KDirOperator
#include <KDirOperator>
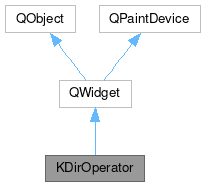
Public Types | |
enum | Action { PopupMenu , Up , Back , Forward , Home , Reload , New , NewFolder , Rename , Trash , Delete , SortMenu , SortByName , SortBySize , SortByDate , SortByType , SortAscending , SortDescending , SortFoldersFirst , SortHiddenFilesLast , ViewModeMenu , ViewIconsView , ViewCompactView , ViewDetailsView , DecorationMenu , DecorationAtTop , DecorationAtLeft , ShortView , DetailedView , TreeView , DetailedTreeView , AllowExpansionInDetailsView , ShowHiddenFiles , ShowPreviewPanel , ShowPreview , OpenContainingFolder , Properties } |
enum | ActionType { SortActions = 1 , ViewActions = 2 , NavActions = 4 , FileActions = 8 , AllActions = 15 } |
![]() | |
enum | RenderFlag |
![]() | |
enum | PaintDeviceMetric |
Signals | |
void | completion (const QString &) |
void | contextMenuAboutToShow (const KFileItem &item, QMenu *menu) |
void | currentIconSizeChanged (int size) |
void | dirActivated (const KFileItem &item) |
void | dropped (const KFileItem &item, QDropEvent *event, const QList< QUrl > &urls) |
void | fileHighlighted (const KFileItem &item) |
void | fileSelected (const KFileItem &item) |
void | finishedLoading () |
void | keyEnterReturnPressed () |
void | renamingFinished (const QList< QUrl > &urls) |
void | updateInformation (int files, int dirs) |
void | urlEntered (const QUrl &) |
void | viewChanged (QAbstractItemView *newView) |
Public Slots | |
virtual void | back () |
virtual void | cdUp () |
virtual void | deleteSelected () |
virtual void | forward () |
virtual void | home () |
QString | makeCompletion (const QString &) |
QString | makeDirCompletion (const QString &) |
virtual void | mkdir () |
void | renameSelected () |
virtual void | rereadDir () |
void | setIconSize (int value) |
void | setSupportedSchemes (const QStringList &schemes) |
virtual void | trashSelected () |
void | updateDir () |
void | updateSelectionDependentActions () |
Public Member Functions | |
KDirOperator (const QUrl &urlName=QUrl{}, QWidget *parent=nullptr) | |
~KDirOperator () override | |
QAction * | action (KDirOperator::Action action) const |
QList< QAction * > | allActions () const |
void | clearFilter () |
void | clearHistory () |
void | close () |
KCompletion * | completionObject () const |
QStyleOptionViewItem::Position | decorationPosition () const |
virtual KIO::DeleteJob * | del (const KFileItemList &items, QWidget *parent=nullptr, bool ask=true, bool showProgress=true) |
KCompletion * | dirCompletionObject () const |
bool | dirHighlighting () const |
KDirLister * | dirLister () const |
bool | dirOnlyMode () const |
bool | followNewDirectories () const |
bool | followSelectedDirectories () const |
int | iconSize () const |
bool | isInlinePreviewShown () const |
bool | isRoot () const |
bool | isSaving () const |
bool | isSelected (const KFileItem &item) const |
QStringList | mimeFilter () const |
KFile::Modes | mode () const |
QString | nameFilter () const |
QStringList | newFileMenuSupportedMimeTypes () const |
int | numDirs () const |
int | numFiles () const |
bool | onlyDoubleClickSelectsFiles () const |
KFilePreviewGenerator * | previewGenerator () const |
QProgressBar * | progressBar () const |
virtual void | readConfig (const KConfigGroup &configGroup) |
KFileItemList | selectedItems () const |
virtual void | setAcceptDrops (bool b) |
void | setCurrentItem (const KFileItem &item) |
void | setCurrentItem (const QUrl &url) |
void | setCurrentItems (const KFileItemList &items) |
void | setCurrentItems (const QList< QUrl > &urls) |
void | setDecorationPosition (QStyleOptionViewItem::Position position) |
virtual void | setDropOptions (int options) |
virtual void | setEnableDirHighlighting (bool enable) |
void | setFollowNewDirectories (bool enable) |
void | setFollowSelectedDirectories (bool enable) |
void | setInlinePreviewShown (bool show) |
void | setIsSaving (bool isSaving) |
void | setMimeFilter (const QStringList &mimetypes) |
virtual void | setMode (KFile::Modes m) |
void | setNameFilter (const QString &filter) |
void | setNewFileMenuSelectDirWhenAlreadyExist (bool selectOnDirExists) |
void | setNewFileMenuSupportedMimeTypes (const QStringList &mime) |
void | setOnlyDoubleClickSelectsFiles (bool enable) |
virtual void | setPreviewWidget (KPreviewWidgetBase *w) |
virtual void | setShowHiddenFiles (bool s) |
void | setSorting (QDir::SortFlags) |
void | setupMenu (int whichActions) |
virtual void | setUrl (const QUrl &url, bool clearforward) |
virtual void | setViewConfig (KConfigGroup &configGroup) |
void | setViewMode (KFile::FileView viewKind) |
bool | showHiddenFiles () const |
void | showOpenWithActions (bool enable) |
QDir::SortFlags | sorting () const |
QStringList | supportedSchemes () const |
virtual KIO::CopyJob * | trash (const KFileItemList &items, QWidget *parent, bool ask=true, bool showProgress=true) |
QUrl | url () const |
QAbstractItemView * | view () const |
KConfigGroup * | viewConfigGroup () const |
KFile::FileView | viewMode () const |
virtual void | writeConfig (KConfigGroup &configGroup) |
![]() | |
QWidget (QWidget *parent, Qt::WindowFlags f) | |
bool | acceptDrops () const const |
QString | accessibleDescription () const const |
QString | accessibleName () const const |
QList< QAction * > | actions () const const |
void | activateWindow () |
QAction * | addAction (const QIcon &icon, const QString &text) |
QAction * | addAction (const QIcon &icon, const QString &text, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QIcon &icon, const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text) |
QAction * | addAction (const QString &text, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
void | addAction (QAction *action) |
void | addActions (const QList< QAction * > &actions) |
void | adjustSize () |
