const const |
QLocale | locale () const const |
void | lower () |
QPoint | mapFrom (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapFrom (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapFromGlobal (const QPoint &pos) const const |
QPointF | mapFromGlobal (const QPointF &pos) const const |
QPoint | mapFromParent (const QPoint &pos) const const |
QPointF | mapFromParent (const QPointF &pos) const const |
QPoint | mapTo (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapTo (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapToGlobal (const QPoint &pos) const const |
QPointF | mapToGlobal (const QPointF &pos) const const |
QPoint | mapToParent (const QPoint &pos) const const |
QPointF | mapToParent (const QPointF &pos) const const |
QRegion | mask () const const |
int | maximumHeight () const const |
QSize | maximumSize () const const |
int | maximumWidth () const const |
int | minimumHeight () const const |
QSize | minimumSize () const const |
virtual QSize | minimumSizeHint () const const |
int | minimumWidth () const const |
void | move (const QPoint &) |
void | move (int x, int y) |
QWidget * | nativeParentWidget () const const |
QWidget * | nextInFocusChain () const const |
QRect | normalGeometry () const const |
void | overrideWindowFlags (Qt::WindowFlags flags) |
virtual QPaintEngine * | paintEngine () const const override |
const QPalette & | palette () const const |
QWidget * | parentWidget () const const |
QPoint | pos () const const |
QWidget * | previousInFocusChain () const const |
QWIDGETSIZE_MAX QWIDGETSIZE_MAX | |
void | raise () |
QRect | rect () const const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | repaint () |
void | repaint (const QRect &rect) |
void | repaint (const QRegion &rgn) |
void | repaint (int x, int y, int w, int h) |
void | resize (const QSize &) |
void | resize (int w, int h) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const const |
QScreen * | screen () const const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setContentsMargins (const QMargins &margins) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus () |
void | setFocus (Qt::FocusReason reason) |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (const QRect &) |
void | setGeometry (int x, int y, int w, int h) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setInputMethodHints (Qt::InputMethodHints hints) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, Qt::WindowFlags f) |
void | setScreen (QScreen *screen) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
void | setStyleSheet (const QString &styleSheet) |
void | setTabletTracking (bool enable) |
void | setToolTip (const QString &) |
void | setToolTipDuration (int msec) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlag (Qt::WindowType flag, bool on) |
void | setWindowFlags (Qt::WindowFlags type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (Qt::WindowStates windowState) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const const |
QSize | sizeIncrement () const const |
QSizePolicy | sizePolicy () const const |
void | stackUnder (QWidget *w) |
QString | statusTip () const const |
QStyle * | style () const const |
QString | styleSheet () const const |
bool | testAttribute (Qt::WidgetAttribute attribute) const const |
QString | toolTip () const const |
int | toolTipDuration () const const |
QWidget * | topLevelWidget () const const |
bool | underMouse () const const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | update () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | updateGeometry () |
bool | updatesEnabled () const const |
QRegion | visibleRegion () const const |
QString | whatsThis () const const |
int | width () const const |
QWidget * | window () const const |
QString | windowFilePath () const const |
Qt::WindowFlags | windowFlags () const const |
QWindow * | windowHandle () const const |
QIcon | windowIcon () const const |
void | windowIconChanged (const QIcon &icon) |
QString | windowIconText () const const |
void | windowIconTextChanged (const QString &iconText) |
Qt::WindowModality | windowModality () const const |
qreal | windowOpacity () const const |
QString | windowRole () const const |
Qt::WindowStates | windowState () const const |
QString | windowTitle () const const |
void | windowTitleChanged (const QString &title) |
Qt::WindowType | windowType () const const |
WId | winId () const const |
int | x () const const |
int | y () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
int | colorCount () const const |
int | depth () const const |
qreal | devicePixelRatio () const const |
qreal | devicePixelRatioF () const const |
int | height () const const |
int | heightMM () const const |
int | logicalDpiX () const const |
int | logicalDpiY () const const |
bool | paintingActive () const const |
int | physicalDpiX () const const |
int | physicalDpiY () const const |
int | width () const const |
int | widthMM () const const |
Static Public Member Functions | |
static QUrl | getStartUrl (const QUrl &startDir, QString &recentDirClass) |
static QUrl | getStartUrl (const QUrl &startDir, QString &recentDirClass, QString &fileName) |