bool | autoFillBackground () const const |
QPalette::ColorRole | backgroundRole () const const |
QBackingStore * | backingStore () const const |
QSize | baseSize () const const |
QWidget * | childAt (const QPoint &p) const const |
QWidget * | childAt (int x, int y) const const |
QRect | childrenRect () const const |
QRegion | childrenRegion () const const |
void | clearFocus () |
void | clearMask () |
bool | close () |
QMargins | contentsMargins () const const |
QRect | contentsRect () const const |
Qt::ContextMenuPolicy | contextMenuPolicy () const const |
QCursor | cursor () const const |
void | customContextMenuRequested (const QPoint &pos) |
WId | effectiveWinId () const const |
void | ensurePolished () const const |
Qt::FocusPolicy | focusPolicy () const const |
QWidget * | focusProxy () const const |
QWidget * | focusWidget () const const |
const QFont & | font () const const |
QFontInfo | fontInfo () const const |
QFontMetrics | fontMetrics () const const |
QPalette::ColorRole | foregroundRole () const const |
QRect | frameGeometry () const const |
QSize | frameSize () const const |
const QRect & | geometry () const const |
QPixmap | grab (const QRect &rectangle) |
void | grabGesture (Qt::GestureType gesture, Qt::GestureFlags flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const const |
QGraphicsProxyWidget * | graphicsProxyWidget () const const |
bool | hasEditFocus () const const |
bool | hasFocus () const const |
virtual bool | hasHeightForWidth () const const |
bool | hasMouseTracking () const const |
bool | hasTabletTracking () const const |
int | height () const const |
virtual int | heightForWidth (int w) const const |
void | hide () |
Qt::InputMethodHints | inputMethodHints () const const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, const QList< QAction * > &actions) |
bool | isActiveWindow () const const |
bool | isAncestorOf (const QWidget *child) const const |
bool | isEnabled () const const |
bool | isEnabledTo (const QWidget *ancestor) const const |
bool | isFullScreen () const const |
bool | isHidden () const const |
bool | isMaximized () const const |
bool | isMinimized () const const |
bool | isModal () const const |
bool | isTopLevel () const const |
bool | isVisible () const const |
bool | isVisibleTo (const QWidget *ancestor) const const |
bool | isWindow () const const |
bool | isWindowModified () const const |
QLayout * | layout () const const |
Qt::LayoutDirection | layoutDirection () const const |
QLocale | locale () const const |
void | lower () |
QPoint | mapFrom (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapFrom (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapFromGlobal (const QPoint &pos) const const |
QPointF | mapFromGlobal (const QPointF &pos) const const |
QPoint | mapFromParent (const QPoint &pos) const const |
QPointF | mapFromParent (const QPointF &pos) const const |
QPoint | mapTo (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapTo (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapToGlobal (const QPoint &pos) const const |
QPointF | mapToGlobal (const QPointF &pos) const const |
QPoint | mapToParent (const QPoint &pos) const const |
QPointF | mapToParent (const QPointF &pos) const const |
QRegion | mask () const const |
int | maximumHeight () const const |
QSize | maximumSize () const const |
int | maximumWidth () const const |
int | minimumHeight () const const |
QSize | minimumSize () const const |
virtual QSize | minimumSizeHint () const const |
int | minimumWidth () const const |
void | move (const QPoint &) |
void | move (int x, int y) |
QWidget * | nativeParentWidget () const const |
QWidget * | nextInFocusChain () const const |
QRect | normalGeometry () const const |
void | overrideWindowFlags (Qt::WindowFlags flags) |
virtual QPaintEngine * | paintEngine () const const override |
const QPalette & | palette () const const |
QWidget * | parentWidget () const const |
QPoint | pos () const const |
QWidget * | previousInFocusChain () const const |
QWIDGETSIZE_MAX QWIDGETSIZE_MAX | |
void | raise () |
QRect | rect () const const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | repaint () |
void | repaint (const QRect &rect) |
void | repaint (const QRegion &rgn) |
void | repaint (int x, int y, int w, int h) |
void | resize (const QSize &) |
void | resize (int w, int h) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const const |
QScreen * | screen () const const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setContentsMargins (const QMargins &margins) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus () |
void | setFocus (Qt::FocusReason reason) |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (const QRect &) |
void | setGeometry (int x, int y, int w, int h) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setInputMethodHints (Qt::InputMethodHints hints) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, Qt::WindowFlags f) |
void | setScreen (QScreen *screen) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
void | setStyleSheet (const QString &styleSheet) |
void | setTabletTracking (bool enable) |
void | setToolTip (const QString &) |
void | setToolTipDuration (int msec) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlag (Qt::WindowType flag, bool on) |
void | setWindowFlags (Qt::WindowFlags type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (Qt::WindowStates windowState) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const const |
virtual QSize | sizeHint () const const |
QSize | sizeIncrement () const const |
QSizePolicy | sizePolicy () const const |
void | stackUnder (QWidget *w) |
QString | statusTip () const const |
QStyle * | style () const const |
QString | styleSheet () const const |
bool | testAttribute (Qt::WidgetAttribute attribute) const const |
QString | toolTip () const const |
int | toolTipDuration () const const |
QWidget * | topLevelWidget () const const |
bool | underMouse () const const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | update () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | updateGeometry () |
bool | updatesEnabled () const const |
QRegion | visibleRegion () const const |
QString | whatsThis () const const |
int | width () const const |
QWidget * | window () const const |
QString | windowFilePath () const const |
Qt::WindowFlags | windowFlags () const const |
QWindow * | windowHandle () const const |
QIcon | windowIcon () const const |
void | windowIconChanged (const QIcon &icon) |
QString | windowIconText () const const |
void | windowIconTextChanged (const QString &iconText) |
Qt::WindowModality | windowModality () const const |
qreal | windowOpacity () const const |
QString | windowRole () const const |
Qt::WindowStates | windowState () const const |
QString | windowTitle () const const |
void | windowTitleChanged (const QString &title) |
Qt::WindowType | windowType () const const |
WId | winId () const const |
int | x () const const |
int | y () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
int | colorCount () const const |
int | depth () const const |
qreal | devicePixelRatio () const const |
qreal | devicePixelRatioF () const const |
int | height () const const |
int | heightMM () const const |
int | logicalDpiX () const const |
int | logicalDpiY () const const |
bool | paintingActive () const const |
int | physicalDpiX () const const |
int | physicalDpiY () const const |
int | width () const const |
int | widthMM () const const |
Static Public Member Functions | |
static bool | dirOnlyMode (uint mode) |
![]() | |
QWidget * | createWindowContainer (QWindow *window, QWidget *parent, Qt::WindowFlags flags) |
QWidget * | find (WId id) |
QWidget * | keyboardGrabber () |
QWidget * | mouseGrabber () |
void | setTabOrder (QWidget *first, QWidget *second) |
void | setTabOrder (std::initializer_list< QWidget * > widgets) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Slots | |
void | highlightFile (const KFileItem &item) |
void | pathChanged () |
void | resetCursor () |
virtual void | selectDir (const KFileItem &item) |
void | selectFile (const KFileItem &item) |
void | slotCompletionMatch (const QString &match) |
void | sortByDate () |
void | sortByName () |
void | sortBySize () |
void | sortByType () |
void | sortReversed () |
void | toggleDirsFirst () |
void | toggleIgnoreCase () |
Protected Member Functions | |
virtual void | activatedMenu (const KFileItem &item, const QPoint &pos) |
void | changeEvent (QEvent *event) override |
bool | checkPreviewSupport () |
virtual QAbstractItemView * | createView (QWidget *parent, KFile::FileView viewKind) |
bool | eventFilter (QObject *watched, QEvent *event) override |
void | prepareCompletionObjects () |
void | resizeEvent (QResizeEvent *event) override |
virtual void | setDirLister (KDirLister *lister) |
void | setupActions () |
void | setupMenu () |
void | updateSortActions () |
void | updateViewActions () |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | closeEvent (QCloseEvent *event) |
virtual void | contextMenuEvent (QContextMenuEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual void | enterEvent (QEnterEvent *event) |
virtual bool | event (QEvent *event) override |
virtual void | focusInEvent (QFocusEvent *event) |
bool | focusNextChild () |
virtual bool | focusNextPrevChild (bool next) |
virtual void | focusOutEvent (QFocusEvent *event) |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | initPainter (QPainter *painter) const const override |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual void | keyPressEvent (QKeyEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | leaveEvent (QEvent *event) |
virtual int | metric (PaintDeviceMetric m) const const override |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
virtual void | mouseReleaseEvent (QMouseEvent *event) |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | nativeEvent (const QByteArray &eventType, void *message, qintptr *result) |
virtual void | paintEvent (QPaintEvent *event) |
virtual void | showEvent (QShowEvent *event) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus (Qt::InputMethodQuery query) |
virtual void | wheelEvent (QWheelEvent *event) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
This widget works as a network transparent filebrowser.
You specify a URL to display and this url will be loaded via KDirLister. The user can browse through directories, highlight and select files, delete or rename files.
It supports different views, e.g. a detailed view (see KFileDetailView), a simple icon view (see KFileIconView), a combination of two views, separating directories and files ( KCombiView).
Additionally, a preview view is available (see KFilePreview), which can show either a simple or detailed view and additionally a preview widget (see setPreviewWidget()). KImageFilePreview is one implementation of a preview widget, that displays previews for all supported filetypes utilizing KIO::PreviewJob.
Currently, those classes don't support Drag&Drop out of the box – there you have to use your own view-classes. You can use some DnD-aware views from Björn Sahlström bjorn.nosp@m.@kbe.nosp@m.ar.or.nosp@m.g until they will be integrated into this library. See http://devel-home.kde.org/~pfeiffer/DnD-classes.tar.gz
This widget is the one used in the KFileWidget.
Basic usage is like this:
This will create a childwidget of 'this' showing the directory contents of /home/gis in the default-view. The view is determined by the readConfig() call, which will read the KDirOperator settings, the user left your program with (and which you saved with op->writeConfig()).
A widget for displaying files and browsing directories.
Definition at line 90 of file kdiroperator.h.