static void | setStartDir (const QUrl &directory) |
![]() | |
QWidget * | createWindowContainer (QWindow *window, QWidget *parent, Qt::WindowFlags flags) |
QWidget * | find (WId id) |
QWidget * | keyboardGrabber () |
QWidget * | mouseGrabber () |
void | setTabOrder (QWidget *first, QWidget *second) |
void | setTabOrder (std::initializer_list< QWidget * > widgets) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
bool | eventFilter (QObject *watched, QEvent *event) override |
void | resizeEvent (QResizeEvent *event) override |
void | showEvent (QShowEvent *event) override |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | changeEvent (QEvent *event) |
virtual void | closeEvent (QCloseEvent *event) |
virtual void | contextMenuEvent (QContextMenuEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual void | enterEvent (QEnterEvent *event) |
virtual bool | event (QEvent *event) override |
virtual void | focusInEvent (QFocusEvent *event) |
bool | focusNextChild () |
virtual bool | focusNextPrevChild (bool next) |
virtual void | focusOutEvent (QFocusEvent *event) |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | initPainter (QPainter *painter) const const override |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual void | keyPressEvent (QKeyEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | leaveEvent (QEvent *event) |
virtual int | metric (PaintDeviceMetric m) const const override |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
virtual void | mouseReleaseEvent (QMouseEvent *event) |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | nativeEvent (const QByteArray &eventType, void *message, qintptr *result) |
virtual void | paintEvent (QPaintEvent *event) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus (Qt::InputMethodQuery query) |
virtual void | wheelEvent (QWheelEvent *event) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
File selector widget.
This is the contents of the KDE file dialog, without the actual QDialog around it. It can be embedded directly into applications.
Definition at line 48 of file kfilewidget.h.
Member Enumeration Documentation
◆ OperationMode
Defines some default behavior of the filedialog.
E.g. in mode Opening
and Saving
, the selected files/urls will be added to the "recent documents" list. The Saving mode also implies setKeepLocation() being set.
Other
means that no default actions are performed.
- See also
- setOperationMode
- operationMode
Definition at line 102 of file kfilewidget.h.
Constructor & Destructor Documentation
◆ KFileWidget()
Constructs a file selector widget.
- Parameters
-
startDir This can either be: - An empty URL (QUrl()) to start in the current working directory, or the last directory where a file has been selected.
- The path or URL of a starting directory.
- An initial file name to select, with the starting directory being the current working directory or the last directory where a file has been selected.
- The path or URL of a file, specifying both the starting directory and an initially selected file name.
- A URL of the form
kfiledialog:///<keyword>
to start in the directory last used by a filedialog in the same application that specified the same keyword. - A URL of the form
kfiledialog:///<keyword>/<filename>
to start in the directory last used by a filedialog in the same application that specified the same keyword, and to initially select the specified filename. - Deprecated: A URL of the form
kfiledialog:///<keyword>
?global to start in the directory last used by a filedialog in any application that specified the same keyword. - Deprecated: A URL of the form
kfiledialog:///<keyword>/<filename>
?global to start in the directory last used by a filedialog in any application that specified the same keyword, and to initially select the specified filename.
- Note
- Since 5.96, the "?global" syntax is deprecated, for lack of usage.
- Parameters
-
parent The parent widget of this widget
Definition at line 351 of file kfilewidget.cpp.
◆ ~KFileWidget()
|
override |
Destructor.
Definition at line 498 of file kfilewidget.cpp.
Member Function Documentation
◆ accept
|
slot |
Definition at line 848 of file kfilewidget.cpp.
◆ accepted
|
signal |
Emitted by slotOk() (directly or asynchronously) once everything has been done.
Should be used by the caller to call accept().
◆ baseUrl()
QUrl KFileWidget::baseUrl | ( | ) | const |
- Returns
- the currently shown directory.
Definition at line 1974 of file kfilewidget.cpp.
◆ cancelButton()
QPushButton * KFileWidget::cancelButton | ( | ) | const |
- Returns
- a pointer to the Cancel-Button in the filedialog. Note that the button is hidden and unconnected when using KFileWidget alone; KFileDialog shows it and connects to it.
Definition at line 2156 of file kfilewidget.cpp.
◆ clearFilter()
void KFileWidget::clearFilter | ( | ) |
Clears any MIME type or name filter.
Does not reload the directory.
Definition at line 528 of file kfilewidget.cpp.
◆ currentFilter()
KFileFilter KFileWidget::currentFilter | ( | ) | const |
Returns the current filter as entered by the user or one of the predefined set via setFilters().