Member Enumeration Documentation
◆ Action
enum KDirOperator::Action |
Actions provided by KDirOperator that can be accessed from the outside using action()
Enumerator | |
---|---|
PopupMenu | An ActionMenu presenting a popupmenu with all actions. |
Up | Changes to the parent directory. |
Back | Goes back to the previous directory. |
Forward | Goes forward in the history. |
Home | Changes to the user's home directory. |
Reload | Reloads the current directory. |
NewFolder | Opens a dialog box to create a directory. |
Delete | Deletes the selected files/directories. |
SortMenu | An ActionMenu containing all sort-options. |
SortByName | Sorts by name. |
SortBySize | Sorts by size. |
SortByDate | Sorts by date. |
SortByType | Sorts by type. |
SortAscending | Changes sort order to ascending. |
SortDescending | Changes sort order to descending. |
SortFoldersFirst | Sorts folders before files. |
SortHiddenFilesLast | Sorts hidden files last. |
ViewModeMenu | an ActionMenu containing all actions concerning the view |
ShortView | Shows a simple fileview. |
DetailedView | Shows a detailed fileview (dates, permissions ,...) |
ShowHiddenFiles | shows hidden files |
ShowPreviewPanel | shows a preview next to the fileview |
Properties | Shows a KPropertiesDialog for the selected files. |
Definition at line 109 of file kdiroperator.h.
◆ ActionType
The various action types.
These values can be or'd together
Definition at line 98 of file kdiroperator.h.
Constructor & Destructor Documentation
◆ KDirOperator()
Constructs the KDirOperator with no initial view.
As the views are configurable, call readConfig() to load the user's configuration and then setView to explicitly set a view.
This constructor doesn't start loading the url, setView will do it.
Definition at line 264 of file kdiroperator.cpp.
◆ ~KDirOperator()
|
override |
Destroys the KDirOperator.
Definition at line 327 of file kdiroperator.cpp.
Member Function Documentation
◆ action()
QAction * KDirOperator::action | ( | KDirOperator::Action | action | ) | const |
Obtain a given action from the KDirOperator's set of actions.
You can e.g. use
to add a button into a toolbar, which makes the dirOperator change to its parent directory.
- Since
- 5.100
Definition at line 492 of file kdiroperator.cpp.
◆ activatedMenu()
Called upon right-click to activate the popupmenu.
Definition at line 1188 of file kdiroperator.cpp.
◆ allActions()
A list of all actions for this KDirOperator.
See action()
- Since
- 5.100
Definition at line 497 of file kdiroperator.cpp.
◆ back
|
virtualslot |
Goes one step back in the history and opens that url.
Definition at line 1068 of file kdiroperator.cpp.
◆ cdUp
|
virtualslot |
Goes one directory up from the current url.
Definition at line 1113 of file kdiroperator.cpp.
◆ changeEvent()
|
overrideprotectedvirtual |
Reimplemented from QWidget.
Definition at line 1242 of file kdiroperator.cpp.
◆ checkPreviewSupport()
|
protected |
Checks if there support from KIO::PreviewJob for the currently shown files, taking mimeFilter() and nameFilter() into account Enables/disables the preview-action accordingly.
Definition at line 1174 of file kdiroperator.cpp.
◆ clearFilter()
void KDirOperator::clearFilter | ( | ) |
Clears both the namefilter and MIME type filter, so that all files and directories will be shown.
Call updateDir() to apply it.
- See also
- setMimeFilter
- setNameFilter
Definition at line 1130 of file kdiroperator.cpp.
◆ clearHistory()
void KDirOperator::clearHistory | ( | ) |
Clears the forward and backward history.
Definition at line 2563 of file kdiroperator.cpp.
◆ close()
void KDirOperator::close | ( | ) |
Stops loading immediately.
You don't need to call this, usually.
Definition at line 875 of file kdiroperator.cpp.
◆ completionObject()
KCompletion * KDirOperator::completionObject | ( | ) | const |
- Returns
- a KCompletion object, containing all filenames and directories of the current directory/URL. You can use it to insert it into a KLineEdit or KComboBox Note: it will only contain files, after prepareCompletionObjects() has been called. It will be implicitly called from makeCompletion() or makeDirCompletion()
Definition at line 482 of file kdiroperator.cpp.
◆ contextMenuAboutToShow
Emitted just before the context menu is shown, allows users to extend the menu with custom actions.
- Parameters
-
item the file on which the context menu was invoked menu the context menu, pre-populated with the file-management actions
◆ createView()
|
protectedvirtual |
A view factory for creating predefined fileviews.
Called internally by setView, but you can also call it directly. Reimplement this if you depend on self defined fileviews.
- Parameters
-
parent is the QWidget to be set as parent viewKind is the predefined view to be set, note: this can be several ones OR:ed together
- Returns
- the created view
- See also
- KFile::FileView
- setView
Definition at line 1474 of file kdiroperator.cpp.
◆ currentIconSizeChanged
|
signal |
Will notify that the icon size has changed.
Since we save the icon size depending on the view type (list view or a different kind of view), a call to setView() can trigger this signal to be emitted.
◆ decorationPosition()
QStyleOptionViewItem::Position KDirOperator::decorationPosition | ( | ) | const |
Returns the position where icons are shown relative to the labels of file items in the icon view.
- Since
- 4.2.3
Definition at line 2878 of file kdiroperator.cpp.
◆ del()
|
virtual |
Starts and returns a KIO::DeleteJob to delete the given items
.
- Parameters
-
items the list of items to be deleted parent the parent widget used for the confirmation dialog ask specifies whether a confirmation dialog should be shown showProgress passed to the DeleteJob to show a progress dialog
Definition at line 709 of file kdiroperator.cpp.
◆ deleteSelected
|
virtualslot |
Deletes the currently selected files/directories.
Definition at line 740 of file kdiroperator.cpp.
◆ dirCompletionObject()
KCompletion * KDirOperator::dirCompletionObject | ( | ) | const |
- Returns
- a KCompletion object, containing only all directories of the current directory/URL. You can use it to insert it into a KLineEdit or KComboBox Note: it will only contain directories, after prepareCompletionObjects() has been called. It will be implicitly called from makeCompletion() or makeDirCompletion()
Definition at line 487 of file kdiroperator.cpp.
◆ dirHighlighting()
bool KDirOperator::dirHighlighting | ( | ) | const |
- Returns
- whether the last directory will be made the current item (and hence highlighted) when going up or back in the directory hierarchy
Directories are highlighted by default.
Definition at line 2579 of file kdiroperator.cpp.
◆ dirLister()
KDirLister * KDirOperator::dirLister | ( | ) | const |
- Returns
- the object listing the directory
Definition at line 357 of file kdiroperator.cpp.
◆ dirOnlyMode() [1/2]
bool KDirOperator::dirOnlyMode | ( | ) | const |
- Returns
- true if we are in directory-only mode, that is, no files are shown.
Definition at line 2584 of file kdiroperator.cpp.
◆ dirOnlyMode() [2/2]
|
static |
Definition at line 2589 of file kdiroperator.cpp.
◆ dropped
|
signal |
Emitted when files are dropped.
Dropping files is disabled by default. You need to enable it with setAcceptDrops()
- Parameters
-
item the item on which the drop occurred or 0. event the drop event itself. urls the urls that where dropped.
◆ eventFilter()
Reimplemented from QObject.
Definition at line 1247 of file kdiroperator.cpp.
◆ fileHighlighted
|
signal |
Emitted when a file is highlighted or generally the selection changes in multiselection mode.
In the latter case, item
is a null KFileItem. You can access the selected items with selectedItems().
◆ followNewDirectories()
bool KDirOperator::followNewDirectories | ( | ) | const |
- Returns
- true if setUrl is called on newly created directories, false otherwise. Enabled by default.
- Since
- 5.62
- See also
- setFollowNewDirectories
Definition at line 2500 of file kdiroperator.cpp.
◆ followSelectedDirectories()
bool KDirOperator::followSelectedDirectories | ( | ) | const |
- Returns
- whether setUrl is called on selected directories when a tree view is used. Enabled by default.
- Since
- 5.62
Definition at line 2510 of file kdiroperator.cpp.
◆ forward
|
virtualslot |
Goes one step forward in the history and opens that url.
Definition at line 1095 of file kdiroperator.cpp.
◆ highlightFile
|
protectedslot |
Emits fileHighlighted(item)
Definition at line 1780 of file kdiroperator.cpp.
◆ home
|
virtualslot |
Enters the home directory.
Definition at line 1125 of file kdiroperator.cpp.
◆ iconSize()
int KDirOperator::iconSize | ( | ) | const |
Returns the icon size in pixels, ranged from KIconLoader::SizeSmall (16) to KIconLoader::SizeEnormous (128).
- Since
- 5.76
Definition at line 795 of file kdiroperator.cpp.
◆ isInlinePreviewShown()
bool KDirOperator::isInlinePreviewShown | ( | ) | const |
Returns whether the inline previews are shown or not.
Definition at line 790 of file kdiroperator.cpp.
◆ isRoot()
bool KDirOperator::isRoot | ( | ) | const |
- Returns
- true if we are displaying the root directory of the current url
Definition at line 343 of file kdiroperator.cpp.
◆ isSaving()
bool KDirOperator::isSaving | ( | ) | const |
Returns whether KDirOperator will force a double click to accept.
- Note
- this is false by default
Definition at line 805 of file kdiroperator.cpp.
◆ isSelected()
bool KDirOperator::isSelected | ( | const KFileItem & | item | ) | const |
- Returns
- true if
item
is currently selected, or false otherwise.
Definition at line 461 of file kdiroperator.cpp.
◆ keyEnterReturnPressed
|
signal |
Triggered when the user hit Enter/Return.
- Since
- 5.57
◆ makeCompletion
Tries to complete the given string (only completes files).
Definition at line 1878 of file kdiroperator.cpp.
◆ makeDirCompletion
Tries to complete the given string (only completes directories).
Definition at line 1889 of file kdiroperator.cpp.
◆ mimeFilter()
QStringList KDirOperator::mimeFilter | ( | ) | const |
- Returns
- the current MIME type filter.
Definition at line 1154 of file kdiroperator.cpp.
◆ mkdir
|
virtualslot |
Opens a dialog to create a new directory.
Definition at line 703 of file kdiroperator.cpp.
◆ mode()
KFile::Modes KDirOperator::mode | ( | ) | const |
- Returns
- the listing/selection mode.
Definition at line 1552 of file kdiroperator.cpp.
◆ nameFilter()
QString KDirOperator::nameFilter | ( | ) | const |
- Returns
- the current namefilter.
- See also
- setNameFilter
Definition at line 1143 of file kdiroperator.cpp.
◆ newFileMenuSupportedMimeTypes()
QStringList KDirOperator::newFileMenuSupportedMimeTypes | ( | ) | const |
- Returns
- the current Supported Mimes Types.
Definition at line 1164 of file kdiroperator.cpp.
◆ numDirs()
int KDirOperator::numDirs | ( | ) | const |
- Returns
- the number of directories in the currently listed url. Returns 0 if there is no view.