- See also
- setFilters()
- filterChanged()
- Since
- 6.0
Definition at line 523 of file kfilewidget.cpp.
◆ dialogSizeHint()
QSize KFileWidget::dialogSizeHint | ( | ) | const |
Provides a size hint, useful for dialogs that embed the widget.
- Returns
- a QSize, calculated to be optimal for a dialog.
- Since
- 5.0
Definition at line 3044 of file kfilewidget.cpp.
◆ dirOperator()
KDirOperator * KFileWidget::dirOperator | ( | ) |
- Returns
- the KDirOperator used to navigate the filesystem
Definition at line 2986 of file kfilewidget.cpp.
◆ eventFilter()
Reimplemented from QObject.
Definition at line 2007 of file kfilewidget.cpp.
◆ fileHighlighted
|
signal |
Emitted when the user highlights a file.
- Since
- 4.4
◆ fileSelected
|
signal |
Emitted when the user selects a file.
It is only emitted in single- selection mode. The best way to get notified about selected file(s) is to connect to the okClicked() signal inherited from KDialog and call selectedFile(), selectedFiles(), selectedUrl() or selectedUrls().
- Since
- 4.4
◆ filterChanged
|
signal |
Emitted when the filter changed, i.e. the user entered an own filter or chose one of the predefined set via setFilters().
- Parameters
-
filter contains the new filter (only the extension part, not the explanation), i.e. "*.cpp" or "*.cpp *.cc".
- See also
- setFilters()
- currentFilter()
- Since
- 6.0
◆ filterWidget()
KFileFilterCombo * KFileWidget::filterWidget | ( | ) | const |
- Returns
- the combobox that contains the filters
Definition at line 2733 of file kfilewidget.cpp.
◆ getStartUrl() [1/2]
This method implements the logic to determine the user's default directory to be listed.
E.g. the documents directory, home directory or a recently used directory.
- Parameters
-
startDir A URL specifying the initial directory, or using the kfiledialog:///
syntax to specify a last used directory. If this URL specifies a file name, it is ignored. Refer to the KFileWidget::KFileWidget() documentation for thekfiledialog:///
URL syntax.recentDirClass If the kfiledialog:///
syntax is used, this will return the string to be passed to KRecentDirs::dir() and KRecentDirs::add().
- Returns
- The URL that should be listed by default (e.g. by KFileDialog or KDirSelectDialog).
- See also
- KFileWidget::KFileWidget()
Definition at line 2823 of file kfilewidget.cpp.
◆ getStartUrl() [2/2]
|
static |
Similar to getStartUrl(const QUrl& startDir,QString& recentDirClass), but allows both the recent start directory keyword and a suggested file name to be returned.
- Parameters
-
startDir A URL specifying the initial directory and/or filename, or using the kfiledialog:///
syntax to specify a last used location. Refer to the KFileWidget::KFileWidget() documentation for thekfiledialog:///
URL syntax.recentDirClass If the kfiledialog:///
syntax is used, this will return the string to be passed to KRecentDirs::dir() and KRecentDirs::add().fileName The suggested file name, if specified as part of the StartDir
URL.
- Returns
- The URL that should be listed by default (e.g. by KFileDialog or KDirSelectDialog).
- See also
- KFileWidget::KFileWidget()
Definition at line 2830 of file kfilewidget.cpp.
◆ keepsLocation()
bool KFileWidget::keepsLocation | ( | ) | const |
- Returns
- whether the contents of the location edit are kept when changing directories.
Definition at line 2173 of file kfilewidget.cpp.
◆ locationEdit()
KUrlComboBox * KFileWidget::locationEdit | ( | ) | const |
- Returns
- the combobox used to type the filename or full location of the file.
Definition at line 2728 of file kfilewidget.cpp.
◆ mode()
KFile::Modes KFileWidget::mode | ( | ) | const |
Returns the mode of the filedialog.
- See also
- setMode()
Definition at line 2043 of file kfilewidget.cpp.
◆ okButton()
QPushButton * KFileWidget::okButton | ( | ) | const |
- Returns
- a pointer to the OK-Button in the filedialog. Note that the button is hidden and unconnected when using KFileWidget alone; KFileDialog shows it and connects to it.
Definition at line 2151 of file kfilewidget.cpp.
◆ operationMode()
KFileWidget::OperationMode KFileWidget::operationMode | ( | ) | const |
- Returns
- the current operation mode, Opening, Saving or Other. Default is Other.