Definition at line 472 of file kdiroperator.cpp.
◆ numFiles()
int KDirOperator::numFiles | ( | ) | const |
- Returns
- the number of files in the currently listed url. Returns 0 if there is no view.
Definition at line 477 of file kdiroperator.cpp.
◆ onlyDoubleClickSelectsFiles()
bool KDirOperator::onlyDoubleClickSelectsFiles | ( | ) | const |
- Returns
- whether files (not directories) should only be select()ed by double-clicks.
- See also
- setOnlyDoubleClickSelectsFiles
Definition at line 2490 of file kdiroperator.cpp.
◆ pathChanged
|
protectedslot |
Called after setUrl() to load the directory, update the history, etc.
Definition at line 1028 of file kdiroperator.cpp.
◆ prepareCompletionObjects()
|
protected |
Synchronizes the completion objects with the entries of the currently listed url.
Automatically called from makeCompletion() and makeDirCompletion()
Definition at line 1900 of file kdiroperator.cpp.
◆ previewGenerator()
KFilePreviewGenerator * KDirOperator::previewGenerator | ( | ) | const |
Returns the preview generator for the current view.
Definition at line 780 of file kdiroperator.cpp.
◆ progressBar()
QProgressBar * KDirOperator::progressBar | ( | ) | const |
- Returns
- the progress widget, that is shown during directory listing. You can for example reparent() it to put it into a statusbar.
Definition at line 2558 of file kdiroperator.cpp.
◆ readConfig()
|
virtual |
Reads the default settings for a view, i.e. the default KFile::FileView.
Also reads the sorting and whether hidden files should be shown. Note: the default view will not be set - you have to call
to apply it.
- See also
- setView
- setViewConfig
- writeConfig
Definition at line 2314 of file kdiroperator.cpp.
◆ renameSelected
|
slot |
Initiates a rename operation on the currently selected files/directories, prompting the user to choose a new name(s) for the currently selected items.
- See also
- renamingFinished
- Since
- 5.67
Definition at line 810 of file kdiroperator.cpp.
◆ renamingFinished
Emitted when renaming selected files has finished.
- Parameters
-
urls URL list of the renamed files
- Since
- 5.96
◆ rereadDir
|
virtualslot |
Re-reads the current url.
Definition at line 955 of file kdiroperator.cpp.
◆ resetCursor
|
protectedslot |
Restores the normal cursor after showing the busy-cursor.
Also hides the progressbar.
Definition at line 362 of file kdiroperator.cpp.
◆ resizeEvent()
|
overrideprotectedvirtual |
Reimplemented from QWidget.
Definition at line 2453 of file kdiroperator.cpp.
◆ selectDir
|
protectedvirtualslot |
Enters the directory specified by the given item
.
Definition at line 1768 of file kdiroperator.cpp.
◆ selectedItems()
KFileItemList KDirOperator::selectedItems | ( | ) | const |
- Returns
- a list of all currently selected items. If there is no view, or there are no selected items, an empty list is returned.
Definition at line 441 of file kdiroperator.cpp.
◆ selectFile
|
protectedslot |
Emits fileSelected( item )
Definition at line 1773 of file kdiroperator.cpp.
◆ setAcceptDrops()
|
virtual |
Reimplemented - allow dropping of files if b
is true, defaults to true since 5.59.
- Parameters
-
b true if the widget should allow dropping of files
Definition at line 1488 of file kdiroperator.cpp.
◆ setCurrentItem() [1/2]
void KDirOperator::setCurrentItem | ( | const KFileItem & | item | ) |
Clears the current selection and attempts to set item
as the current item.
Definition at line 1807 of file kdiroperator.cpp.
◆ setCurrentItem() [2/2]
void KDirOperator::setCurrentItem | ( | const QUrl & | url | ) |
Clears the current selection and attempts to set url
the current url file.
Definition at line 1789 of file kdiroperator.cpp.
◆ setCurrentItems() [1/2]
void KDirOperator::setCurrentItems | ( | const KFileItemList & | items | ) |
Clears the current selection and attempts to set items
as the current items.
Definition at line 1853 of file kdiroperator.cpp.
◆ setCurrentItems() [2/2]
Clears the current selection and attempts to set urls
the current url files.
Definition at line 1826 of file kdiroperator.cpp.
◆ setDecorationPosition()
void KDirOperator::setDecorationPosition | ( | QStyleOptionViewItem::Position | position | ) |
Sets the position where icons shall be shown relative to the labels of file items in the icon view.
- Since
- 4.2.3
Definition at line 2883 of file kdiroperator.cpp.
◆ setDirLister()
|
protectedvirtual |
Sets a custom KDirLister to list directories.
The KDirOperator takes ownership of the given KDirLister.
Definition at line 1702 of file kdiroperator.cpp.
◆ setDropOptions()
|
virtual |
Sets the options for dropping files.
CURRENTLY NOT IMPLEMENTED
Definition at line 1501 of file kdiroperator.cpp.
◆ setEnableDirHighlighting()
|
virtual |
When using the up or back actions to navigate the directory hierarchy, KDirOperator can highlight the directory that was just left.
For example:
- starting in /a/b/c/, going up to /a/b, "c" will be highlighted
- starting in /a/b/c, going up (twice) to /a, "b" will be highlighted; using the back action to go to /a/b/, "c" will be highlighted
- starting in /a, going to "b", then going to "c", using the back action to go to /a/b/, "c" will be highlighted; using the back action again to go to /a/, "b" will be highlighted
- See also
- dirHighlighting. The default is to highlight directories when going back/up.
Definition at line 2574 of file kdiroperator.cpp.
◆ setFollowNewDirectories()
void KDirOperator::setFollowNewDirectories | ( | bool | enable | ) |
Toggles whether setUrl is called on newly created directories.
- Since
- 5.62
Definition at line 2495 of file kdiroperator.cpp.
◆ setFollowSelectedDirectories()
void KDirOperator::setFollowSelectedDirectories | ( | bool | enable | ) |
Toggles whether setUrl is called on selected directories when a tree view is used.
- Since
- 5.62
Definition at line 2505 of file kdiroperator.cpp.
◆ setIconSize
|
slot |
Notifies that the icons size should change.
value
is the icon size in pixels, ranged from KIconLoader::SizeSmall (16) to KIconLoader::SizeEnormous (128).
- Since
- 5.76
Definition at line 852 of file kdiroperator.cpp.
◆ setInlinePreviewShown()
void KDirOperator::setInlinePreviewShown | ( | bool | show | ) |
Forces the inline previews to be shown or hidden, depending on show
.
- Parameters
-
show Whether to show inline previews or not.
Definition at line 785 of file kdiroperator.cpp.
◆ setIsSaving()
void KDirOperator::setIsSaving | ( | bool | isSaving | ) |
If the system is set up to trigger items on single click, if isSaving
is true, we will force to double click to accept.
- Note
- this is false by default
Definition at line 800 of file kdiroperator.cpp.
◆ setMimeFilter()
void KDirOperator::setMimeFilter | ( | const QStringList & | mimetypes | ) |
Sets a list of MIME types as filter.
Only files of those MIME types will be shown.
Example:
Node: Without the MIME type inode/directory, only files would be shown. Call updateDir() to apply it.
- See also
- KDirLister::setMimeFilter
- mimeFilter
Definition at line 1148 of file kdiroperator.cpp.
◆ setMode()
|
virtual |
Sets the listing/selection mode for the views, an OR'ed combination of.
- File
- Directory
- Files
- ExistingOnly
- LocalOnly
You cannot mix File and Files of course, as the former means single-selection mode, the latter multi-selection.
Definition at line 1557 of file kdiroperator.cpp.
◆ setNameFilter()
void KDirOperator::setNameFilter | ( | const QString & | filter | ) |
Sets a filter like "*.cpp *.h *.o".
Only files matching that filter will be shown.
- See also
- KDirLister::setNameFilter
- nameFilter
Definition at line 1137 of file kdiroperator.cpp.
◆ setNewFileMenuSelectDirWhenAlreadyExist()
void KDirOperator::setNewFileMenuSelectDirWhenAlreadyExist | ( | bool | selectOnDirExists | ) |
Setting this to true will make a directory get selected when trying to create a new one that has the same name.
- Since
- 5.76
Definition at line 1169 of file kdiroperator.cpp.
◆ setNewFileMenuSupportedMimeTypes()
void KDirOperator::setNewFileMenuSupportedMimeTypes | ( | const QStringList & | mime | ) |
Only show the files in a given set of MIME types.
This is useful in specialized applications (while file managers, on the other hand, want to show all MIME types). Internally uses KNewFileMenu::setSupportedMimeTypes
Example:
Note: If the list is empty, all options will be shown. Otherwise, without the MIME type inode/directory, only file options will be shown.
Definition at line 1159 of file kdiroperator.cpp.
◆ setOnlyDoubleClickSelectsFiles()
void KDirOperator::setOnlyDoubleClickSelectsFiles | ( | bool | enable | ) |
This toggles between double/single click file and directory selection mode.
When argument is true, files and directories are highlighted with single click and selected (executed) with double click.
NOTE: this currently has no effect.
The default follows the single/double click system setting.
Definition at line 2481 of file kdiroperator.cpp.
◆ setPreviewWidget()
|
virtual |
Sets a preview-widget to be shown next to the file-view.
The ownership of w
is transferred to KDirOperator, so don't delete it yourself!
Definition at line 419 of file kdiroperator.cpp.
◆ setShowHiddenFiles()
|
virtual |
Enables/disables showing hidden files.
Definition at line 2868 of file kdiroperator.cpp.
◆ setSorting()
void KDirOperator::setSorting | ( | QDir::SortFlags | spec | ) |
Sets the way to sort files and directories.
Definition at line 333 of file kdiroperator.cpp.
◆ setSupportedSchemes
|
slot |
Set the URL schemes that the file widget should allow navigating to.
If the returned list is empty, all schemes are supported. Examples for schemes are "file"
or "ftp"
.
- See also
- QFileDialog::setSupportedSchemes
- Since
- 5.43
Definition at line 2911 of file kdiroperator.cpp.
◆ setupActions()
|
protected |
Sets up all the actions.
Called from the constructor, you usually better not call this.
Definition at line 1928 of file kdiroperator.cpp.
◆ setupMenu() [1/2]
|
protected |
Sets up the context-menu with all the necessary actions.
Called from the constructor, you usually don't need to call this.
Definition at line 2201 of file kdiroperator.cpp.
◆ setupMenu() [2/2]
void KDirOperator::setupMenu | ( | int | whichActions | ) |
Sets up the action menu.
- Parameters
-
whichActions is an value of OR'd ActionTypes that controls which actions to show in the action menu
Definition at line 2206 of file kdiroperator.cpp.
◆ setUrl()
|
virtual |
Sets a new url to list.
- Parameters
-
clearforward specifies whether the "forward" history should be cleared. url the URL to set
Definition at line 885 of file kdiroperator.cpp.