Definition at line 2207 of file kfilewidget.cpp.
◆ readConfig()
void KFileWidget::readConfig | ( | KConfigGroup & | group | ) |
reads the configuration for this widget from the given config group
- Parameters
-
group the KConfigGroup to read from
- Deprecated
- since 6.3, no known use case.
Definition at line 2992 of file kfilewidget.cpp.
◆ resizeEvent()
|
overrideprotectedvirtual |
Reimplemented from QWidget.
Definition at line 1979 of file kfilewidget.cpp.
◆ selectedFile()
QString KFileWidget::selectedFile | ( | ) | const |
Returns the full path of the selected file in the local filesystem.
(Local files only)
Definition at line 1932 of file kfilewidget.cpp.
◆ selectedFiles()
QStringList KFileWidget::selectedFiles | ( | ) | const |
Returns a list of all selected local files.
Definition at line 1947 of file kfilewidget.cpp.
◆ selectedUrl()
QUrl KFileWidget::selectedUrl | ( | ) | const |
- Returns
- The selected fully qualified filename.
Definition at line 1820 of file kfilewidget.cpp.
◆ selectedUrls()
- Returns
- The list of selected URLs.
Definition at line 1831 of file kfilewidget.cpp.
◆ selectionChanged
|
signal |
Emitted when the user highlights one or more files in multiselection mode.
Note: fileHighlighted() or fileSelected() are not emitted in multiselection mode. You may use selectedItems() to ask for the current highlighted items.
- See also
- fileSelected
◆ setConfirmOverwrite()
void KFileWidget::setConfirmOverwrite | ( | bool | enable | ) |
Sets whether the user should be asked for confirmation when an overwrite might occur.
- Parameters
-
enable Set this to true to enable checking.
Definition at line 3034 of file kfilewidget.cpp.
◆ setCustomWidget() [1/2]
Sets a custom widget that should be added below the location and the filter editors.
- Parameters
-
text Label of the custom widget, which is displayed below the labels "Location:" and "Filter:". widget Any kind of widget, but preferable a combo box or a line editor to be compliant with the location and filter layout. When creating this widget, you don't need to specify a parent, since the widget's parent will be set automatically by KFileWidget.
Definition at line 2976 of file kfilewidget.cpp.
◆ setCustomWidget() [2/2]
void KFileWidget::setCustomWidget | ( | QWidget * | widget | ) |
Set a custom widget that should be added to the file dialog.
- Parameters
-
widget A widget, or a widget of widgets, for displaying custom data in the file widget. This can be used, for example, to display a check box with the title "Open as read-only". When creating this widget, you don't need to specify a parent, since the widget's parent will be set automatically by KFileWidget.
Definition at line 2955 of file kfilewidget.cpp.
◆ setFilters()
void KFileWidget::setFilters | ( | const QList< KFileFilter > & | filters, |
const KFileFilter & | activeFilter = KFileFilter() ) |
Set the filters to be used.
Each item of the list corresponds to a selectable filter.
Only one filter is active at a time.
- Parameters
-
activeFilter the initially active filter
- Since
- 6.0
Definition at line 511 of file kfilewidget.cpp.
◆ setInlinePreviewShown()
void KFileWidget::setInlinePreviewShown | ( | bool | show | ) |
Forces the inline previews to be shown or hidden, depending on show
.
- Parameters
-
show Whether to show inline previews or not.
Definition at line 3039 of file kfilewidget.cpp.
◆ setKeepLocation()
void KFileWidget::setKeepLocation | ( | bool | keep | ) |
Sets whether the filename/url should be kept when changing directories.
This is for example useful when having a predefined filename where the full path for that file is searched.
This is implicitly set when operationMode() is KFileWidget::Saving
getSaveFileName() and getSaveUrl() set this to true by default, so that you can type in the filename and change the directory without having to type the name again.
Definition at line 2168 of file kfilewidget.cpp.
◆ setLocationLabel()
void KFileWidget::setLocationLabel | ( | const QString & | text | ) |
Sets the text to be displayed in front of the selection.
The default is "Location". Most useful if you want to make clear what the location is used for.
Definition at line 506 of file kfilewidget.cpp.
◆ setMode()
void KFileWidget::setMode | ( | KFile::Modes | m | ) |
Sets the mode of the dialog.
The mode is defined as (in kfile.h):
You can OR the values, e.g.
Definition at line 2029 of file kfilewidget.cpp.