◆ setViewConfig()
|
virtual |
Sets the config object and the to be used group in KDirOperator.
This will be used to store the view's configuration. If you don't set this, the views cannot save and restore their configuration.
Usually you call this right after KDirOperator creation so that the view instantiation can make use of it already.
Note that KDirOperator does NOT take ownership of that object (typically it's KSharedConfig::openConfig() anyway.
You must not delete the KConfig or KConfigGroup object (and master config object) before either deleting the KDirOperator or calling setViewConfig(0); or something like that
- See also
- viewConfig
- viewConfigGroup
Definition at line 2857 of file kdiroperator.cpp.
◆ setViewMode()
void KDirOperator::setViewMode | ( | KFile::FileView | viewKind | ) |
Set the view mode to one of the predefined modes.
- See also
- KFile::FileView
- Since
- 5.100
Definition at line 1509 of file kdiroperator.cpp.
◆ showHiddenFiles()
bool KDirOperator::showHiddenFiles | ( | ) | const |
- Returns
- true when hidden files are shown or false otherwise.
Definition at line 2873 of file kdiroperator.cpp.
◆ showOpenWithActions()
void KDirOperator::showOpenWithActions | ( | bool | enable | ) |
Call with true
to add open-with actions to items in the view.
This can be useful when you're attaching an image or text file to an email or uploading an image to some online service, and need to check the contents before going forward.
- Since
- 5.87
Definition at line 1237 of file kdiroperator.cpp.
◆ slotCompletionMatch
|
protectedslot |
Tries to make the given match
as current item in the view and emits completion( match )
Definition at line 1918 of file kdiroperator.cpp.
◆ sortByDate
|
protectedslot |
Changes sorting to sort by date.
Definition at line 380 of file kdiroperator.cpp.
◆ sortByName
|
protectedslot |
Changes sorting to sort by name.
Definition at line 370 of file kdiroperator.cpp.
◆ sortBySize
|
protectedslot |
Changes sorting to sort by size.
Definition at line 375 of file kdiroperator.cpp.
◆ sortByType
|
protectedslot |
Changes sorting to sort by date.
Definition at line 385 of file kdiroperator.cpp.
◆ sorting()
QDir::SortFlags KDirOperator::sorting | ( | ) | const |
- Returns
- the current way of sorting files and directories
Definition at line 338 of file kdiroperator.cpp.
◆ sortReversed
|
protectedslot |
Changes sorting to reverse sorting.
Definition at line 390 of file kdiroperator.cpp.
◆ supportedSchemes()
QStringList KDirOperator::supportedSchemes | ( | ) | const |
Returns the URL schemes that the file widget should allow navigating to.
If the returned list is empty, all schemes are supported.
- See also
- QFileDialog::supportedSchemes
- Since
- 5.43
Definition at line 2917 of file kdiroperator.cpp.
◆ toggleDirsFirst
|
protectedslot |
Toggles showing directories first / having them sorted like files.
Definition at line 396 of file kdiroperator.cpp.
◆ toggleIgnoreCase
|
protectedslot |
Toggles case sensitive / case insensitive sorting.
Definition at line 401 of file kdiroperator.cpp.
◆ trash()
|
virtual |
Starts and returns a KIO::CopyJob to trash the given items
.
- Parameters
-
items the list of items to be trashed parent the parent widget used for the confirmation dialog ask specifies whether a confirmation dialog should be shown showProgress passed to the CopyJob to show a progress dialog
Definition at line 753 of file kdiroperator.cpp.
◆ trashSelected
|
virtualslot |
Trashes the currently selected files/directories.
This function used to take activation reason and keyboard modifiers, in order to call deleteSelected() if the user wanted to delete. Instead, call deleteSelected().
FIXME KAction Port: link deleteSelected() up correctly
Definition at line 830 of file kdiroperator.cpp.
◆ updateDir
|
slot |
to update the view after changing the settings
Definition at line 948 of file kdiroperator.cpp.
◆ updateSelectionDependentActions
|
slot |
Enables/disables actions that are selection dependent.
Call this e.g. when you are about to show a popup menu using some of KDirOperators actions.
Definition at line 410 of file kdiroperator.cpp.
◆ updateSortActions()
|
protected |
Updates the sorting-related actions to comply with the current sorting.
- See also
- sorting
Definition at line 2268 of file kdiroperator.cpp.
◆ updateViewActions()
|
protected |
Updates the view-related actions to comply with the current KFile::FileView.
Definition at line 2295 of file kdiroperator.cpp.
◆ url()
QUrl KDirOperator::url | ( | ) | const |
- Returns
- the current url
Definition at line 1108 of file kdiroperator.cpp.
◆ view()
QAbstractItemView * KDirOperator::view | ( | ) | const |
◆ viewChanged
|
signal |
Emitted whenever the current fileview is changed, either by an explicit call to setView() or by the user selecting a different view thru the GUI.
◆ viewConfigGroup()
KConfigGroup * KDirOperator::viewConfigGroup | ( | ) | const |
- Returns
- the group set by setViewConfig configuration.
Definition at line 2863 of file kdiroperator.cpp.
◆ viewMode()
KFile::FileView KDirOperator::viewMode | ( | ) | const |
Returns the current view mode.
- Returns
- KFile::FileView
- See also
- KFile::FileView
- Since
- 5.0
Definition at line 1542 of file kdiroperator.cpp.
◆ writeConfig()
|
virtual |
Saves the current settings like sorting, simple or detailed view.
- See also
- readConfig
- setViewConfig
Definition at line 2377 of file kdiroperator.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Tue Mar 26 2024 11:18:52 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.