◆ setOperationMode()
void KFileWidget::setOperationMode | ( | OperationMode | mode | ) |
Sets the operational mode of the filedialog to Saving
, Opening
or Other
.
This will set some flags that are specific to loading or saving files. E.g. setKeepLocation() makes mostly sense for a save-as dialog. So setOperationMode( KFileWidget::Saving ); sets setKeepLocation for example.
The mode Saving
, together with a default filter set via setMimeFilter() will make the filter combobox read-only.
The default mode is Opening
.
Call this method right after instantiating KFileWidget.
Definition at line 2178 of file kfilewidget.cpp.
◆ setPreviewWidget()
void KFileWidget::setPreviewWidget | ( | KPreviewWidgetBase * | w | ) |
Adds a preview widget and enters the preview mode.
In this mode the dialog is split and the right part contains your preview widget.
Ownership is transferred to KFileWidget. You need to create the preview-widget with "new", i.e. on the heap.
- Parameters
-
w The widget to be used for the preview.
Definition at line 538 of file kfilewidget.cpp.
◆ setSelectedUrl()
void KFileWidget::setSelectedUrl | ( | const QUrl & | url | ) |
Sets the URL to preselect to url
.
This method handles absolute URLs (remember to use fromLocalFile for local paths). It also handles relative URLs, which you should construct like this: QUrl relativeUrl; relativeUrl.setPath(fileName);
- Since
- 5.33
Definition at line 1762 of file kfilewidget.cpp.
◆ setSelectedUrls()
Sets a list of URLs as preselected.
- See also
- setSelectedUrl
- Since
- 5.75
Definition at line 1771 of file kfilewidget.cpp.
◆ setStartDir()
|
static |
Used by KDirSelectDialog to share the dialog's start directory.
Definition at line 2907 of file kfilewidget.cpp.
◆ setSupportedSchemes()
void KFileWidget::setSupportedSchemes | ( | const QStringList & | schemes | ) |
Set the URL schemes that the file widget should allow navigating to.
If the returned list is empty, all schemes are supported.
- See also
- QFileDialog::setSupportedSchemes
- Since
- 5.43
Definition at line 3060 of file kfilewidget.cpp.
◆ setUrl()
void KFileWidget::setUrl | ( | const QUrl & | url, |
bool | clearforward = true ) |
Sets the directory to view.
- Parameters
-
url URL to show. clearforward Indicates whether the forward queue should be cleared.
Definition at line 1652 of file kfilewidget.cpp.
◆ setViewMode()
void KFileWidget::setViewMode | ( | KFile::FileView | mode | ) |
Sets how the view should be displayed.
- See also
- KFile::FileView
- Since
- 5.0
Definition at line 3054 of file kfilewidget.cpp.
◆ showEvent()
|
overrideprotectedvirtual |
Reimplemented from QWidget.
Definition at line 1990 of file kfilewidget.cpp.
◆ sizeHint()
|
overridevirtual |
◆ slotCancel
|
slot |
Definition at line 2162 of file kfilewidget.cpp.
◆ slotOk
|
slot |
Called when clicking ok (when this widget is used in KFileDialog) Might or might not call accept().
Logic of the next part of code (ends at "end multi relative urls").
We allow for instance to be at "/" and insert '"home/foo/bar.txt" "boot/grub/menu.lst"'. Why we need to support this ? Because we provide tree views, which aren't plain.
Now, how does this logic work. It will get the first element on the list (with no filename), following the previous example say "/home/foo" and set it as the top most url.
After this, it will iterate over the rest of items and check if this URL (topmost url) contains the url being iterated.
As you might have guessed it will do "/home/foo" against "/boot/grub" (again stripping filename), and a false will be returned. Then we upUrl the top most url, resulting in "/home" against "/boot/grub", what will again return false, so we upUrl again. Now we have "/" against "/boot/grub", what returns true for us, so we can say that the closest common ancestor of both is "/".
This example has been written for 2 urls, but this works for any number of urls.
end multi relative urls
Definition at line 595 of file kfilewidget.cpp.
◆ supportedSchemes()
QStringList KFileWidget::supportedSchemes | ( | ) | const |
Returns the URL schemes that the file widget should allow navigating to.
If the returned list is empty, all schemes are supported. Examples for schemes are "file"
or "ftp"
.
- See also
- QFileDialog::supportedSchemes
- Since
- 5.43
Definition at line 3067 of file kfilewidget.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:24 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.